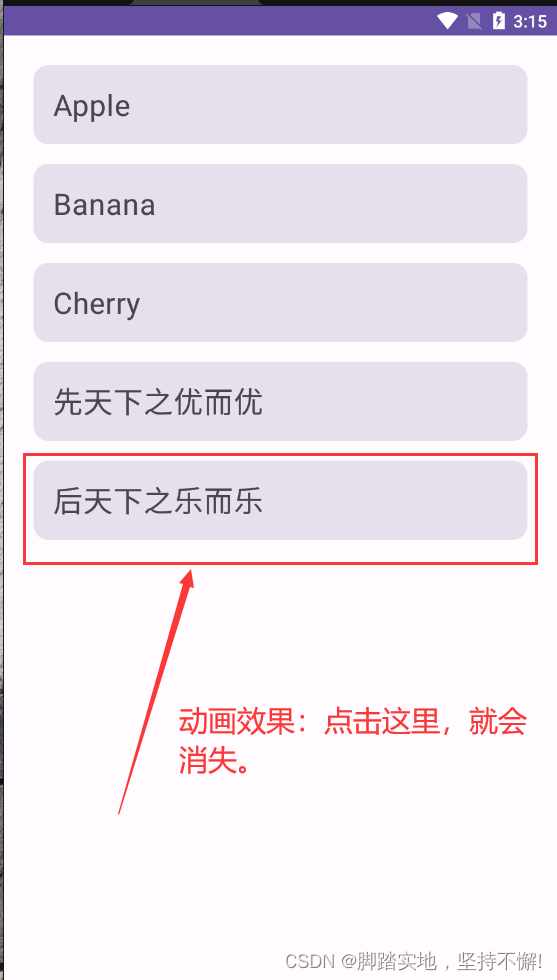
android,Compose,消息列表和动画(点击item的时候,就会删除)
Compose,消息列表和动画(点击item的时候,就会删除)
·
Compose,消息列表和动画(点击item的时候,就会删除)
package com.example.mycompose08
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.tooling.preview.Preview
import com.example.mycompose08.ui.theme.MyApplicationTheme
import androidx.compose.animation.*
import androidx.compose.foundation.clickable
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.lazy.*
import androidx.compose.material3.*
import androidx.compose.material3.Card
import androidx.compose.runtime.*
import androidx.compose.ui.*
import androidx.compose.ui.unit.*
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
// A surface container using the 'background' color from the theme
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
AnimatedList()
}
}
}
}
}
// 定义一个可组合函数,用于显示一个列表
@Composable
fun AnimatedList() {
// 创建一个状态变量,用于存储列表的数据
val list = remember { mutableStateListOf("Apple", "Banana", "Cherry","先天下之优而优","后天下之乐而乐") }
// 创建一个懒加载列表,用于显示列表的每一项
LazyColumn(
modifier = Modifier.fillMaxSize(),
contentPadding = PaddingValues(16.dp)
) {
// 遍历列表的数据,为每一项创建一个可组合函数
items(list) { item ->
// 使用AnimatedVisibility函数,根据列表项是否可见来添加淡入淡出的动画效果
AnimatedVisibility(
visible = list.contains(item),
enter = fadeIn(),
exit = fadeOut()
) {
// 使用Card函数,创建一个卡片样式的列表项
Card(
modifier = Modifier
.fillMaxWidth()
.padding(8.dp)
.clickable {
// 点击卡片时,从列表中移除该项
list.remove(item)
},
//elevation = 4.dp
// shadowElevation = 4.dp
) {
// 在卡片中显示列表项的文本内容
Text(
text = item,
modifier = Modifier.padding(16.dp),
fontSize = 24.sp
)
}
}
}
}
}
@Preview(showBackground = true)
@Composable
fun GreetingPreview() {
MyApplicationTheme {
AnimatedList()
}
}
案例二:
package com.example.mycompose09 // 定义包名
import android.os.Bundle // 导入Bundle类
import androidx.activity.ComponentActivity // 导入ComponentActivity类
import androidx.activity.compose.setContent // 导入setContent函数
import androidx.compose.foundation.Image // 导入Image可组合项
import androidx.compose.foundation.border // 导入border修饰符
import androidx.compose.foundation.clickable // 导入clickable修饰符
import androidx.compose.foundation.layout.Column // 导入Column可组合项
import androidx.compose.foundation.layout.Row // 导入Row可组合项
import androidx.compose.foundation.layout.Spacer // 导入Spacer可组合项
import androidx.compose.foundation.layout.fillMaxSize // 导入fillMaxSize修饰符
import androidx.compose.foundation.layout.height // 导入height修饰符
import androidx.compose.foundation.layout.padding // 导入padding修饰符
import androidx.compose.foundation.layout.size // 导入size修饰符
import androidx.compose.foundation.layout.width // 导入width修饰符
import androidx.compose.foundation.lazy.LazyColumn // 导入LazyColumn可组合项
import androidx.compose.foundation.shape.CircleShape // 导入CircleShape形状
import androidx.compose.material3.MaterialTheme // 导入MaterialTheme主题
import androidx.compose.material3.Surface // 导入Surface可组合项
import androidx.compose.material3.Text // 导入Text可组合项
import androidx.compose.runtime.Composable // 导入Composable注解
import androidx.compose.runtime.mutableStateOf // 导入mutableStateOf函数
import androidx.compose.runtime.remember // 导入remember函数
import androidx.compose.ui.Modifier // 导入Modifier类
import androidx.compose.ui.draw.clip // 导入clip修饰符
import androidx.compose.ui.res.painterResource // 导入painterResource函数
import androidx.compose.ui.tooling.preview.Preview // 导入Preview注解
import androidx.compose.ui.unit.dp // 导入dp单位
import com.example.mycompose09.ui.theme.MyApplicationTheme // 导入自定义主题
// 下面是导入的其他库,用于实现更多功能
import androidx.compose.runtime.setValue
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.lazy.items
import androidx.compose.material.*
import androidx.compose.runtime.getValue
import androidx.compose.runtime.mutableStateListOf
import androidx.compose.ui.*
import androidx.compose.ui.unit.*
class MainActivity : ComponentActivity() {// 定义MainActivity类,继承自ComponentActivity类
override fun onCreate(savedInstanceState: Bundle?) { // 重写onCreate方法,接收一个Bundle对象作为参数
super.onCreate(savedInstanceState) // 调用父类的onCreate方法,并传递参数
setContent { // 调用setContent函数,设置界面内容为一个可组合函数的lambda表达式
MyApplicationTheme { // 调用自定义主题函数,应用主题样式到界面内容中
// A surface container using the 'background' color from the theme
Surface(
// 设置Surface的修饰符为填充最大尺寸,即占满整个屏幕空间
modifier = Modifier.fillMaxSize(),
// 设置Surface的颜色为主题中定义的背景色
color = MaterialTheme.colorScheme.background
) {
// Call the Conversation function here and pass a list of messages as a parameter.
Conversation(messages = listOf( // 调用Conversation函数,并传递一个Message列表作为参数,显示一个对话界面。
Message("Alice", "Hello, how are you?", R.drawable.p62, true),
Message("Bob", "I'm fine, thank you. And you?", R.drawable.p19, false),
Message("Alice", "I'm good too. What are you doing?", R.drawable.p2, true),
Message("Bob", "Just learning Jetpack Compose. It's awesome!",R.drawable.p82,false)
))
}
}
}
}
}
// 定义一个数据类,表示一条消息,包含两个属性:作者和内容
data class Message(val author:String,val body:String,val imageId: Int, val isSent: Boolean)
@Composable
fun MessageCard(msg: Message) {// 定义一个MessageCard函数,接收一个Message对象作为参数,用于显示一条消息的卡片
// 创建一个状态变量,用于存储列表的数据
val list = remember {
// 调用remember函数,创建一个可变状态列表,并初始化为三个字符串
mutableStateListOf("Apple", "Banana", "Cherry")
}
Row(modifier = Modifier.padding(all = 8.dp)) {// 调用Row函数,创建一个水平排列的容器,并设置修饰符为8dp的内边距
Image(// 调用Image函数,创建一个图片组件
painter = painterResource(msg.imageId),
contentDescription = null,
modifier = Modifier
.size(40.dp)
.clip(CircleShape)
.border(1.5.dp, MaterialTheme.colorScheme.secondary, CircleShape)
)
Spacer(modifier = Modifier.width(8.dp))
var isExpanded by remember { mutableStateOf(false) }
// We toggle the isExpanded variable when we click on this Column
Column(modifier = Modifier.clickable { isExpanded = !isExpanded }) {
Text(
text = msg.author,
color = MaterialTheme.colorScheme.secondary,
style = MaterialTheme.typography.labelSmall
)
Spacer(modifier = Modifier.height(4.dp))
Surface(
shape = MaterialTheme.shapes.medium,
) {
Text(
text = msg.body,
modifier = Modifier.padding(all = 4.dp),
// If the message is expanded, we display all its content
// otherwise we only display the first line
maxLines = if (isExpanded) Int.MAX_VALUE else 1,
style = MaterialTheme.typography.bodyMedium
)
}
}
}
}
@Composable
fun Conversation(messages: List<Message>) {
LazyColumn {
items(messages){
// Call the MessageCard function here and pass each message as a parameter.
MessageCard(msg = it)
}
}
}
@Preview(showBackground = true)
@Composable
fun ConversationPreview() {
val messages = listOf(
Message("Alice", "Hello, how are you?", R.drawable.p62, true),
Message("Bob", "I'm fine, thank you. And you?", R.drawable.p19, false),
Message("Alice", "I'm good too. What are you doing?", R.drawable.p2, true),
Message("Bob", "Just learning Jetpack Compose. It's awesome!",R.drawable.p82,false)
)
Conversation(messages = messages)
}
更多推荐
所有评论(0)