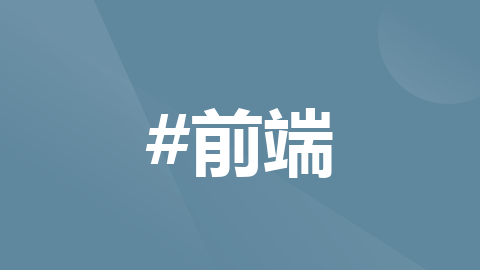
uniapp上传文件,预览,下载
例如:以上就是今天要讲的内容,本文仅仅简单介绍了uniapp附件的使用
·
uniapp上传文件,预览,下载
这是封装的上传文件的方法
export const fileUploadRequest = (options) => {
return new Promise((resolve, reject) => {
let token = ''
uni.getStorage({
key: 'token',
success: function(result) {
token = result.data
},
complete: function(result) {
uni.uploadFile({
url: BASE_URL + options.url,
header: {
'token': token,
},
filePath: options.filePath, // 本地文件资源
name: 'file',
formData: options.formData,
success: (res) => {
if (res.statusCode === 200) {
let _res = JSON.parse(res.data)
if (_res.code === 0) {
resolve(_res);
} else if (_res.code === 401) {
uni.showModal({
title: '提示!',
content: '当前登录已失效,请重新登录!',
confirmText: '立即登录',
cancelText: '返回首页',
success(res) {
if (res.confirm) {
uni.navigateTo({
url: '/pages/Login/login/login'
})
} else if (res.cancel) {
uni.switchTab({
url: '/pages/Patient-list/patient-list/patient-list'
});
}
}
});
} else {
uni.showToast({
title: _res.msg,
icon: 'none',
duration: 3000
});
resolve(res);
}
} else {
uni.showToast({
title: '未知异常,请联系管理员!',
icon: 'none',
duration: 3000
});
resolve(res);
}
},
fail: (err) => {
uni.showToast({
title: '请求接口失败',
duration: 3000
})
reject(err);
}
})
}
})
})
}
上传
async fileUpload(tempFilePath) {
const res = await this.$fileUploadRequest({
url: '/expand/rhTherapyRecordExpand/fileUpload',
filePath: tempFilePath,
formData: {
rhPatientId: this.list.rhPatientId,
patientName: this.list.patientName,
moduleClass: '7', //业务类别:1:治疗记录,2:康复评估 见公共字典 ,
subjectId: this.timeVal
}
});
if (res.code === 0) {
this.fileList.push(res.data);
this.fileList.forEach(item => (item.isShowFileVisible = false));
}
},
预览
showFileBtn(item, index) {
// 1-图片 2-视频 3-文档 4-音频
if (item.fileType === '1') {
uni.previewImage({
urls: [this.$BASE_URL + '/expand/rhTherapyRecordExpand/getShowFile?therapyRecordExpandId=' + item.therapyRecordExpandId]
});
} else if (item.fileType === '2') {
this.showVideo = true;
this.videoSrc = this.$BASE_URL + '/expand/rhTherapyRecordExpand/getShowFile?therapyRecordExpandId=' + item.therapyRecordExpandId;
} else if (item.fileType === '3') {
uni.downloadFile({
url: this.$BASE_URL + '/expand/rhTherapyRecordExpand/getShowFile?therapyRecordExpandId=' + item.therapyRecordExpandId,
success: function(res) {
var filePath = res.tempFilePath;
uni.openDocument({
filePath: filePath,
fail: function() {
uni.showToast({
title: '文件预览失败',
icon: 'none',
duration: 3000
});
}
});
}
});
} else {
this.$set(this.fileList[index], 'recordPopupShow', true);
this.$forceUpdate();
this.recordShowControl = true;
//这里是mp3播放
this.music = uni.createInnerAudioContext();
this.music.src = this.$BASE_URL + '/expand/rhTherapyRecordExpand/getShowFile?therapyRecordExpandId=' + item.therapyRecordExpandId;
this.music.play();
this.music.onEnded(() => {
// this.music = null;
this.recordShowControl = false;
this.$forceUpdate();
});
}
},
下载
Download(item) {
uni.downloadFile({
url: this.$BASE_URL + '/expand/rhTherapyRecordExpand/fileDownload?therapyRecordExpandId=' + item.therapyRecordExpandId,
success: data => {
if (data.statusCode === 200) {
if (item.fileType === '1') {
uni.saveImageToPhotosAlbum({
filePath: data.tempFilePath,
success: function(res) {
uni.showToast({
title: '已下载至相册'
});
},
fail: function() {
uni.showToast({
title: '下载失败'
});
}
});
} else if (item.fileType === '2') {
uni.saveVideoToPhotosAlbum({
filePath: data.tempFilePath,
success: function(res) {
uni.showToast({
title: '已下载至相册'
});
},
fail: function() {
uni.showToast({
title: '下载失败'
});
}
});
} else {
//这里是mp3下载到安卓文件管理文件里的内存地址文件里
let task = plus.downloader.createDownload(
this.$BASE_URL + '/expand/rhTherapyRecordExpand/fileDownload?therapyRecordExpandId=' + item.therapyRecordExpandId,
{
filename: 'file://storage/emulated/0/Download/' + item.filename
},
function(d, status) {
uni.hideLoading();
if (status === 200) {
uni.showToast({
icon: 'none',
title: '下载成功'
});
let fileSaveUrl = plus.io.convertLocalFileSystemURL(d.filename);
plus.runtime.openFile(d.filename);
} else {
uni.showToast({
icon: 'none',
title: '下载失败成功'
});
plus.downloader.clear();
}
}
);
uni.showLoading({
title: '文件下载中...'
});
task.start();
// console.log(data.tempFilePath)
// uni.saveFile({
// tempFilePath: data.tempFilePath,
// success: function(_res) {
// var savedFilePath = _res.savedFilePath;
// uni.showToast({
// title: '下载成功,请在本地资源查看!',
// icon: 'none',
// duration: 3000
// });
// }
// });
}
}
}
});
总结
提示:这里对文章进行总结:
例如:以上就是今天要讲的内容,本文仅仅简单介绍了pandas的使用,而pandas提供了大量能使我们快速便捷地处理数据的函数和方法。
更多推荐
所有评论(0)