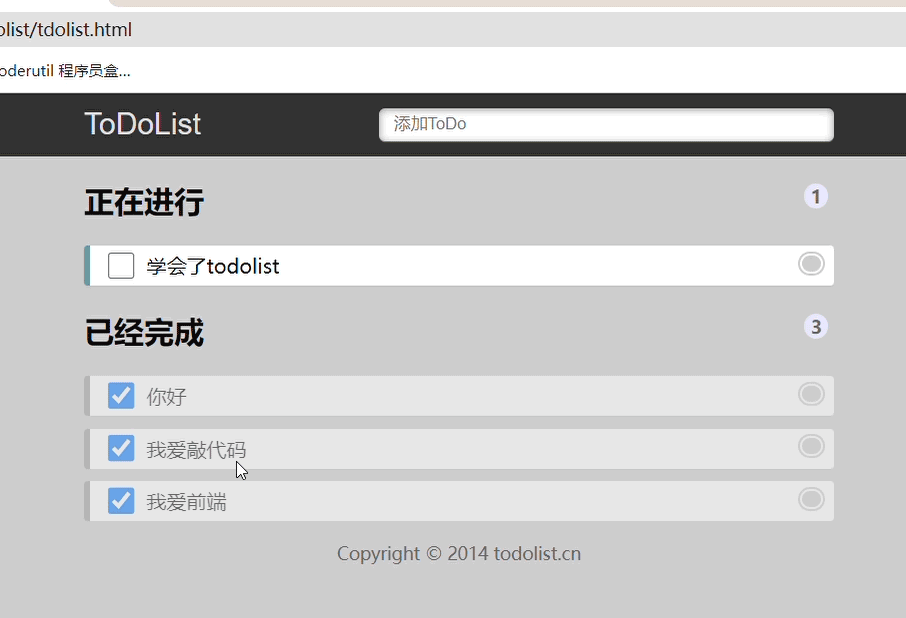
ToDoList—最简单的待办事项列表(经典案例)重点
案例说明案例分析案例实现代码在这里插入代码片代码运行结果插入结果图片
·
代码运行结果
案例说明
案例分析
案例实现代码
tdlist.html代码
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />
<title>ToDoList—最简单的待办事项列表</title>
<link rel="stylesheet" href="css/index.css">
<script src="js/jquery.min.js"></script>
<script src="js/todolist.js"></script>
</head>
<body>
<header>
<section>
<label for="title">ToDoList</label>
<input type="text" id="title" name="title" placeholder="添加ToDo" required="required" autocomplete="off" />
</section>
</header>
<section>
<h2>正在进行 <span id="todocount"></span></h2>
<ol id="todolist" class="demo-box">
</ol>
<h2>已经完成 <span id="donecount"></span></h2>
<ul id="donelist">
</ul>
</section>
<footer>
Copyright © 2014 todolist.cn
</footer>
</body>
</html>
index.css代码
body {
margin: 0;
padding: 0;
font-size: 16px;
background: #CDCDCD;
}
header {
height: 50px;
background: #333;
background: rgba(47, 47, 47, 0.98);
}
section {
margin: 0 auto;
}
label {
float: left;
width: 100px;
line-height: 50px;
color: #DDD;
font-size: 24px;
cursor: pointer;
font-family: "Helvetica Neue", Helvetica, Arial, sans-serif;
}
header input {
float: right;
width: 60%;
height: 24px;
margin-top: 12px;
text-indent: 10px;
border-radius: 5px;
box-shadow: 0 1px 0 rgba(255, 255, 255, 0.24), 0 1px 6px rgba(0, 0, 0, 0.45) inset;
border: none
}
input:focus {
outline-width: 0
}
h2 {
position: relative;
}
span {
position: absolute;
top: 2px;
right: 5px;
display: inline-block;
padding: 0 5px;
height: 20px;
border-radius: 20px;
background: #E6E6FA;
line-height: 22px;
text-align: center;
color: #666;
font-size: 14px;
}
ol,
ul {
padding: 0;
list-style: none;
}
li input {
position: absolute;
top: 2px;
left: 10px;
width: 22px;
height: 22px;
cursor: pointer;
}
p {
margin: 0;
}
li p input {
top: 3px;
left: 40px;
width: 70%;
height: 20px;
line-height: 14px;
text-indent: 5px;
font-size: 14px;
}
li {
height: 32px;
line-height: 32px;
background: #fff;
position: relative;
margin-bottom: 10px;
padding: 0 45px;
border-radius: 3px;
border-left: 5px solid #629A9C;
box-shadow: 0 1px 2px rgba(0, 0, 0, 0.07);
}
ol li {
cursor: move;
}
ul li {
border-left: 5px solid #999;
opacity: 0.5;
}
li a {
position: absolute;
top: 2px;
right: 5px;
display: inline-block;
width: 14px;
height: 12px;
border-radius: 14px;
border: 6px double #FFF;
background: #CCC;
line-height: 14px;
text-align: center;
color: #FFF;
font-weight: bold;
font-size: 14px;
cursor: pointer;
}
footer {
color: #666;
font-size: 14px;
text-align: center;
}
footer a {
color: #666;
text-decoration: none;
color: #999;
}
@media screen and (max-device-width: 620px) {
section {
width: 96%;
padding: 0 2%;
}
}
@media screen and (min-width: 620px) {
section {
width: 600px;
padding: 0 10px;
}
}
todolist.js代码
$(function() {
// alert(11);
// 1. 按下回车 把完整数据 存储到本地存储里面
// 存储的数据格式 var todolist = [{title: "xxx", done: false}]
// 每次页面打开就加载数据
load();
$("#title").on("keydown", function(event) {
if (event.keyCode == 13) {
if ($(this).val() === "") {
alert("请输入你要输入的内容!")
} else {
// 先读取本地存储的数据(声明一个数组保存数据)
var local = getDate();
// console.log(local);
// 把local数组进行更新数据 把最新的数据追加给local数组
local.push({ title: $(this).val(), done: false });
// 把这个数组local存储到本地存储
saveData(local);
// todolist本地存储数据渲染加载到页面
load();
// 清空输入框
$(this).val("");
}
}
});
// 3.todolist 删除操作
$("ol,ul").on("click", "a", function() {
// 先获取本地存储
var data = getDate();
console.log(data);
// 修改数据
var index = $(this).attr("id");
console.log(index);
// 根据这个索引号删除相关的数据--数组的splice(i,1)方法
data.splice(index, 1);
// 保存的本地存储
saveData(data);
// 重新渲染页面
load();
});
// 4.todolist正在进行和已完成选项操作
$("ol,ul").on("click", "input", function() {
// alert(11);
// 先获取本地存储的数据
var data = getDate();
// 修改数据 自定义属性attr 固有属性prop
var index = $(this).siblings("a").attr("id");
console.log(index);
// data[?].done=?选中这个复选框的选中按钮
data[index].done = $(this).prop("checked");
console.log(data);
// 保存到本地存储
saveData(data);
// 重新渲染页面
load();
});
// 读取本地存储的数据
function getDate() {
var data = localStorage.getItem("todolist");
if (data !== null) {
// 本地存储里面的数据时字符串格式的 但是我们需要的是对象格式JSON.parse()
return JSON.parse(data);
} else {
return [];
}
}
// 保存本地存储数据
function saveData(data) {
localStorage.setItem("todolist", JSON.stringify(data));
}
// 渲染加载数据
function load() {
// 读取本地存储的数据
var data = getDate();
console.log(data);
// 遍历之前要先清空ol和ul里面的元素内容
$("ol,ul").empty();
var todoCount = 0; //正在进行的个数
var doneCount = 0; //已经完成的个数
// 遍历这个数据
$.each(data, function(i, n) {
// console.log(n);
// id=" + i + "为每个小a生成索引号
// $("ol").prepend("<li> <input type='checkbox'> <p>" + n.title + "</p> <a href='javascript:;' id=" + i + "></a> </li>");
if (n.done) {
$("ul").prepend("<li> <input type='checkbox' checked='checked'> <p>" + n.title + "</p> <a href='javascript:;' id=" + i + "></a> </li>");
doneCount++;
} else {
$("ol").prepend("<li> <input type='checkbox'> <p>" + n.title + "</p> <a href='javascript:;' id=" + i + "></a> </li>");
todoCount++;
}
});
// 前面的是元素,后面的是变量统计的个数
$("#donecount").text(doneCount);
$("#todocount").text(todoCount);
}
})
更多推荐
所有评论(0)