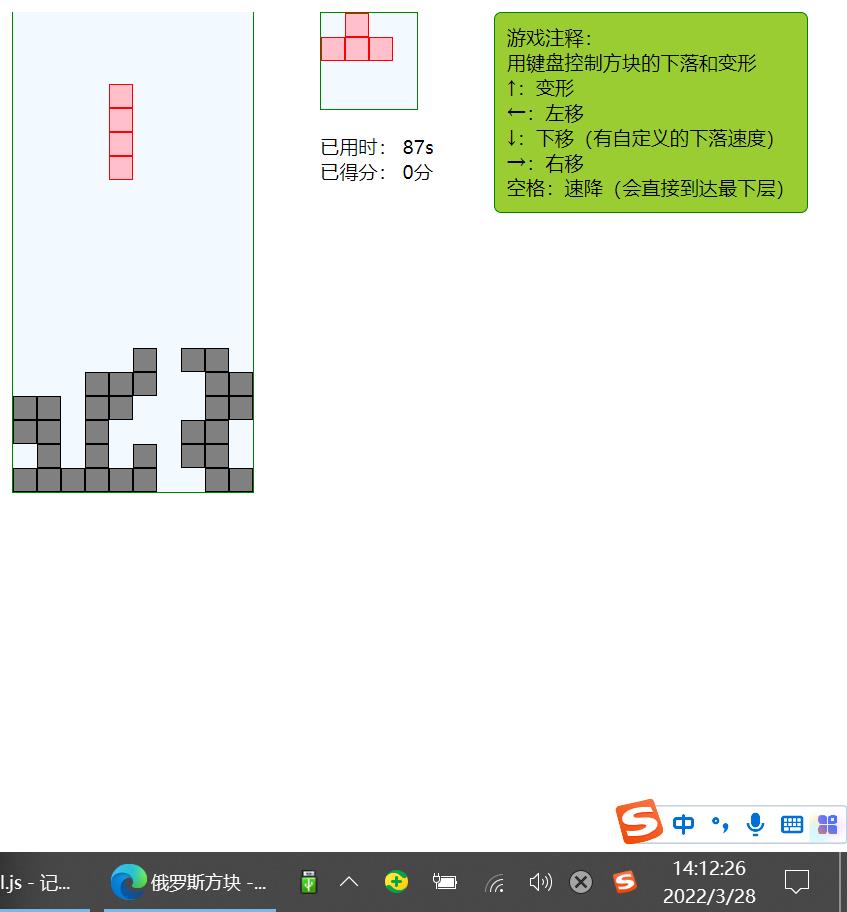
HTML5俄罗斯方块网页游戏代码
HTML5俄罗斯方块网页游戏代码 非常好用 代码如下注意把所有文件放在一个文件夹里!把所有css文件夹里,名'css'把所有js文件夹里,命名'js'先看'index.html'<!DOCTYPE html><html><head><meta charset="utf-8"><title>俄罗斯方块</title><li
·
HTML5俄罗斯方块网页游戏代码 非常好用 代码如下
注意
把所有文件放在一个文件夹里!
把所有css文件夹里,命名'css'
把所有js文件夹里,命名'js'
先看'index.html'
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>俄罗斯方块</title>
<link rel="stylesheet" type="text/css" href="css/style.css">
</head>
<body>
<div class="game" id="game"></div>
<div class="gameOver" id="gameOver">
<p>游戏结束啦</p>
</div>
<div class="info">
<div class="next" id="next"></div>
<div class="time">
<p>已用时: <span id="time">0</span>s</p>
<p>已得分: <span id="recode">0</span>分</p>
</div>
</div>
<div class="tips">
<p>游戏注释:</p>
<p>用键盘控制方块的下落和变形</p>
<p>↑:变形</p>
<p>←:左移</p>
<p>↓:下移(有自定义的下落速度)</p>
<p>→:右移</p>
<p>空格:速降(会直接到达最下层)</p>
</div>
</body>
<script type="text/javascript" src="js/square.js"></script>
<script type="text/javascript" src="js/squareFactory.js"></script>
<script type="text/javascript" src="js/game.js"></script>
<script type="text/javascript" src="js/local.js"></script>
<script type="text/javascript" src="js/script.js"></script>
</html>
JS:
game.js
//游戏核心代码
var Game = function(){
//dom元素
var gameDiv,nextDiv,scoreDiv;
//游戏矩阵
var gameData = [
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0],
[0,0,0,0,0,0,0,0,0,0]
];
//当前方块 下一个方块
var cur,next;
//渲染div
var nextDivs = [];
var gameDivs = [];
//初始化div
var initDiv = function(initData, initDivArray,elem){
for(var i = 0; i < initData.length; i++){
var gameDiv = [];
for(var j = 0; j <initData[0].length; j++){
var newNode = document.createElement("div");
newNode.className = "none";
newNode.style.top = (i*20) + "px";
newNode.style.left = (j*20) +"px";
elem.appendChild(newNode);
gameDiv.push(newNode);
}
initDivArray.push(gameDiv);
}
}
//刷新div
var refresh = function(initData,initDivArray){
for(var i = 0; i<initData.length; i++){
for(var j = 0; j<initData[0].length; j++){
switch(initData[i][j]){
case 0:
initDivArray[i][j].className = 'none'
break;
case 1:
initDivArray[i][j].className = 'done';
break;
case 2:
initDivArray[i][j].className = 'current';
break;
default:
break;
}
}
}
}
//检测点是否合法
var check = function(pos,x,y){
if(pos.x + x < 0){
//上边界
return false;
}else if(pos.x + x >= gameData.length){
//下边界
return false;
}else if(pos.y + y < 0){
//左边界
return false;
}else if(pos.y + y >=gameData[0].length){
//右边界
return false;
}else if(gameData[pos.x + x][pos.y + y] ==1){
//位置上已经有完成的方块
return false;
}else{
return true;
}
}
//检测数据是否合理
var isValid = function(pos,data){
for(var i = 0; i < data.length; i++){
for(var j = 0; j <data[0].length; j++){
if(data[i][j] != 0){
if(!check(pos,i,j)){
return false;
}
}
}
}
return true;
}
//清除数据
var clearData = function(){
for(var i = 0; i < cur.data.length; i++){
for(var j = 0; j< cur.data[0].length; j++){
if(check(cur.origin,i,j)){
gameData[cur.origin.x + i][cur.origin.y + j] = 0;
}
}
}
}
//设置数据,修改方块下落位置
var setData = function (){
for(var i = 0; i < cur.data.length; i++){
for(var j = 0; j< cur.data[0].length; j++){
if(check(cur.origin,i,j)){
gameData[cur.origin.x + i][cur.origin.y + j] = cur.data[i][j];
}
}
}
}
//旋转
var rotate = function(){
if(cur.canRotate(isValid)){
clearData();
cur.rotate();
setData();
refresh(gameData,gameDivs);
}
}
//下移
var down = function(){
//初始化原点位置
if(cur.canDown(isValid)){
clearData();
cur.down();
setData();
refresh(gameData,gameDivs);
return true;
}else{
return false;
}
}
//右移动
var right = function(){
if(cur.canRight(isValid)){
clearData();
cur.right();
setData();
refresh(gameData,gameDivs);
}
}
//左移动
var left = function(){
if(cur.canLeft(isValid)){
clearData();
cur.left();
setData();
refresh(gameData,gameDivs);
}
}
//方块移动到底部的时候,固定
var fixed = function(){
for(var i = 0; i <cur.data.length; i++){
for(var j=0;j<cur.data[0].length; j++){
if(check(cur.origin,i,j)){
if(gameData[cur.origin.x + i][cur.origin.y + j] == 2){
gameData[cur.origin.x + i][cur.origin.y + j] = 1
}
}
}
}
refresh(gameData,gameDivs);
}
//使用下一个方块
var performNext = function(){
cur = next;
setData();
next = SquareFactory.prototype.make();
refresh(gameData,gameDivs);
refresh(next.data,nextDivs)
}
//消行方法
var checkClear = function(){
//从底部开始判断,从下往上,遇到一整排符合条件的,删除
for(var i =gameData.length -1;i>=0;i--){
var clear = true;
for(var j =0;j<gameData[0].length; j++){
if(gameData[i][j]!==1){
clear = false;
break;
}
}
if(clear){
for(var m=i; m>0;m--){
for(var n =0;n<gameData[0].length;n++){
gameData[m][n] = gameData[m-1][n]
}
}
for(var n=0;n<gameData[0].length;n++){
gameData[0][n] = 0;
}
setRecord();
i++;
}
}
}
//判断游戏是否结束
var checkGameOver = function(){
var gameOver = false;
for(var i = 0;i<gameData[0].length;i++){
if(gameData[1][i] == 1){
gameOver = true;
}
}
return gameOver;
}
//初始化
var init = function(doms){
gameDiv = doms.gameDiv;
nextDiv = doms.nextDiv;
scoreDiv=doms.recodeDiv;
//实例化方块数据
cur = SquareFactory.prototype.make();
next = SquareFactory.prototype.make();
//初始化游戏区的所有方块布局
initDiv(gameData,gameDivs,gameDiv);//用div填充游戏区
initDiv(next.data,nextDivs,nextDiv);//用div填充待出现方块区
//在game游戏区更新掉落方块的位置。所以就是需要把当前方块的位置赋值到game游戏区的对应位置。
setData();
//刷新游戏区方块布局
refresh(gameData,gameDivs);
refresh(next.data,nextDivs);
}
//设置分数
var record = 0;
var setRecord = function(){
record++;
scoreDiv.innerHTML = record;
}
//导出API
this.init = init;
this.down = down;
this.right = right;
this.left = left;
this.rotate = rotate;
this.fall= function(){
while(down()){
down();
}
return false;
}
this.fixed = fixed;
this.performNext = performNext;
this.checkClear = checkClear;
this.checkGameOver = checkGameOver;
}
local.js
// 本地游戏区
var Local = function(){
//游戏对象
var game;
//定时器
var timer = null;
//时间间隔
var INITERVAL = 600;
//计时效果
timeCount = 0;
//时间
var time = 0;
//绑定键盘事件
var bindKeyEvent = function(){
document.onkeydown = function(e){
switch(e.keyCode){
case 32:
//space;
game.fall();
break;
case 37:
//left;
game.left();
break;
case 38:
//top 切换形态
game.rotate();
break;
case 39:
//right;
game.right();
break;
case 40:
//down;
game.down();
break;
default :
break;
}
}
}
//移动
var move = function(){
if(!game.down()){
game.fixed();//判断是否固定游戏块
game.checkClear();//判断是否清行
if(game.checkGameOver()){
//判断游戏是否结束
stop();
}else{
game.performNext();
}
}
}
//计时函数
var timeunc = null;
var timeFunc = function(doms){
timeunc = setInterval(function(){
time++;
doms.timeDiv.innerHTML = time;
},1000) ;
}
//设置时间
//开始
var start = function(){
var doms = {
gameDiv :document.getElementById("game"),
nextDiv :document.getElementById("next"),
recodeDiv :document.getElementById("recode"),
timeDiv :document.getElementById("time")
}
game = new Game();
game.init(doms);
bindKeyEvent();
//计时系统
timeFunc(doms);
//定时器效果,方块自由下落效果
timer = setInterval (move ,INITERVAL)
}
//结束
var stop = function(){
if(timer){
clearInterval(timer);
timer = null;
}
if(timeunc){
clearInterval(timeunc);
timeunc = null;
}
document.onkeydown = null;
document.getElementById("gameOver").style.display = "block";
}
//导出API
this.start = start;
}
remote.js 不要漏掉这个
// 对战玩家游戏区
script.js 不要漏掉这个
var local = new Local();
local.start();
square.js
// 方块处理 左移,右移,旋转,下落。
var Square = function(){
//方块数据
this.data = [
[0,0,0,0],
[0,0,0,0],
[0,0,0,0],
[0,0,0,0]
];
//原点
this.origin = {
x : 0,
y : 3
};
//旋转数组
this.totates =function(data){
var sArray=[
[0,0,0,0],
[0,0,0,0],
[0,0,0,0],
[0,0,0,0]
];
for(var i = 0;i < data.length; i++){
var newIndex =3-i;
for(var j =0; j <data[0].length; j++){
sArray[j][newIndex] = data[i][j]
}
}
return sArray;
}
}
Square.prototype.canRotate = function(isValid){
/* this.data = this.totates(this.data);*/
return isValid(this.origin,this.totates(this.data));
}
Square.prototype.rotate = function(isValid){
this.data = this.totates(this.data);
}
Square.prototype.canDown = function(isValid){
var test = {};
test.x = this.origin.x + 1;
test.y = this.origin.y;
return isValid(test,this.data);
}
Square.prototype.down = function(){
this.origin.x = this.origin.x+1;
}
Square.prototype.canRight = function(isValid){
var test = {};
test.x = this.origin.x ;
test.y = this.origin.y +1;
return isValid(test,this.data);
}
Square.prototype.right = function(){
this.origin.y = this.origin.y + 1;
}
Square.prototype.canLeft = function(isValid){
var test = {};
test.x = this.origin.x ;
test.y = this.origin.y - 1;
return isValid(test,this.data);
}
Square.prototype.left = function(){
this.origin.y = this.origin.y - 1;
}
squareFactory.js
//负责生成方块,代码生成工厂
//七种不同类型的方块
/**
* 口
* 口
* 口
* 口*/
var Square1 = function(){
Square.call(this)
//方块数据
this.data = [
[0,2,0,0],
[0,2,0,0],
[0,2,0,0],
[0,2,0,0]
];
}
Square1.prototype = Square.prototype;
/*var square1 = [
[0,2,0,0],
[0,2,0,0],
[0,2,0,0],
[0,2,0,0]
];*/
/**
* 口
* 口口口*/
/*var square2 = [
[0,2,0,0],
[2,2,2,0],
[0,0,0,0],
[0,0,0,0]
];*/
var Square2 = function(){
Square.call(this)
//方块数据
this.data = [
[0,2,0,0],
[2,2,2,0],
[0,0,0,0],
[0,0,0,0]
];
}
Square2.prototype = Square.prototype;
/**
* 口口
* 口口*/
/*var square3 = [
[0,0,0,0],
[0,2,2,0],
[0,2,2,0],
[0,0,0,0]
];*/
var Square3 = function(){
Square.call(this)
//方块数据
this.data = [
[0,0,0,0],
[0,2,2,0],
[0,2,2,0],
[0,0,0,0]
];
}
Square3.prototype = Square.prototype;
/**
* 口
* 口口口*/
/*var square4 = [
[0,0,2,0],
[2,2,2,0],
[0,0,0,0],
[0,0,0,0]
];*/
var Square4 = function(){
Square.call(this)
//方块数据
this.data = [
[0,0,2,0],
[2,2,2,0],
[0,0,0,0],
[0,0,0,0]
];
}
Square4.prototype = Square.prototype;
/**
* 口口
* 口
* 口*/
/*var square5 = [
[2,2,0,0],
[2,0,0,0],
[2,0,0,0],
[0,0,0,0]
];*/
var Square5 = function(){
Square.call(this)
//方块数据
this.data = [
[2,2,0,0],
[2,0,0,0],
[2,0,0,0],
[0,0,0,0]
];
}
Square5.prototype = Square.prototype;
/**
* 口口
* 口口*/
/*var square6 = [
[0,2,2,0],
[0,0,2,2],
[0,0,0,0],
[0,0,0,0]
];*/
var Square6 = function(){
Square.call(this)
//方块数据
this.data = [
[0,2,2,0],
[0,0,2,2],
[0,0,0,0],
[0,0,0,0]
];
}
Square6.prototype = Square.prototype;
/**
* 口口
* 口口*/
/*var square7 = [
[0,0,2,2],
[0,2,2,0],
[0,0,0,0],
[0,0,0,0]
];*/
var Square7 = function(){
Square.call(this)
//方块数据
this.data = [
[0,0,2,2],
[0,2,2,0],
[0,0,0,0],
[0,0,0,0]
];
}
Square7.prototype = Square.prototype;
var SquareFactory = function(){}
SquareFactory.prototype.make = function(){
var index = Math.ceil(Math.random()*7);
var s;
switch(index){
case 1:
s = new Square1();
break;
case 2:
s = new Square2();
break;
case 3:
s = new Square3();
break;
case 4:
s = new Square4();
break;
case 5:
s = new Square5();
break;
case 6:
s = new Square6();
break;
case 7:
s = new Square7();
break;
default :
break;
}
return s;
}
css部分 就一个
style.css
*{
padding: 0px;
margin: 0px;
}
body{
padding: 10px;
}
.game{
position: relative;
width: 200px;
height: 400px;
background-color: #f2faff;
border-width: 0 1px 1px ;
border-style: solid;
border-color: green;
display: inline-block;
}
.gameOver{
width: 200px;
height: 400px;
background:rgba(0,0,0,.5);
position: absolute;
top: 10px;
left: 10px;
text-align: center;
display: none;
}
.gameOver>p{
color: #fff;
margin-top: 180px;
}
.next{
width: 80px;
height: 80px;
position: relative;
border: 1px solid green;
vertical-align: top;
background-color: #f2faff;
display: inline-block;
}
.info{
/*border:1px solid red;*/
padding-left: 50px;
display: inline-block;
/*width: 80px;*/
vertical-align: top;
}
.time{
margin-top: 20px;
}
/*定义div样式*/
.none,.current,.done{
height: 20px;
width: 20px;
position: absolute;
box-sizing: border-box;
}
.none{
background: #f2faff;
}
.current{
background-color: pink;
border:1px solid red;
}
.done{
background-color: gray;
border:1px solid #000;
}
.tips{
border: 1px solid green;
background: yellowgreen;
/*color: #fff;*/
display: inline-block;
vertical-align: top;
margin-left: 45px;
padding: 10px;
border-radius: 6px;
}
好了就到这里了
希望大家自己理解吧
更多推荐
所有评论(0)