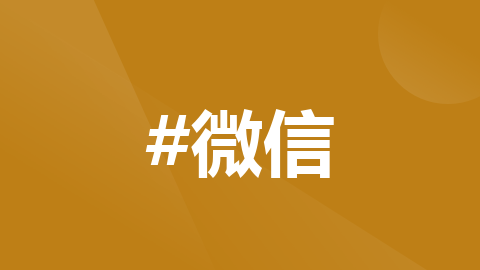
第三期 小程序云开发之如何使用云函数查询云数据库
在我的小程序中有这么一个业务——查询当前登录用户所有位置打卡记录,在微信js中只能一次性获取20条,但是不足以达到我的目的,于是使用云函数获取数据库数据(一次可获取100条),但是要达到该用户全部数据都查询出来,需要加个分页循环查询。
·
背景
在我的小程序中有这么一个业务——查询当前登录用户所有位置打卡记录,在微信js中只能一次性获取20条,但是不足以达到我的目的,于是使用云函数获取数据库数据(一次可获取100条),但是要达到该用户全部数据都查询出来,需要加个分页循环查询。
第一步:创建云函数
找到云函数列表,单击鼠标右键,创建云函数,创建云函数前需要有云开发环境,具体介绍请点击看我另一篇博客有介绍,需要创建云开发小程序
第二步:找到云函数里面的index.js,编写查询数据库数据的方法
// 云函数入口文件
const cloud = require('wx-server-sdk')
cloud.init()
const db = cloud.database();
// 云函数入口函数
exports.main = async (event, context) => {
// 在云函数中获取微信调用上下文
const wxContext = cloud.getWXContext();
// 根据当前用户openid获取位置打卡总条数
const count = await getCount(wxContext.OPENID);
let total = count.total;
let list = [];
// 分页查询数据,每次查询100条
for (let i = 0; i < total; i += 100) {
list = list.concat(await getList(wxContext.OPENID, i));
}
return {
event,
openid: wxContext.OPENID,
appid: wxContext.APPID,
unionid: wxContext.UNIONID,
total: total,
list: list
}
}
// 统计用户位置打卡次数
async function getCount(openid) {
let count = await db.collection('position').where({
"_openid": openid
}).count();
return count;
}
// 查询用户位置打卡列表
async function getList(openid,skip) {
let list = await db.collection('position').where({
"_openid": openid
}).orderBy('_id', 'asc').skip(skip).get();
return list.data;
}
第三步:上传云函数
点击云函数,单机鼠标右键创建并上传云函数即可
第四步:在需要使用到的功能js调用该云函数
这里有时候上传后还是调用不了,可能是上传失败了,重新上传即可
//查询打卡记录
onList: function () {
var that = this;
wx.cloud.callFunction({
// 自己定义的云函数名称
name: 'getmyfoot',
// 传给云函数的参数
data: {
openid: that.data.openid
},
success: function (res) {
//这里的res就是云函数的返回值
console.log(res)
}
},
fail: console.error
})
}
扩展:云开发之查询数据分页
在我们开发过程中经常需要对数据以列表的形式展现
小程序中常见的分页显示方式常见的就有两种:1、按钮式切换下一页 2、下滑自动刷新下一页数据
1.按钮式切换下一页具体实现代码
wxml
<view class="container">
<block wx:if="{{clock_in}}">
<view wx:for="{{clock_in}}" wx:key="item">
{{clock_in[index].name}} {{clock_in[index].time}}
</view>
</block>
<view class="i-page-c">
<button bindtap="handleChange" id="prev" disabled="{{current == 1}}">上一页</button>
<view class="page-current">{{current + '/' + pageSize}}</view>
<button bindtap="handleChange" id="next" disabled="{{current == pageSize}}">下一页</button>
</view>
</view>
js
// pages/myClockIn/myClockIn.js
const app = getApp();
var util = require('../../utils/util.js');
Page({
/**
* 页面的初始数据
*/
data: {
clock_in: [{}],
// 当前页
current: 1,
// 总页数
pageSize: 0,
show: true
},
/**
* 生命周期函数--监听页面加载
*/
onLoad: function (options) {
this.queryData(0);
},
// 查询数据
queryData(pageNum) {
this.setData({
show: true
})
var userid = app.getOpenid();
var that = this;
wx.cloud.callFunction({
name: 'callback',
data: {_openid: userid, pageNum: pageNum},
success: res => {
this.setData({
show: false
})
if (res.result.total % 10 > 0) {
that.setData({
pageSize: parseInt(res.result.total/10) == 0? 1: parseInt(res.result.total/10) + 1
})
} else {
that.setData({
pageSize: parseInt(res.result.total/10) == 0? 1: parseInt(res.result.total/10)
})
}
var playurl = res.result.data;
var timefo = [{}];
for (var i = 0; i < playurl.length;i++){
var date = new Date(playurl[i].time);
var date_value = util.formatTime(date);
timefo[i] = { time: date_value, name: playurl[i].name, _id: playurl[i]._id};
}
this.setData({
clock_in: timefo
})
},
fail: err => {
console.error('[云函数] [callback] 调用失败',err)
wx.navigateTo({
url: '../deployFunctions/deployFunctions',
})
}
})
},
// 分页显示
handleChange (e) {
console.log(e)
const type = e.currentTarget.id;
if (type === 'next') {
this.setData({
current: this.data.current + 1
});
} else if (type === 'prev') {
this.setData({
current: this.data.current - 1
});
}
this.queryData(this.data.current -1);
},
})
效果:
2.下滑自动切换下一页
wxml和上面一致,需要注意的是页面需要足够长,这样才可以触发上滑事件
可以在数据底部加进行测试,前提是你要有10条以上的数据
js
// 查询数据
queryData(pageNum) {
this.setData({
show: true
})
var userid = app.getOpenid();
var that = this;
wx.cloud.callFunction({
name: 'callback',
data: {
_openid: userid,
pageNum: pageNum
},
success: res => {
this.setData({
show: false
})
if (res.result.total % 10 > 0) {
that.setData({
pageSize: parseInt(res.result.total / 10) == 0 ? 1 : parseInt(res.result.total / 10) + 1
})
} else {
that.setData({
pageSize: parseInt(res.result.total / 10) == 0 ? 1 : parseInt(res.result.total / 10)
})
}
var playurl = res.result.data;
var timefo = [{}];
for (var i = 0; i < playurl.length; i++) {
var date = new Date(playurl[i].time);
var date_value = util.formatTime(date);
timefo[i] = {
time: date_value,
name: playurl[i].name,
_id: playurl[i]._id
};
}
this.setData({
clock_in: that.data.clock_in.concat(timefo)
})
},
fail: err => {
console.error('[云函数] [callback] 调用失败', err)
wx.navigateTo({
url: '../deployFunctions/deployFunctions',
})
}
})
},
/**
* 页面上拉触底事件的处理函数
*/
onReachBottom: function () {
var that = this;
// 还不是最后一页
if (that.data.current != that.data.pageSize) {
this.setData({
current: this.data.current + 1
});
this.queryData(this.data.current - 1);
}
},
json
{
// 当滑动到距离底部50rpx时调用onReachBottom方法
"onReachBottomDistance": 50,
}
更多推荐
所有评论(0)