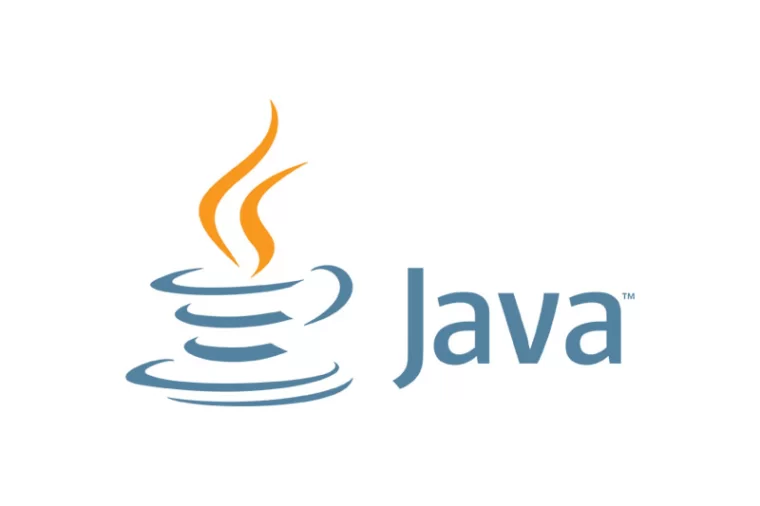
SpringBoot整合MiniIo
MiniIo是一款开源的、轻量级的、分布式的云存储服务。与其他云存储服务相比,MiniIo偏向于“自部署”的架构,也就是说,它更适合部署在自己的服务器上,而不是类似于阿里云、腾讯云等云服务商提供的云存储服务。MiniIo可以支持海量的数据存储,让用户可以轻松地存储、管理和访问自己的数据。MiniIo是一款自部署的、轻量级的、分布式的云存储服务,它具有自主管理、可定制性等优点,但也存在部署和运维较复
什么是MiniIo?
MiniIo是一款开源的、轻量级的、分布式的云存储服务。与其他云存储服务相比,MiniIo偏向于“自部署”的架构,也就是说,它更适合部署在自己的服务器上,而不是类似于阿里云、腾讯云等云服务商提供的云存储服务。MiniIo可以支持海量的数据存储,让用户可以轻松地存储、管理和访问自己的数据。
MiniIo的优缺点
作为“自部署”的云存储服务,MiniIo具有以下优点:
-
轻量级:MiniIo基于Golang开发,部署简单,需要的系统资源较少。
-
分布式:MiniIo可以分布式部署,可以通过集群的方式提高可靠性和性能。
-
自主管理:用户可以自主地管理存储在MiniIo上的数据,可以自己决定是否需要进行备份和还原。
-
可定制性:MiniIo提供了丰富的自定义配置选项,用户可以根据自己的需求进行定制。
当然,MiniIo也存在一些缺点:
-
部署和运维较复杂:MiniIo需要用户自己进行部署和运维,对于没有相关经验的用户来说,可能存在一定的门槛。
-
功能相对简单:与其他云存储服务相比,MiniIo的功能相对简单,可能无法满足某些特殊需求。
如何在项目开发中使用
接下来,我们将介绍在Spring Boot项目中如何整合MiniIo,以便在开发中使用。
添加依赖
在pom.xml中添加MiniIo的maven依赖:
<dependency>
<groupId>io.minio</groupId>
<artifactId>minio</artifactId>
<version>8.2.5</version>
</dependency>
配置文件
在application.yml中添加以下配置:
spring:
minio:
url: http://localhost:9000 # MiniIo服务的URL
access-key: <your-access-key> # MiniIo的Access Key
secret-key: <your-secret-key> # MiniIo的Secret Key
bucket: <your-bucket-name> # 桶的名称
region: <your-bucket-region> # 桶的地区
编写代码
使用@Autowired将MinioTemplate注入到Controller中:
@RestController
public class MiniIoController {
@Autowired
private MinioTemplate minioTemplate;
@PostMapping("/upload")
public void upload(@RequestParam("file") MultipartFile file) throws IOException, InvalidKeyException, NoSuchAlgorithmException, XmlPullParserException, InvalidPortException, InvalidEndpointException, RegionConflictException, NoResponseException, ErrorResponseException, InternalException, InvalidArgumentException {
String filename = file.getOriginalFilename();
InputStream inputStream = file.getInputStream();
minioTemplate.putObject(filename, inputStream);
}
@GetMapping("/download")
public void download(HttpServletResponse response) throws InvalidKeyException, NoSuchAlgorithmException, IOException, InvalidPortException, InvalidEndpointException, XmlPullParserException, ErrorResponseException, NoResponseException, InternalException, InvalidArgumentException {
String filename = "test.jpg";
InputStream inputStream = minioTemplate.getObject(filename);
response.setContentType("image/jpeg");
response.setHeader("Content-Disposition", "attachment; filename=" + filename);
ServletOutputStream outputStream = response.getOutputStream();
byte[] buf = new byte[1024];
int len = 0;
while ((len = inputStream.read(buf, 0, 1024)) != -1) {
outputStream.write(buf, 0, len);
}
outputStream.flush();
outputStream.close();
}
}
在上述代码中,我们使用@Autowired注解将MinioTemplate注入到Controller中,然后就可以调用minioTemplate提供的方法进行文件的上传和下载了。
至此,我们已经完成了在Spring Boot项目中整合MiniIo的步骤,您可以尝试在自己的项目中使用MiniIo来进行文件的存储和管理。
总结一下:
MiniIo是一款自部署的、轻量级的、分布式的云存储服务,它具有自主管理、可定制性等优点,但也存在部署和运维较复杂、功能相对简单等缺点。在Spring Boot项目中使用MiniIo需要添加相应的依赖、配置文件,并通过MinioTemplate进行文件的上传和下载。
希望本篇博文能够对您有所帮助,如果有疑问或不足之处,欢迎指正和补充。
更多推荐
所有评论(0)