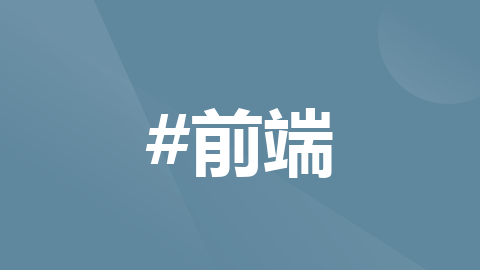
vue2 + vite + tailwind css 搭建项目
vue+vite 项目。发现启动项目速度是真快。就自己尝试搭建了一个基础框架。
前言
vue+vite 项目 。发现启动项目速度是真快。就自己尝试搭建了一个基础框架。
一、基础搭建
- cnpm init vue@2
执行上面代码会提示:
✔ Add TypeScript? … No / Yes
✔ Add JSX Support? … No / Yes
✔ Add Vue Router for Single Page Application development? … No / Yes
✔ Add Pinia for state management? … No / Yes
✔ Add Vitest for Unit Testing? … No / Yes
✔ Add Cypress for both Unit and End-to-End testing? … No / Yes
✔ Add ESLint for code quality? … No / Yes
不知道选择什么就直接一路回车就好啦~
根据提示完成:
cd init
npm install
npm run dev
二、vite 配置文件
vite.config.js 代码如下:
import { fileURLToPath, URL } from 'node:url'
import { defineConfig } from 'vite'
import legacy from '@vitejs/plugin-legacy'
import { createVuePlugin } from 'vite-plugin-vue2'
export default defineConfig({
base:'./',
plugins: [
createVuePlugin(),
],
resolve: {
alias: {
'@': fileURLToPath(new URL('./src', import.meta.url))
}
},
server: {
port: 2234,
open: true,
host: true,
proxy: {
'/api/': {
target: "http//xxx",
changeOrigin: true,
rewrite: (path) => path.replace(/^\/api/, ''),
},
'/mock/': {
target: "http//xxx",
changeOrigin: true,
rewrite: (path) => path.replace(/^\/mock/, ''),
},
},
},
})
三、vue-router 的安装
安装依赖 (vue 2.x 只能使用 Vue Router 3 版本)
npm i vue-router@3
创建router/index.js文件
代码如下:
import Vue from 'vue'
import VueRouter from 'vue-router'
// 注册路由插件
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'home',
component: () => import('@/views/Home/index.vue'),
},
{
path: '/other',
name: 'Other',
component: () => import('@/views/Other/index.vue'),
},
]
const router = new VueRouter({
mode: 'history',
base: import.meta.env.BASE_URL,
routes,
scrollBehavior() {
return { x: 0, y: 0 };
},
});
export default router;
在main.js挂载路由配置
代码如下:
import router from './router/index'```
new Vue({
// el: "#app",
router: router,
render: h => h(App),
}).$mount("#app");
四、vuex 安装
安装依赖 (Vue 2.x 只能使用 Vuex 3 版本)
npm i vuex@3
五、axios 安装
安装依赖
npm i axios
创建utils/request.js文件
代码如下:
import axios from 'axios';
import { Notification, MessageBox, Message } from "element-ui";
const service = axios.create({
baseURL: import.meta.env.VITE_BASE_URL,
timeout: 20000,
headers: {
'Content-Type': 'application/json; charset=utf-8',
},
});
// 请求拦截器
service.interceptors.request.use(
(config) => {
config.headers['Fawkes-Auth'] = localStorage.getItem('token');
return config;
},
(error) => {
console.log(error);
Promise.reject(error);
}
);
// 响应拦截器
service.interceptors.response.use(
(response) => {
const { status, data, statusText } = response;
if (status === 200) { //根据自己项目的成功状态码设置
const code = data.code || 8000000; // 未设置状态码则默认成功状态
if (code === 8000000 || code === 200) { // 成功为8000000
return data;
} else {
Notification({
title: String(code),
message: data.msg || '系统未知错误,请反馈给管理员',
type: 'error',
duration: 3 * 1000,
});
return Promise.reject(data);
}
} else {
const msg = statusText || '系统未知错误,请反馈给管理员';
Notification({
title: String(status),
message: msg,
type: 'error',
duration: 3 * 1000,
});
return Promise.reject(msg);
}
},
(error) => {
let { message } = error;
if (message === 'Network Error') {
message = '网络连接异常';
} else if (message.includes('timeout')) {
message = '系统接口请求超时';
} else if (message.includes('Request failed with status code')) {
message = '系统接口' + message.substr(message.length - 3) + '异常';
}
Notification({
title: '出错了',
message: message,
type: 'error',
duration: 3 * 1000,
});
return Promise.reject(error);
}
);
export default service;
创建api/xxx.js文件并使用
import request from '@/utils/request';
export function getData() {
return request({
url: "/xxx",
method: "get",
});
}
六、tailwindcss 安装
安装依赖
npm install -D tailwindcss@latest postcss@latest autoprefixer@latest
npx tailwindcss init
创建postcss.config文件
代码如下:
module.exports = {
plugins: {
tailwindcss: {},
autoprefixer: {},
},
}
创建tailwind.config文件
代码如下:
module.exports = {
purge: [],
purge: ['./index.html', './src/**/*.{vue,js,ts,jsx,tsx}'],
darkMode: false, // or 'media' or 'class'
theme: {
extend: {},
},
variants: {
extend: {},
},
plugins: [],
}
创建assets/styles/tailwind.css文件
代码如下:
@tailwind base;
@tailwind components;
@tailwind utilities;
在main.js中引入
import from ‘@/assets/styles/tailwind.css’
可以使用测试一下,如果没有效果重新启动一下项目~
7、在项目中使用scss
安装依赖
npm install node-sass
npm install sass --save-dev
各种报错处理
- scss 安装报错
yarn add --dev sass sass-loader@latest
- 组件引入报错
import Other from '@/views/Other/index' //错误写法
import Other from '@/views/Other/index.vue' //正确写法
更多推荐
所有评论(0)