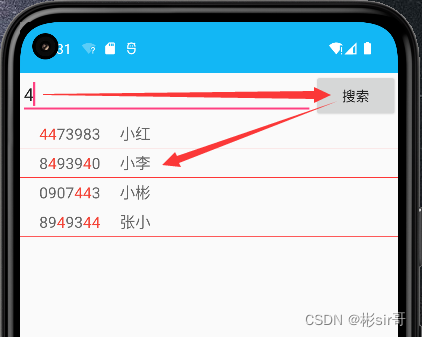
Android kotlin 实现搜索关键字高亮显示功能(RecyclerView+BRVAH3.0.6+androidx)
RecyclerView+BRVAH3.0.6+androidx
·
一、实现效果
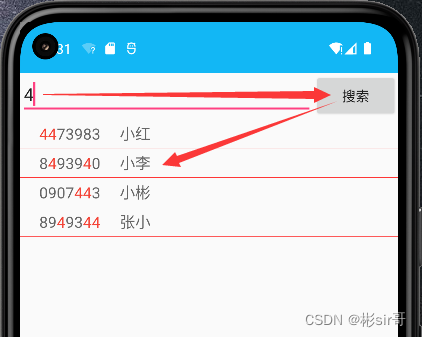
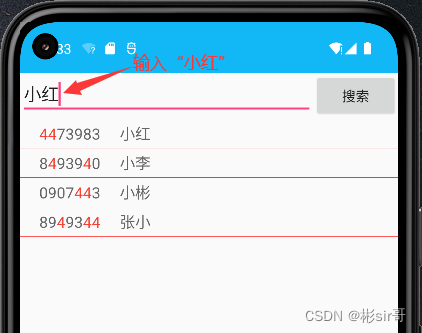
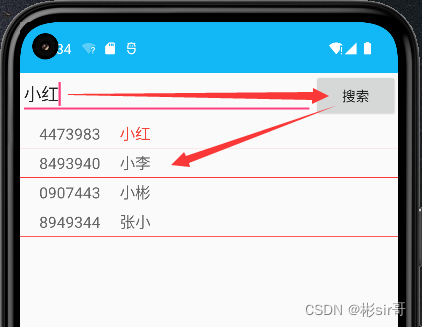
二、引入依赖
在app
的build.gradle
在添加以下代码
1、implementation 'com.github.CymChad:BaseRecyclerViewAdapterHelper:3.0.6'
,这个里面带的适配器,直接调用就即可
BaseRecyclerViewAdapterHelper
简称BRVAH
Android SDK | 是否支持BaseRecyclerViewAdapterHelper:3.0.6 |
---|---|
android compileSdkVersion 29 | 是 |
android compileSdkVersion 30 | 是 |
android compileSdkVersion 31 | 是 |
android compileSdkVersion 32 | 是 |
android compileSdkVersion 33 | 是 |
这依赖包还需要得到要添加,在Project
的build.gradle
在添加以下代码,不添加就不行
allprojects {
repositories {
...
maven { url "https://jitpack.io" }//加上
}
}
三、实现源码
1、文字变色工具类
KeyWordUtil.kt
package com.example.myapplication3.util
import android.text.SpannableString
import android.text.Spanned
import android.text.TextUtils
import android.text.style.ForegroundColorSpan
import java.util.regex.Matcher
import java.util.regex.Pattern
object KeyWordUtil {
/**
* 关键字高亮变色
*
* @param color 变化的色值
* @param text 文字
* @param keyword 文字中的关键字
* @return 结果SpannableString
*/
fun matcherSearchTitle(color: Int, text: String, keyword: String): SpannableString {
var text = text
var keyword = keyword
val s = SpannableString(text)
keyword = escapeExprSpecialWord(keyword)
text = escapeExprSpecialWord(text)
if (text.contains(keyword) && !TextUtils.isEmpty(keyword)) {
try {
val p: Pattern = Pattern.compile(keyword)
val m: Matcher = p.matcher(s)
while (m.find()) {
val start: Int = m.start()
val end: Int = m.end()
s.setSpan(
ForegroundColorSpan(color),
start,
end,
Spanned.SPAN_EXCLUSIVE_EXCLUSIVE
)
}
} catch (e: Exception) {
}
}
return s
}
/**
* 转义正则特殊字符 ($()*+.[]?\^{},|)
*
* @param keyword
* @return keyword
*/
private fun escapeExprSpecialWord(keyword: String): String {
var keyword = keyword
if (!TextUtils.isEmpty(keyword)) {
val fbsArr =
arrayOf("\\", "$", "(", ")", "*", "+", ".", "[", "]", "?", "^", "{", "}", "|")
for (key in fbsArr) {
if (keyword.contains(key)) {
keyword = keyword.replace(key, "\\" + key)
}
}
}
return keyword
}
}
2、实体类
User.java
package com.example.myapplication3.Bean;
public class User {
Integer id; //Id
String number;//账号
String nick; //昵称
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public String getNick() {
return nick;
}
public void setNick(String nick) {
this.nick = nick;
}
public User() {
super();
}
public User(Integer id, String number, String nick) {
super();
this.id = id;
this.number = number;
this.nick = nick;
}
}
3、适配器
搜索的列表适配器SearchRvAdapter.kt
package com.example.myapplication3.adapter
import com.chad.library.adapter.base.BaseQuickAdapter
import com.chad.library.adapter.base.viewholder.BaseViewHolder
import com.example.myapplication3.Bean.User
import com.example.myapplication3.R
class SearchRvAdapter(layoutResId: Int = R.layout.search_rv_item) : BaseQuickAdapter<User, BaseViewHolder>(layoutResId) {
override fun convert(holder: BaseViewHolder, item: User) {
if (mCallBack != null) {
mCallBack!!.convert(holder, item);
}
}
//回调
private var mCallBack: ItemSelectedCallBack? = null
fun setItemSelectedCallBack(CallBack: ItemSelectedCallBack?) {
mCallBack = CallBack
}
interface ItemSelectedCallBack {
fun convert(holder: BaseViewHolder?, item:User)
}
}
item
布局search_rv_item.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/search_rv_item_tv_userNumber"
android:layout_width="wrap_content"
android:layout_height="30dp"
android:layout_marginLeft="20dp"
android:gravity="center"
android:text="123456"
android:textColor="#666"
android:textSize="16sp" />
<TextView
android:id="@+id/search_rv_item_tv_userNick"
android:layout_width="wrap_content"
android:layout_height="30dp"
android:layout_marginLeft="20dp"
android:gravity="center"
android:textColor="#666"
android:textSize="16sp"
android:text="小彬"/>
</LinearLayout>
<View
android:layout_width="match_parent"
android:layout_height="0.5dp"
android:background="@color/red" />
</LinearLayout>
4、实现视图
MainActivity.kt
package com.example.myapplication3
import android.content.Context
import android.graphics.Color
import android.os.Bundle
import android.text.SpannableString
import android.view.inputmethod.InputMethodManager
import androidx.appcompat.app.AppCompatActivity
import androidx.recyclerview.widget.LinearLayoutManager
import com.chad.library.adapter.base.viewholder.BaseViewHolder
import com.example.myapplication3.Bean.User
import com.example.myapplication3.adapter.SearchRvAdapter
import com.example.myapplication3.util.KeyWordUtil
import kotlinx.android.synthetic.main.activity_main.*
import kotlinx.android.synthetic.main.search_rv_item.view.*
class MainActivity : AppCompatActivity() {
private val mAdapter by lazy {
SearchRvAdapter()
}
//用户输入的搜索内容
private var searchContent = ""
//用户集合
private var mUserList: MutableList<User> = ArrayList()
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
init()
}
private fun init() {
val layoutManager = LinearLayoutManager(this@MainActivity)
layoutManager.orientation = LinearLayoutManager.VERTICAL
recyclerview.layoutManager = layoutManager
recyclerview.adapter = mAdapter
mAdapter.setItemSelectedCallBack(object : SearchRvAdapter.ItemSelectedCallBack {
override fun convert(holder: BaseViewHolder?, item: User) {
//设置控件的值
//搜索高亮显示
if (item.number != null && item.number.length > 0) {
val number: SpannableString = KeyWordUtil.matcherSearchTitle(
Color.parseColor("#F44336"),
item.number + "",
searchContent
)
holder!!.itemView.run { search_rv_item_tv_userNumber.text = number }
}
if (item.nick != null && item.nick.length > 0) {
val nick: SpannableString = KeyWordUtil.matcherSearchTitle(
Color.parseColor("#F44336"),
item.nick,
searchContent
)
holder!!.itemView.run { search_rv_item_tv_userNick.text = nick }
}
}
})
//点击搜索框
search_editText_searchContent.setOnClickListener {
search_editText_searchContent.isFocusable = true;
search_editText_searchContent.isFocusableInTouchMode = true;
search_editText_searchContent.requestFocus();
val imm = getSystemService(Context.INPUT_METHOD_SERVICE) as InputMethodManager
imm.showSoftInput(search_editText_searchContent, 0);
}
//点击搜索
btn_search.setOnClickListener {
if (mAdapter.data.size > 0) {
mUserList.clear()
}
//获取用户搜索的内容
searchContent = search_editText_searchContent.text.toString()
mAdapter.notifyDataSetChanged()
//初始化一些数据(实际开发中,是去数据库中读取)
val u1 = User(1, "4473983", "小红");
mUserList.add(u1);
val u2 = User(2, "8493940", "小李");
mUserList.add(u2);
val u3 = User(3, "0907443", "小彬");
mUserList.add(u3);
val u4 = User(4, "8949344", "张小");
mUserList.add(u4)
mAdapter.setList(mUserList)
}
}
}
activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<EditText
android:id="@+id/search_editText_searchContent"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1" />
<Button
android:id="@+id/btn_search"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="搜索" />
</LinearLayout>
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerview"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
更多推荐
所有评论(0)