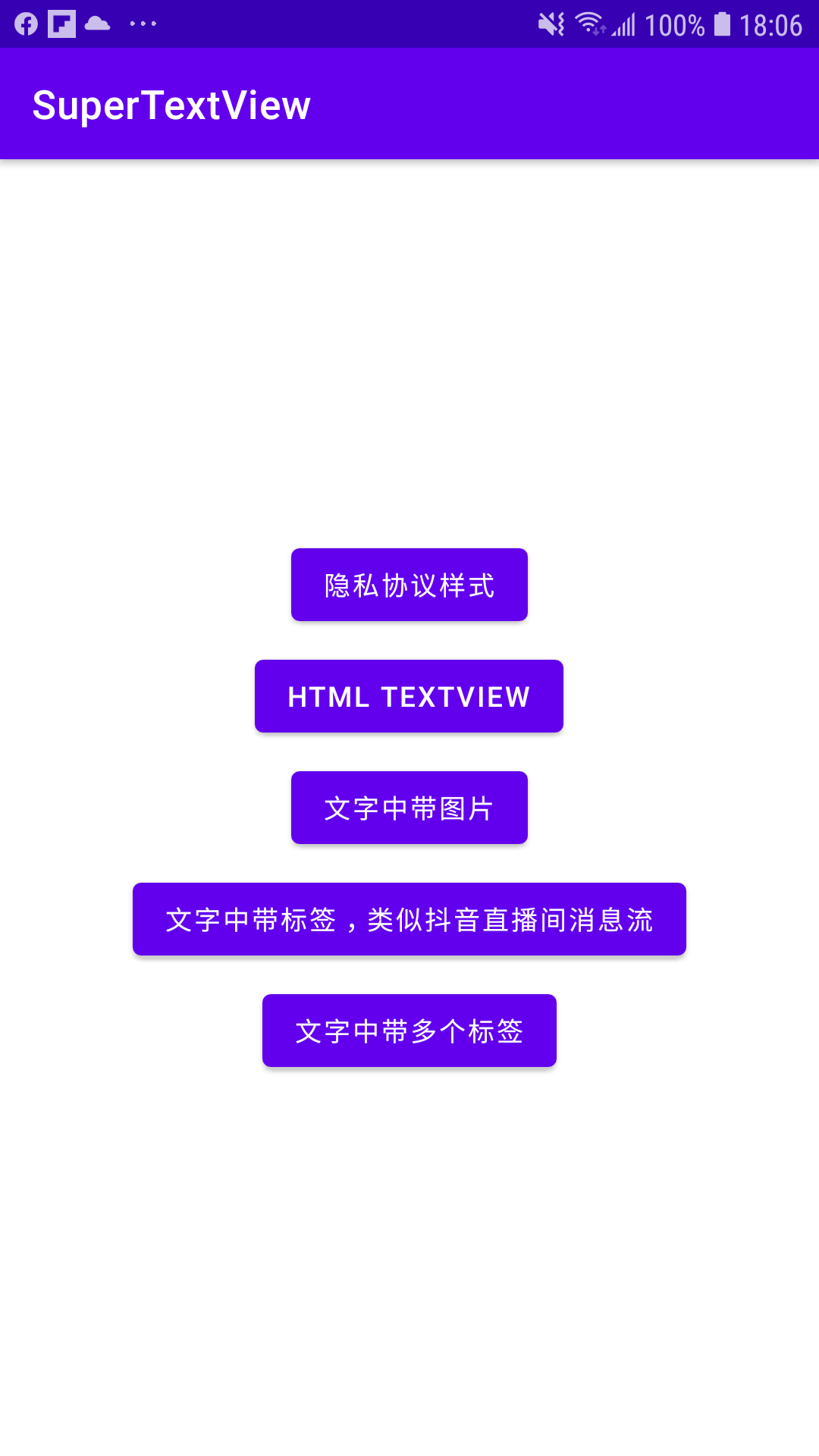
总结安卓中常用的TextView的样式(SuperTextView)
总结安卓中常用的TextView的样式(SuperTextView)
·
引言:TextView在平时的开发中一般是显示文案,但是在实际开发中文字经常要显示一些特殊的样式,为了满足不同场景下的需求,特此总结常用的TextView,命名为:SuperTextView
场景一:使用TextView显示服务条款和隐私协议,并实现点击跳转H5。
核心代码:
public class PrivacyPolicyTextActivity extends AppCompatActivity {
private TextView mPrivacyPolicyView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_privacy_policy);
mPrivacyPolicyView = findViewById(R.id.privacyPolicy);
initPrivacyPolicy();
}
private void initPrivacyPolicy() {
String contentString = getString(R.string.login_des_privacy_desc, getString(R.string.terms_of_service_desc), getString(R.string.privacy_policy_desc));
String termsOfService = getString(R.string.terms_of_service_desc);
SpannableString spannableString = new SpannableString(contentString);
int termsOfIndex = contentString.indexOf(termsOfService);
ClickableSpan termsOfClick = new ClickableSpan() {
@Override
public void onClick(@NonNull View widget) {
Intent intent = new Intent(PrivacyPolicyTextActivity.this, WebViewActivity.class);
intent.putExtra("url", "https://www.baidu.com/");
intent.putExtra("title", termsOfService);
startActivity(intent);
}
@Override
public void updateDrawState(@NonNull TextPaint ds) {
super.updateDrawState(ds);
ds.setColor(ResourceUtil.getColor(R.color.red_common_pure));
ds.setUnderlineText(true);
}
};
spannableString.setSpan(termsOfClick, termsOfIndex, termsOfIndex + termsOfService.length(), Spannable.SPAN_EXCLUSIVE_INCLUSIVE);
String privacyPolicy = ResourceUtil.getString(R.string.privacy_policy_desc);
int privacyIndex = contentString.indexOf(privacyPolicy);
ClickableSpan privacyClick = new ClickableSpan() {
@Override
public void onClick(@NonNull View widget) {
Intent intent = new Intent(PrivacyPolicyTextActivity.this, WebViewActivity.class);
intent.putExtra("url", "https://www.baidu.com/");
intent.putExtra("title", privacyPolicy);
startActivity(intent);
}
@Override
public void updateDrawState(@NonNull TextPaint ds) {
super.updateDrawState(ds);
ds.setColor(ResourceUtil.getColor(R.color.red_common_pure));
ds.setUnderlineText(true);
}
};
spannableString.setSpan(privacyClick, privacyIndex, privacyIndex + privacyPolicy.length(), Spannable.SPAN_EXCLUSIVE_INCLUSIVE);
ForegroundColorSpan colorSpan = new ForegroundColorSpan(ContextCompat.getColor(this, R.color.red_common_pure));
spannableString.setSpan(colorSpan, termsOfIndex, termsOfIndex + termsOfService.length(), Spannable.SPAN_EXCLUSIVE_INCLUSIVE);
ForegroundColorSpan colorSpan2 = new ForegroundColorSpan(ContextCompat.getColor(this, R.color.red_common_pure));
spannableString.setSpan(colorSpan2, privacyIndex, privacyIndex + privacyPolicy.length(), Spannable.SPAN_EXCLUSIVE_INCLUSIVE);
mPrivacyPolicyView.setText(spannableString);
mPrivacyPolicyView.setMovementMethod(LinkMovementMethod.getInstance());
}
}
场景二:平时开发中设计经常要求在一段话中一些文字要加粗,显示不同的颜色等,这个时候有不同的做法,常用也是最简单的就是借助HTML:
核心代码:
public class HTMLTextActivity extends AppCompatActivity {
private TextView mHtmlTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_htmltext);
mHtmlTextView = findViewById(R.id.html_text);
String str1 = "<font color='#FFF258'><b>" + "程序猿" + "</b> </font>";
String str2 = "<font color='#03DAC5'><b>" + "北京" + "</b> </font>";
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) {
mHtmlTextView.setText(Html.fromHtml(ResourceUtil.getString(R.string.html_message, str1, str2), Html.FROM_HTML_MODE_LEGACY));
} else {
mHtmlTextView.setText(Html.fromHtml(ResourceUtil.getString(R.string.html_message, str1, str2)));
}
}
}
场景三:文字中经常要穿插一些图片,就是文字加图片的样式:
代码:
public class ImageTextViewActivity extends AppCompatActivity {
private TextView mTitleTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_image_text_view);
mTitleTextView = findViewById(R.id.tv_title);
String texValue01 = " 你好 ";
String texValue02 = " 我在北京 ";
String allTexValue = "A" + texValue01 + "B" + texValue02 + "C";
int tex01ValueIndex = allTexValue.indexOf(texValue01);
int tex01ValueEndIndex = tex01ValueIndex + texValue01.length();
int tex02ValueIndex = allTexValue.indexOf(texValue02);
int tex02ValueEndIndex = tex02ValueIndex + texValue02.length();
SpannableString spannableString = new SpannableString(allTexValue);
ForegroundColorSpan colorSpan = new ForegroundColorSpan(ResourceUtil.getColor(R.color.red_common_pure));
spannableString.setSpan(colorSpan, tex01ValueIndex, tex01ValueEndIndex, Spanned.SPAN_INCLUSIVE_EXCLUSIVE);
ForegroundColorSpan colorSpan2 = new ForegroundColorSpan(ResourceUtil.getColor(R.color.teal_200));
spannableString.setSpan(colorSpan2, tex02ValueIndex, tex02ValueEndIndex, Spanned.SPAN_INCLUSIVE_EXCLUSIVE);
ImageSpan imgSpan = new ImageSpan(this, R.drawable.e_1);
spannableString.setSpan(imgSpan, 0, 1, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
ImageSpan imgSpan2 = new ImageSpan(this, R.drawable.e_2);
spannableString.setSpan(imgSpan2, tex01ValueEndIndex, tex01ValueEndIndex + 1, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
ImageSpan imgSpan3 = new ImageSpan(this, R.drawable.e_3);
spannableString.setSpan(imgSpan3, tex02ValueEndIndex, tex02ValueEndIndex + 1, Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
mTitleTextView.setText(spannableString);
}
}
场景四:文字中要求加特殊的UI样式,这个时候可以在TextView中加一个View
public class TagTextViewActivity extends AppCompatActivity {
private TextView mTitleTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_tag_text_view);
mTitleTextView = findViewById(R.id.tv_title);
View markerView = LayoutInflater.from(this).inflate(R.layout.item_marker_fore_title, null);
SpannableStringBuilder builder = new SpannableStringBuilder();
final String REPLACE_TEXT = "A";
builder.append(REPLACE_TEXT);
builder.setSpan(new MarkerViewSpan(markerView), 0, REPLACE_TEXT.length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
String oriText = "你好,我在北京";
builder.append(oriText);
mTitleTextView.setText(builder);
}
}
更多推荐
所有评论(0)