STL学习笔记(二),函数模板
#include <iostream>#include <string>using namespace std;//函数模板template <typename T>//也可以使用template <class T>声明函数模板void print_(const T &v
·
#include <iostream>
#include <string>
using namespace std;
//函数模板
template <typename T>//也可以使用template <class T>声明函数模板
void print_(const T &var)
{
cout<<var<<endl;
}
int main()
{
int x = 1;
string str = "abc";
float f = 1.2;
print_(x);
print_(str);
print_(f);
system("pause");
return 0;
}
#include <iostream>
#include <string>
using namespace std;
//多个参数的函数模板定义
template <class T1, class T2>
void print1(T1 &t1, T2 &t2)
{
cout<<t1<<", "<<t2<<endl;
}
int main()
{
int n = 123;
string str = "abc";
print1(n, str);
print1(str, n);
system("pause");
return 0;
}
#include <iostream>
#include <string>
#include <sstream>
//将字符串转换成其他数据类型
template <typename T>
T fromString(const std::string &str)
{
std::istringstream is(str);
T t;
is >> t;
return t;
}
//将其他数据类型转换成字符串
template <typename T>
std::string toString(const T &t)
{
std::ostringstream s;
s << t;
return s.str();
}
int main()
{
int x = 123;
std::string str = "456";
int x1 = fromString<int>(str);
std::string str1 = toString(x);
std::cout<<"x1 = "<<x1<<std::endl;
std::cout<<"str1 = "<<str1<<std::endl;
system("pause");
return 0;
}
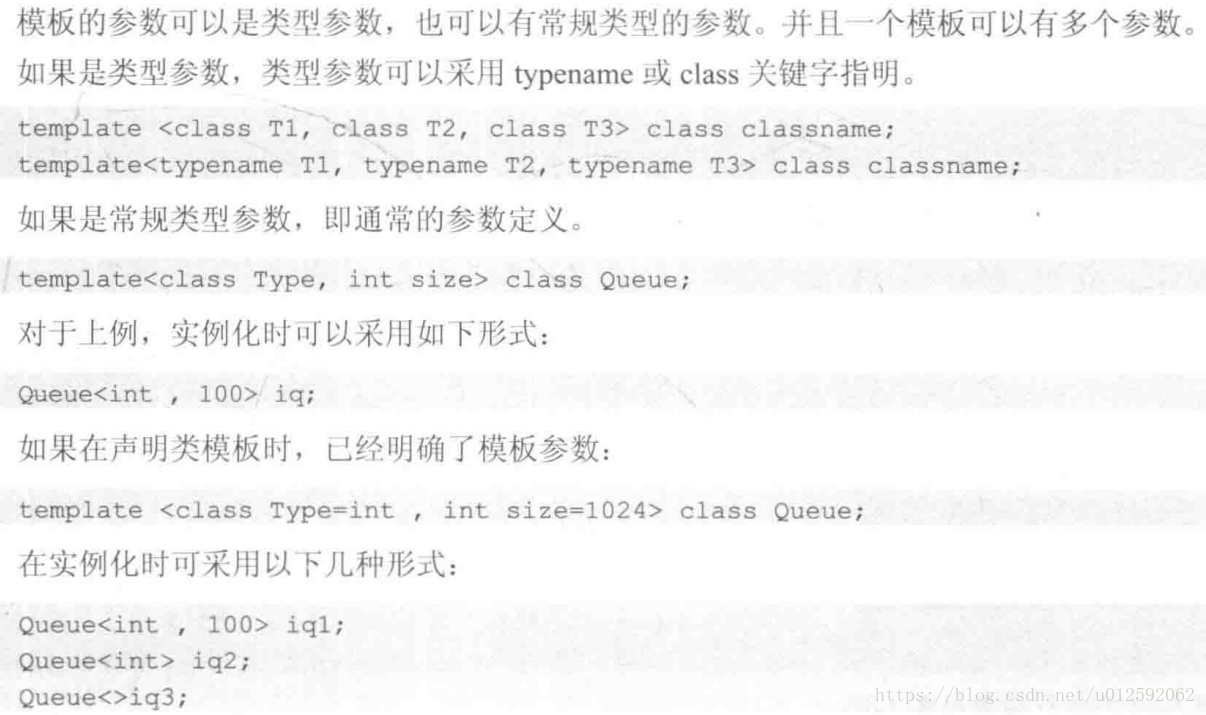
更多推荐
所有评论(0)