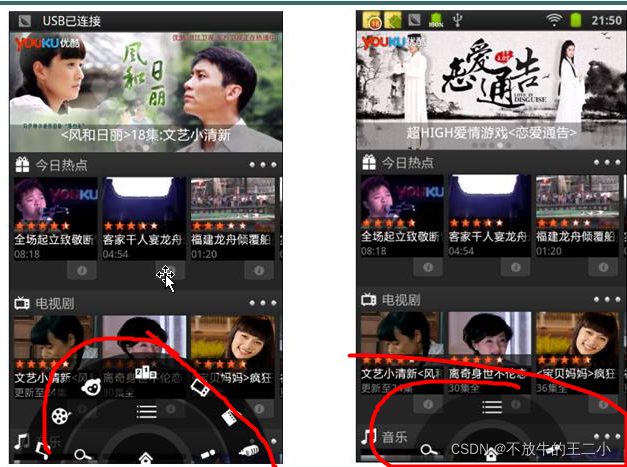
安卓实现菜单栏导航
效果展示:一、设置布局文件:1、指定按钮的id的时候,不使用@+id,使用@id,会在values中生成对应的id<?xml version="1.0" encoding="utf-8"?><resources><item name="level1" type="id"/><item name="level2" type="id" /><ite
效果展示:
一、设置布局文件:
1、指定按钮的id的时候,不使用@+id,使用@id,会在values中生成对应的id
<?xml version="1.0" encoding="utf-8"?>
<resources>
<item name="level1" type="id"/>
<item name="level2" type="id" />
<item name="level3" type="id" />
<item name="home" type="id" />
<item name="search" type="id" />
<item name="menu" type="id" />
<item name="myyouku" type="id" />
<item name="c1" type="id" />
<item name="c2" type="id" />
<item name="c3" type="id" />
<item name="c4" type="id" />
<item name="c5" type="id" />
<item name="c6" type="id" />
<item name="c7" type="id" />
</resources>
2、添加对应的按钮:
①如果是按钮(Button):需要使用属性background
②如果是图片按钮(ImageView):需要使用src
3、在二级导航菜单和三级导航菜单中添加相应的按钮:
设置每个按钮的id
二、代码实现动画效果:
一)创建动画效果MyAnimation:
创建入动画和出动画的方法:
1、入动画:
①当动画进入的时候,要显示出每个按钮,这时候需要设置每个按钮的动作:
设置显示每个按钮、可获取焦点、可点击等
Tips:两种不显示的常量的区别
INVISIBLE:不显示还占用空间
GONE:不显示且不占用空间
②创建旋转动画:
@使用Animation的子类RotateAnimation中参数最多的构造函数
* fromDegrees 起始旋转角度 显示 左-->右 -180---> 0 隐藏 右 --> 左 0 --> -180
* toDegrees 结束旋转角度
* pivotXType x轴 参照物
* pivotXValue 指定参照 x轴 参数物的哪个位置进行旋转
* pivotYType y轴 参照物
* pivotYValue 指定参照 y轴 参数物的哪个位置进行旋转
@设置动画运行时间:setDuration(duration)
@设置动画结束时停留在结束的位置:setFillAfter(true)
@开始动画:startAnimation(animation)
2、出动画
二级菜单先启动,然后三级菜单启动,需要有一个延时启动的效果
①创建RotateAnimation,和出动画类似,只是旋转的起始和终止的位置不同:
②设置动画的监听事件:setAnimationListener:
其中的onAnimationEnd,当动画出去的时候,按钮都不能有效果了
③开始动画效果:startAnimation(animation)
二)设置按钮的相关操作(在MainActivity中)
1、初始化菜单和按钮
在onCreate方法中调用自定义初始化方法initView()
①找到导航菜单
②创建是否显示的标记
在initView()方法中初始化home按钮的点击监听事件:
Home按钮(二级菜单):
如果要隐藏:三级菜单显示了,需要先隐藏三级菜单,然后再隐藏二级菜单(二级菜单需要有延迟);
三级菜单隐藏了,直接隐藏二级菜单
如果要显示:直接显示二级菜单
2、设置menu按钮:(三级菜单)
当点击的时候,菜单隐藏了,要显示出来,反之要隐藏
点击二级菜单上的menu按钮进行显示和隐藏
示例代码:
布局:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/root"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
<RelativeLayout
android:id="@id/level1"
android:layout_width="100dip"
android:layout_height="50dip"
android:background="@drawable/level1"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
>
<ImageButton
android:id="@id/home"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/icon_home"
android:layout_centerInParent="true"
/>
</RelativeLayout>
<RelativeLayout
android:id="@id/level2"
android:layout_width="180dip"
android:layout_height="90dip"
android:background="@drawable/level2"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
>
<ImageButton
android:id="@id/search"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/icon_search"
android:layout_margin="10dip"
android:layout_alignParentBottom="true"
/>
<ImageButton
android:id="@id/menu"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/icon_menu"
android:layout_centerHorizontal="true"
android:layout_margin="6dip"
/>
<ImageButton
android:id="@id/myyouku"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/icon_myyouku"
android:layout_margin="10dip"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
/>
</RelativeLayout>
<RelativeLayout
android:id="@id/level3"
android:layout_width="280dip"
android:layout_height="140dip"
android:background="@drawable/level3"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
>
<ImageButton
android:id="@id/c1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/channel1"
android:layout_alignParentBottom="true"
android:layout_marginLeft="12dip"
android:layout_marginBottom="6dip"
/>
<ImageButton
android:id="@id/c2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/channel2"
android:layout_above="@id/c1"
android:layout_marginLeft="30dip"
android:layout_marginBottom="12dip"
/>
<ImageButton
android:id="@id/c3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/channel3"
android:layout_above="@id/c2"
android:layout_toRightOf="@id/c2"
android:layout_marginLeft="8dip"
android:layout_marginBottom="6dip"
/>
<ImageButton
android:id="@id/c4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/channel4"
android:layout_centerHorizontal="true"
android:layout_margin="6dip"
/>
<ImageButton
android:id="@id/c5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/channel5"
android:layout_above="@id/c6"
android:layout_toLeftOf="@id/c6"
android:layout_marginRight="8dip"
android:layout_marginBottom="6dip"
/>
<ImageButton
android:id="@id/c6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/channel6"
android:layout_above="@id/c7"
android:layout_marginRight="30dip"
android:layout_marginBottom="12dip"
android:layout_alignParentRight="true"
/>
<ImageButton
android:id="@id/c7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/channel7"
android:layout_alignParentBottom="true"
android:layout_marginRight="12dip"
android:layout_marginBottom="6dip"
android:layout_alignParentRight="true"
/>
</RelativeLayout>
</RelativeLayout>
自定义动画效果(MyAnimation):
入动画:
//入动画
public static void startAminationIn(ViewGroup viewGroup, int duration) {
//循环设置group中的每个子view的状态(可点击,可获取焦点,显示)
for (int i = 0; i < viewGroup.getChildCount(); i++) {
viewGroup.getChildAt(i).setClickable(true);
viewGroup.getChildAt(i).setFocusable(true);
viewGroup.getChildAt(i).setVisibility(View.VISIBLE);
}
//创建动画,选择旋转效果
Animation animation;
animation = new RotateAnimation(-180, 0, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 1.0f);
//设置动画的运行时间
animation.setDuration(duration);
//设置动画结束后停留在结束的位置
animation.setFillAfter(true);
//显示动画
viewGroup.startAnimation(animation);
}
出动画:
//出动画
public static void startAminationOut(final ViewGroup viewGroup, int duration, int startOffSet) {
//创建动画,选择旋转效果
Animation animation;
animation = new RotateAnimation(
0, -180,
Animation.RELATIVE_TO_SELF, 0.5f,
Animation.RELATIVE_TO_SELF, 1.0f);
//设置动画的运行时间
animation.setDuration(duration);
//设置动画结束后停留在结束的位置
animation.setFillAfter(true);
//动画延迟启动时间
animation.setStartOffset(startOffSet);
//设置动画监听器
animation.setAnimationListener(new AnimationListener() {
//动画结束的时候,循环让每个按钮的效果不可以
public void onAnimationEnd(Animation animation) {
for (int i = 0; i < viewGroup.getChildCount(); i++) {
viewGroup.getChildAt(i).setClickable(false);
viewGroup.getChildAt(i).setFocusable(false);
viewGroup.getChildAt(i).setVisibility(Animation.INFINITE);
}
}
public void onAnimationStart(Animation animation) {
}
@Override
public void onAnimationRepeat(Animation animation) {
}
});
//开启动画
viewGroup.startAnimation(animation);
}
效果的实现:
private void initView() {
home = (ImageButton) findViewById(R.id.home);
menu = (ImageButton) findViewById(R.id.menu);
level2 = (RelativeLayout) findViewById(R.id.level2);
level3 = (RelativeLayout) findViewById(R.id.level3);
home.setOnClickListener(new MyHomeOnClickListener());
menu.setOnClickListener(new MyMenuOnClickListener());
}
//设置home的监听事件
private class MyHomeOnClickListener implements OnClickListener{
public void onClick(View v) {
//进行判断,如果二级菜单隐藏时,显示出来,否则隐藏
if(!isShowLevel2){
//显示二级菜单
MyAnimation.startAminationIn(level2, 500);
root.setBackgroundResource(R.drawable.photo5);
}else{
//隐藏二级菜单
//进行判断,如果三级菜单未隐藏时,先隐藏三级菜单,在隐藏二级菜单
//显示出来,否则隐藏
if(isShowLevel3){
//隐藏三级菜单
MyAnimation.startAminationOut(level3, 500, 0);
//隐藏二级菜单
MyAnimation.startAminationOut(level2, 500, 500);
isShowLevel3 = !isShowLevel3;
root.setBackgroundResource(R.drawable.photo2);
}else{
//隐藏二级菜单
MyAnimation.startAminationOut(level2, 500, 0);
}
}
isShowLevel2 = !isShowLevel2;
}
}
//设置menu的监听事件
private class MyMenuOnClickListener implements OnClickListener{
public void onClick(View v) {
if(isShowLevel3){
//隐藏三级菜单
MyAnimation.startAminationOut(level3, 500, 0);
}else{
//显示三级菜单
MyAnimation.startAminationIn(level3, 500);
root.setBackgroundResource(R.drawable.photo4);
}
isShowLevel3 = !isShowLevel3;
}
}
更多推荐
所有评论(0)