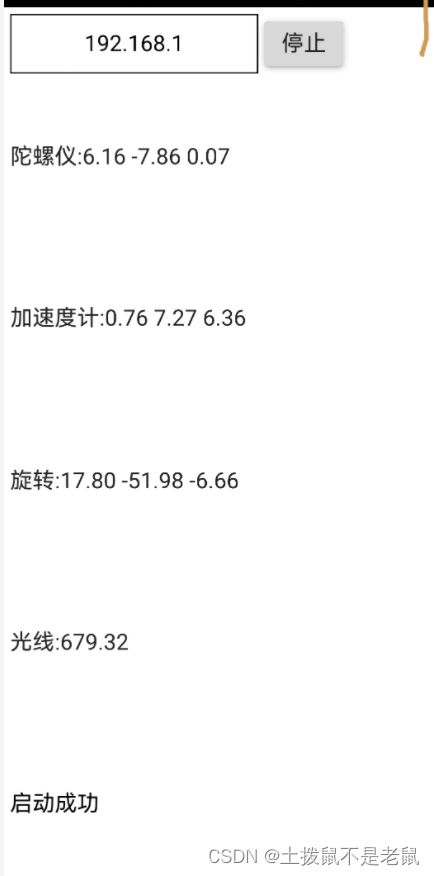
QML android 采集手机传感器数据 并通过udp 发送
利用 qt 开发 安卓 app ,采集手机传感器数据 并通过udp 发送。
·
利用 qt 开发 安卓 app ,采集手机传感器数据 并通过udp 发送
#ifndef UDPLINK_H
#define UDPLINK_H
#include <QObject>
#include <QUdpSocket>
#include <QHostAddress>
class UdpLink : public QObject
{
Q_OBJECT
public:
explicit UdpLink(QObject *parent = nullptr);
void setAddress(QString _ip,quint16 _port);
void sendData(QByteArray ba);
signals:
private:
QString ip;
quint16 port;
QUdpSocket socket;
};
#endif // UDPLINK_H
#include "udplink.h"
UdpLink::UdpLink(QObject *parent)
: QObject{parent}
{
}
void UdpLink::setAddress(QString _ip, quint16 _port)
{
ip=_ip;
port = _port;
}
void UdpLink::sendData(QByteArray ba)
{
socket.writeDatagram(ba, QHostAddress(ip), port);
}
#ifndef APP_H
#define APP_H
#include <QObject>
#include <udplink.h>
#include <atomic>
#include <QAccelerometer>
#include <QGyroscope>
#include <QRotationSensor>
#include <QLightSensor>
class App : public QObject
{
Q_OBJECT
Q_PROPERTY(bool isRuning READ getIsRuning WRITE setIsRuning NOTIFY isRuningChanged)
public:
explicit App(QObject *parent = nullptr);
Q_INVOKABLE void start(QString ip);
Q_INVOKABLE void stop();
bool getIsRuning() const;
void setIsRuning(bool newIsRuning);
signals:
void gyroValue(qreal x,qreal y,qreal z);
void accelerValue(qreal x,qreal y,qreal z);
void rotationValue(qreal x,qreal y,qreal z);
void lightValue(qreal lux);
void logInfo(QString str);
void isRuningChanged();
private:
UdpLink udplink;
bool isRuning{false};
//陀螺
QGyroscope *gyroscope;
QGyroscopeReading *gyroreader;
//加速度计
QAccelerometer *acceler;
QAccelerometerReading *accelereader;
//旋转
QRotationSensor *rotationSensor;
QRotationReading *rotationReading;
//光线
QLightSensor *lightSensor;
QLightReading *lightReading;
};
#endif // APP_H
#include "app.h"
#include <QtConcurrent>
#include <chrono>
#include <thread>
#include <QJsonDocument>
#include <QJsonArray>
#include <QJsonObject>
#include <QJsonValue>
App::App(QObject *parent)
: QObject{parent}
{
}
void App::start(QString ip)
{
udplink.setAddress(ip,8023);
qDebug()<<"start "<<ip;
gyroscope = new QGyroscope(this);
connect(gyroscope, &QGyroscope::readingChanged, this, [&](){
gyroreader = gyroscope->reading();
QJsonObject obj_root;
QJsonArray arr;
qreal gyroscopex = gyroreader->x();
qreal gyroscopey = gyroreader->y();
qreal gyroscopez = gyroreader->z();
arr.append(QString::number(gyroscopex,'f',2));
arr.append(QString::number(gyroscopey,'f',2));
arr.append(QString::number(gyroscopez,'f',2));
obj_root.insert("QGyroscope",arr);
QJsonDocument jsonDocu(obj_root);
QByteArray jsonData = jsonDocu.toJson();
udplink.sendData(jsonData);
emit gyroValue(gyroscopex,gyroscopey,gyroscopez);
});
acceler = new QAccelerometer(this);
acceler->setAccelerationMode(QAccelerometer::Combined);
connect(acceler, &QAccelerometer::readingChanged, this, [&](){
accelereader = acceler->reading();
QJsonObject obj_root;
QJsonArray arr;
qreal accelerx = accelereader->x();
qreal accelery = accelereader->y();
qreal accelerz = accelereader->z();
arr.append(QString::number(accelerx,'f',2));
arr.append(QString::number(accelery,'f',2));
arr.append(QString::number(accelerz,'f',2));
obj_root.insert("QAccelerometer",arr);
QJsonDocument jsonDocu(obj_root);
QByteArray jsonData = jsonDocu.toJson();
udplink.sendData(jsonData);
emit accelerValue(accelerx,accelery,accelerz);
});
rotationSensor = new QRotationSensor(this);
connect(rotationSensor, &QRotationSensor::readingChanged, this, [&](){
rotationReading = rotationSensor->reading();
QJsonObject obj_root;
QJsonArray arr;
qreal rotationx = rotationReading->x();
qreal rotationy = rotationReading->y();
qreal rotationz = rotationReading->z();
arr.append(QString::number(rotationx,'f',2));
arr.append(QString::number(rotationy,'f',2));
arr.append(QString::number(rotationz,'f',2));
obj_root.insert("QRotationSensor",arr);
QJsonDocument jsonDocu(obj_root);
QByteArray jsonData = jsonDocu.toJson();
udplink.sendData(jsonData);
emit rotationValue(rotationx,rotationy,rotationz);
});
lightSensor = new QLightSensor(this);
connect(lightSensor, &QLightSensor::readingChanged, this, [&](){
lightReading = lightSensor->reading();
QJsonObject obj_root;
QJsonArray arr;
qreal lux = lightReading->lux();
arr.append(QString::number(lux,'f',2));
obj_root.insert("QLightSensor",arr);
QJsonDocument jsonDocu(obj_root);
QByteArray jsonData = jsonDocu.toJson();
udplink.sendData(jsonData);
emit lightValue(lux);
});
if(gyroscope->start()&&acceler->start()&&rotationSensor->start()&&lightSensor->start()){
setIsRuning(true);
emit logInfo(QString::fromUtf8("启动成功"));
}else{
setIsRuning(false);
emit logInfo(QString::fromUtf8("启动失败"));
}
}
void App::stop()
{
gyroscope->stop();
acceler->stop();
rotationSensor->stop();
lightSensor->stop();
setIsRuning(false);
}
bool App::getIsRuning() const
{
return isRuning;
}
void App::setIsRuning(bool newIsRuning)
{
if (isRuning == newIsRuning)
return;
isRuning = newIsRuning;
emit isRuningChanged();
}
import QtQuick 2.15
import QtQuick.Window 2.15
import QtQuick.Controls 2.15
import QtQuick.Layouts 1.15
import App 1.0
Window {
id:root
width: 640
height: 480
visible: true
title: qsTr("数据采集")
App{
id:app
onGyroValue: {
var str = '陀螺仪:'+x.toFixed(2)+' '+y.toFixed(2)+' '+z.toFixed(2)
gyroLabel.text = str
}
onAccelerValue: {
var str = '加速度计:'+x.toFixed(2)+' '+y.toFixed(2)+' '+z.toFixed(2)
accelerLabel.text = str
}
onRotationValue: {
var str = '旋转:'+x.toFixed(2)+' '+y.toFixed(2)+' '+z.toFixed(2)
rotationLabel.text = str
}
onLightValue: {
var str = '光线:'+lux.toFixed(2)
lightLabel.text=str
}
onLogInfo: {
debugInof.text=str
}
}
RowLayout{
id:topBar
anchors.margins: 5
anchors.top: parent.top
anchors.left: parent.left
spacing: 5
Rectangle{
id:address
Layout.alignment: Qt.AlignHCenter
height: linkBtn.height
width: 200
border.color: "black"
border.width: 1
TextInput{
id:ip
anchors.fill: parent
verticalAlignment:Text.AlignVCenter
horizontalAlignment:Text.AlignHCenter
text: "192.168.1"
}
}
Button{
id:linkBtn
Layout.alignment: Qt.AlignHCenter
text: !app.isRuning?"启动":"停止"
onClicked: {
if(!app.isRuning){
app.start(ip.text)
}else{
app.stop()
}
}
}
}
ColumnLayout{
anchors.left:parent.left
anchors.right:parent.right
anchors.top:topBar.bottom
anchors.bottom:parent.bottom
anchors.margins: 5
Label{
id:gyroLabel
width: 200
height: 50
text: "陀螺仪"
}
Label{
id:accelerLabel
width: 200
height: 50
text: "加速度计"
}
Label{
id:rotationLabel
width: 200
height: 50
text: "旋转"
}
Label{
id:lightLabel
width: 200
height: 50
text:"光线"
}
TextEdit{
id:debugInof
height: 50
}
}
}
更多推荐
所有评论(0)