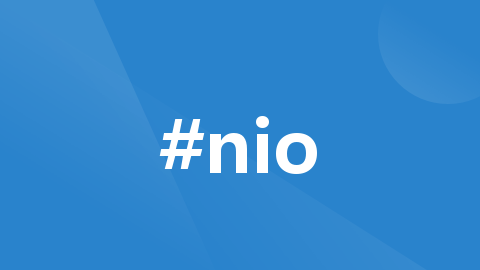
NIO - MappedByteBuffer 内存映射踩坑
NIO - MappedByteBuffer 内存映射踩坑
·
1. 出现问题
本人在公司的时候开发功能的时候,由于要使用 IO 流对大量的文件进行操作,但又因为效率问题,需要快速的对文件进行拷贝操作,所以经过再三思考后,决定使用 NIO 的 MappedByteBuffer 做内存映射的拷贝
因为功能要支持重跑,即要多次调用的时候,要将本地的文件清空,然后将新的文件从别的服务器拉取过来。此时问题出现了,当我第二次调用时,出现了下面的错误:
无法在使用用户映射区域打开的文件上执行
我下意识的认为,这个应该是我使用的流没有关闭完,导致程序还占用着资源;但经过排查发现,并不是这个问题
后来在几经调查后发现 MappedByteBuffer 的弊端:
- MappedByteBuffer 虽然是通过通道获取来的,但是并不会随着通道的关闭而释放在内存中该文件的
句柄
,所以无法进行删除;这个就是我在项目中无法重复调用的问题 - MappedByteBuffer 在内存中映射的大小是有限的,通过源码可以看见,在内存中映射的大小为
Integer.MAX_VALUE
2. 解决问题
最后经过自己的摸索和调试,编写出了下面解决上述两种弊端的程序,示例代码如下:
public class NioFileChannelDemo {
private static final long fileMaxSize = 1L << 25; // 32M
public static void main(String[] args) throws IOException {
long cur = 0L;
String sourceFile = "路径/文件名.后缀";
File source = new File(sourceFile);
File dest = new File("目标路径/目标文件名.目标后缀");
FileChannel inChannel = null;
FileChannel outChannel = null;
try {
MappedByteBuffer buf = null;
inChannel = new RandomAccessFile(source, "rw").getChannel();
outChannel = new FileOutputStream(dest).getChannel();
long inSize = inChannel.size();
if (inSize > fileMaxSize) { // 文件大于32M则采取分片拷贝
long num = inSize / fileMaxSize;
if ((inSize % fileMaxSize) != 0) {
num += 1; // 临界值考虑,最后一次拷贝
}
for (int i = 0; i < num; i++, cur += fileMaxSize) {
buf = inChannel.map(FileChannel.MapMode.READ_ONLY, cur, fileMaxSize);
outChannel.write(buf);
buf.force(); // 将此缓冲区所做的内容更改强制写入包含映射文件的存储设备中
buf.clear();
Method method = FileChannelImpl.class.getDeclaredMethod("unmap", MappedByteBuffer.class);
method.setAccessible(true);
method.invoke(FileChannelImpl.class, buf); // 释放内存中持有的句柄
}
} else {
buf = inChannel.map(FileChannel.MapMode.READ_ONLY, cur, inSize);
outChannel.write(buf);
buf.force(); // 将此缓冲区所做的内容更改强制写入包含映射文件的存储设备中
buf.clear();
}
inChannel.close();
outChannel.close();
Method method = FileChannelImpl.class.getDeclaredMethod("unmap", MappedByteBuffer.class);
method.setAccessible(true);
method.invoke(FileChannelImpl.class, buf); // 释放内存中持有的句柄
Files.delete(Paths.get(sourceFile)); //文件复制完成后,删除源文件
}catch(Exception e){
e.printStackTrace();
} finally {
inChannel.close();
outChannel.close();
}
}
}
可以看到,上面使用了反射的方式将 MappedByteBuffer 所占用的句柄释放掉了;不过本人也觉得挺奇怪的,不知道为什么这种方法作者要把他私有 [捂脸]
更多推荐
所有评论(0)