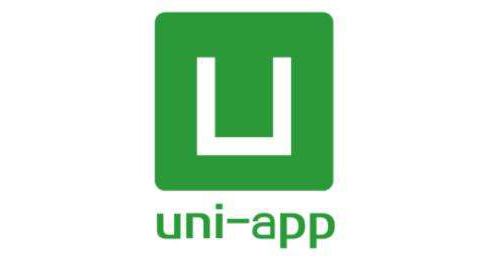
uni-app封装api请求(requery)
2. 在`api`文件夹下新建一个`api.js`文件,用来存放api请求的具体方法。3. 在需要使用的页面中引入`api.js`文件,然后即可使用封装的api方法。1. 在项目根目录下新建一个`api`文件夹,用来存放api请求。二、util 目录下新建 api.js。一、项目根目录新建 util 文件夹。五、在 index.vue 使用。三、编辑 api .js。四、main.js 导入。
·
方法一
一、项目根目录新建 util 文件夹
mkdir util
二、util 目录下新建 api.js
三、编辑 api .js
// uni.request、uni.showToast 为 uni-app官方API
const BASE_URL = 'http://localhost:8082/'
export const myRequery = (options) => {
return new Promise((resolve, reject) => {
uni.request({
url: BASE_URL + options.url,
method: options.method || 'GET',
data: options.data || {},
success: (res) => {
if (res.data.status !== 0) {
return uni.showToast({
title: "获取数据失败",
icon: 'error'
})
}
resolve(res)
},
fail: (err) => {
uni.showToast({
title: "请求失败",
icon: 'error'
})
reject(err)
}
})
})
}
四、main.js 导入
import Vue from 'vue'
// 导入
import { myRequery } from 'util/api.js'
// vue 全局挂载
Vue.prototype.$myRequery = myRequery
五、在 index.vue 使用
<template>
<view class="content">
首页
</view>
</template>
<script>
export default {
data() {
return {
swipers: []
}
},
onLoad() {
this.getSwipers()
},
methods: {
async getSwipers() {
const res = await this.$myRequery({ url: 'api/getlunbo' })
this.swipers = res.data.message
console.log(this.swipers)
}
}
}
</script>
<style>
</style>
方法二
1. 在项目根目录下新建一个`api`文件夹,用来存放api请求
2. 在`api`文件夹下新建一个`api.js`文件,用来存放api请求的具体方法
const baseUrl = 'http://xxxx.com/api' // 接口请求地址
// 封装请求方法
const request = (url, method, data) => {
wx.showLoading({
title: '加载中' // 数据请求前loading
})
return new Promise((resolve, reject) => {
wx.request({
url: baseUrl + url, // 开发者服务器接口地址
method: method,
data: data,
header: {
'content-type': 'application/json' // 默认值
},
success: function(res) {
// 请求成功操作
wx.hideLoading()
resolve(res.data)
},
fail: function(err) {
// 请求失败操作
wx.hideLoading()
reject(err)
}
})
})
}
// 封装网络请求
const get = (url, data) => {
return request(url, 'GET', data)
}
const post = (url, data) => {
return request(url, 'POST', data)
}
module.exports = {
get,
post
}
3. 在需要使用的页面中引入`api.js`文件,然后即可使用封装的api方法
// pages/index/index.js
import { get, post } from '../../api/api.js'
Page({
data: {},
onLoad: function () {
// 调用封装的get方法
get('/getData', {
type: 'getData'
}).then(res => {
// 请求成功操作
}).catch(err => {
// 请求失败操作
})
}
})
end;
更多推荐
所有评论(0)