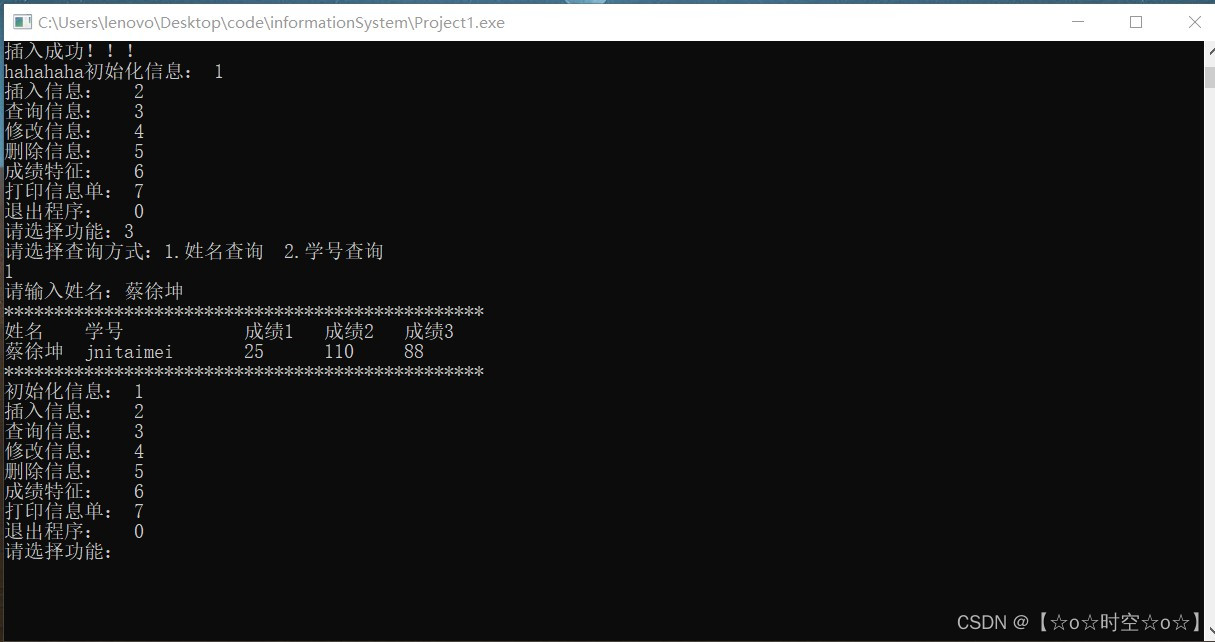
C语言学生成绩信息管理系统(超详细版)
学生信息管理系统
主题:一个简易成绩管理系统
功能介绍:
1.以文本形式存储学生信息
2.对以存信息进行 1. 插入 2. 修改3. 删除 4.查询 5.成绩数字特征
目录
一:分析
要进行 插入 查询 删除 修改 就还需要进行查询。
这些函数内部都需要调用同一种功能的函数因此
查询函数模块需要精心设计
接下来先看查询函数
在这之前确定数据结构 链式结构(链表), 顺序结构(数组)
分析:
0)要进行初始化可以链表头插法(建议带头结点优点接下来可以看到增删查改省去很多判断)
student_information *head;
head = (student_information *)malloc(sizeof(student_information));
head->next = NULL;
while (1)
{
printf("是否继续输入成绩: 1(是)/0(否) ");
scanf("%d", &judge);
if (judge == 1)
{
student_information *p;
p = (student_information *)malloc(sizeof(student_information));
if (!head->next)
{
head->next = p;
p->next = NULL;
}
else
{
p->next = head->next;
head->next = p;
}
else
{
return head;
}
}
也可以结构体数组
1)要进行插入用链表较方便方法如下:
//p为目标结点
p->next = pf->next;
pf->next = p;
数组需要进行整体向后挪移 时间复杂度大,写起来不方便
2)要进行删除链表也较方便:
pf->next = pf->next->next;
同样数组需要进行整体向后挪移 时间复杂度大,写起来不方便
3)要进行修改,查询两者相差不大
综上所述:链表较优
于是
typedef struct student_information
{
char name[15]; //姓名
char number[15]; //学号
int score[3]; //三科成绩
//还可以加入其他信息类型
struct student_information *next;
} student_information;
OK
二:查询
这是姓名进行查询
/// @brief 姓名查询函数
/// @param head
/// @param name
/// @return 返回目标前指针
student_information *inquery_name(student_information *head, char name[])
{
student_information *p = head;
while (p->next)
{
if (strcmp((p->next)->name, name) != 0)//strcmp()返回值为-+0相等为零
p = p->next;
else
return p;
}
return p;
}
下面是学号查询
/// @brief 学号查询函数
/// @param head
/// @param number
/// @return 返回目标前指针
student_information *inquery_number(student_information *head, char number[])
{
student_information *p = head;
while (p->next)
{
if (strcmp((p->next)->number, number) != 0)
p = p->next;
else
return p;
}
return p;
}
/*这两个函数可以改成QQ号,手机号等信息查询,也可以进行双重查询(将姓名QQ一起查询)只需将函数进行双重判断就行。 这两个也可以合并为一个函数因为参数都是string,但是要进行提示使用者要进行何种查询。 */
接下来分析函数
1.参数:是用链表进行的线性结构存储方式,自然参数要有头指针。 2.返回值:为目标结点的前一结点(利于插入删除,都需要前一结点较方便,上面的函数可以看出来)。 3.主体较简单,逐个比较即可。
将两函数进行合并为一个函数块
/// @brief 分为 姓名查询与学号查询
/// @param head
/// @return 返回头指针 目标信息的前指针
student_information **inquery_info(student_information *head)
{
student_information *q, *qf;
int b;
char name_inquery[15];
char number_inquery[15];
printf("请选择查询方式:1.姓名查询 2.学号查询\n");
scanf("%d", &b);
switch (b)
{
case 1:
scanf("%s", name_inquery);
qf = q = inquery_name(head, name_inquery); //接收前指针
q = q->next;
if (q)
{
printf("************************************************\n");
printf("姓名\t学号\t\t成绩1\t成绩2\t成绩3\n");
printf("%s\t%s\t%d\t%d\t%d\n", q->name, q->number, q->score[0], q->number[1], q->score[2]);
printf("************************************************\n");
}
else
printf("查询的信息不存在!!!\n");
break;
case 2:
printf("请输入学号:");
scanf("%s", number_inquery);
qf = q = inquery_number(head, number_inquery); //接收前指针
q = q->next;
if (q)
{
printf("************************************************\n");
printf("姓名\t学号\t\t成绩1\t成绩2\t成绩3\n ");
printf("%s\t%s\t%d\t%d\t%d\n", q->name, q->number, q->score[0], q->number[1], q->score[2]);
printf("************************************************\n");
}
else
printf("查询的信息不存在!!!\n");
break;
default:
printf("输入错误!!!\n");
break;
}
point[0] = head;//此处将head,和qf一并传回
point[1] = qf;
return point;
}
三:菜单
int main(void)
{
student_information *head;
student_information **temp; //临时存储头指针与目标前指针
int flag = 1;
do
{
printf("初始化信息: 1\n");
printf("插入信息: 2\n");
printf("查询信息: 3\n");
printf("修改信息: 4\n");
printf("删除信息: 5\n");
printf("成绩特征: 6\n");
printf("打印信息单: 7\n");
printf("退出程序: 0\n");
int o;
printf("请选择功能:");
scanf("%d", &o);
switch (o)
{
case 1:
head = init_info();
break;
case 2:
head = insert_info(head);
break;
case 3:
temp = inquery_info(head);
head = temp[0];
break;
case 4:
head = modify_info(head);
break;
case 5:
head = dele_info(head);
break;
case 6:
digitalFeature(head);
break;
case 7:
show_info(head);
break;
case 0:
flag = 0;
printf("************************************************\n");
printf("感谢使用!!!\n");
printf("************************************************\n");
break;
default:
printf("\n输入错误,请重新输入!!!!\n\n");
break;
}
} while (flag);
}
四:初始化函数
要求需要将信息存储进文件如下
//文件写入
FILE *fp = fopen("information.txt", "w+");
if (!fp)
{
printf("文件打开失败!!");
}
char str_1[] = "s";
fputs(p->name, fp);
fputs("\t", fp);
fputs(p->number, fp);
fputs("\t", fp);
itoa(p->score[0], str_1, 10);//integer to array 整形转化为字符串
fputs(str_1, fp);
fputs("\t", fp);
itoa(p->score[1], str_1, 10);
fputs(str_1, fp);
puts("\t", fp);
itoa(p->score[2], str_1, 10);
fputs(str_1, fp);
fputs("\n", fp);
fclose(fp);
初始化:
student_information *init_info()
{
int judge;
//文件写入
FILE *fp = fopen("information.txt", "w+");
if (!fp)
{
printf("文件打开失败!!");
}
//带头结点链表
student_information *head;
head = (student_information *)malloc(sizeof(student_information));
head->next = NULL;
while (1)
{
printf("是否继续输入成绩: 1(是)/0(否) ");
scanf("%d", &judge);
if (judge == 1)
{
student_information *p;
p = (student_information *)malloc(sizeof(student_information));
printf("请输入信息:\n姓名\t学号\t成绩1\t成绩2\t成绩3\n");
scanf("%s%s%d%d%d", p->name, p->number, &(p->score[0]), &(p->score[1]), &(p->score[2]));
if (!head->next)
{
head->next = p;
p->next = NULL;
}
else
{
p->next = head->next;
head->next = p;
}
//文件写入部分
//
}
else
{
fclose(fp);
return head;
}
}
}
五:插入函数
/// @brief 显示系统所有信息 将新信息插入到指定位序上
/// @param head
/// @return 返回新链表的头指针
student_information *insert_info(student_information *head)
{
//文件写入
int j, i;
show_info(head);
printf("请输入要插入的位序 \n");
scanf("%d", &j);
student_information *p;
p = (student_information *)malloc(sizeof(student_information));
printf("请输入信息:姓名\t学号\t成绩1\t成绩2\t成绩3\n");
scanf("%s%s%d%d%d", p->name, p->number, &(p->score[0]), &(p->score[1]), &(p->score[2]));
student_information *pf = head;
for (i = 1; i < j; i++)
{
pf = pf->next;
}
p->next = pf->next;
pf->next = p;
printf("插入成功!!!\n");
p = head->next;
//写入文件
char str_2[] = "s";
while (p)
{
//即使存入文件
//文件写入部分
//
p = p->next;
}
//fclose(fp_1);
return head;
}
六:修改函数
/// @brief 修改信息 并重新存储到文档中
/// @param head
/// @return 返回新链表的头指针
student_information *modify_info(student_information *head)
{
//文件写入
//
student_information **p;
student_information *q;
p = inquery_info(head); //接受返回的头指针与目标前指针
q = p[1]; //接收目标指针
q = q->next; //移动到目标指针
if (q == NULL)
{
printf("!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!");
printf("输入信息错误,信息不存在!!\n");
}
else
{
int flag = 1;
while (flag)
{
int s;
printf("请选择要修改的信息:\n1.姓名, 2.学号, 3.成绩1, 4.成绩2, 5.成绩3, 0.不修改退出\n");
scanf("%d", &s);
switch (s)
{
case 1:
printf("请输入新姓名\n");
scanf("%s", q->name);
printf("修改成功!!!!\n");
break;
case 2:
printf("请输入新学号\n");
scanf("%s", q->number);
printf("修改成功!!!!\n");
break;
case 3:
printf("请输入新成绩1\n");
scanf("%d", &(q->score[0]));
printf("修改成功!!!!\n");
break;
case 4:
printf("请输入新成绩2\n");
scanf("%d", &(q->score[0]));
printf("修改成功!!!!\n");
break;
case 5:
printf("请输入新成绩3\n");
scanf("%d", &(q->score[0]));
printf("修改成功!!!!\n");
break;
case 0:
flag = 0;
break;
default:
printf("请重新输入!!\n");
break;
}
}
}
student_information *p_2;
p_2 = head->next;
char str_3[] = "j";
//写入文件
while (p_2)
{
//
p_2 = p_2->next;
}
//fclose(fp_2);
return p[0]; //返回头指针
}
七:删除函数
/// @brief 删除信息 并重新存储到文档中
/// @param head
/// @return 返回新链表的头指针
student_information *dele_info(student_information *head)
{
//文件写入
student_information **p;
student_information *q;
p = inquery_info(head); //接受返回的头指针与目标前指针
q = p[1]; //接收目标指针的前指针 不移动到目标指针利于删除
if ((q->next) != NULL)
{
q->next = q->next->next;
printf("删除成功!!!!\n");
}
else
{
printf("输入信息错误,信息不存在!!\n");
}
head = p[0]; //接收头指针
student_information *p_3;
p_3 = head->next;
char str_4[] = "j";
//写入文件
while (p_3)
{
//
p_3 = p_3->next;
}
//fclose(fp_3);
return head; //返回新链表的头指针
}
八:数字特征
自由发挥部分
/// @brief 显示平均分 最高分 不及格人数 优秀率
/// @param head
void digitalFeature(student_information *head)
{
int h;
student_information *sco = head->next;
int total[subjectTotal] = {0}; //临时变量 存分数之和
double average[subjectTotal]; //平均分
int max_score[subjectTotal] = {0}; //最高分
double people = 0.0; //总人数
int people_fail[subjectTotal] = {0}; //三科不及格人数
int people_good[subjectTotal] = {0}; //优秀人数
double goodRate[subjectTotal] = {0}; //三科优秀率
while (sco != NULL)
{
people += 1;
for (h = 0; h < subjectTotal; h++)
{
total[h] += sco->score[h];
if (sco->score[h] < 60)
people_fail[h]++;
if (sco->score[h] >= 85)
people_good[h]++;
if (sco->score[h] > max_score[h])
max_score[h] = sco->score[h];
}
sco = sco->next;
}
for (h = 0; h < subjectTotal; h++)
{
average[h] = total[h] / people;
goodRate[h] = people_good[h] / people;
}
printf("**********************************************************************\n");
printf(" 成绩数字特征 \n");
for (h = 0; h < subjectTotal; h++)
{
printf("科目%d\t平均分%6.2f\t最高分%d\t不及格人数%d\t优秀率%6.2f\n", h + 1, average[h], max_score[h], people_fail[h], goodRate[h]);
}
printf("**********************************************************************\n");
}
九:显示函数
/// @brief 显示全部信息
/// @param head
void show_info(student_information *head)
{
student_information *p = head->next;
int i = 1;
printf("************************************************\n");
printf("序号\t姓名\t学号\t\t成绩\t成绩\t成绩\n ");
while (p)
{
printf("%d\t%s\t%s\t%d\t%d\t%d\n", i, p->name, p->number, p->score[0], p->number[1], p->score[2]);
p = p->next;
i++;
}
printf("************************************************\n");
}
十:运行结果
菜单
初始化
插入
查询
修改
删除
就到这了!!!!!!
内容仅供自己学习记录,欢迎指正!!!!感谢!!!!
更多推荐
所有评论(0)