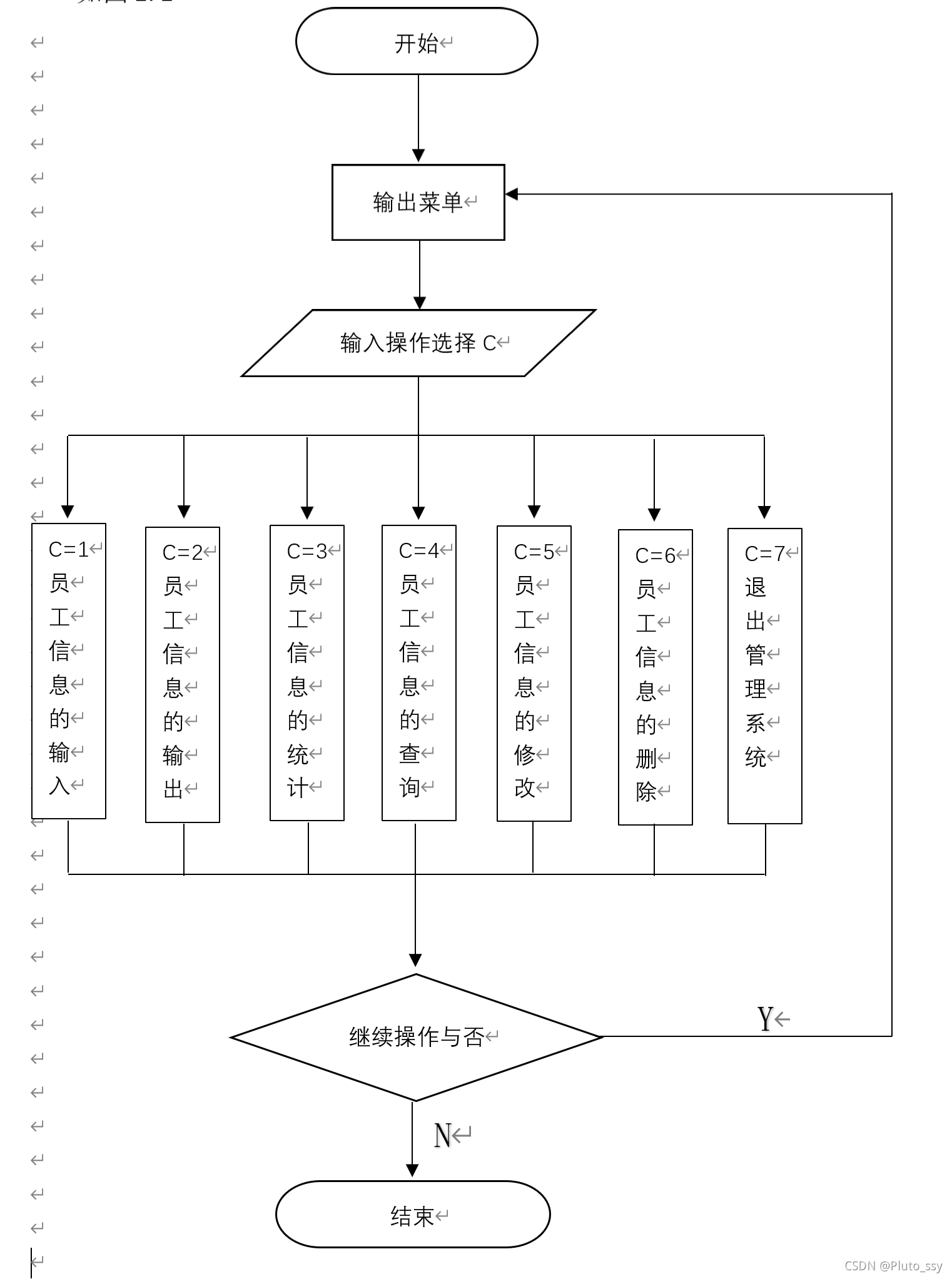
C++实现员工信息管理系统
一、问题描述及要求设计题目员工信息管理系统成绩课程设计主要内容【问题描述】编写一个程序来管理员工信息。通过一个类来存放输入的每一位员工的记录(包括员工号、姓名、性别、所属部门、职称、入职时间、联系电话等),然后将其信息保存到文件中。通过几个函数来创建新员工号,并对职工信息进行删除及修改,输入一...
·
一、问题描述及要求
设计题目 | 员工信息管理系统 | 成绩 |
|
课 程 设 计 主 要 内 容 | 【问题描述】 编写一个程序来管理员工信息。通过一个类来存放输入的每一位员工的记录(包括员工号、姓名、性别、所属部门、职称、入职时间、联系电话等),然后将其信息保存到文件中。通过几个函数来创建新员工号,并对职工信息进行删除及修改,输入一个员工号查询该职工的信息,并显示在屏幕上。 【任务要求】 运用类的思想来实现员工信息管理系统的编写。实现以下操作:
|
二、运营环境及开发工具
1、C语言以及C++语言
2、vc++6.0
3、Dev-C++
三、流程图
1、系统流程图
2、主函数流程图
3、具体函数流程图略
很好画的,可以参考上面的流程图。
四、实现代码
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
class staff
{
private:
string employee_number;//职工号
string name;//姓名
int age;//年龄
string sex;//性别
string zip_code;//职称
string department;//部门
string time; //入职时间
string tel; //联系电话
class staff* next;
public:
staff():next(NULL){}
void registration(class staff* head);//注册 尾插
void query_employeenumber(class staff* t);//按员工号查询
void query_name(class staff* t);//按照姓名查询员工信息
void query_tel(class staff* t);//按照联系电话查询员工信息
void delete_num(class staff* t);//按工号删除员工信息
void delete_name(class staff* t);//按姓名删除员工信息
void show_all(class staff* head);//输出所有员工信息
void query_sex(class staff* t);
void update(class staff* head);
void update_num(class staff* head);//修改工号
void update_name(class staff* head);//修改姓名
void update_age(class staff* head);//修改年龄
void update_sex(class staff* head);//修改性别
void update_zip(class staff* head);//修改职称
void update_depart(class staff* head);//修改部门
void update_time(class staff* head);//修改入职时间
void update_tel(class staff* head);//修改联系电话
void update_all(class staff* head);//修改全部
int read(class staff* head);
void write(class staff* t);
};
void staff::registration(class staff* head)//注册 尾插
{
while(head->next != NULL)
{
head = head->next;
}
staff* newnode = new staff;
head->next = newnode;
newnode->next = NULL;
cout << "请输入员工信息" << endl;
cout << "员工号: " << endl;
cin >> newnode->employee_number;
cout << "员工姓名: " << endl;
cin >> newnode->name;
cout << "员工年龄: " << endl;
cin >> newnode->age;
cout << "职工性别: " << endl;
cin >> newnode->sex;
cout << "员工职称: " << endl;
cin >> newnode->zip_code;
cout << "员工部门: " << endl;
cin >> newnode->department;
cout<<"员工入职时间: "<<endl;
cin >> newnode->time;
cout<<"员工联系电话: "<<endl;
cin >> newnode->tel;
}
void staff::show_all(class staff* head)//输出
{
int i = 0;
while(head->next != NULL)
{
i++;
cout << i << "." << "工号:" ;
cout << head->next->employee_number <<'\t';
cout << "名字:" ;
cout << head->next->name <<'\t';
cout << "性别:" ;
cout << head->next->sex <<'\t';
cout << "年龄:" ;
cout << head->next->age <<'\t';
cout << "职称:" ;
cout << head->next->zip_code << '\t';
cout << "部门:" ;
cout << head->next->department << '\t';
cout << "入职时间:" ;
cout << head->next->time << '\t';
cout << "联系电话:" ;
cout << head->next->tel << endl;
head = head->next;
}
}
void staff::query_employeenumber(class staff* t)//按员工工号查询
{
string n;
cout << "请输入你想查询员工工号:" << endl;
cin >> n;
int count = 0;
while(t->next != NULL)
{
if(t->next->employee_number == n)
{
cout << "工号:" ;
cout << t->next->employee_number <<'\t';
cout << "名字:";
cout << t->next->name <<'\t';
cout << "性别:" ;
cout << t->next->sex <<'\t';
cout << "年龄:" ;
cout << t->next->age <<'\t';
cout << "邮编:" ;
cout << t->next->zip_code << '\t';
cout << "部门:";
cout << t->next->department << '\t';
cout << "入职时间:" ;
cout << t->next->time << '\t';
cout << "联系电话:" ;
cout << t->next->tel << endl;
count++;
}
t = t->next;
}
if(count == 0)
{
cout << "查无此人!" << endl;
}
}
void staff::query_name(class staff* t)//按员工姓名查询
{
string n;
cout << "请输入你想查询员工姓名:" << endl;
cin >> n;
int count = 0;
while(t->next != NULL)
{
if(t->next->name == n)
{
cout << "工号:" ;
cout << t->next->employee_number <<'\t';
cout << "名字:";
cout << t->next->name <<'\t';
cout << "性别:" ;
cout << t->next->sex <<'\t';
cout << "年龄:" ;
cout << t->next->age <<'\t';
cout << "职称:" ;
cout << t->next->zip_code << '\t';
cout << "部门:";
cout << t->next->department << '\t';
cout << "入职时间:" ;
cout << t->next->time << '\t';
cout << "联系电话:" ;
cout << t->next->tel << endl;
count++;
}
t = t->next;
}
if(count == 0)
{
cout << "查无此人!" << endl;
}
}
void staff::query_tel(class staff* t)//按员工联系电话查询
{
string n;
cout << "请输入你想查询联系电话:" << endl;
cin >> n;
int count = 0;
while(t->next != NULL)
{
if(t->next->tel == n)
{
cout << "工号:" ;
cout << t->next->employee_number <<'\t';
cout << "名字:";
cout << t->next->name <<'\t';
cout << "性别:" ;
cout << t->next->sex <<'\t';
cout << "年龄:" ;
cout << t->next->age <<'\t';
cout << "邮编:" ;
cout << t->next->zip_code << '\t';
cout << "部门:";
cout << t->next->department << '\t';
cout << "入职时间:" ;
cout << t->next->time << '\t';
cout << "联系电话:" ;
cout << t->next->tel << endl;
count++;
}
t = t->next;
}
if(count == 0)
{
cout << "查无此人!" << endl;
}
}
void staff::delete_num(class staff* t)//按员工号删除
{
string n;
cout << "请输入你想删除员工工号:" << endl;
cin >> n;
int count = 0;
while(t->next != NULL)
{
if(t->next->employee_number == n)
{
staff* temp = t->next;
t->next = t->next->next;
delete temp;
temp = NULL;
cout << "删除成功!" << endl;
count++;
break;
}
t = t->next;
}
if(count == 0)
{
cout << "查无此人!" << endl;
}
}
void staff::delete_name(class staff* t)//按员工姓名删除
{
string n;
cout << "请输入你想删除员工的姓名:" << endl;
cin >> n;
int count = 0;
while(t->next != NULL)
{
if(t->next->name == n)
{
staff* temp = t->next;
t->next = t->next->next;
delete temp;
temp = NULL;
cout << "删除成功!" << endl;
count++;
break;
}
t = t->next;
}
if(count == 0)
{
cout << "查无此人!" << endl;
}
}
void staff::update(class staff* head)
{
int num = 0;
cout << "1.修改员工号" << endl;
cout << "2.修改员工姓名" << endl;
cout << "3.修改员工年龄" << endl;
cout << "4.修改员工性别" << endl;
cout << "5.修改员工职称" << endl;
cout << "6.修改员工部门" << endl;
cout << "7.修改员工入职时间" << endl;
cout << "8.修改员工联系电话" << endl;
cout << "9.修改全部" << endl;
cin >> num;
switch(num)
{
case 1:
update_num(head);
break;
case 2:
update_name(head);
break;
case 3:
update_age(head);
break;
case 4:
update_sex(head);
break;
case 5:
update_zip(head);
break;
case 6:
update_depart(head);
break;
case 7:
update_time(head);
break;
case 8:
update_tel(head);
case 9:
update_all(head);
break;
default:
cout << "请重新输入正确的指令!" << endl;
}
}
void staff::query_sex(class staff* t)//统计
{
string n;
cout << "请输入你要统计的员工的姓别(男或女):" << endl;
cin >> n;
int count = 0;
while(t->next != NULL)
{
if(t->next->sex == n)
{
count++;
break;
}
t = t->next;
}
cout << "共有"<<n<<"员工: " <<count<<"个"<< endl;
}
void staff::update_num(class staff* head)//修改工号
{
cout << "输入要修改对象的工号:"<< endl;
int count = 0;
string temp;
cin >> temp;
while(head->next != NULL)
{
if(temp == head->next->employee_number)
{
count = 1;
cout<< "输入新工号:"<< endl;
string temp2;
cin>>temp2;
head->next->employee_number = temp2;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
void staff::update_name(class staff* head)//修改姓名
{
cout << "输入要修改对象的工号:"<< endl;
int count = 0;
string temp;
cin >> temp;
while(head->next != NULL)
{
if(temp == head->next->employee_number)
{
count = 1;
cout << "输入新名字:"<< endl;
string temp2;
cin >> temp2;
head->next->name = temp2;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
void staff::update_age(class staff* head)//修改年龄
{
cout << "输入要修改对象的工号:"<< endl;
int count = 0;
string temp;
cin >> temp;
while(head->next != NULL)
{
if(temp == head->next->employee_number)
{
count = 1;
cout << "输入新年龄:"<< endl;
int temp2;
cin >> temp2;
head->next->age = temp2;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
void staff::update_sex(class staff* head)//修改性别
{
cout << "输入要修改对象的工号:"<< endl;
int count = 0;
string temp;
cin >> temp;
while(head->next != NULL)
{
if(temp == head->next->employee_number)
{
count = 1;
cout << "输入新性别:"<< endl;
string temp2;
cin >> temp2;
head->next->sex = temp2;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
void staff::update_zip(class staff* head)//修改职称
{
cout << "输入要修改对象的工号:"<< endl;
int count = 0;
string temp;
cin >> temp;
while(head->next != NULL)
{
if(temp == head->next->employee_number)
{
count = 1;
cout << "输入职称:"<< endl;
string temp2;
cin >> temp2;
head->next->zip_code = temp2;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
void staff::update_depart(class staff* head)//修改部门
{
cout << "输入要修改对象的工号:"<< endl;
int count = 0;
string temp;
cin >> temp;
while(head->next != NULL)
{
if(temp == head->next->employee_number)
{
count = 1;
cout << "输入新部门:"<< endl;
string temp2;
cin >> temp2;
head->next->department = temp2;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
void staff::update_time(class staff* head)//修改入职时间
{
cout << "输入要修改对象的工号:"<< endl;
int count = 0;
string temp;
cin >> temp;
while(head->next != NULL)
{
if(temp == head->next->employee_number)
{
count = 1;
cout << "输入新入职时间:"<< endl;
int temp2;
cin >> temp2;
head->next->time = temp2;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
void staff::update_tel(class staff* head)//修改联系电话
{
cout << "输入要修改对象的工号:"<< endl;
int count = 0;
string temp;
cin >> temp;
while(head->next != NULL)
{
if(temp == head->next->employee_number)
{
count = 1;
cout << "输入新入职时间:"<< endl;
int temp2;
cin >> temp2;
head->next->tel = temp2;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
void staff::update_all(class staff* head)//修改全部
{
cout<<"输入要修改对象的工号:"<<flush;
int count = 0;
string tmp;
cin >> tmp;
while(head->next != NULL)
{
if(tmp == head->next->employee_number)
{
count = 1;
cout << "输入新工号:"<< endl;
string temp;
int tmp;
cin >> temp;
head->next->employee_number = temp;
cout<< "输入新名字:"<< endl;
cin >> temp;
head->next->name = temp;
cout<< "输入新性别:"<< endl;
cin >> temp;
head->next->sex = temp;
cout<< "输入新年龄:"<< endl;
cin >> tmp;
head->next->age = tmp;
cout<< "输入新职称:"<< endl;
cin >> temp;
head->next->zip_code = temp;
cout<< "输入新部门:"<< endl;
cin >> temp;
head->next->department = temp;
cout<< "输入新入职时间:"<< endl;
cin >> temp;
head->next->time = tmp;
cout<< "输入新入联系电话:"<< endl;
cin >> temp;
head->next->tel = tmp;
cout << "修改成功" << endl;
break;
}
head = head->next;
}
if(count == 0)
{
cout << "查无此人" << endl;
}
}
int staff::read(class staff* head)
{
ofstream infile1;
infile1.open("员工系统.txt",ios::app);
if(!infile1)
{
return 0;
}
else
{
infile1.close();
ifstream infile;
infile.open("员工系统.txt",ios::in);
while(!infile.eof())
{
staff* newnode = new staff;
infile >> newnode->employee_number;
if(newnode->employee_number.length() == 0)
{
delete newnode;
break;
}
infile >> newnode->name;
infile >> newnode->sex;
infile >> newnode->age;
infile >> newnode->zip_code;
infile >> newnode->department;
infile >> newnode->time;
infile >> newnode->tel;
head->next = newnode;
head = head->next;
}
infile.close();
}
return 0;
}
void staff::write(class staff* t)
{
ofstream outfile;
outfile.open("员工系统.txt",ios::out);
while(t->next != NULL)
{
outfile << t->next->employee_number << '\t';
outfile << t->next->name << '\t';
outfile << t->next->sex << '\t';
outfile << t->next->age << '\t';
outfile << t->next->zip_code << '\t';
outfile << t->next->department << '\t';
outfile << t->next->time << '\t';
outfile << t->next->tel << endl;
t = t->next;
}
outfile.close();
}
int main()
{
staff* head = new staff;
head->read(head);
string temp;
cout << "********欢迎来到员工管理系统!********" << endl;
while(1)
{
cout << "------------------------------------" << endl;
head->show_all(head);
cout << "------------------------------------" << endl;
cout << endl;
cout << "1.输入员工信息" << endl;
cout << "2.输出员工信息" << endl;
cout << "3.按员工工号查询员工信息"<< endl;
cout << "4.按照姓名查询员工信息" << endl;
cout << "5.按照联系电话查询员工信息" << endl;
cout << "6.按工号删除员工信息" << endl;
cout << "7.按姓名删除员工信息" << endl;
cout << "8.统计员工个数" << endl;
cout << "9.修改员工信息" << endl;
cout << "0.退出" << endl;
cout << endl;
cout << "请输入你想选择的功能:" << endl;
int number;
cin >> number;
switch(number)
{
case 1:
{
head->registration(head);
break;
}
case 2:
{head->show_all(head);
break;
}
case 3:
{
head->query_employeenumber(head);
break;
}
case 4:
{
head->query_name(head);
break;
}
case 5:
{
head->query_tel(head);
break;
}
case 6:
{
head->delete_num(head);
break;
}
case 7:
{
head->delete_name(head);
break;
}
case 8:
{
head->query_sex(head);
break;
}
case 9:
{
head->update(head);
break;
}
case 0:
{
head->write(head);
return 0;
}
default: cout << "请重新输入正确的操作指令" << endl;
}
}
}
五、结果举例
更多推荐
所有评论(0)