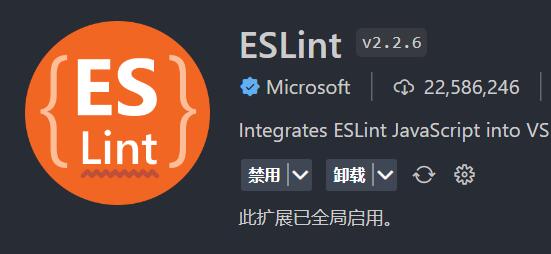
vue3 + vite + JS 配置eslint自动验证
vue3 + vite + JS 配置eslint自动验证
·
1、创建项目 这里是已经安装过yarn 和vite的
执行命令: yarn create vite
? Project name: 输入你的项目名称?(如: esvue)
? Select a framework: 选择安装的脚手架 (这里选vue)
vanilla
> vue
react
Done. Now run:
cd esvue
yarn
yarn dev
2、安装Eslint
yarn add -D eslint
3、初始化配置EsLint
npx eslint --init
3.1、选择模式: (To check syntax and find problems)
You can also run this command directly using 'npm init @eslint/config'.
? How would you like to use ESLint? ...
To check syntax only
> To check syntax and find problems
To check syntax, find problems, and enforce code style
3.2、选择语言模块: (选JavaScript modules)
? What type of modules does your project use? ...
> JavaScript modules (import/export)
CommonJS (require/exports)
None of these
3.3、选择语言框架 (选Vue.js)
? Which framework does your project use? ...
React
> Vue.js
None of these
3.4、是否使用ts (视自己情况而定,我这里不用选No)
? Does your project use TypeScript? » No / Yes
3.5代码在哪里运行 (用空格选中 Browser+Node)
? Where does your code run? ... (Press <space> to select, <a> to toggle all, <i> to invert selection)
√ Browser
√ Node
3.6、您希望您的配置文件是什么格式? (选JavaScript)
? What format do you want your config file to be in? ...
> JavaScript
YAML
JSON
3.7、您想现在安装它们吗? (选择Yes)
? Would you like to install them now? » No / Yes
3.8、您要使用哪个软件包管理器? (选择yarn)
? Which package manager do you want to use? ...
npm
> yarn
pnpm
4、安装完成后 (在项目根目录会出现.eslintrc.cjs文件) 直接把文件拷过去安装必要的包同样起效果
// .eslintrc.cjs 文件
// 这里就是上面3步骤初始化的文件,后面的配置都写这里
module.exports = {
env: {
browser: true,
es2021: true,
node: true
},
extends: "eslint:recommended",
parserOptions: {
ecmaVersion: 'latest',
sourceType: 'module'
},
rules: {
}
}
4.1 创建.eslintignore文件,配置不需要eslint检验的文件
# eslint检验排除的文件
/public
**/Cesium.js
5、继续安装 vite-plugin-eslint
// 说明: 该包是用于配置vite运行的时候自动检测eslint规范
// 问题: 不装这个包可以吗? 答案是“可以的”,使用yarn dev时并不会主动检查代码
yarn add -D vite-plugin-eslint
6、继续安装 eslint-parser
yarn add -D @babel/core
yarn add -D @babel/eslint-parser
7、继续安装prettier (用于规范代码格式,不需要可以不装---我没装)
yarn add -D prettier
yarn add -D eslint-config-prettier // eslint兼容的插件
yarn add -D eslint-plugin-prettier // eslint的prettier
8、配置 vite.config.js
import { defineConfig } from 'vite'
import vue from '@vitejs/plugin-vue'
import eslintPlugin from 'vite-plugin-eslint' //导入包
export default defineConfig({
plugins: [
vue(),
// 增加下面的配置项,这样在运行时就能检查eslint规范
eslintPlugin({
include: ['src/**/*.js', 'src/**/*.vue', 'src/*.js', 'src/*.vue']
})
]
})
9、配置 .prettierrc
如果您没有安装第7步骤的那三个包,这个配置你可以忽略跳过
这里主要配置代码的格式规范的,有些设置要与.eslintrc.js配置一致,防止冲突
// 在项目根目录创建文件 .prettierrc
// 以下配置视自己情况而定,并步是每个都需要的
{
tabWidth: 4, // 使用4个空格缩进
semi: false, // 代码结尾是否加分号
trailingComma: 'none', // 代码末尾不需要逗号
singleQuote: true, // 是否使用单引号
printWidth: 100, // 超过多少字符强制换行
arrowParens: 'avoid', // 单个参数的箭头函数不加括号 x => x
bracketSpacing: true, // 对象大括号内两边是否加空格 { a:0 }
endOfLine: 'auto', // 文件换行格式 LF/CRLF
useTabs: false, // 不使用缩进符,而使用空格
quoteProps: 'as-needed', // 对象的key仅在必要时用引号
jsxSingleQuote: false, // jsx不使用单引号,而使用双引号
jsxBracketSameLine: false, // jsx标签的反尖括号需要换行
rangeStart: 0, // 每个文件格式化的范围是文件的全部内容
rangeEnd: Infinity, // 结尾
requirePragma: false, // 不需要写文件开头的 @prettier
insertPragma: false, // 不需要自动在文件开头插入 @prettier
proseWrap: 'preserve', // 使用默认的折行标准
htmlWhitespaceSensitivity: 'css' // 根据显示样式决定html要不要折行
}
10、配置 .eslintrc.cjs 根据自己的习惯和要求进行配置检查警告或错误
module.exports = {
"env": {
"browser": true,
"es2021": true,
"node": true
},
"extends": [
"eslint:recommended", // 使用推荐的eslint
'plugin:vue/vue3-recommended' // 使用插件支持vue3
// 如果你没有安装第7步,以下两个包不要引入,否则报错
'plugin:prettier/recommended',
'eslint-config-prettier'
],
"parserOptions": {
"ecmaVersion": 13,
"sourceType": "module",
"ecmaFeatures": {
"modules": true,
'jsx': true
},
"requireConfigFile": false,
"parser": '@babel/eslint-parser'
},
// eslint-plugin-vue
'plugins': [
'vue' // 引入vue的插件 vue <==> eslint-plugin-vue
// 这个包需要安装了第7步的三个包再引入
'prettier' // 引入规范插件 prettier <==> eslint-plugin-prettier
],
'globals': {
defineProps: 'readonly',
defineEmits: 'readonly',
defineExpose: 'readonly',
withDefaults: 'readonly'
},
// 这里时配置规则的,自己看情况配置
"rules": {
'semi': ['warn', 'never'], // 禁止尾部使用分号
'no-console': 'warn', // 禁止出现console
'no-debugger': 'warn', // 禁止出现debugger
'no-duplicate-case': 'warn', // 禁止出现重复case
'no-empty': 'warn', // 禁止出现空语句块
'no-extra-parens': 'warn', // 禁止不必要的括号
'no-func-assign': 'warn', // 禁止对Function声明重新赋值
'no-unreachable': 'warn', // 禁止出现[return|throw]之后的代码块
'no-else-return': 'warn', // 禁止if语句中return语句之后有else块
'no-empty-function': 'warn', // 禁止出现空的函数块
'no-lone-blocks': 'warn', // 禁用不必要的嵌套块
'no-multi-spaces': 'warn', // 禁止使用多个空格
'no-redeclare': 'warn', // 禁止多次声明同一变量
'no-return-assign': 'warn', // 禁止在return语句中使用赋值语句
'no-return-await': 'warn', // 禁用不必要的[return/await]
'no-self-compare': 'warn', // 禁止自身比较表达式
'no-useless-catch': 'warn', // 禁止不必要的catch子句
'no-useless-return': 'warn', // 禁止不必要的return语句
'no-mixed-spaces-and-tabs': 'warn', // 禁止空格和tab的混合缩进
'no-multiple-empty-lines': 'warn', // 禁止出现多行空行
'no-trailing-spaces': 'warn', // 禁止一行结束后面不要有空格
'no-useless-call': 'warn', // 禁止不必要的.call()和.apply()
'no-var': 'warn', // 禁止出现var用let和const代替
'no-delete-var': 'off', // 允许出现delete变量的使用
'no-shadow': 'off', // 允许变量声明与外层作用域的变量同名
'dot-notation': 'warn', // 要求尽可能地使用点号
'default-case': 'warn', // 要求switch语句中有default分支
'eqeqeq': 'warn', // 要求使用 === 和 !==
'curly': 'warn', // 要求所有控制语句使用一致的括号风格
'space-before-blocks': 'warn', // 要求在块之前使用一致的空格
'space-in-parens': 'warn', // 要求在圆括号内使用一致的空格
'space-infix-ops': 'warn', // 要求操作符周围有空格
'space-unary-ops': 'warn', // 要求在一元操作符前后使用一致的空格
'switch-colon-spacing': 'warn', // 要求在switch的冒号左右有空格
'arrow-spacing': 'warn', // 要求箭头函数的箭头前后使用一致的空格
'array-bracket-spacing': 'warn', // 要求数组方括号中使用一致的空格
'brace-style': 'warn', // 要求在代码块中使用一致的大括号风格
'camelcase': 'warn', // 要求使用骆驼拼写法命名约定
'indent': ['warn', 4], // 要求使用JS一致缩进4个空格
'max-depth': ['warn', 4], // 要求可嵌套的块的最大深度4
'max-statements': ['warn', 100], // 要求函数块最多允许的的语句数量20
'max-nested-callbacks': ['warn', 3], // 要求回调函数最大嵌套深度3
'max-statements-per-line': ['warn', { max: 1 }], // 要求每一行中所允许的最大语句数量
"quotes": ["warn", "single", "avoid-escape"], // 要求统一使用单引号符号
"vue/require-default-prop": 0, // 关闭属性参数必须默认值
"vue/singleline-html-element-content-newline": 0, // 关闭单行元素必须换行符
"vue/multiline-html-element-content-newline": 0, // 关闭多行元素必须换行符
// 要求每一行标签的最大属性不超五个
'vue/max-attributes-per-line': ['warn', { singleline: 5 }],
// 要求html标签的缩进为需要4个空格
"vue/html-indent": ["warn", 4, {
"attribute": 1,
"baseIndent": 1,
"closeBracket": 0,
"alignAttributesVertically": true,
"ignores": []
}],
// 取消关闭标签不能自闭合的限制设置
"vue/html-self-closing": ["error", {
"html": {
"void": "always",
"normal": "never",
"component": "always"
},
"svg": "always",
"math": "always"
}]
}
}
11、配置VScode
1、禁用 vue2 代码检查插件 Vetur
2、安装 vue3 代码检查插件 Vue Language Features (Volar)
3、安装 “ESLint” 插件【作者: Microsoft】
4、安装“Prettier ESLint”插件 【作者:Rebecca Vest】-- 选装
// 配置vscode
// 打开:设置 -> 文本编辑器 -> 字体 -> 在 settings.json 中编辑
// settings.json文件
{
// 每次保存的时候自动格式化--按照eslint配置格式进行修复
"editor.codeActionsOnSave": {
"source.fixAll.eslint": true
},
// #让prettier使用eslint的代码格式进行校验 --选填
"prettier.eslintIntegration": true
12、其它 (整个流程下来安装的包)
"devDependencies": {
"@babel/core": "^7.19.3",
"@babel/eslint-parser": "^7.19.1",
"@vitejs/plugin-vue": "^3.1.0",
"eslint": "^8.25.0",
"eslint-plugin-vue": "^9.6.0",
"vite": "^3.1.0",
"vite-plugin-eslint": "^1.8.1"
}
13、寄语
个人不太喜欢prettier, 有时候还是喜欢按照自己的格式直接在.eslintrc.cjs配置是同样的效果。
比如说有很多配置项,我需要在每个配置加上注释,并且让注释对齐,如果你使用了prettier你的注释是没办法对齐的,会自动缩回去
参考:
vue3 - Vite + Vue3 + EsLint + Prettier 超简单配置步骤_个人文章 - SegmentFault 思否
更多推荐
所有评论(0)