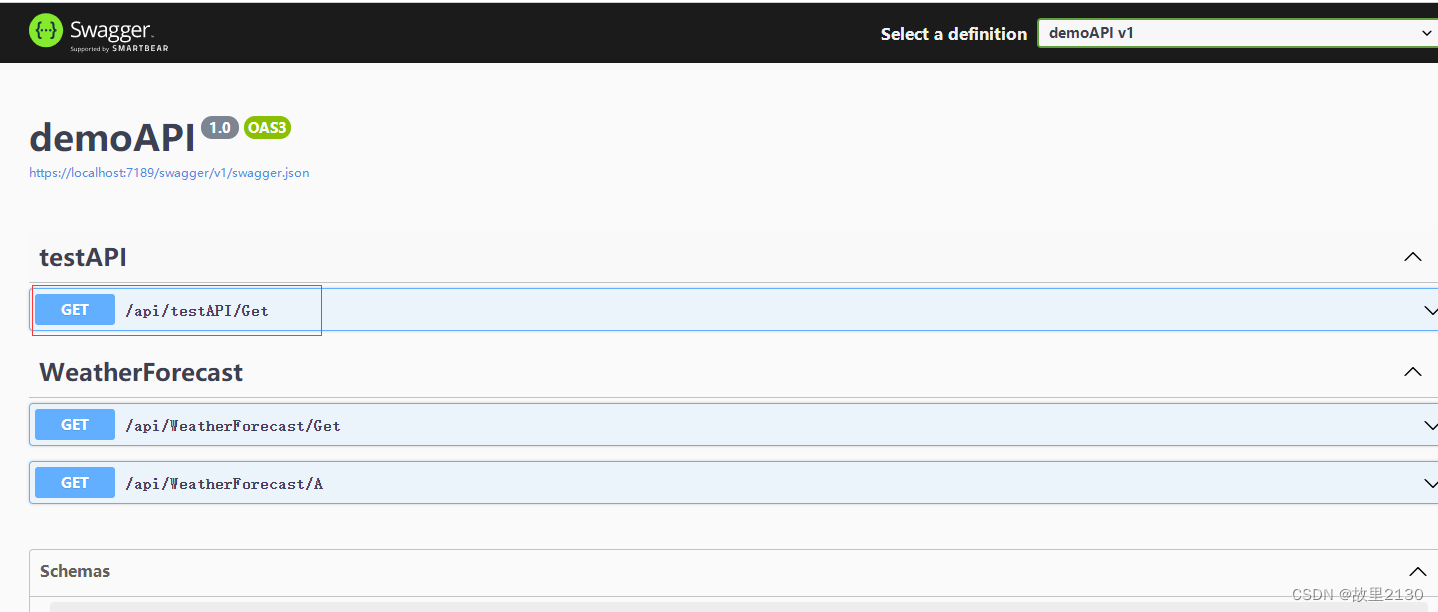
.net6 web api中使用SqlSugar(MySQL数据库)
其中SqlSugar,也可以是EFcore,或者Dapper,或者其他ORM框架。其中mysql,也可以是SqlServer,或者oracle,或者其他数据库类型。1.首先使用vs2022建立.net6 web api2.增加SqlSugar和MySQL依赖项。Newtonsoft.Json是序列化 3. 根据官网说明进行注入SqlSugar.IOC/依赖注入 - SqlSugar 5x - .N
·
其中SqlSugar,也可以是EFcore,或者Dapper,或者其他ORM框架。
其中mysql,也可以是SqlServer,或者oracle,或者其他数据库类型。
1.首先使用vs2022建立.net6 web api
2.增加SqlSugar和MySQL依赖项。
Newtonsoft.Json是序列化
3. 根据官网说明进行注入
SqlSugar.IOC/依赖注入 - SqlSugar 5x - .NET果糖网
using SqlSugar;
namespace demoAPI.Db
{
public static class SqlsugarSetup
{
public static void AddSqlsugarSetup(this IServiceCollection services, IConfiguration configuration,
string dbName = "ConnectString")
{
SqlSugarScope sqlSugar = new SqlSugarScope(new ConnectionConfig()
{
DbType = SqlSugar.DbType.MySql,
ConnectionString = configuration[dbName],
IsAutoCloseConnection = true,
},
db =>
{
//单例参数配置,所有上下文生效
db.Aop.OnLogExecuting = (sql, pars) =>
{
Console.WriteLine(sql);//输出sql
};
//技巧:拿到非ORM注入对象
//services.GetService<注入对象>();
});
services.AddSingleton<ISqlSugarClient>(sqlSugar);//这边是SqlSugarScope用AddSingleton
}
}
}
注入操作
builder.Services.AddSqlsugarSetup(builder.Configuration);
其中ConnectString就是MySQL数据库的连接字符串
"ConnectString": "Server=127.0.0.1;Port=3306;Database=test;Uid=root;Pwd=123456;"
4. 建立实体类
这个要和数据库的一致
注意: 建立实体类,可以使用DBfirst,也可以使用codefirst,也可以手动,最终只要有实体就行了。
4. 建立测试控制类
using demoAPI.Model;
using Microsoft.AspNetCore.Mvc;
using SqlSugar;
namespace demoAPI.Controllers
{
[ApiController]
[Route("api/[controller]/[action]")]
public class testAPI : ControllerBase
{
private readonly ISqlSugarClient db;
public testAPI(ISqlSugarClient db)
{
this.db = db;
}
[HttpGet]
public void Get()
{
var a = db.Queryable<student>().ToList();
var b = db.Queryable<student>().Where(a => a.id == "22").ToList();
}
}
}
5.运行代码,看结果
点击第一个执行。
拓展:数据返回类型
对webapi操作的时候,会有返回的数据,返回的数据各有不同,有集合,有单体,有数值,有字节流等等方式。也可以对他们进行统一的封装,进行标识,后面将会写,下面的代码,目前可以进行参考一下。
using demoAPI.Model;
using Microsoft.AspNetCore.Mvc;
using SqlSugar;
namespace demoAPI.Controllers
{
[ApiController]
[Route("api/[controller]/[action]")]
public class testAPI : ControllerBase
{
private readonly ISqlSugarClient db;
public testAPI(ISqlSugarClient db)
{
this.db = db;
}
[HttpGet]
public void Get()
{
var a = db.Queryable<student>().ToList();
var b = db.Queryable<student>().Where(a => a.id == "22").ToList();
}
/// <summary>
/// 返回所有数据
/// </summary>
/// <returns></returns>
[HttpGet]
public async Task<ActionResult<IEnumerable<student>>> AAA()
{
return await db.Queryable<student>().ToListAsync();
}
/// <summary>
/// 返回单条数据
/// </summary>
/// <returns></returns>
[HttpGet("{id}")]
public async Task<ActionResult<IEnumerable<student>>> AAA(string id)
{
var data = await db.Queryable<student>().Where(s => s.id == id).ToListAsync();
return data;
}
/// <summary>
/// 返回体单个数值
/// </summary>
/// <returns></returns>
[HttpGet]
public ActionResult<string> B( )
{
return "12231";
}
/// <summary>
/// 返回状态码
/// </summary>
/// <returns></returns>
[HttpGet]
public IActionResult B1()
{
return NotFound();
}
}
}
更多推荐
所有评论(0)