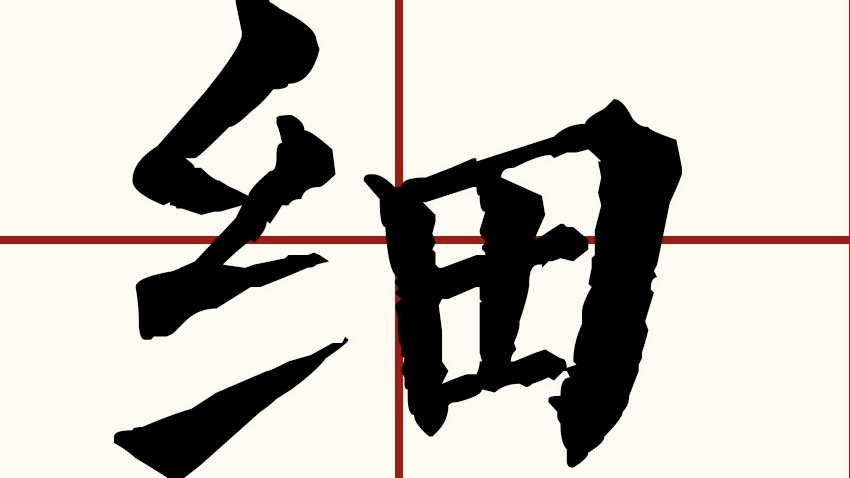
Cascader 级联选择器(三级)
Cascader 级联选择器(三级)
·
业务场景
点击分组id=1修改,获得当前行的所有信息,其中所属分类id是用来控制*所属分类
这里是通过点击修改按钮获取此时的
一个分类可以有多个属性数组,一个属性分组可以有多个属性
*所属分类这里v-model绑定,调用后端接口得到数据如下
后端实现
controller层
@RequestMapping("/info/{attrGroupId}")
public R info(@PathVariable("attrGroupId") Long attrGroupId){
System.out.println(attrGroupId);
// 通过groudId找到attrGroup,这是mybatis内置方法
AttrGroupEntity attrGroup = attrGroupService.getById(attrGroupId);
System.out.println(attrGroup);
// 通过attrGroup主体找到对应的catelogId
Long catelogId = attrGroup.getCatelogId();
System.out.println(catelogId);
Long[] path = categoryService.findCatelogPath(catelogId);
attrGroup.setCatelogPath(path);
return R.ok().put("attrGroup", attrGroup);
}
service层
AttrGroupService
package com.atguigu.gulimall.product.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.atguigu.common.utils.PageUtils;
import com.atguigu.gulimall.product.entity.AttrGroupEntity;
import java.util.Map;
/**
* 属性分组
*
* @author maplechen
* @email 1146706468@qq.com
* @date 2021-12-26 12:23:21
*/
public interface AttrGroupService extends IService<AttrGroupEntity> {
PageUtils queryPage(Map<String, Object> params);
PageUtils queryPage(Map<String,Object> params,Long catelogId);
}
Bean层
AttrGroupEntity
package com.atguigu.gulimall.product.entity;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import java.io.Serializable;
import java.util.Date;
import lombok.Data;
/**
* 属性分组
*
* @author maplechen
* @email 1146706468@qq.com
* @date 2021-12-26 12:23:21
*/
@Data
@TableName("pms_attr_group")
public class AttrGroupEntity implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 分组id
*/
@TableId
private Long attrGroupId;
/**
* 组名
*/
private String attrGroupName;
/**
* 排序
*/
private Integer sort;
/**
* 描述
*/
private String descript;
/**
* 组图标
*/
private String icon;
/**
* 所属分类id
*/
private Long catelogId;
//这里是分类的路径的id,例如 即[3,14,19] 前端渲染为 手机/手机通讯/手机
//@TableField(exist = false)是数据库中不存在的列
@TableField(exist = false)
private Long[] catelogPath;
}
CategoryEntity
package com.atguigu.gulimall.product.entity;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableLogic;
import com.baomidou.mybatisplus.annotation.TableName;
import java.io.Serializable;
import java.util.Date;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import lombok.Data;
/**
* 商品三级分类
*
* @author maplechen
* @email 1146706468@qq.com
* @date 2021-12-26 12:23:21
*/
@Data
@TableName("pms_category")
public class CategoryEntity implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 分类id
*/
@TableId
private Long catId;
/**
* 分类名称
*/
private String name;
/**
* 父分类id
*/
private Long parentCid;
/**
* 层级
*/
private Integer catLevel;
/**
* 是否显示[0-不显示,1显示]
*/
@TableLogic(value = "1",delval = "0")
private Integer showStatus;
/**
* 排序
*/
private Integer sort;
/**
* 图标地址
*/
private String icon;
/**
* 计量单位
*/
private String productUnit;
/**
* 商品数量
*/
private Integer productCount;
@TableField(exist=false)
/* 不为空时返回的json才带该字段,如果是 手机/手机通讯/手机。第三级(手机)应该没有children这
字段*/
@JsonInclude(JsonInclude.Include.NON_EMPTY)
private List<CategoryEntity> children;
}
CategoryService
package com.atguigu.gulimall.product.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.atguigu.common.utils.PageUtils;
import com.atguigu.gulimall.product.entity.CategoryEntity;
import java.util.List;
import java.util.Map;
/**
* 商品三级分类
*
* @author maplechen
* @email 1146706468@qq.com
* @date 2021-12-26 12:23:21
*/
public interface CategoryService extends IService<CategoryEntity> {
PageUtils queryPage(Map<String, Object> params);
List<CategoryEntity> listWithTree();
void removeMenuByIds(List<Long> asList);
// 找到catelogId完整路径
Long[] findCatelogPath(Long catelogId);
}
最重要是实现
Long[] path = categoryService.findCatelogPath(catelogId);
attrGroup.setCatelogPath(path);
return R.ok().put("attrGroup", attrGroup);
思想:递归
当前第n级分类,查找是否有它的父id
若有则它的父(n-1级)在查找是否是父id
。。。
等到不满足后就退出循环
serviceImpl层
CategoryServiceImpl(核心代码)
@Override
public Long[] findCatelogPath(Long catelogId) {
List<Long> paths = new ArrayList<>();
List<Long> parentPath = findParentPath(catelogId, paths);
// [225, 34, 2]
Collections.reverse(parentPath);
System.out.println(parentPath);
return parentPath.toArray(new Long[parentPath.size()]);
}
private List<Long> findParentPath(Long catelogId,List<Long> paths){
// 1。收集当前节点id
paths.add(catelogId);
//当前id对应的分类级别是否有父,如果有则递归
CategoryEntity byId = this.getById(catelogId);
if(byId.getParentCid()!=0){
findParentPath(byId.getParentCid(),paths);
}
return paths;
}
前端实现
核心代码
v-model是用来显示目前得到的级别分类,例如,家用电器/厨房大件/油烟机
:options是显示所有的层级分类,这里涉及到了需要获得所有分类节点
<el-cascader filterable placeholder="试试搜索:手机" v-model="catelogPath" :options="categorys" :props="props"></el-cascader>
请求后端接口后赋值
catelogPath: [],
this.catelogPath = data.attrGroup.catelogPath;
点击修改,出现的弹框,代码如下
<template>
<el-dialog
:title="!dataForm.id ? '新增' : '修改'"
:close-on-click-modal="false"
:visible.sync="visible"
@closed="dialogClose"
>
<el-form
:model="dataForm"
:rules="dataRule"
ref="dataForm"
@keyup.enter.native="dataFormSubmit()"
label-width="120px"
>
<el-form-item label="组名" prop="attrGroupName">
<el-input v-model="dataForm.attrGroupName" placeholder="组名"></el-input>
</el-form-item>
<el-form-item label="排序" prop="sort">
<el-input v-model="dataForm.sort" placeholder="排序"></el-input>
</el-form-item>
<el-form-item label="描述" prop="descript">
<el-input v-model="dataForm.descript" placeholder="描述"></el-input>
</el-form-item>
<el-form-item label="组图标" prop="icon">
<el-input v-model="dataForm.icon" placeholder="组图标"></el-input>
</el-form-item>
<el-form-item label="所属分类" prop="catelogId">
<!-- <el-input v-model="dataForm.catelogId" placeholder="所属分类id"></el-input> @change="handleChange" -->
<el-cascader filterable placeholder="试试搜索:手机" v-model="catelogPath" :options="categorys" :props="props"></el-cascader>
<!-- :catelogPath="catelogPath"自定义绑定的属性,可以给子组件传值 -->
<!-- <category-cascader :catelogPath.sync="catelogPath"></category-cascader> -->
</el-form-item>
</el-form>
<span slot="footer" class="dialog-footer">
<el-button @click="visible = false">取消</el-button>
<el-button type="primary" @click="dataFormSubmit()">确定</el-button>
</span>
</el-dialog>
</template>
<script>
import CategoryCascader from '../common/category-cascader'
export default {
data() {
return {
props:{
value:"catId",
label:"name",
children:"children"
},
visible: false,
categorys: [],
catelogPath: [],
dataForm: {
attrGroupId: 0,
attrGroupName: "",
sort: "",
descript: "",
icon: "",
catelogId: 0
},
dataRule: {
attrGroupName: [
{ required: true, message: "组名不能为空", trigger: "blur" }
],
sort: [{ required: true, message: "排序不能为空", trigger: "blur" }],
descript: [
{ required: true, message: "描述不能为空", trigger: "blur" }
],
icon: [{ required: true, message: "组图标不能为空", trigger: "blur" }],
catelogId: [
{ required: true, message: "所属分类id不能为空", trigger: "blur" }
]
}
};
},
components:{CategoryCascader},
methods: {
dialogClose(){
this.catelogPath = [];
},
getCategorys(){
this.$http({
url: this.$http.adornUrl("/product/category/list/tree"),
method: "get"
}).then(({ data }) => {
this.categorys = data.data;
});
},
init(id) {
this.dataForm.attrGroupId = id || 0;
this.visible = true;
this.$nextTick(() => {
this.$refs["dataForm"].resetFields();
if (this.dataForm.attrGroupId) {
this.$http({
url: this.$http.adornUrl(
`/product/attrgroup/info/${this.dataForm.attrGroupId}`
),
method: "get",
params: this.$http.adornParams()
}).then(({ data }) => {
if (data && data.code === 0) {
this.dataForm.attrGroupName = data.attrGroup.attrGroupName;
this.dataForm.sort = data.attrGroup.sort;
this.dataForm.descript = data.attrGroup.descript;
this.dataForm.icon = data.attrGroup.icon;
this.dataForm.catelogId = data.attrGroup.catelogId;
//查出catelogId的完整路径
this.catelogPath = data.attrGroup.catelogPath;
}
});
}
});
},
// 表单提交
dataFormSubmit() {
this.$refs["dataForm"].validate(valid => {
if (valid) {
this.$http({
url: this.$http.adornUrl(
`/product/attrgroup/${
!this.dataForm.attrGroupId ? "save" : "update"
}`
),
method: "post",
data: this.$http.adornData({
attrGroupId: this.dataForm.attrGroupId || undefined,
attrGroupName: this.dataForm.attrGroupName,
sort: this.dataForm.sort,
descript: this.dataForm.descript,
icon: this.dataForm.icon,
catelogId: this.catelogPath[this.catelogPath.length-1]
})
}).then(({ data }) => {
if (data && data.code === 0) {
this.$message({
message: "操作成功",
type: "success",
duration: 1500,
onClose: () => {
this.visible = false;
this.$emit("refreshDataList");
}
});
} else {
this.$message.error(data.msg);
}
});
}
});
}
},
created(){
this.getCategorys();
}
};
</script>
更多推荐
所有评论(0)