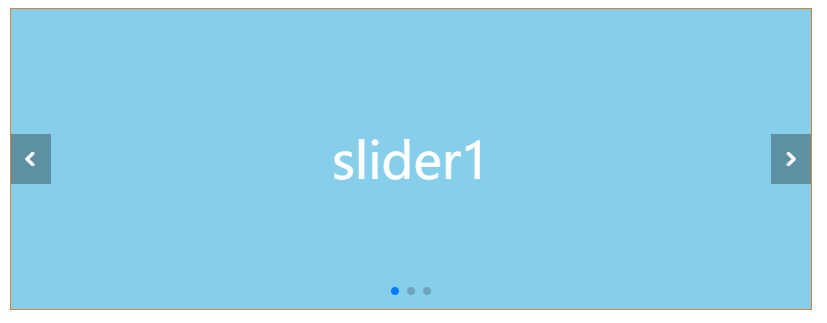
swiper.js插件的使用
1. 自动播放、滑动<!DOCTYPE html><html><head><meta charset="utf-8"><title></title><link rel="stylesheet" type="text/css" href="libs/swiper-bundle.min.css" /><style
·
1. 自动播放、滑动
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<link rel="stylesheet" type="text/css" href="libs/swiper-bundle.min.css" />
<style>
.swiper-container {
width: 800px;
margin: 50px auto;
overflow: hidden;
border: 1px solid peru;
}
.swiper-slide {
width: 800px;
height: 300px;
line-height: 300px;
font-size: 50px;
text-align: center;
color: white;
}
.blue-slide {
background-color: skyblue;
}
.red-slide {
background-color: indianred;
}
.orange-slide {
background-color: orange;
}
</style>
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide blue-slide">slider1</div>
<div class="swiper-slide red-slide">slider2</div>
<div class="swiper-slide orange-slide">slider3</div>
</div>
</div>
<script src="libs/swiper-bundle.min.js"></script>
<script>
var mySwiper = new Swiper('.swiper-container', {
autoplay: true, //等同于以下设置
/*autoplay: {
delay: 3000,
stopOnLastSlide: false,
disableOnInteraction: true,
},*/
});
</script>
</body>
</html>
2. 自动播放、滑动、图片循环
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<link rel="stylesheet" type="text/css" href="libs/swiper-bundle.min.css" />
<style>
.swiper-container {
width: 800px;
margin: 50px auto;
overflow: hidden;
border: 1px solid peru;
}
.swiper-slide {
width: 800px;
height: 300px;
line-height: 300px;
font-size: 50px;
text-align: center;
color: white;
}
.blue-slide {
background-color: skyblue;
}
.red-slide {
background-color: indianred;
}
.orange-slide {
background-color: orange;
}
</style>
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide blue-slide">slider1</div>
<div class="swiper-slide red-slide">slider2</div>
<div class="swiper-slide orange-slide">slider3</div>
</div>
</div>
<script src="libs/swiper-bundle.min.js"></script>
<script>
var mySwiper = new Swiper('.swiper-container', {
autoplay: true, //等同于以下设置
loop:true
/*autoplay: {
delay: 3000,
stopOnLastSlide: false,
disableOnInteraction: true,
},*/
});
</script>
</body>
</html>
3. 自动播放、滑动、图片循环、翻页按钮
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<link rel="stylesheet" type="text/css" href="libs/swiper-bundle.min.css" />
<link rel="stylesheet" type="text/css" href="libs/iconfont.css" />
<style>
* {
margin: 0;
padding: 0;
}
ul,
li {
list-style: none;
}
.swiper-container {
position: relative;
width: 800px;
margin: 50px auto;
overflow: hidden;
border: 1px solid peru;
}
.swiper-container:hover .turn-btns {
display: block;
}
.swiper-slide {
width: 800px;
height: 300px;
line-height: 300px;
font-size: 50px;
text-align: center;
color: white;
}
.blue-slide {
background-color: skyblue;
}
.red-slide {
background-color: indianred;
}
.orange-slide {
background-color: orange;
}
.turn-btns {
display: none;
position: absolute;
top: 125px;
width: 100%;
height: 50px;
z-index: 999;
}
.turn-btns li:hover {
background-color: rgba(0, 0, 0, 0.6);
}
.turn-btns .pre-btn,
.turn-btns .next-btn {
width: 40px;
height: 50px;
line-height: 50px;
text-align: center;
color: white;
background-color: rgba(0, 0, 0, 0.3);
cursor: pointer;
}
.turn-btns .pre-btn {
float: left;
}
.turn-btns .next-btn {
float: right;
}
</style>
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide blue-slide">slider1</div>
<div class="swiper-slide red-slide">slider2</div>
<div class="swiper-slide orange-slide">slider3</div>
</div>
<ul class="turn-btns">
<li class="pre-btn"><span class="iconfont icon-left"></span></li>
<li class="next-btn"><span class="iconfont icon-right"></span></li>
</ul>
</div>
<script src="libs/jquery.min.js"></script>
<script src="libs/swiper-bundle.min.js"></script>
<script>
var mySwiper = new Swiper('.swiper-container', {
autoplay: true, //等同于以下设置
loop: true
/*autoplay: {
delay: 3000,
stopOnLastSlide: false,
disableOnInteraction: true,
},*/
});
$('.pre-btn').click(function() {
mySwiper.slidePrev();
})
$('.next-btn').click(function() {
mySwiper.slideNext();
})
</script>
</body>
</html>
4. 自动播放、滑动、图片循环、翻页按钮、分页器
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<link rel="stylesheet" type="text/css" href="libs/swiper-bundle.min.css" />
<link rel="stylesheet" type="text/css" href="libs/iconfont.css" />
<style>
* {
margin: 0;
padding: 0;
}
ul,
li {
list-style: none;
}
.swiper-container {
position: relative;
width: 800px;
margin: 50px auto;
overflow: hidden;
border: 1px solid peru;
}
.swiper-container:hover .turn-btns {
display: block;
}
.swiper-slide {
width: 800px;
height: 300px;
line-height: 300px;
font-size: 50px;
text-align: center;
color: white;
}
.blue-slide {
background-color: skyblue;
}
.red-slide {
background-color: indianred;
}
.orange-slide {
background-color: orange;
}
.turn-btns {
display: none;
position: absolute;
top: 125px;
width: 100%;
height: 50px;
z-index: 999;
}
.turn-btns li:hover {
background-color: rgba(0, 0, 0, 0.6);
}
.turn-btns .pre-btn,
.turn-btns .next-btn {
width: 40px;
height: 50px;
line-height: 50px;
text-align: center;
color: white;
background-color: rgba(0, 0, 0, 0.3);
cursor: pointer;
}
.turn-btns .pre-btn {
float: left;
}
.turn-btns .next-btn {
float: right;
}
</style>
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide blue-slide">slider1</div>
<div class="swiper-slide red-slide">slider2</div>
<div class="swiper-slide orange-slide">slider3</div>
</div>
<div class="swiper-pagination"></div>
<ul class="turn-btns">
<li class="pre-btn"><span class="iconfont icon-left"></span></li>
<li class="next-btn"><span class="iconfont icon-right"></span></li>
</ul>
</div>
<script src="libs/jquery.min.js"></script>
<script src="libs/swiper-bundle.min.js"></script>
<script>
var mySwiper = new Swiper('.swiper-container', {
autoplay: {
delay: 3000,
stopOnLastSlide: false,
disableOnInteraction: false,//用户操作swiper后是否继续自动播放
},
loop: true,
pagination: {
el: '.swiper-pagination',
}
});
$('.pre-btn').click(function() {
mySwiper.slidePrev();
})
$('.next-btn').click(function() {
mySwiper.slideNext();
})
</script>
</body>
</html>
更多推荐
所有评论(0)