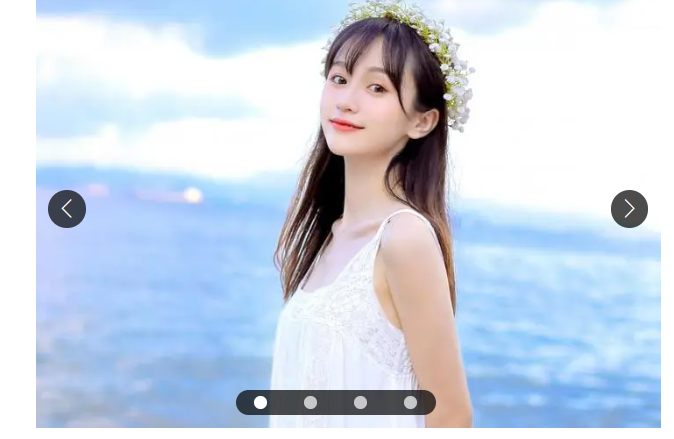
都可以看懂的JS轮播图(全代码注释)
js轮播图的实现,所有代码加注释+++
·
最近一直在写dom的项目,这里给大家看一个最常见的轮播图吧,虽热现在许多的轮播图我们只需要去网站搜一搜就可以找到我们各种想要的,但是我们在学习阶段,还是能够自己来完成一个轮播图案例,这样可以让我们更好的去理解dom 这里就不多说了,直接上代码,所有的代码,除了获取标签基本上都写了注释,小白都可以看懂~~~
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
.wrap {
width: 500px;
height: 350px;
margin: 0 auto;
position: relative;
background-color: orange;
background-repeat: no-repeat;
background-position: center center;
background-size: cover;
}
@font-face {
font-family: 'arraw';
src: url(font/iconfont.ttf);
}
.arraw {
width: 30px;
height: 30px;
background-color: rgba(0, 0, 0, .7);
color: #fff;
line-height: 30px;
text-align: center;
position: absolute;
top: 160px;
border-radius: 50%;
font-family: 'arraw';
}
.prev {
left: 10px;
}
.next {
right: 10px;
}
.dots {
position: absolute;
width: 160px;
height: 20px;
background-color: rgba(0, 0, 0, .7);
bottom: 10px;
left: 160px;
display: flex;
justify-content: space-around;
align-items: center;
border-radius: 10px;
}
.dot {
width: 10px;
height: 10px;
background-color: rgba(255, 255, 255, .7);
border-radius: 50%;
}
.now {
background-color: #fff;
}
.move {
width: 2400px;
height: 300px;
position: absolute;
left: 0;
}
.move img {
width: 400px;
height: 300px;
float: left;
}
</style>
</head>
<body>
<div class="wrap">
<div class="arraw prev"></div>
<div class="arraw next"> </div>
<div class="dots">
<div class="dot now"></div>
<div class="dot"></div>
<div class="dot"></div>
<div class="dot"></div>
</div>
</div>
</body>
<script>
var data = ['https://img2.baidu.com/it/u=2468362699,2612376962&fm=253&fmt=auto&app=138&f=JPEG?w=889&h=500',
'https://img2.baidu.com/it/u=1842436282,1471089086&fm=253&fmt=auto&app=120&f=JPEG?w=1280&h=800',
'https://img1.baidu.com/it/u=2461392816,2086174431&fm=253&fmt=auto&app=120&f=JPEG?w=1280&h=800',
'https://img0.baidu.com/it/u=2066710797,1295269268&fm=253&fmt=auto&app=120&f=JPEG?w=1422&h=800'
];
//先来完成点击左右箭头可以切换
var dWrap = document.querySelector('.wrap')
var dNext = document.querySelector('.next')
var dPrev = document.querySelector('.prev')
var showIndex = 0
// 还有一个图片和小圆点同步切换的效果
// 在这里写下思路
// 我们上面给了一个属性now来代表小白点的颜色
// 我们可以通过在改变下标的同时改变now的添加对象
var dots = document.querySelectorAll('.dot')
// 左箭头添加点击事件
dPrev.onclick = function() {
// 点击之后给这个圆圈去除now属性
dots[showIndex].classList.remove('now')
// 我们在上面定义一个showIndex来表示我们当前显示的下标
showIndex = showIndex - 1 //每点击一次让下标-1 就是返回上一张
// changeBg(data[showIndex])
// 之后我们要进行越界判断,我们在每一次点击的时候showindex的值都会增加,但是我们的图只有四张
// 所以我们需要判断
// 在点击上一张的时候,showIndex的值在等于-1之后,再次点击返回最后一张
if (showIndex === -1) {
showIndex = data.length - 1
} else {
showIndex = showIndex
}
changeBg(data[showIndex])
// 在showIndex的值变化后给新的重新添加属性,下面的思路和这个一样
dots[showIndex].classList.add('now')
}
//右箭头添加点击事件,道理和上面相同
dNext.onclick = function() {
dots[showIndex].classList.remove('now')
showIndex = showIndex + 1
// changeBg(data[showIndex])
// 这边的越界处理需要判断
// 当点击到最后一张图的时候,返回第一张
if (showIndex === data.length) {
showIndex = 0
} else {
showIndex = showIndex
}
changeBg(data[showIndex])
dots[showIndex].classList.add('now')
}
// 我们点击切换的是dWrap背景图,可以先来设置一个改变背景图的函数,在我们点击事件中添加即可
function changeBg(pic) {
dWrap.style.backgroundImage = `url(${pic})`
}
// 默认显示第一张图片
changeBg(data[0])
// 上面就完成了点击箭头的切换,我们在来实现点击圆圈来选择图片的方法
// 我们需要给每一个圆圈都添加上点击事件,这个我们就可以在添加点击事件的同时,来得到每个圆圈的下标
// 我们只需要让我们点击的这个圆圈的下标的值等于showIndex的值就可以
//在之后就是圆圈的变色问题,和上面的解决思路一样,先删除后添加
for (var i = 0; i < dots.length; i++) {
dots[i].index = i //我们用.语法来给dots添加一个下标
dots[i].onclick = function() {
dots[showIndex].classList.remove('now')
showIndex = this.index
changeBg(data[showIndex])
dots[showIndex].classList.add('now')
}
}
// 上面我们完成了所有的点击效果 最后一步我们来完成自动轮播的效果
// 这个肯定就用到了我们的计时器,我们还是采用改变下标的做法来完成
var timer = null
timer = setInterval(function() {
dots[showIndex].classList.remove('now')
showIndex = showIndex + 1
//越界问题我们写到这里比较好,在判断完成之后,我们再执行函数
// 既然图片是向后面走,那么这个越界处理就和我们上面dNext的越界处理一样了
if (showIndex === data.length) {
showIndex = 0
} else {
showIndex = showIndex
}
changeBg(data[showIndex])
// 这样就可以让图片滚动,还是解决我们的两个老问题,越界和圆圈的跟随显示
//圆圈跟随问题好解决,在我们改变下标前后完成now属性的添加和删除就可以了
dots[showIndex].classList.add('now')
}, 5000)
</script>
</html>
代码给大家放到这里了,当然也有做的不好的地方,大家多多指点
更多推荐
所有评论(0)