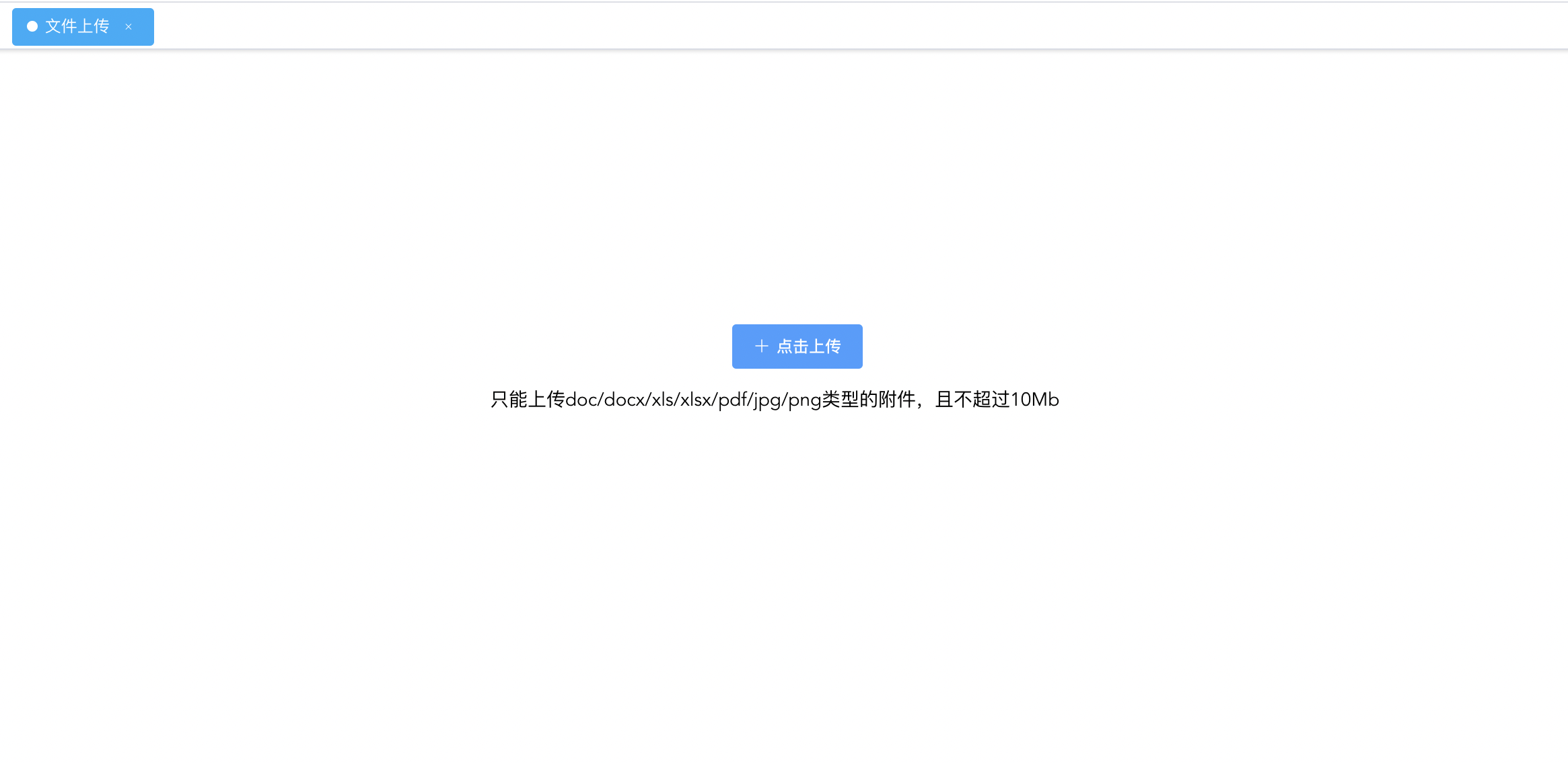
vue使用el-upload组件上传附件,文件压缩上传、文件类型限制、文件上传个数限制、文件预览下载等功能
el-upload组件上传文件,包括限制文件类型、限制文件大小、限制文件上传个数、文件预览下载等功能
·
vue使用el-upload组件上传附件,文件压缩上传、文件类型限制、文件上传个数限制、文件预览下载等功能
<template>
<div class="uploadfile-content">
<el-form>
<el-col>
<el-form-item>
<el-upload
ref="upload"
:http-request="upload"
class="upload-demo"
action=""
:on-preview="handlePreview"
:on-remove="handleRemove"
:on-change="handleChange"
:on-success="handleSuccess"
:on-exceed="handleExceed"
:limit="10"
:file-list="fileLists"
:disabled="publicdisabled"
:before-upload="beforeUpload"
multiple
>
<el-button size="small" type="primary" icon="el-icon-plus">点击上传</el-button>
<div slot="tip">只能上传doc/docx/xls/xlsx/pdf/jpg/png类型的附件,且不超过10Mb</div>
</el-upload>
</el-form-item>
</el-col>
</el-form>
</div>
</template>
<script>
export default {
data(){
return {
fileList:[],
fileLists:[],
publicdisabled:false
}
},
methods:{
// 上传接口
upload(val){
this.loading=this.$loading({
lock:true,
text:'上传中...',
spinner:'el-icon-loading',
backfround:'rgba(0,0,0,0.7)'
});
let param=new FormData();
param.append('file',val.file)
param.append('isPermission','N')
return common.uploadFile(this.$api,param).then((res)=>{
}).catch((err)=>{
this.loading.close()
})
},
// 删除文件
handleRemove(file,fileList){
var filePash
if(file.response&&file.response.attachId){
filePash=file.response.attachId
}else{
filePash=file.attachmentId
}
let y=this.fileList.findIndx((x)=>x.attachmentId===filePash)
if(y===-1){
return
}
this.fileList.splice(y,1)
this.fileLists=fileList
},
// 限制上传文件类型
handleChange(file,fileList){
let testmsg=file.name.substring(file.name.lastIndexOf('.')+1)
let extension1=testmsg==='jpg'
let extension2=testmsg==='jpeg'
let extension3=testmsg==='png'
let extension4=testmsg==='doc'
let extension5=testmsg==='docx'
let extension6=testmsg==='xls'
let extension7=testmsg==='xlsx'
let extension8=testmsg==='pdf'
if(!extension1&&!extension2&&!extension3&&!extension4&&!extension5&&!extension6&&!extension7&&!extension8){
this.$message.error("上传附件子能是doc/docx/xls/xlsx/pdf/jpg/png/jpeg 格式!")
let filePash
if(file.response&&file.response.attachId){
filePash=file.response.attachId
}else{
filePash=file.attachmentId
}
let y=this.fileList.findIndx((x)=>x.attachmentId===filePash)
if(y===-1){
return
}
this.fileList.splice(y,1)
this.fileLists=fileList
return false
}
let isLt10M=file.size/1024/1024<10
if(!isLt10M){
this.$message.error("上传图片大小不能超过 10MB!")
let filePash
if(file.response&&file.response.attachId){
filePash=file.response.attachId
}else{
filePash=file.attachmentId
}
let y=this.fileList.findIndx((x)=>x.attachmentId===filePash)
if(y===-1){
return
}
this.fileList.splice(y,1)
this.fileLists=fileList
return false
}
this.fileLists=fileList
},
// 上传成功回调
handleSuccess(res,fileList){
// 这里一定要加判断,不然上传失败后删除文件会找不到id导致报错
if(res){
this.loading.close()
this.fileLists=fileList
this.fileList.push({attachmentId:res.attachId,attachmentName:res.attachName,name:res.attachOriginalName})
}
},
// 文件预览
handlePreview(file){
let url=''
if(file.name.endsWith('docx')||file.name.endsWith('doc')||file.name.endsWith('pdf')||file.name.endsWith('xlsx')||file.name.endsWith('xls')){
if(file.attachmentId){
// 这里调用接口方法以实际的接口为准
common.downFile(this.$api,file.attachmentId).then((res)=>{
let url =window.URL.createObjectURL(new Blob([res.file],{type:'application/octet-stream;charset=UFT-8'}))
let link=document.createElement('a')
link.href=url
link.setAttribute('download',file.name)
document.body.appendChild(link)
link.click()
document.body.removeChild(link)
window.URL.revokeObjectURL(url)
})
}else if(file.response.attachId){
common.downFile(this.$api,file.response.attachId).then((res)=>{
let url =window.URL.createObjectURL(new Blob([res.file],{type:'application/octet-stream;charset=UFT-8'}))
let link=document.createElement('a')
link.href=url
link.setAttribute('download',file.name)
document.body.appendChild(link)
link.click()
document.body.removeChild(link)
window.URL.revokeObjectURL(url)
})
}
}else{
if(file.url){
url=file.url
let width=1000
let height=800
let top=(window.screen.availHeight-height)/2
let left=(window.screen.availWidth-width)/2
window.open(url,'','width='+width+',height='+height+',top='+top+',left='+left)
}else if(file.response.attachId){
common.downFile(this.$api,file.response.attachId).then((res)=>{
let imgUrl=''
imgUrl=window.URL.createObjectURL(res.file)
let width=1000
let height=800
let top=(window.screen.availHeight-height)/2
let left=(window.screen.availWidth-width)/2
window.open(imgUrl,'','width='+width+',height='+height+',top='+top+',left='+left)
})
}
}
},
// 文件限制个数
handleExceed(files,fileList){
this.$message.warning(`当前限制选择 10 个文件,本次选择了 ${files.length} 个文件,共选择了 ${files.length+fileList.length} 个文件`)
},
// 文件上传前回调方法
beforeUpload(file){
let isJPG=file.name.split('.').splice(-1)=='pdf'
||file.name.split('.').splice(-1)=='doc'
||file.name.split('.').splice(-1)=='docx'
||file.name.split('.').splice(-1)=='jpg'
||file.name.split('.').splice(-1)=='jpeg'
||file.name.split('.').splice(-1)=='png'
||file.name.split('.').splice(-1)=='xls'
||file.name.split('.').splice(-1)=='xlsx'
let isLt2M =file.size/1024/1024<10
let isJpgOrPng=file.type==='image/jpeg'
||file.type==='image/png'
||file.type==='image/jpg'
||file.name.split('.').splice(-1)=='jpg'
||file.name.split('.').splice(-1)=='jpeg'
||file.name.split('.').splice(-1)=='png'
if(!isJPG){
return isJPG
}
if(!isLt2M){
return isLt2M
}
if(isJpgOrPng){
let that=this
return new Promise(resolve=>{
let reader=new FileReader()
let image=new Image()
image.onload=(imageEvent)=>{
let canvas=document.createElement('canvas')
let context=canvas.getContext('2d')
let originWidth=image.width
let originHeight=image.height
// 最大尺寸限制
let maxWidth=1600,maxHeight=1600
let targetWidth=originWidth,targetHeight=originHeight
// 超过1800X1800的限制
if(originWidth>maxWidth||originHeight>maxHeight){
if(originWidth/originHeight>maxWidth/maxHeight){
targetWidth=maxWidth
targetHeight=Math.round(maxWidth*(originHeight/originWidth))
}else{
targetHeight=maxHeight
targetWidth=Math.round(maxWidth*(originWidth/originHeight))
}
}
canvas.width=targetWidth
canvas.height=targetHeight
context.clearRect(0,0,targetWidth,targetHeight)
context.drawImage(image,0,0,targetWidth,targetHeight)
let dataUrl=canvas.toDataURL(file.type,0.8)
let blobData=that.dataURItoBlob(dataUrl,file.type)
resolve(blobData)
}
reader.onload=(e=>{image.src=e.target.result})
reader.readAsDataURL(file)
})
}
},
// 文件压缩方法
dataURItoBlob(dataURI,type){
let binary=atob(dataURI.split(',')[1])
let array=[]
for(let i=0;i<binary.length;i++){
array.push(binary.charCodeAt(i))
}
return new Blob([new Uint8Array(array)],{type:type})
}
}
}
</script>
<style>
.uploadfile-content{
margin: auto;
margin-top:200px;
width: 500px;
height: 200px;
}
.el-upload {
padding-left: 180px;
}
</style>
更多推荐
所有评论(0)