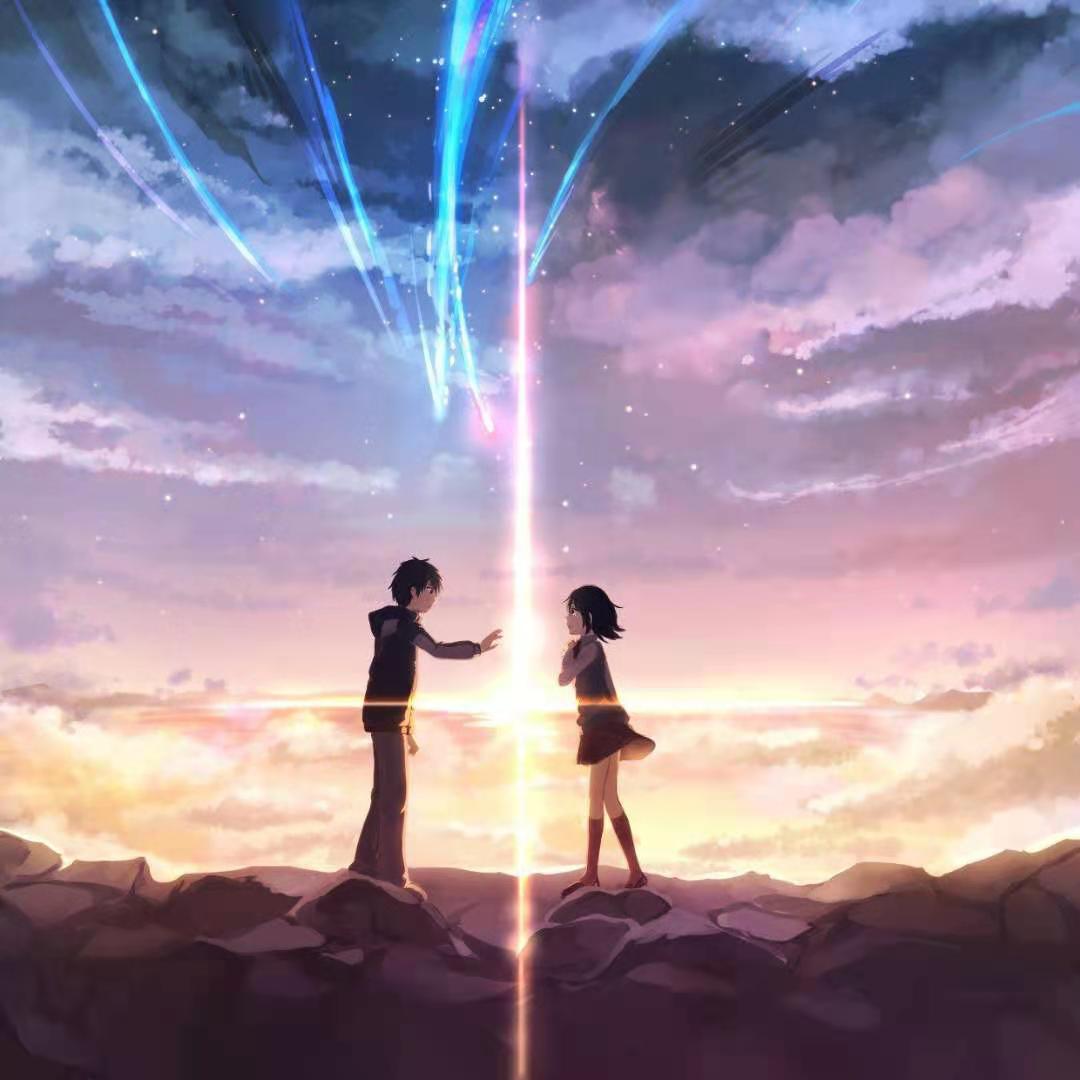
Ajax发送请求的几种方法
Ajax 的全称是 Asynchronous JavaScript And XML(异步 JavaScript 和 xml)通俗理解:在网页中利用 XMLHttpRequest 对象和服务器进行数据交互的方式,就是Ajax。
·
Ajax是什么?
Ajax
的全称是 Asynchronous JavaScript And XML
(异步 JavaScript
和 xml
)
通俗理解:在网页中利用 XMLHttpRequest
对象和服务器进行数据交互的方式,就是Ajax
为什么要学Ajax?
Ajax
能让我们轻松实现 网页 与 服务器之间的 数据交互
什么是接口?
使用 Ajax
请求数据时,被请求的 URL
地址,就叫做 数据接口(简称接口)
接口请求的过程
GET方式请求接口的过程
POST方式请求接口的过程
什么是axios?
Axios是一个基于promise的可用在浏览器和node.js中的异步通信框架;主要作用是实现AJAX异步通信。
发送Ajax的三种方法
第一种:JQuery发送请求
1.发送不带参数的GET请求
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="btnGET">发起不带参数的GET请求</button>
<script>
$(function () {
$('#btnGET').on('click', function () {
$.get('发送请求的URL地址', function (res) {
console.log(res)
})
})
})
</script>
</body>
2.发送带参数的GET请求
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="btnGETINFO">发起单参数的GET请求</button>
<script>
$(function () {
$('#btnGETINFO').on('click', function () {
$.get('发送请求的URL地址', {
id: 1 //携带的参数 这里我携带的是id,但是具体要看接口的需要
}, function (res) {
console.log(res)
})
})
})
</script>
</body>
3.使用$.ajax发送GET请求
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="btnGET">发起GET请求</button>
<script>
$(function () {
$('#btnGET').on('click', function () {
$.ajax({
type: 'GET',
url: 'http://www.liulongbin.top:3006/api/getbooks',
data: {
id: 1
},
success: function (res) {
console.log(res)
}
})
})
})
</script>
</body>
4.发送不带参数的POST请求
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="btnPOST">发起POST请求</button>
<script>
$(function () {
$('#btnPOST').on('click', function () {
$.post('发送请求的URL地址', {
//携带的参数
bookname: '学霸养成手册',
author: 'dy',
publisher: '北京图书出版社'
}, function (res) {
console.log(res)
})
})
})
</script>
</body>
5.使用$.ajax发送POST请求
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id="btnPOST">发起POST请求</button>
<script>
$(function () {
$('#btnPOST').on('click', function () {
$.ajax({
type: 'POST',
url: '发送请求的URL地址',
data: {
bookname: '偶像自我修养',
author: '娱乐圈风纪委',
publisher: '国家出版社'
},
success: function (res) {
console.log(res)
}
})
})
})
</script>
</body>
第二种:原生JS发送Ajax请求
1.使用xhr发起GET不带参数的数据请求
<script>
// 1.创建XHR对象
var xhr = new XMLHttpRequest();
// 2.调用open函数
xhr.open('GET', '请求的URL地址');
// 3.调用send函数
xhr.send()
// 4.监听 onreadystatechange 事件
xhr.onreadystatechange = function () {
// 判断条件是固定的代码 这里的status和获取看到的Status Code不一样
if (xhr.readyState === 4 && xhr.status === 200) {
// 获取服务器响应的数据
console.log(xhr.responseText)
}
}
</script>
2.使用xhr发起GET带参数的数据请求
<script>
// 1.创建
var xhr = new XMLHttpRequest();
// 2. open 地址后面跟的是携带的参数
xhr.open('GET', '发送请求的URL地址?id=1&bookname=西游记');
// 3. send()
xhr.send();
// 4. onreadystatechange
xhr.onreadystatechange = function () {
// 判断成功 信息
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText)
}
}
</script>
3.使用xhr发送POST请求
<script>
// 1. 创建 xhr 对象
var xhr = new XMLHttpRequest()
// 2. 调用 open 函数
xhr.open('POST', '请求的URL地址')
// 3. 设置 Content-Type 属性
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded')
// 4. 调用 send 函数
xhr.send('bookname=水浒传&author=施耐庵&publisher=上海图书出版社')
// 5. 监听事件
xhr.onreadystatechange = function () {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText)
}
}
</script>
第三种:axios发送请求
1.axios发送GET请求
//需要引入axios.js文件
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
</head>
<body>
<button id="btn1">axios发起GET请求</button>
<script>
document.querySelector('#btn1').addEventListener('click', function () {
var url = '发送请求的URL';
var paramsData = {
//携带的数据
name: '赫敏',
age: 16
};
axios({
method: 'GET',
url: url,
params: paramsData
}).then(function (res) {
res = res.data;
console.log(res)
})
})
</script>
</body>
2.axios发送POST请求
//需要引入axios.js文件
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
<body>
<button id="btn2">axios发送POST请求</button>
<script>
document.querySelector('#btn2').addEventListener('click', function () {
axios({
method: 'POST',
url: '发送请求的URL地址',
data: {
name: 'dy',
age: 3,
sex: '女'
}
}).then(function (res) {
res = res.data
console.log(res)
})
})
</script>
更多推荐
所有评论(0)