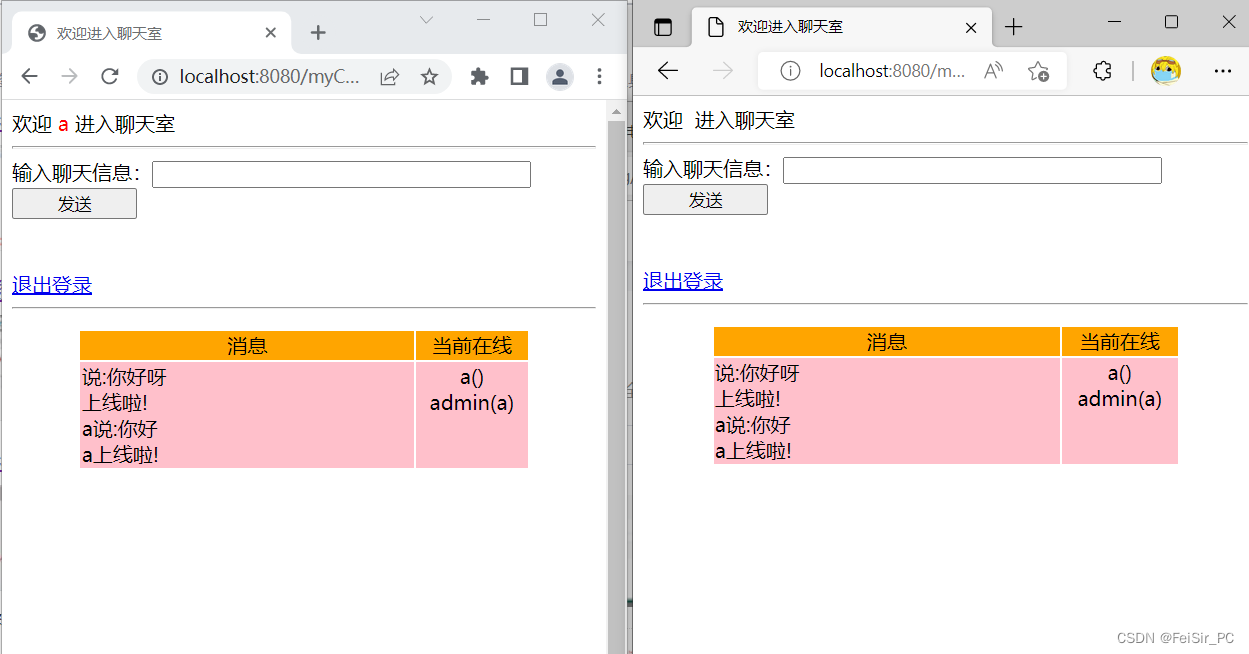
基于Java语言的Web在线聊天室
能够实现登录,注册,聊天功能,最终效果如下图所示注册页面注册失败页面登录界面聊天界面消息界面,因为需要异步刷新,采用iframe标签进行刷新OK,前端完毕,下面开始编写后端,这个项目是jsp的,用的原生Servlet进行转发请求,首先,需要连接数据库的代码然后需要一个用户的类,用来存储信息然后就是4个servlet转发请求,注册的请求如下登录的请求如下发消息的请求如下退出登录的消息如下上面这些这就
·
在线聊天室
能够实现登录,注册,聊天功能,最终效果如下图所示
注册页面
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="Register" name="form1" method="post">
输入账号:<input name="account" type="text"><BR> 输入密码:<input
name="password" type="password"><BR> 输入真实姓名:<input
name="realname" type="text"><br/><input type="submit"
value="注册并登录">
<br/>
</form>
</body>
</html>
注册失败页面
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
注册失败
</body>
</html>
登录界面
<%@page import="java.util.ArrayList" %>
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Insert title here</title>
<style>
.mainlogin {
width: 500px;
height: 500px;
margin: 0px auto;
}
.logindiv {
margin-top: 100px;
}
</style>
</head>
<body>
<% /*初始化application*/ ArrayList customers=(ArrayList) application.getAttribute("customers"); if
(customers==null) { customers=new ArrayList(); application.setAttribute("customers", customers); }
ArrayList msgs=(ArrayList) application.getAttribute("msgs"); if (msgs==null) { msgs=new ArrayList();
application.setAttribute("msgs", msgs); } %>
<div class="mainlogin">
<div class="logindiv">
<div style="width: 100%; text-align: center;">欢迎登录聊天系统</div>
<div style="width: 100%; text-align: center;">
<form action="loginAction" name="form1" method="post">
输入账号:<input name="account" type="text"><BR> 输入密码:<input name="password" type="password">
<br /><br /> <input
type="submit" style="width: 150px;" value="登录">
<br />
<span>还没有账号?</span>去<a href="register.jsp">注册</a>
</form>
</div>
</div>
</div>
</body>
</html>
聊天界面
<%@page import="renaofeiChatRoom.bean.Customer"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>欢迎进入聊天室</title>
</head>
<body>
<%
Customer customer = (Customer)session.getAttribute("customer");
%>
<span>欢迎 <span style="color:red" ><%=customer.getCname() %></span> 进入聊天室</span>
<HR>
<form action="chatAction" name="form1" method="post">
输入聊天信息:<input name="msg" type="text" size="40"> <input
type="submit" style="width: 100px;" value="发送">
</form><br><br>
<a href="logout">退出登录</a>
<HR>
<iframe src="msgs.jsp" width="100%" height="100%" style="height: 500px;" frameborder="0"></iframe>
</body>
</html>
消息界面,因为需要异步刷新,采用iframe标签进行刷新
<%@page import="renaofeiChatRoom.bean.Customer" %>
<%@page import="java.util.ArrayList" %>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<% response.setHeader("Refresh","5"); %>
<table width="80%" border="0" align="center">
<tr bgcolor="orange" align="center">
<td width="75%">消息</td>
<td width="25%">当前在线</td>
</tr>
<tr bgcolor='pink'><td>
<% ArrayList msgs=(ArrayList)application.getAttribute("msgs"); for(int
i=msgs.size()-1;i>=0;i--){
out.println(msgs.get(i) + "<br>");
}
%>
</td>
<td valign='top' style="text-align: center;">
<% ArrayList customers=(ArrayList)application.getAttribute("customers"); for(int
i=customers.size()-1;i>=0;i--){
Customer customer = (Customer)customers.get(i);
out.println(customer.getAccount() + "(" + customer.getCname() + ")"+"<br>");
}
%>
</td>
</tr>
</table>
</body>
</html>
OK,前端完毕,下面开始编写后端,这个项目是jsp的,用的原生Servlet进行转发请求,首先,需要连接数据库的代码
public class CustomerDao {
private Connection conn = null;
public void initConnection() throws Exception {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/chatdb?severTimezone=UTC&useUnicode=true&characterEncoding=UTF-8", "root", "");//数据库连接配置
}
public Customer getCustomerByAccount(String account) throws Exception {
Customer cus = null;
initConnection();
String sql = "SELECT ACCOUNT,PASSWORD,CNAME FROM T_CUSTOMER WHERE ACCOUNT=?";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, account);
ResultSet rs = ps.executeQuery();
if(rs.next()){
cus = new Customer();
cus.setAccount(rs.getString("ACCOUNT"));
cus.setPassword(rs.getString("PASSWORD"));
cus.setCname(rs.getString("CNAME"));
}
closeConnection();
return cus;
}
public boolean regeditNewCustomer(String name,String pwd,String rname) throws Exception {
Customer cus = null;
initConnection();
String sql = "INSERT INTO `t_customer` (`ACCOUNT`, `PASSWORD`, `CNAME`) VALUES (?, ?, ?)";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, name);
ps.setString(2, pwd);
ps.setString(3, rname);
int flag=0;
try {
flag = ps.executeUpdate();
closeConnection();
} catch (Exception e) {
closeConnection();
}
return flag>=1;
}
public void closeConnection() throws Exception {
conn.close();
}
}
然后需要一个用户的类,用来存储信息
public class Customer {
private String account;
private String password;
private String cname;
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getCname() {
return cname;
}
public void setCname(String cname) {
this.cname = cname;
}
}
然后就是4个servlet转发请求,注册的请求如下
/**
* Servlet implementation class Register
*/
@WebServlet("/Register")
public class Register extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public Register() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
//response.getWriter().append("Served at: ").append(request.getContextPath());
ServletContext application=this.getServletContext();
HttpSession session=request.getSession();
request.setCharacterEncoding("UTF-8");
String account = request.getParameter("account");
String password = request.getParameter("password");
String realname = request.getParameter("realname");
CustomerDao cdao = new CustomerDao();
boolean flag=false;
try {
flag=cdao.regeditNewCustomer(account, password, realname);
} catch (Exception e) {
}
if(flag) {
Customer customer=null;
try {
customer = cdao.getCustomerByAccount(account);
} catch (Exception e) {
// TODO: handle exception
}
if (customer == null || !customer.getPassword().equals(password)) {
response.sendRedirect("loginForm.jsp");
} else {
session.setAttribute("customer", customer);
ArrayList customers = (ArrayList) application.getAttribute("customers");
if (customers == null) {
customers = new ArrayList();
application.setAttribute("customers", customers);
}
ArrayList msgs = (ArrayList) application.getAttribute("msgs");
if (msgs == null) {
msgs = new ArrayList();
application.setAttribute("msgs", msgs);
}
customers.add(customer);
msgs.add(customer.getCname() + "上线啦!");
response.sendRedirect("chatForm.jsp");
}
}else {response.sendRedirect("regFailed.jsp");
}
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
登录的请求如下
/**
* Servlet implementation class loginAction
*/
@WebServlet("/loginAction")
public class loginAction extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public loginAction() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
// response.getWriter().append("Served at: ").append(request.getContextPath());
//��¼����
ServletContext application=this.getServletContext();
HttpSession session=request.getSession();
request.setCharacterEncoding("UTF-8");
String account = request.getParameter("account");
String password = request.getParameter("password");
//out.println(account);
CustomerDao cdao = new CustomerDao();
Customer customer=null;
try {
customer = cdao.getCustomerByAccount(account);
} catch (Exception e) {
// TODO: handle exception
}
if (customer == null || !customer.getPassword().equals(password)) {
response.sendRedirect("loginForm.jsp");
} else {
session.setAttribute("customer", customer);
ArrayList customers = (ArrayList) application.getAttribute("customers");
if (customers == null) {
customers = new ArrayList();
application.setAttribute("customers", customers);
}
ArrayList msgs = (ArrayList) application.getAttribute("msgs");
if (msgs == null) {
msgs = new ArrayList();
application.setAttribute("msgs", msgs);
}
customers.add(customer);
msgs.add(customer.getCname() + "上线啦!");
response.sendRedirect("chatForm.jsp");
}
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
发消息的请求如下
/**
* Servlet implementation class chatAction
*/
@WebServlet("/chatAction")
public class chatAction extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public chatAction() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
// response.getWriter().append("Served at: ").append(request.getContextPath());
ServletContext application = this.getServletContext();
HttpSession session = request.getSession();
Customer customer = (Customer) session.getAttribute("customer");
request.setCharacterEncoding("utf-8");
String msg = request.getParameter("msg");
ArrayList<String> msgs = (ArrayList) application.getAttribute("msgs");
if (msgs == null) msgs = new ArrayList();
msgs.add(customer.getCname() + "说:" + msg);
response.sendRedirect("chatForm.jsp");
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse
* response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
退出登录的消息如下
/**
* Servlet implementation class logout
*/
@WebServlet("/logout")
public class logout extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public logout() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
//response.getWriter().append("Served at: ").append(request.getContextPath());
ServletContext application=this.getServletContext();
HttpSession session=request.getSession();
Customer customer = (Customer)session.getAttribute("customer");// session.getAttribute("customer");
ArrayList customers = (ArrayList) application.getAttribute("customers");
customers.remove(customer);
ArrayList msgs = (ArrayList)application.getAttribute("msgs");
msgs.add(customer.getCname() + "下线啦!");
session.invalidate();
response.sendRedirect("loginForm.jsp");
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
上面这些这就是全部代码了,资源地址如下
基于Java的在线的聊天室
更多推荐
所有评论(0)