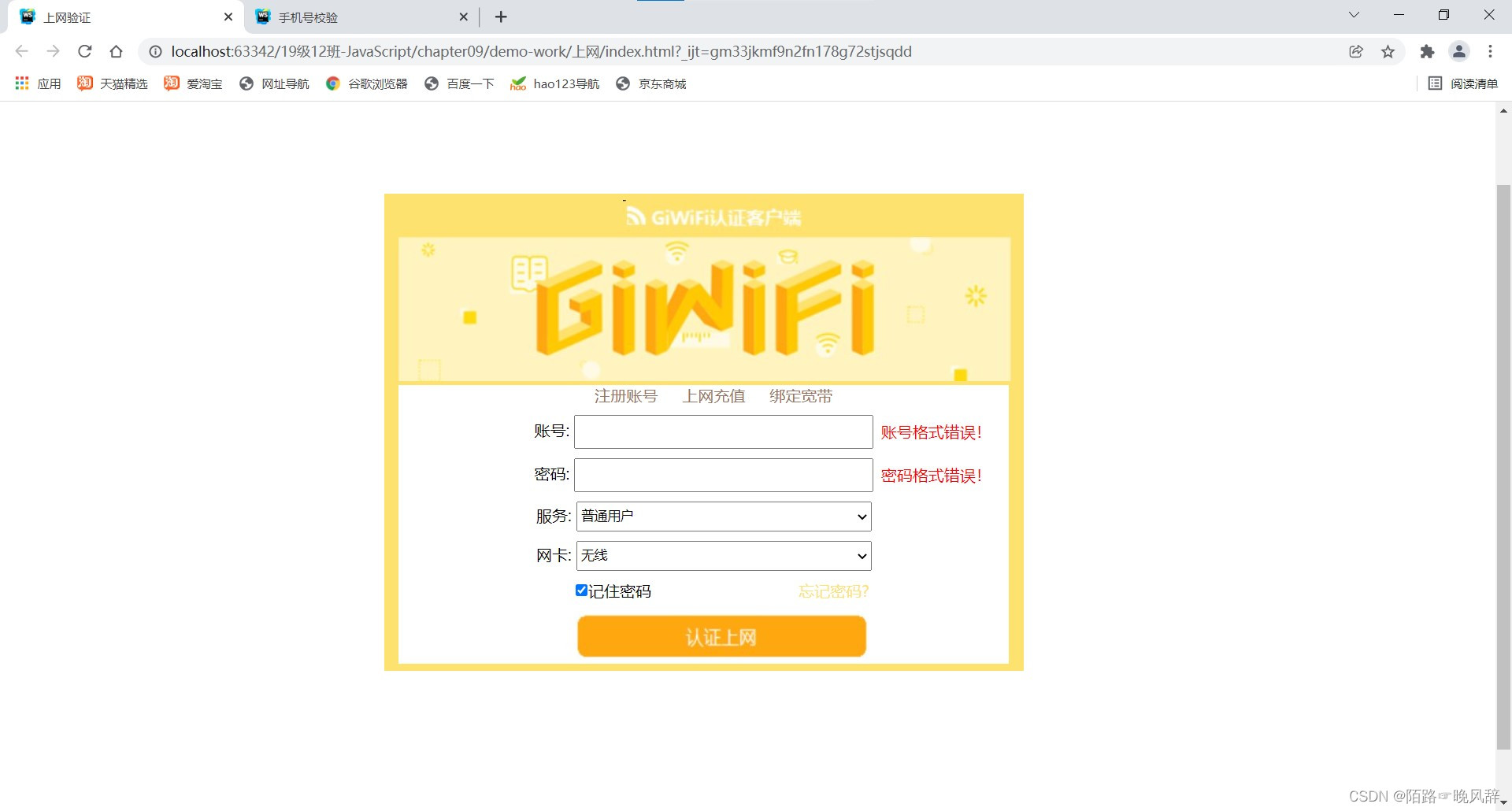
JQuery-表单验证
GiWiFI页面验证登录()表单验证
1.什么是表单验证?
表单验证是javascript中的高级选项之一。
可用来在数据被送往服务器前对 HTML 表单中的这些输入数据进行验证。
2.为什么表单验证?
减轻服务器的压力 。
保证输入的数据符合要求。
3.案例
实现表单验证的案例在这里用GiWiFI页面进行实现。其由三部分构成
1.登录页面
2.登陆成功页面
3.注册页面
4.效果图
1.如果账号和密码为空或与所写的条件不符,则无法登录。
2.下方为输入符合的条件。
3.点击认证上网则进入登陆成功页面。
4.修改密码时无输入信息点击修改密码则修改失败。
5.输入信息后,点击修改密码时则弹出密码修改成功并进入登录页面。
5.实现思路
首先登录、登陆成功、修改密码三个页面相互串联:登录页面正确进入登陆成功页面,点击登录页面的忘记密码进入修改密码,修改成功进入登录页面。
1.登录页面
第一步: 新建一个验证账号的函数A
在新建一个验证账号的函数B
在函数内写入功能:
获取与其对应的两个验证的value值及文本提示框(即红字部分)。
在写入正则表达式
最后判断校验
第二步: 将函数绑定触发事件->失去焦点事件
第三步:用表单提交事件(submit)判断是否提交页面
2.登陆成功页面
在body中创建h1标签并写入(认证成功!欢迎使用!!!)即可;
3.修改密码页面
大致与登录页面相同
只说一下获取随机验证码和密码是否修改成功的判断
验证码:
第一步:为获取验证码按钮写一个点击事件click,在里面用for循环获取随机数并赋给按钮前方的(input)框。
第二步:新建一个验证账号的函数C,获取(input)的value值与条件进行对比,相同则true相同则false。
密码:
为修改密码按钮添加点击事件,将函数A、B、C、赋给变量a、b、c,用判断如果a、b、c结果都是正确则修改成功,如果a、b、c任何一个结果错误则修改失败。
6.代码
1.登录页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>上网登录验证</title>
<script src="../../js/jquery-1.12.4.js"></script>
</head>
<style>
*{
margin: 0;
padding: 0;
}
body{
margin: 180px 390px;
}
#header_1{
width: 650px;
height: 40px;
line-height: 40px;
background: #fee26f;
text-indent: 20px;
}
#header_1>img{
vertical-align: middle;
}
#header_2{
width: 650px;
background: #fee26f;
}
#header_2>img{
margin-left: 3px;
}
#header_3{
width: 620px;
margin-left: 15px;
text-align: center;
background: white;
}
#header_3>a{
margin-left: 20px;
color: #977363;
}
/*主体*/
section{
width: 650px;
height: 270px;
background: #fee26f;
}
form{
width: 620px;
text-align: center;
background: white;
margin-left: 15px;
}
form>p,div{
text-align: center;
}
p>input{
width: 300px;
height: 30px;
line-height: 30px;
margin-top: 10px;
}
select{
width: 300px;
height: 30px;
line-height: 30px;
margin-top: 10px;
}
form>div{
margin-top: 10px;
}
div>input{
margin-left: 38px;
}
div>a{
color: #fee26f;
text-decoration: none;
margin-left: 145px;
}
p:last-of-type>input{
width: 300px;
height: 50px;
line-height: 50px;
margin-left: 37px;
}
#w{
width: 650px;
background: #fee26f;
}
#checkPhone{
position: relative;
top: -254px;
left: 505px;
}
#checkPass{
position: absolute;
top: 455px;
left: 895px;
}
</style>
<body>
<header>
<div id="header_1">
<img src="../../img/GiWiFi-LOGO.jpg" alt="">
</div>
<div id="header_2">
<img src="../../img/GiWiFi-header1.jpg" alt="">
</div>
<div id="w">
<div id="header_3">
<a href="#">注册账号</a>
<a href="#">上网充值</a>
<a href="#">绑定宽带</a>
</div>
</div>
</header>
<section>
<form action="index1.html" method="post" id="form_one">
<p>
<span>账号:</span>
<input type="text" id="first">
</p>
<p>
<span>密码:</span>
<input type="password" id="pass">
</p>
<p>
<span>服务:</span>
<select name="fu" id="FUwu">
<option value="普通用户">普通用户</option>
<option value="中国移动">中国移动</option>
<option value="中国联通">中国联通</option>
</select>
</p>
<p>
<span>网卡:</span>
<select name="WAN" id="wangKA">
<option value="无线">无线</option>
<option value="网卡1">网卡1</option>
<option value="网卡2">网卡2</option>
</select>
</p>
<div>
<input type="checkbox" id="ji" checked="checked"><label for="ji">记住密码</label>
<a href="text.html">忘记密码?</a>
</div>
<p>
<input type="image" src="../../img/button1.jpg">
</p>
</form>
</section>
<span id="checkPhone" style="color: red"></span>
<span id="checkPass" style="color: red"></span>
</body>
<script>
$(document).ready(function () {
//绑定失去焦点事件
$("#first").blur(checkPhone);
$("#pass").blur(checkPwd1);
//表单提交判断
$("#form_one").submit(function () {
var flag = true;
if (!checkPwd1()) {flag = false;}
if (!checkPhone()) {flag = false;}
$("form input").val("");
return flag;
});
//验证账号的方法
function checkPhone() {
var phone = $("#first").val();
var checkPhone = $("#checkPhone");
var phones= /^1\d{10}$/;
checkPhone.html("");
if (phones.test(phone)==false){
checkPhone.html("账号格式错误!");
return false
}
return true
}
//验证密码的方法
function checkPwd1() {
var $pwd = $("#pass").val();
var $checkPwd1 = $("#checkPass");
var paws = /^[0-9]{6,12}$/;
$checkPwd1.html("");
if (paws.test($pwd)==false){
$checkPwd1.html("密码格式错误!");
return false
}
return true
}
})
</script>
</html>
2.登录成功页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>成功页面</title>
</head>
<body>
<h1>认证成功!欢迎使用!!!</h1>
</body>
</html>
3.修改密码页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>修改密码</title>
<script src="../../js/jquery-1.12.4.js"></script>
</head>
<style>
*{
margin: 0;
padding: 0;
}
img:nth-of-type(1){
width: 400px;
height: 300px;
}
body{
width: 400px;
margin: 100px 500px;
}
section{
width: 400px;
background: #ededed;
}
p>input{
margin-top: 15px;
margin-left: 25px;
width: 350px;
height: 40px;
line-height: 40px;
text-indent: 20px;
}
p:nth-of-type(2)>input{
width: 250px;
height: 40px;
line-height: 40px;
}
p:last-of-type>input{
width: 310px;
height: 45px;
line-height: 45px;
margin: 10px 45px;
}
button{
width: 95px;
height: 44px;
line-height: 40px;
background: #e6e6e6;
border: none;
}
</style>
<body>
<section>
<img src="../../img/GiWfi_header.jpg" alt="">
<form action="index.html">
<p>
<input type="text" placeholder="请输入11位手机号" id="phone">
</p>
<p>
<input type="text" id="rom">
<button>获取验证码</button>
</p>
<p>
<input type="text" placeholder="请输入6-16位密码" id="pass">
</p>
<p>
<input type="image" src="../../img/button.jpg">
</p>
</form>
</section>
</body>
<script>
$(document).ready(function () {
//绑定失去焦点事件
$("#phone").blur(checkPhone);
$("#pass").blur(checkPwd1);
//表单提交判断
$("form").submit(function () {
var flag = true;
if (!checkPwd1()) {flag = false;}
if (!checkPwd2()) {flag = false;}
if (!checkPhone()) {flag = false;}
return flag;
});
//验证账号的方法
function checkPhone() {
var phone = $("#phone").val();
var phones= /^1\d{10}$/;
if (phones.test(phone)==false){
return false
}
return true
}
//验证密码的方法
function checkPwd1() {
var $pwd = $("#pass").val();
var paws = /^[0-9]{6,12}$/;
if (paws.test($pwd)==false){
return false
}
return true
}
//验证验证码
//点击事件
var str="";
$("button").click(function () {
str="";
for (var i = 0; i < 4; i++) {
var j = Math.floor(Math.random()*10);//向下取整
str+=j;//累加
}
$("#rom").val(str);
return false
});
//验证验证码方法(函数)
function checkPwd2() {
var $pwd = $("#rom").val();
var paws = /^[0-9]{4}$/;
if (paws.test($pwd)==false){
return false
}
return true
}
//修改密码
$("input:last").click(function () {
var w =checkPhone();
var a =checkPwd2();
var d =checkPwd1();
if (w===true&&a===true&&d===true){
alert("密码修改成功!");
}else{
alert("修改失败!")
}
})
})
</script>
</html>
更多推荐
所有评论(0)