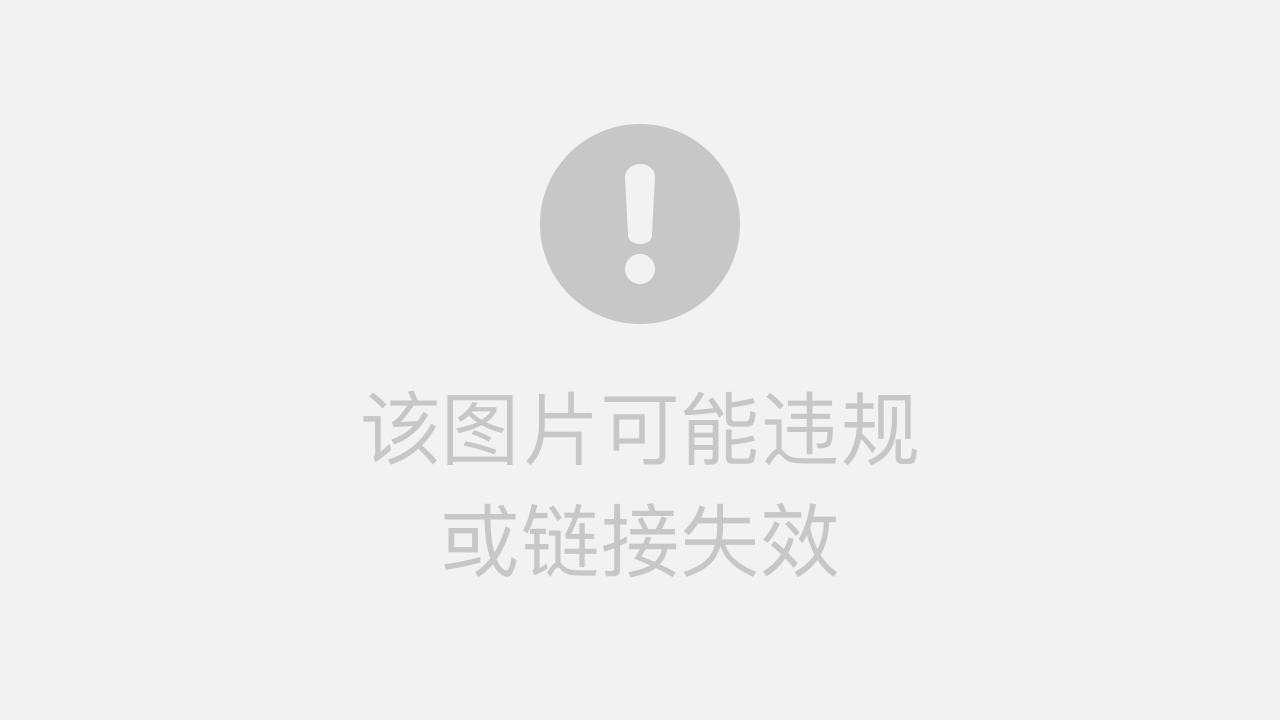
制作京东登陆页面 HTML+CSS
简易版京东登陆页面
先来看一下最终的效果:
HTML的代码如下:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<link href="css/style2.css" rel="stylesheet" type="text/css" />
<script src="js/jquery-3.2.0.min.js"></script>
<script>
$(function() {
$("#smContent").hide();
$("#zhContent").show();
$("#zhLogin").addClass("active");
// 给用户输入框添加onfucs事件
$("#nameInput>input").bind("focus", function() {
// 将对应前面的图片src值换掉
$("#nameInput>img").attr("src", "img/nameInput.png");
});
$("#pwdInput>input").bind("focus", function() {
$("#pwdInput>img").attr("src", "img/pwdInput.png");
});
// 给用户输入框添加onblur事件
$("#nameInput>input").bind("blur", function() {
// 将对应前面的图片src值换掉
$("#nameInput>img").attr("src", "img/name.jpg");
});
$("#pwdInput>input").bind("blur", function() {
$("#pwdInput>img").attr("src", "img/pwd.jpg");
});
$(".loginMenu").click(function() {
var indexLogin = $(this).index();
if (indexLogin == 1) { //扫码登录
//给扫码添加样式
$("#smLogin").addClass("active");
//给账号登录去掉样式
$("#zhLogin").removeClass("active");
//让扫码登录显示
$("#smContent").show();
//让账户登录隐藏
$("#zhContent").hide();
} else { //账号登录
//给扫码去掉样式
$("#smLogin").removeClass("active");
//给账号登录添加样式
$("#zhLogin").addClass("active");
//让扫码登录隐藏
$("#smContent").hide();
//让账户登录显示
$("#zhContent").show();
}
})
});
</script>
</head>
<body>
<div id="headBox">
<img src="img/logo.jpg" height="80px" />
<img src="img/login.jpg" class="welcome" />
<img src="img/msg-login.jpg" class="rightImg" />
<a href="#" class="rightLogin">登录页面调查问卷</a>
</div>
<div id="mainBox">
<img src="img/bj-login.jpg" />
<div id="loginBox">
<div id="hintBox">
<img src="img/icon-tips.png" />
京东不以任何理由要求您转账汇款,谨慎诈骗.
</div>
<span class="loginMenu" id="smLogin">扫码登录</span>
<span class="loginMenu" id="zhLogin">账户登录</span>
<hr />
<div id="smContent">
<img src="img/erweima.jpg" />
<div id="box8">打开<a href="#">手机京东</a> 扫描二维码</div>
<div><img src="img/IMG_3337(20220819-114417).PNG" >免输入
<img src="img/IMG_3336(20220819-114432).PNG" >更快
<img src="img/IMG_3337(20220819-114500).PNG" >更安全
</div>
</div>
<div id="zhContent">
<div class="inputBox" id="nameInput">
<img src="img/name.jpg" width="35px" height="35px" />
<input type="text" name="username" placeholder="请输入用户名" />
</div>
<div class="inputBox" id="pwdInput">
<img src="img/pwd.jpg" width="35px" height="35px" />
<input type="password" name="pwd" placeholder="请输入密码" />
</div>
<a href="#">忘记密码</a>
<div class="inputBox">登录</div>
</div>
<div id="loginFoot">
<img src="img/qq.jpg" />QQ
<img src="img/weixin.jpg" />微信
<a href="#">
<img src="img/arrow.jpg" />立即注册
</a>
</div>
</div>
</div>
<div id="footBox">
<span>关于我们 | 联系我们 | 人才招聘 | 商家入驻 | 广告服务 | 手机京东 | 友情链接 | 销售联盟 | 京东社区 | 京东公益 | English Site</span>
<br />
<span>Copyright © 2004-2017 京东JD.com 版权所有</span>
</div>
</body>
</html>
CSS代码如下:
* {
padding: 0px;
margin: 0px;
}
#headBox {
width: 1000px;
height: 80px;
position: relative;
left: 50%;
margin-left: -500px;
}
.welcome {
position: relative;
top: -27px;
}
.rightLogin {
position: absolute;
bottom: 5px;
right: 0px;
color: gray;
text-decoration: none;
font-size: 12px;
}
.rightImg {
position: absolute;
right: 100px;
bottom: 3px;
}
#mainBox {
width: 100%;
height: 474px;
background-color: #E93854;
position: relative;
}
#mainBox>img {
position: absolute;
left: 450px;
top: 0px;
}
#loginBox {
width: 350px;
height: 430px;
background-color: #ffffff;
position: absolute;
left: 1110px;
top: 15px;
}
#hintBox {
width: 100%;
height: 37px;
background-color: #fff8f0;
text-align: center;
line-height: 37px;
font-size: 12px;
color: darkgray;
}
#hintBox>img {
position: relative;
top: 5px;
}
.loginMenu {
display: inline-block;
margin: 18px 0px;
font-size: 18px;
font-weight: 800;
width: 170px;
text-align: center;
color: gray;
}
.active {
color: red;
}
#smLogin {
border-right: 1px solid gray;
}
#smContent,
#zhContent {
width: 100%;
height: 280px;
position: absolute;
left: 0px;
top: 95px;
border-bottom: 1px solid gray;
}
#loginFoot {
width: 100%;
height: 50px;
background-color: #fefefe;
position: absolute;
bottom: 0px;
line-height: 50px;
}
#loginFoot img {
position: relative;
top: 6px;
}
#loginFoot>a {
color: red;
text-decoration: none;
}
#smContent>img {
position: absolute;
left: 90px;
top: 30px;
cursor: pointer;
}
#footBox {
width: 730px;
height: 50px;
margin: 10px auto;
font-size: 12px;
color: gray;
text-align: center;
}
.inputBox {
width: 300px;
height: 35px;
border: 1px solid gray;
margin: 15px 25px;
text-align: center;
line-height: 35px;
}
#zhContent>a {
position: relative;
right: -265px;
text-decoration: none;
color: purple;
}
#nameInput,
#pwdInput {
margin-top: 35px;
margin-bottom: 35px;
}
.inputBox>input {
width: 255px;
height: 32px;
position: relative;
top: -13px;
font-size: 18px;
border: none;
outline: none;
}
#box8{
position: relative;
left: 90px;
top: 200px;
}
a{
color: red;
text-decoration: none;
}
更多推荐
所有评论(0)