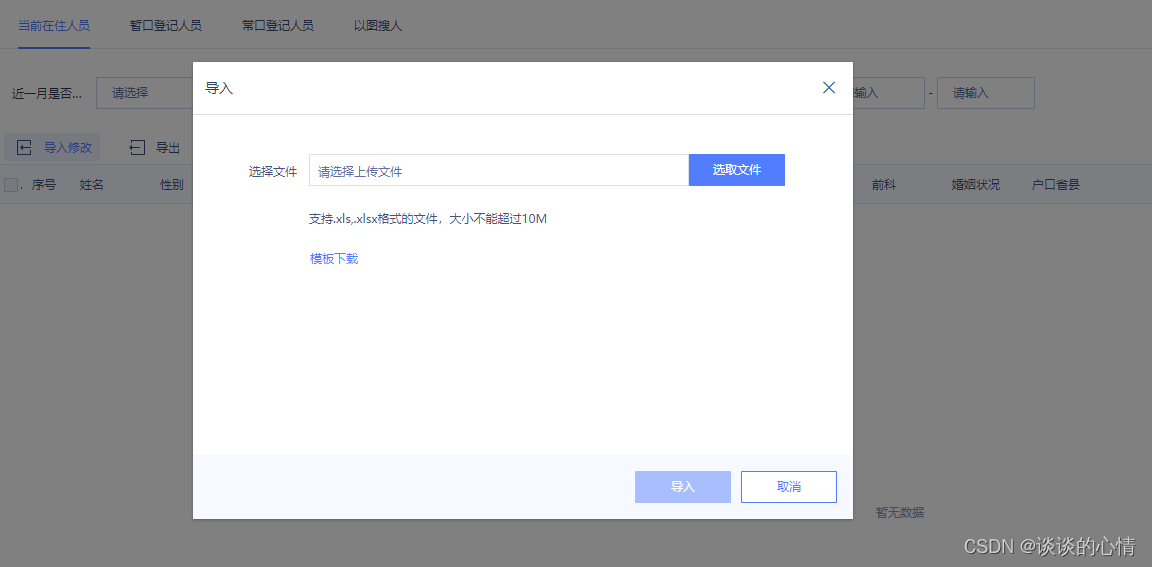
Vue项目文件导入、导出
Vue项目中文件导入和导出使用比较频繁,这里把最常用的布局和实现代码记录下来,避免以后频繁造轮子。
·
Vue项目中文件导入和导出使用比较频繁,这里把最常用的布局和实现代码记录下来,避免以后频繁造轮子。
1、导出一般就是在列表中选择几条数据,然后点击导出按钮把选中的数据下载下来;导入的话一般会有一个单独的弹框页面,需要先选择文件,然后统一导入。
导入弹框
2、导入弹框对应的页面代码:
<!--
* @Author: lujinwei
* @Date: 2022-08-13 17:29:58
* @LastEditTime: 2022-08-30 14:22:20
* @Description: 导入数据
-->
<template>
<el-dialog
title="导入"
:visible.sync="dialogVisible"
width="660px"
:close-on-click-modal="false"
:before-close="onClose"
@closed="onAfterClose"
>
<div
v-loading="loading"
class="dialog-content"
>
<el-form
ref="formRef"
:model="formData"
label-width="80px"
>
<el-form-item
label="选择文件"
prop="fileList"
>
<el-upload
ref="upload"
action="#"
:accept="extAcceptStr"
:auto-upload="false"
:limit="limit"
:file-list="formData.fileList"
:on-change="onFileChange"
:on-remove="onFileMove"
:on-exceed="onFileExceed"
>
<div class="select-file">
<p class="select-p">
请选择上传文件
</p>
<el-button
size="small"
type="primary"
>
选取文件
</el-button>
</div>
<div
slot="tip"
class="el-upload__tip"
>
{{ `支持${extAcceptStr}格式的文件,大小不能超过10M` }}
</div>
</el-upload>
<el-button
type="text"
@click="onDownload"
>
模板下载
</el-button>
</el-form-item>
</el-form>
</div>
<template slot="footer">
<el-button
type="primary"
:loading="!!loading"
:disabled="!formData.fileList.length > 0"
@click="onSubmit"
>
导入
</el-button>
<el-button @click="dialogVisible = false">
取消
</el-button>
</template>
</el-dialog>
</template>
<script>
export default {
props: {
loading: Boolean,
limit: { //限制文件上传个数
type: Number,
default: 1,
},
},
data () {
return {
// # config
extAccept: ["xls", "xlsx"],
// # state
dialogVisible: false,
// # data
formData: {
fileList: [],
},
}
},
computed: {
extAcceptStr () {
return this.extAccept.map(ext => `.${ext}`).join(",")
},
},
methods: {
onShow () {
this.dialogVisible = true
},
onClose () {
this.dialogVisible = false
},
onAfterClose () {
this.formData = {
fileList: [],
}
},
// # upload
// 校验
validateFile (file) {
if (!file) return "至少添加一个文件!"
const ext = (file.name || "").split(".").reverse()[0]
if (!this.extAccept.includes(ext))
return `仅支持${this.extAcceptStr}格式的文件`
return ""
},
onFileChange (file, fileList) {
const errMsg = this.validateFile(file)
if (errMsg) {
this.$message.warning(errMsg)
this.formData.fileList.splice(0, 1)
} else {
this.formData.fileList = fileList
}
},
onFileMove (file, fileList) {
this.formData.fileList = fileList
},
onFileExceed () {
this.$message.warning(`仅允许上传 ${this.limit} 个文件!`)
},
// # emit
// 模板下载
onDownload () {
this.$emit("download")
},
// 导入
onSubmit () {
this.$emit("submit", this.formData)
},
},
}
</script>
<style lang="less" scope>
.dialog-content {
height: 300px;
padding: 16px;
.select-file {
display: flex;
height: 36px;
.select-p {
width: 380px;
height: 32px;
line-height: 32px;
border: 1px solid #dcdfe6;
text-align: left;
padding-left: 8px;
color: #636fa1;
font-size: 12px;
}
}
}
</style>
3、在父布局中使用弹框组件:
①导入
②布局中使用
<dialog-import
ref="dialogImportRef"
:loading="importLoading"
@download="onTempDownload"
@submit="onImportSubmit"
/>
③子组件对应的方法
----------------------------------------------- 模板下载相关 -------------------------------------------
//模板下载
onTempDownload () {
inlivePersonApi.downloadTemplate('1').then(res => {
console.log(res)
saveBlobToFile(res)
})
}
// 下载人员导入修改模板
downloadTemplate: (templateKey) => http.getBlob(`/v1/apartment/user/downloadTemplate?templateKey=${templateKey}`)
saveBlobToFile是在公共方法中封装的下载方法:
export function saveBlobToFile (res, filename) {
if (res.headers['content-disposition']) {
var fileName = decodeURIComponent(res.headers['content-disposition'].replace('attachment;filename=', ''))
fileName = decodeURIComponent(fileName)
var blob = res.data
// var blob = new Blob([res.data], {
// type: 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet'
// })
if (navigator.msSaveBlob) {
navigator.msSaveBlob(blob, fileName)
} else {
const oA = document.createElement('a')
try {
oA.href = URL.createObjectURL(blob)
} catch (err) {
const binaryData = []
binaryData.push(blob)
oA.href = window.URL.createObjectURL(new Blob(binaryData, { type: 'application/zip' }))
}
oA.download = filename || fileName
oA.click()
}
}
}
----------------------------------------------- 导入相关 -------------------------------------------
async onImportSubmit ({ fileList }) {
this.importLoading = true
let fd = new FormData()
fd.append('excelFile', fileList[0].raw)
inlivePersonApi.importExcel(fd).then(res => {
this.importLoading = false
if (res.success) {
this.$refs.dialogImportRef.onClose()
this.pageParams.pageNo = 1
this.requestTableData()
this.$message({
message: '导入成功',
type: 'success'
})
}
})
}
// 人员导入
importExcel: params => http.post('/v1/apartment/user/importExcel', params)
4、导出业务其实和上面的模板下载业务一样,也是通过接口获取数据,然后通过文件形式下载下来。
更多推荐
所有评论(0)