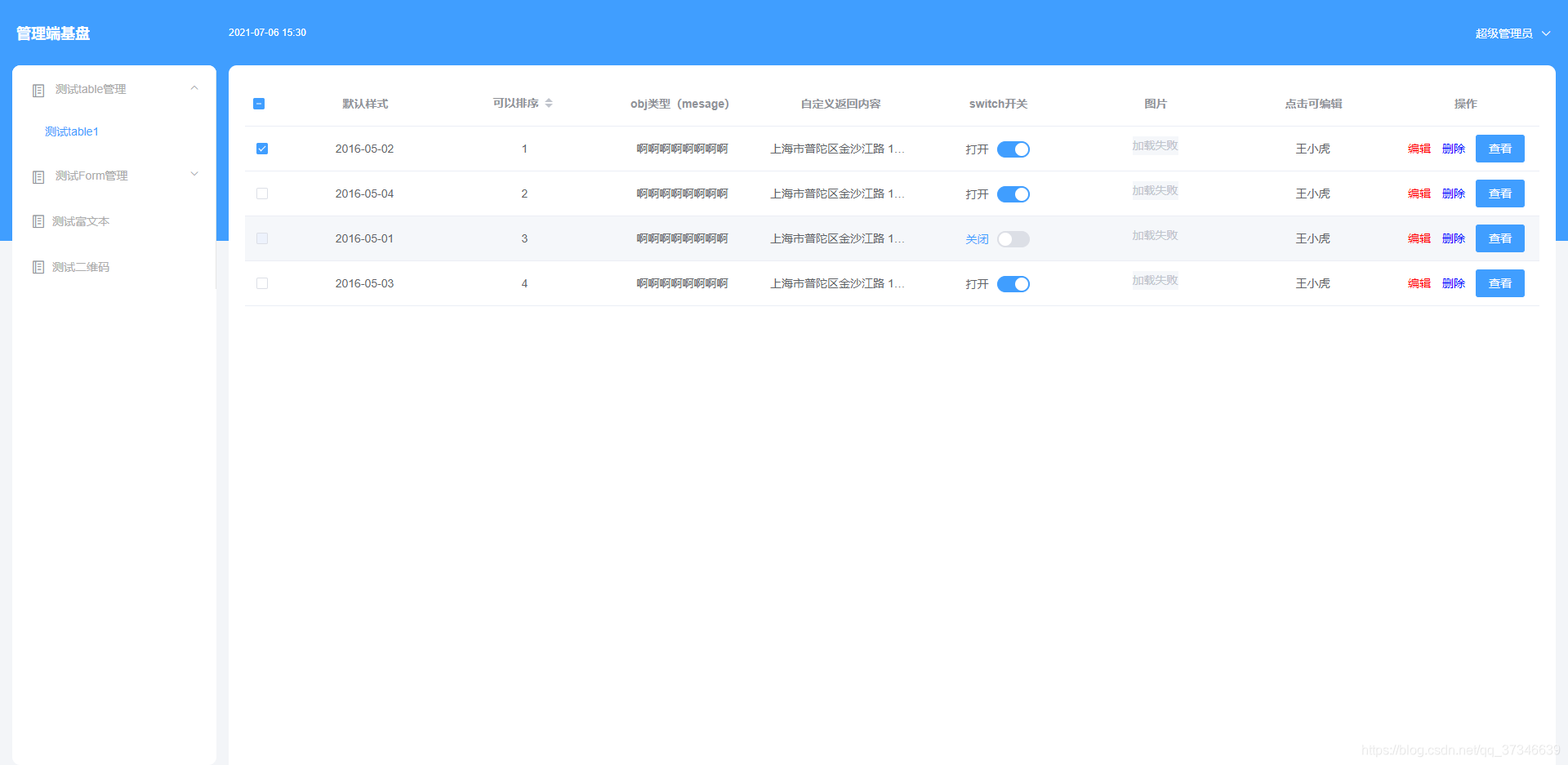
el-table封装,elementtable封装,eltable封装详解(含分页)
前言:个人理解所谓封装,就是把经常都要公用的东西,拿出来,可以多次复用。公用方法,公用页面都可以封装。其实封装也并不是有多难,思路也很简单,就是用JS来控制页面。页面动态性越强,组件越灵活,适用范围越广。就vue+element的组件封装而言,先把所有功能在子页面实现。然后把js里面的动态值,拿到父组件里面去传过来,就完成了,其中技术也就掌握父子组件传值而已。1、下面就是el-table的一个简单
·
首次发布时间:2021-04-09 18:23:36
最后更新时间:2022-09-24 20:51
前言:仔细看懂本篇博客,玩转element table 不成问题 ,个人理解所谓封装,就是把经常都要公用的东西,拿出来,可以多次复用。公用方法,公用页面都可以封装。
其实封装也并不是有多难,思路也很简单,就是用JS来控制页面。页面动态性越强,组件越灵活,适用范围越广。
就vue+element的组件封装而言,先把所有功能在子页面实现。然后把js里面的动态值,拿到父组件里面去传过来,就完成了,其中技术也就掌握父子组件传值而已。
本次封装包含功能:多选(单行根据条件禁用)、排序、常见状态{code,msg}、自定义column内容、switch开关、图片、点击任意不含前面类型的文字,可编辑、行操作。基本满足于常见table列表
1、组件
<!--
@author Zou Tiancong
@version 创建时间:2021年4月8日 下午4:53:36
@return
-->
<template>
<div>
<el-table size="medium" :data="tableData" :stripe="false" :border="false" :fit="true" :show-header="true"
:highlight-current-row="true" v-loading="columObj.loading" :row-class-name="tableRowClassName"
@row-click="rowClick">
<!-- 选择框是否开启,selectable控制是否单行禁用 -->
<el-table-column v-if="columObj.selection" type="selection" :selectable="columObj.selectable" width="50px"/>
<!-- 普通列 -->
<el-table-column v-for="(column,columIndex) in columObj.columnData" :key="columIndex" :prop="column.prop"
:label="column.label" :width="column.width" :fixed="column.fixed" :align="column.align || 'center'"
:sortable="column.sortable" :index="columIndex" show-overflow-tooltip>
<template slot-scope="{row,$index}">
<!-- 默认展示 -->
<span v-if="column.text && column.editRow != $index">{{row[column.prop]}}</span>
<!-- 状态对象展示 -->
<span v-if="column.status && row[column.prop]">{{row[column.prop].msg}}</span>
<!-- 自定义内容 -->
<span v-if="column.ownDefined">{{column.ownDefinedReturn(row,$index)}}</span>
<!-- switch开关 -->
<el-switch v-if="column.switch" v-model="row[column.prop]" :inactive-text="row[column.prop] ? column.openText:column.closeText" @change="switchChange(row,$index,column.prop)"/>
<!-- 图片展示 -->
<el-popover trigger="hover" placement="top" popper-class="popper">
<img v-if="column.image" :src="viewUrl + row[column.prop]" />
<el-image slot="reference" v-if="column.image" :src="viewUrl + row[column.prop]"/>
</el-popover>
<!-- 图片数组 -->
<el-popover v-if="column.imageArr" trigger="hover" placement="top" popper-class="popper">
<img v-if="row[column.prop].length>0" :src="row[column.prop][0]" />
<el-image slot="reference" v-if="row[column.prop].length >0" :src="row[column.prop][0]":preview-src-list="row[column.prop]"/>
</el-popover>
<!-- 可编辑input,仅在text默认展示类型才可编辑-->
<el-input v-focus v-if="column.editRow == $index" v-model="row[column.prop]" @blur="editInputBlur(row,$index,column.prop,columIndex)"/>
<!-- 操作按钮 -->
<span v-if="column.isOperation" v-for="(operations, index) in column.operation" :key="index">
<el-button v-if="operations.isShow(row,$index)" :icon="operations.icon" :type="operations.type" @click="operations.buttonClick(row,$index)" :style="{color:operations.color}" size="small">{{operations.label}}</el-button>
</span>
</template>
</el-table-column>
</el-table>
<!-- 分页 -->
<div class="page_div" :style="{textAlign: pageObj.position || 'center'}">
<el-pagination @size-change="handleSizeChange" @current-change="handleCurrentChange"
:hide-on-single-page="false" :current-page="pageObj.pageData.page" :pager-count="7"
:page-sizes="[10, 15, 20, 30,50]" :page-size="pageObj.pageData.size" background
layout="total,sizes,prev, pager, next" :total="pageObj.total">
</el-pagination>
</div>
</div>
</template>
<script>
export default {
directives: {
// 自定义指令,用于可编辑input自动获取焦点
focus: {
inserted: function(e) {
e.querySelector('input').focus()
}
}
},
props: {
tableData: {
type: Array,
required: true
},
columObj: {
type: Object,
required: true
},
//columObj.type(如果为""空,就不会加载多选框,或者index编号),lazy(是否支持懒加载)
//columnData.columType(列类型,可选text(默认为普通文字模式),input(input可编辑框),switch(switch开关),image(图片),operation(操作按钮))
//prop(参数),label(列名),width(宽度),align(对齐方式),sortable(是否支持排序)
//如果为操作列,则需要填写需要的操作按钮,类型为Object。type(按钮样式,参考el—botton类型),label(按钮文字)icon(参考el-icon),color(字体颜色),buttonClick为点击后调用的方法名称
pageObj: {
type: Object,
required: true
}
},
data() {
let readUploadFileUrl = this.$store.state.user.readUploadFileUrl;
return {
viewUrl: readUploadFileUrl,
}
},
methods: {
// switchChange调用
switchChange(row, $index, prop) {
this.$emit("switchChange", row, $index, prop);
},
// 帮助点击行,获取点击的下标
tableRowClassName({
row,
rowIndex
}) {
row.rowIndex = rowIndex;
},
// 点击行
rowClick(row, column, event) {
this.$emit("rowClick", row, column, event);
},
// 可编辑input失去焦点
editInputBlur(row, $index, prop, columIndex) {
this.$emit('editInputBlur', row, $index, prop, columIndex);
},
// 条数变化
handleSizeChange(e) {
this.$emit('handleSizeChange', e);
},
// 页码变化
handleCurrentChange(e) {
this.$emit('handleCurrentChange', e);
}
}
}
</script>
<style lang="scss" scoped>
.el-button {
margin: 0 6px;
}
::v-deep .el-input__inner {
border: none;
}
::v-deep .el-image__inner {
height: 50px;
}
// switch左边文字颜色
::v-deep .el-switch__label--left {
color: #606266;
}
img {
height: 400px;
}
.page_div {
padding: 15px 0;
}
</style>
2、父组件调用
<template>
<div>
<publicTable :tableData="tableData" :columObj="columObj" :pageObj="pageObj" @switchChange="switchChange" @editInputBlur="editInputBlur" @rowClick="rowClick"
@handleSizeChange="handleSizeChange" @handleCurrentChange="handleCurrentChange">
</publicTable>
</div>
</template>
<script>
export default {
components: {},
data() {
return {
pageObj: { //分页对象
position: "left", //分页组件位置
total: 100,
pageData: {
page: 1,
size: 10
}
},
tableData: [{
id: '1',
date: '2016-05-02',
date: '2016-05-02',
name: '王小虎',
address: '上海市普陀区金沙江路 1518 弄',
switchs: true,
img: 'file/964355654_58490652-91c5-412e-a74b-0c3d86ff1525.png',
objmsg: {
msg: '啊啊啊啊啊啊啊啊'
}
}, {
id: '2',
date: '2016-05-04',
name: '王小虎',
address: '上海市普陀区金沙江路 1517 弄',
switchs: true,
img: 'file/964355654_58490652-91c5-412e-a74b-0c3d86ff1525.png',
objmsg: {
msg: '啊啊啊啊啊啊啊啊'
}
}, {
id: '3',
date: '2016-05-01',
name: '王小虎',
address: '上海市普陀区金沙江路 1519 弄',
switchs: true,
img: 'file/964355654_58490652-91c5-412e-a74b-0c3d86ff1525.png',
objmsg: {
msg: '啊啊啊啊啊啊啊啊'
}
}, {
id: '4',
date: '2016-05-03',
name: '王小虎',
address: '上海市普陀区金沙江路 1516 弄',
switchs: true,
img: 'file/964355654_58490652-91c5-412e-a74b-0c3d86ff1525.png',
objmsg: {
msg: '啊啊啊啊啊啊啊啊'
}
}],
columObj: {
// 选择框
selection: true,
// 选择框根据条件是否可选
selectable: (row, index) => {
if (row.switchs) {
return true;
}
},
lazy: "true",
//column列,columType(列类型,可选text(默认为普通文字模式),input(input可编辑框),switch(switch开关),image(图片),operation(操作按钮))
//prop(参数),label(列名),width(宽度),align(对齐方式),sortable(是否支持排序)
columnData: [{
text: true,
prop: "date",
label: "默认样式",
width: "",
align: "center",
}, {
text: true,
prop: "id",
label: "可以排序",
width: "",
align: "center",
sortable: true, //开启排序
},
{
status: true,
prop: "objmsg",
label: "obj类型(mesage)",
width: "",
align: "center",
sortable: false,
},
{
ownDefined: true,
prop: "address",
label: "自定义返回内容",
width: "",
align: "center",
sortable: false,
ownDefinedReturn: (row, $index) => {
return row.address
}
},
{
switch: true,
prop: "switchs",
label: "switch开关",
width: "",
align: "center",
openText: "打开",
closeText: "关闭",
sortable: false,
},
{
image: true,
prop: "img",
label: "图片",
width: "",
align: "center",
sortable: false,
},
{
text: true,
editRow: null,
prop: "name",
label: "点击可编辑",
width: "",
align: "center",
sortable: false,
},
//如果为操作列,则需要填写需要的操作按钮,类型为Object。operation(操作类型,可选edit,delete,see),type(按钮样式,参考el—botton类型),label(按钮文字)icon(参考el-icon),color(字体颜色)
{
isOperation: true,
label: "操作",
width: "180",
align: "center",
sortable: false,
operation: [{
type: "text",
label: "编辑",
icon: "",
color: 'red',
buttonClick:this.rowOperation,
isShow: (row, $index) => {
return true;
}
}, {
type: "text",
label: "删除",
icon: "",
color: 'blue',
buttonClick:undefined,
isShow: (row, $index) => {
return true;
}
}, {
type: "primary",
label: "查看",
icon: "",
color: '',
buttonClick:undefined,
isShow: (row, $index) => {
return true;
}
}]
},
],
},
}
},
beforeCreate() {},
created: function() { //在模板渲染成html前调用
},
beforeMount() {
},
mounted() { //在模板渲染成html后调用
},
beforeUpdate() {
},
updated() {
},
methods: {
rowOperation(row, $index) {
console.log(row, $index)
},
switchChange(row, $index, prop) {
console.log(row, $index, prop)
},
rowClick(row, column, event) {
// 点击行触发,编辑点击的所在列,排除selection选择框
if (column.type != 'selection' && this.columObj.columnData[column.index].editRow === null) {
this.columObj.columnData[column.index].editRow = row.rowIndex;
}
},
editInputBlur(row, $index, prop, columIndex) {
this.columObj.columnData[columIndex].editRow = null;
},
//页码变化
handleCurrentChange(e) {
this.pageObj.pageData.page = e;
},
//条数变化
handleSizeChange(e) {
this.pageObj.pageData.size = e;
this.pageObj.pageData.page = 1;
},
},
watch: { //监听
},
destroyed() { //销毁后
}
}
</script>
<style lang="scss">
</style>
3、附上效果截图,所有代码复制可用,注释也写的很清楚明了!
更多推荐
所有评论(0)