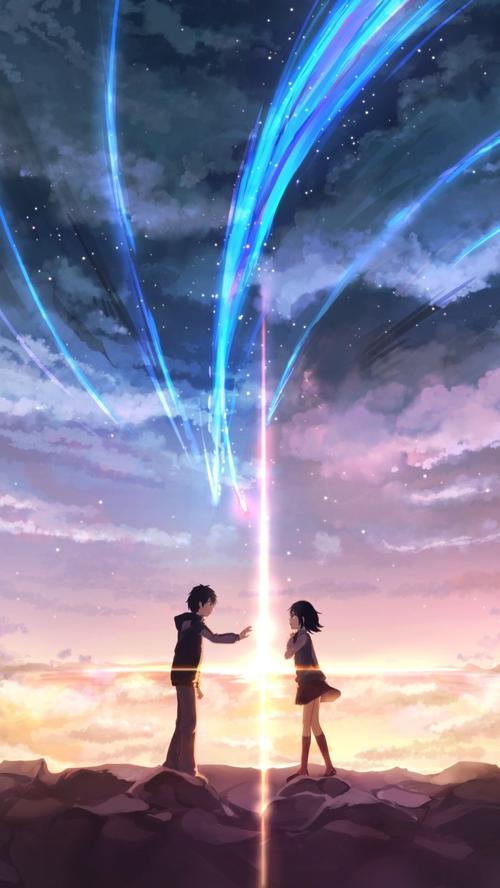
antd 表格设置动态列(动态表头)
1、需求:勾选中某个项,表格就展示对应的数据,反之则不显示2、表格的 columns 动态赋值(刚开始默认全部不展示)html:<div class="checkBox"><a-checkbox @change="changeData" :checked="dataRate">数据传输完整率</a-checkbox><a-checkbox @change=
·
1、需求:勾选中某个项,表格就展示对应的数据,反之则不显示
2、表格的 columns 动态赋值(刚开始默认全部不展示)
html:
<div class="checkBox">
<a-checkbox @change="changeData" :checked="dataRate">
数据传输完整率
</a-checkbox>
<a-checkbox @change="changeTimely" :checked="timelyRate">
及时率
</a-checkbox>
<a-checkbox @change="changeMutation" :checked="mutationRate">
突变率
</a-checkbox>
<a-checkbox @change="changeFault" :checked="faultRate">
故障率
</a-checkbox>
<a-checkbox @change="changeAlarm" :checked="alarmRate">
告警率
</a-checkbox>
</div>
<div class="groupManage-table">
<a-table
:columns="columns"
:data-source="tableData"
rowKey="deviceId"
:loading="dataLoad"
:pagination="pagination"
@change="changePages"
>
<template slot="ownerUnit" slot-scope="text">
<span v-if="text == '1'">本项目</span>
<span v-if="text == '2'">区水务局</span>
<span v-if="text == '3'">排水公司</span>
<span v-if="text == '4'">其他</span>
<span v-if="text == '5'">全部</span>
<span v-if="text == ''">--</span>
</template>
<template slot="dataCompleteRate" slot-scope="text">
<span>{{ text }}%</span>
</template>
<template slot="timelinessRate" slot-scope="text">
<span>{{ text }}%</span>
</template>
<template slot="mutationRate" slot-scope="text">
<span>{{ text }}%</span>
</template>
<template slot="failureRate" slot-scope="text">
<span>{{ text }}%</span>
</template>
<template slot="alarmRate" slot-scope="text">
<span>{{ text }}%</span>
</template>
<template slot="action" slot-scope="record">
<a href="javascript:void(0);" @click="handleDetail(record)"
>查看</a
>
</template>
<template slot="expandedRowRender" slot-scope="record">
<row-detail :record="record" />
</template>
</a-table>
</div>
js:
data() {
return {
dataRate: false,
timelyRate: false,
mutationRate: false,
faultRate: false,
alarmRate: false,
};
},
created() {
this.columns = [
{
title: "设备类型",
dataIndex: "deviceTypeName",
ellipsis: true,
},
{
title: "设备编码",
dataIndex: "deviceCode",
width: 140,
},
{
title: "运维单位",
dataIndex: "ownerUnit",
scopedSlots: { customRender: "ownerUnit" },
ellipsis: true,
},
{
title: "操作",
width: 70,
scopedSlots: { customRender: "action" },
},
];
},
watch: {
// 动态改变表头
dataRate: function (val) {
if (val) {
// 为true添加到表格
this.columns.splice(this.columns.length - 1, 0, {
title: "数据传输完整率",
dataIndex: "dataCompleteRate",
scopedSlots: { customRender: "dataCompleteRate" },
width: 120,
});
} else {
// 为false不显示在表格
this.noRepeat(this.columns, {
title: "数据传输完整率",
dataIndex: "dataCompleteRate",
scopedSlots: { customRender: "dataCompleteRate" },
width: 120,
});
}
},
timelyRate: function (val) {
if (val) {
this.columns.splice(this.columns.length - 1, 0, {
title: "及时率",
dataIndex: "timelinessRate",
scopedSlots: { customRender: "timelinessRate" },
width: 90,
});
} else {
this.noRepeat(this.columns, {
title: "及时率",
dataIndex: "timelinessRate",
scopedSlots: { customRender: "timelinessRate" },
width: 90,
});
}
},
mutationRate: function (val) {
if (val) {
this.columns.splice(this.columns.length - 1, 0, {
title: "突变率",
dataIndex: "mutationRate",
scopedSlots: { customRender: "mutationRate" },
width: 90,
});
} else {
this.noRepeat(this.columns, {
title: "突变率",
dataIndex: "mutationRate",
scopedSlots: { customRender: "mutationRate" },
width: 90,
});
}
},
faultRate: function (val) {
if (val) {
this.columns.splice(this.columns.length - 1, 0, {
title: "故障率",
dataIndex: "failureRate",
scopedSlots: { customRender: "failureRate" },
width: 90,
});
} else {
this.noRepeat(this.columns, {
title: "故障率",
dataIndex: "failureRate",
scopedSlots: { customRender: "failureRate" },
width: 90,
});
}
},
alarmRate: function (val) {
if (val) {
this.columns.splice(this.columns.length - 1, 0, {
title: "告警率",
dataIndex: "alarmRate",
scopedSlots: { customRender: "alarmRate" },
width: 90,
});
} else {
this.noRepeat(this.columns, {
title: "告警率",
dataIndex: "alarmRate",
scopedSlots: { customRender: "alarmRate" },
width: 90,
});
}
},
},
3、需要用到两个方法,一是判断对象相等;二是不通过下标删除数组的某一项(数组的项为对象)
// 判断两个对象是否相等
compareJsonObj(obj1, obj2) {
let result = true;
if (!isJsonObj(obj1) || !isJsonObj(obj2)) return false;
for (let key in obj1) {
if (
(obj1[key] && !obj2[key]) ||
(!obj1[key] && obj2[key]) ||
(obj1[key] &&
obj2[key] &&
obj1[key].toString() !== obj2[key].toString())
) {
result = false;
break;
}
}
return result;
// 判断一个对象是否是 json 对象
function isJsonObj(data) {
return (
data && Object.prototype.toString.call(data) === "[object Object]"
);
}
},
// 不通过下标删除数组中的项(项为对象)
noRepeat(arr1, item) {
arr1.filter((its, index) => {
if (this.compareJsonObj(its, item)) {
arr1.splice(index, 1);
}
});
return arr1;
},
4、代码是多了点儿,但完成了需求
生活没有不请自来的幸运,只有有备而来的惊艳
更多推荐
所有评论(0)