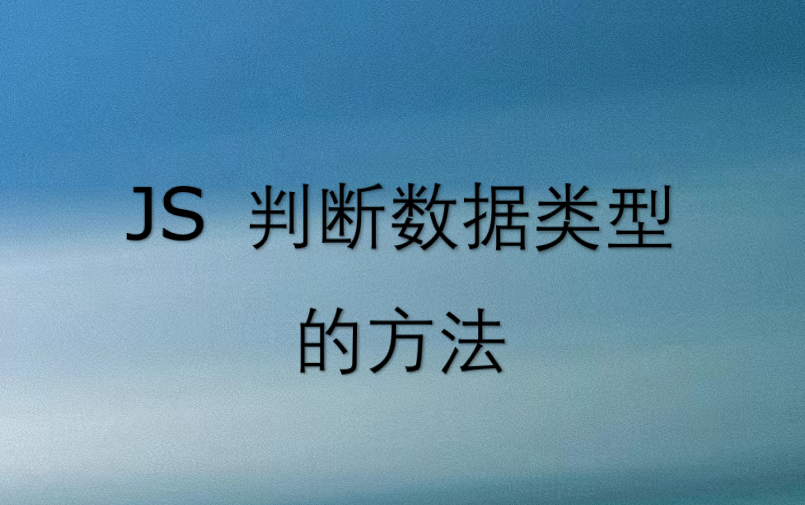
JS 判断数据类型的方法
目录一、JS 中的数据类型二、JS 中判断数据类型的方法1、使用 typeof2、使用 instanceof3、使用Object.prototype.toString.call4、使用constructor一、JS 中的数据类型ECMAScript中有5中简单数据类型(也称为基本数据类型):Undefined、Null、Boolean、Number和String。还有1中复杂的数据类型————Ob
目录
3、使用Object.prototype.toString.call
一、JS 中的数据类型
ECMAScript中有5中简单数据类型(也称为基本数据类型): Undefined、Null、Boolean、Number和String。还有1中复杂的数据类型————Object,Object本质上是由一组无序的名值对组成的。
其中Undefined、Null、Boolean、Number都属于基本类型。
Object、Array和Function则属于引用类型,
数据类型 | 说明 |
---|---|
数值类型Number | 只能是数字或者小数 |
字符串类型String | 字符串类型String,用单引号或者双引号包裹的任何字符 |
布尔类型Boolean | 只能是true或false代表真假 |
未定义undefined | 定义变量后不赋值,这个变量就是undefined |
空null,是对象类型, | null值表示一个空对象指针 |
对象类型object | 有很多种,如数组对象、数学对象、日期对象 |
二、JS 中判断数据类型的方法
1、使用 typeof
let obj={
name:'dawn',
age:21
}
let fn=function() {
console.log('我是 function 类型');
}
console.log(typeof 1); //number
console.log(typeof 'abc'); //string
console.log(typeof true); //boolean
console.log(typeof undefined); //undefined
console.log(typeof fn); //function
console.log(typeof (new Date) ); //object
console.log(typeof null); //object
console.log(typeof [1,2,3]); //object
console.log(typeof obj); //object
由结果可知typeof可以测试出number
、string
、boolean
、undefined
及function
,
【注意】:对于null
及数组、对象,typeof均检测出为object,不能进一步判断它们的类型。
2、使用 instanceof
obj instanceof Object ,可以左边放你要判断的内容,右边放类型来进行JS类型判断,只能用来判断复杂数据类型,因为instanceof 是用于检测构造函数(右边)的 prototype
属性是否出现在某个实例对象(左边)的原型链上。
let arr=[1,2,3,4,5,6,7]
let obj={
name:'dawn',
age:21
}
let fn=function() {
console.log('我是 function 类型');
}
console.log(arr instanceof Array); //true
console.log(obj instanceof Object); //true
console.log(fn instanceof Function); //true
console.log((new Date) instanceof Date); //true
3、使用Object.prototype.toString.call
let obj = {
name: 'dawn',
age: 21
}
let fn = function () {
console.log('我是 function 类型');
}
console.log(Object.prototype.toString.call(1)); // [object Number]
console.log(Object.prototype.toString.call('Hello tomorrow')); // [object String ]
console.log(Object.prototype.toString.call(true)); // [object Boolean]
console.log(Object.prototype.toString.call(undefined)); // [object Undefined]
console.log(Object.prototype.toString.call(fn)); // [object Function]
console.log(Object.prototype.toString.call(new Date)); // [object Date]
console.log(Object.prototype.toString.call(null)); // [object Null]
console.log(Object.prototype.toString.call([1, 2, 3])); // [object Array]
console.log(Object.prototype.toString.call(obj)); // [object Object]
在任何值上调用 Object 原生的 toString() 方法,都会返回一个 [object NativeConstructorName] 格式的字符串。每个类在内部都有一个 [[Class]] 属性,这个属性中就指定了上述字符串中的构造函数名。
但是它不能检测非原生构造函数的构造函数名。
4、使用constructor
let arr = [1, 2, 3, 4, 5, 6, 7]
let obj = {
name: 'dawn',
age: 21
}
let fn = function () {
console.log('我是 function 类型');
}
console.log((9).constructor === Number); //true
console.log('hello'.constructor === String); //true
console.log(true.constructor === Boolean); //true
console.log(fn.constructor === Function); //true
console.log((new Date).constructor === Date); //true
console.log(obj.constructor === Object); //true
console.log([1, 2, 3].constructor === Array); //true
【注意】:constructor不能判断undefined和null,并且使用它是不安全的,因为contructor的指向是可以改变的
更多推荐
所有评论(0)