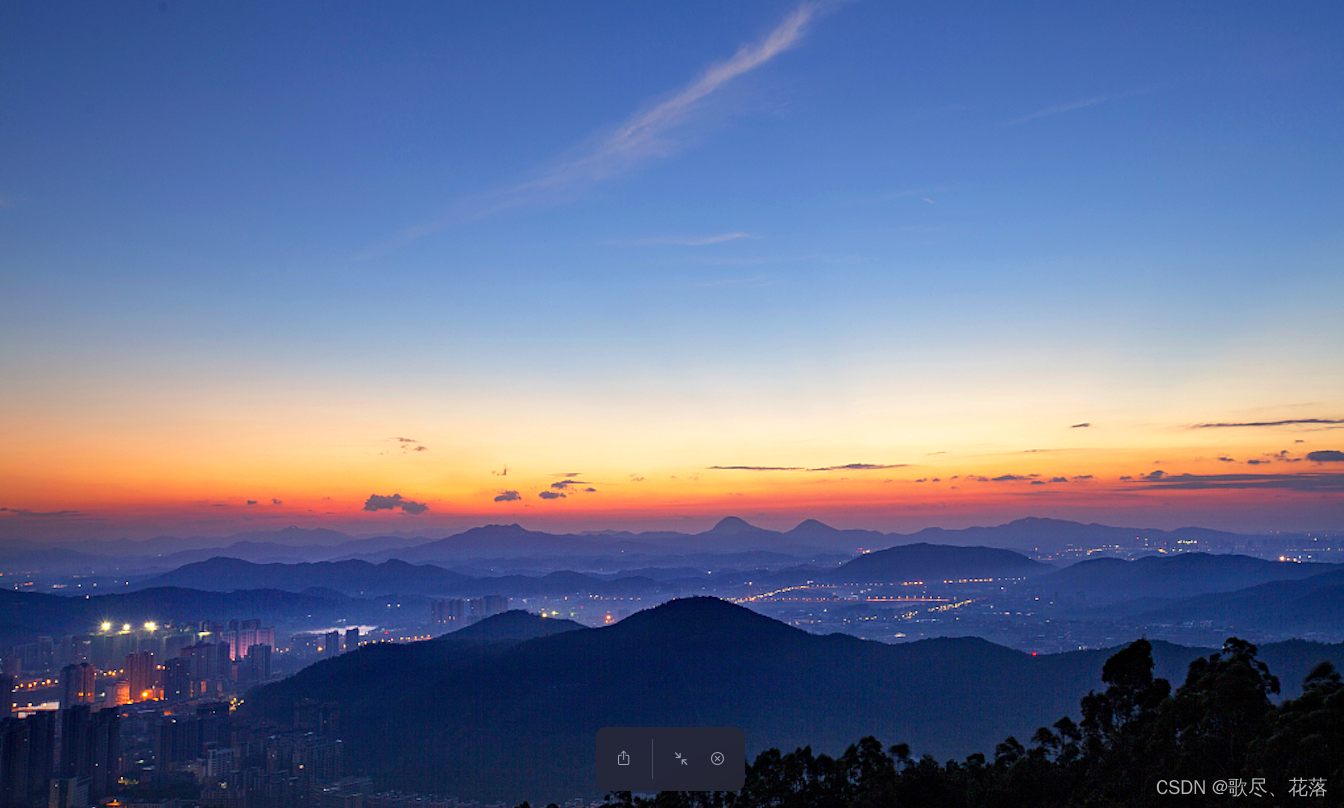
Vue动态组件
等疫情结束了,要开始爬爬山、看看海,做些不会后悔的事情一、概念1. 示例组件是可复用的 Vue 实例,且带有一个名字,这里实现一个最简单的组件:父组件:<template><div><h1>Father</h1><!-- 调用子组件 --><Son /></div></template><scri
·
等疫情结束了,要开始爬爬山、看看海,做些不会后悔的事情
一、概念
1. 示例
组件是可复用的 Vue 实例,且带有一个名字,这里实现一个最简单的组件:
父组件:
<template>
<div>
<h1>Father</h1>
<!-- 调用子组件 -->
<Son />
</div>
</template>
<script>
// 引入子组件模块
import Son from './son'
export default {
components: {
// 声明子组件
Son
},
}
</script>
子组件:
<template>
<h1>Son</h1>
</template>
2. 复用
组件可以被任意次复用,并且每个组件内部都可以独立维护,利用 slot 插槽
可以实现组件自由拓展
父组件:
<template>
<div class="fahter">
<Son></Son>
<i>------------------------------</i>
<Son>
<template v-slot:main>
<h1>Father-main</h1>
</template>
</Son>
<i>------------------------------</i>
<Son>
<template v-slot:footer><div /></template>
<h1>Father</h1>
</Son>
</div>
</template>
<script>
import Son from './son'
export default {
components: {
Son
},
}
</script>
子组件:
<template>
<div>
<slot><h1>header</h1></slot>
<slot name="main"><h1>main</h1></slot>
<slot name="footer"><h1>footer</h1></slot>
</div>
</template>
效果:
3. 组件传值
⑴. 父传子
父组件:
<template>
<div class="father">
<Son :toSonValue="fatherValue"></Son>
</div>
</template>
<script>
import Son from './son'
export default {
data() {
return {
fatherValue: 'value => father'
}
},
components: {
Son
},
}
</script>
子组件:
<template>
<h1>{{ this.toSonValue }}</h1>
</template>
<script>
export default {
props: {
toSonValue: {
type: String
}
},
data() {
return {
sonValue: 'value => son'
}
}
}
</script>
⑵. 子传父
子组件:
<template>
<div>
<h1>son</h1>
<button @click="sendValue">传值</button>
</div>
</template>
<script>
export default {
data() {
return {
sonValue: 'value => son'
}
},
methods: {
sendValue() {
this.$emit('father-methods', this.sonValue)
}
}
}
</script>
父组件:
<template>
<div class="father">
<Son @father-methods="click" />
<h1>{{ this.sonValue }}</h1>
</div>
</template>
<script>
import Son from './son'
export default {
data() {
return {
sonValue: ''
}
},
components: { Son },
methods: {
click(payload) {
console.log('get')
this.sonValue = payload
}
}
}
</script>
⑶. ref 传值(父传子)
父组件:
<template>
<div class="father">
<Son ref="son" />
<button @click="click">传值</button>
</div>
</template>
<script>
import Son from './son'
export default {
data() {
return {
fatherValue: 'value => father'
}
},
components: { Son },
methods: {
click() {
this.$refs.son.getFatherValue(this.fatherValue)
}
}
}
</script>
子组件:
<template>
<h1>{{this.fatherValue}}</h1>
</template>
<script>
export default {
data() {
return {
fatherValue: ''
}
},
methods: {
getFatherValue(payload) {
this.fatherValue = payload
}
}
}
</script>
⑷. parent 传值(子传父)
子组件:
<template>
<div>
<h1>son</h1>
<button @click="sendValue">传值</button>
</div>
</template>
<script>
export default {
data() {
return {
sonValue: 'value => son'
}
},
methods: {
sendValue() {
this.$parent.getSonValue(this.sonValue)
}
}
}
</script>
父组件:
<template>
<div>
<Son />
<h1>{{ this.sonValue }}</h1>
</div>
</template>
<script>
import Son from './son'
export default {
data() {
return {
sonValue: ''
}
},
components: { Son },
methods: {
getSonValue(payload) {
this.sonValue = payload
}
}
}
</script>
二、动态组件
1. is
- 一个标签页面切换不同组件
- 组件不会直接全部挂载,切换的时候才会挂载
<template>
<div>
<component :is="assembly"/>
<div>
<button @click="changeAssembly(1)">son1</button>
<button @click="changeAssembly(2)">son2</button>
<button @click="changeAssembly(3)">son3</button>
</div>
</div>
</template>
<script>
export default {
data() {
return {
assembly: ''
}
},
components: {
son1: () => import('./son1'),
son2: () => import('./son2'),
son3: () => import('./son3'),
},
methods: {
changeAssembly(value) {
if(value === 1) {
this.assembly = 'son1'
} else if(value === 2){
this.assembly = 'son2'
} else if(value === 3) {
this.assembly = 'son3'
} else {}
}
}
}
</script>
预览:
2. keep-alive
动态加载的组件,会导致 DOM 重新注册和销毁,从而重置用户表单、刷新了用户页面等等问题;所以希望那些标签的组件实例,能够被在它们第一次被创建的时候缓存下来。
<keep-alive>
<component :is="assembly" />
</keep-alive>
三、异步组件
太难了,学不动,有大佬了解可以发文章链接我学习一下
更多推荐
所有评论(0)