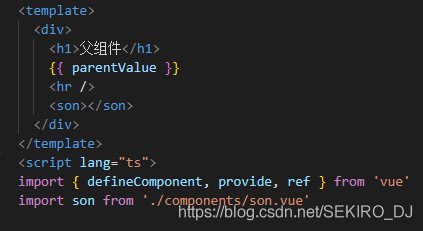
Vue3父子组件间传值
在vue3中,子组件接收父组件值的方式发生了变化,直接用props接收后是无法使用的。让我们先来看看vue2父子间的传值父传子<template><div><h1>父组件</h1><hr /><son :value="parentValue"></son></div></template>&
·
在vue3中,子组件使用父组件值的方式发生了变化,用props接收后在setup中是无法通过this.xxx调用的。
让我们先来看看vue2父子间的传值
父传子
<template>
<div>
<h1>父组件</h1>
<hr />
<son :value="parentValue"></son>
</div>
</template>
<script>
import son from './components/son.vue'
export default {
components: { son },
data () {
return {
parentValue: '父传给子的值'
}
}
}
</script>
子组件通过props接收
<template>
<div>
<p>子组件</p>
<div>{{ value }}</div>
</div>
</template>
<script>
export default {
props: ['value']
}
</script>
子传父
<template>
<div>
<h1>父组件</h1>
<div>{{ parentValue }}</div>
<hr />
<son @handle="handel"></son>
</div>
</template>
<script>
import son from './components/son.vue'
export default {
components: { son },
data () {
return {
parentValue: '父传给子的值'
}
},
methods: {
handel (value) {
this.parentValue = value
}
}
}
</script>
子组件通过$emit触发父组件中的事件传值给父组件
<template>
<div>
<p>子组件</p>
<button @click="fn">点击传值给父组件</button>
</div>
</template>
<script>
export default {
methods: {
fn () {
this.$emit('handle', '子传给父的值')
}
}
}
</script>
接下来看看vue3的传值
父传子
<template>
<div>
<h1>父组件</h1>
<hr />
<son :value="parentValue"></son>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue'
import son from './components/son.vue'
export default defineComponent({
components: { son },
setup () {
const parentValue = ref('父组件传给子组件的值')
return { parentValue }
}
})
</script>
由于vue3的setup中没有this,所以我们想要在setup中使用父组件传来的值,要借助setup函数的第一个参数
<template>
<div>
<p>子组件</p>
<div>{{ value }}</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue'
export default defineComponent({
props: ['value'],
setup (props, context) {
console.log(props.value) // 父组件传给子组件的值
}
})
</script>
子传父
<template>
<div>
<h1>父组件</h1>
{{ parentValue }}
<hr />
<son @handle="handle"></son>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue'
import son from './components/son.vue'
export default defineComponent({
components: { son },
setup () {
let parentValue = ref('父组件传给子组件的值')
const handle = value => {
parentValue.value = value
}
return { parentValue, handle }
}
})
</script>
子通过emit触发父组件中的方法传值
<template>
<div>
<p>子组件</p>
<button @click="fn">点击改变父组件的值</button>
</div>
</template>
<script lang="ts">
import { defineComponent, ref } from 'vue'
export default defineComponent({
setup (props, context) {
const fn = () => {
context.emit('handle', '子组件传给父组件的值')
}
return { fn }
}
})
</script>
provide和inject
vue3还给我们提供了provide和inject,我们可以在父组件中通过provide暴露属性或方法,子组件通过inject接受,并且只要是父组件的后代,都可以通过inject接收到父组件暴露的值
<template>
<div>
<h1>父组件</h1>
{{ parentValue }}
<hr />
<son></son>
</div>
</template>
<script lang="ts">
import { defineComponent, provide, ref } from 'vue'
import son from './components/son.vue'
export default defineComponent({
components: { son },
setup () {
// 传值
let parentValue = ref('父中的值')
provide('parentValue', parentValue)
// 传方法
const handle = (sonValue: any) => {
parentValue.value = sonValue
}
provide('handle', handle)
return { parentValue }
}
})
</script>
<template>
<div>
<p>子组件</p>
<div>{{ value }}</div>
<button @click="fn">点击修改父组件的值</button>
</div>
</template>
<script lang="ts">
import { defineComponent, inject } from 'vue'
export default defineComponent({
setup () {
const value = inject('parentValue') // 使用父组件传来的值
const handle: any = inject('handle')
// 修改父组件的值
const fn = () => {
handle('修改父组件的值')
}
return { value, fn }
}
})
</script>
更多推荐
所有评论(0)