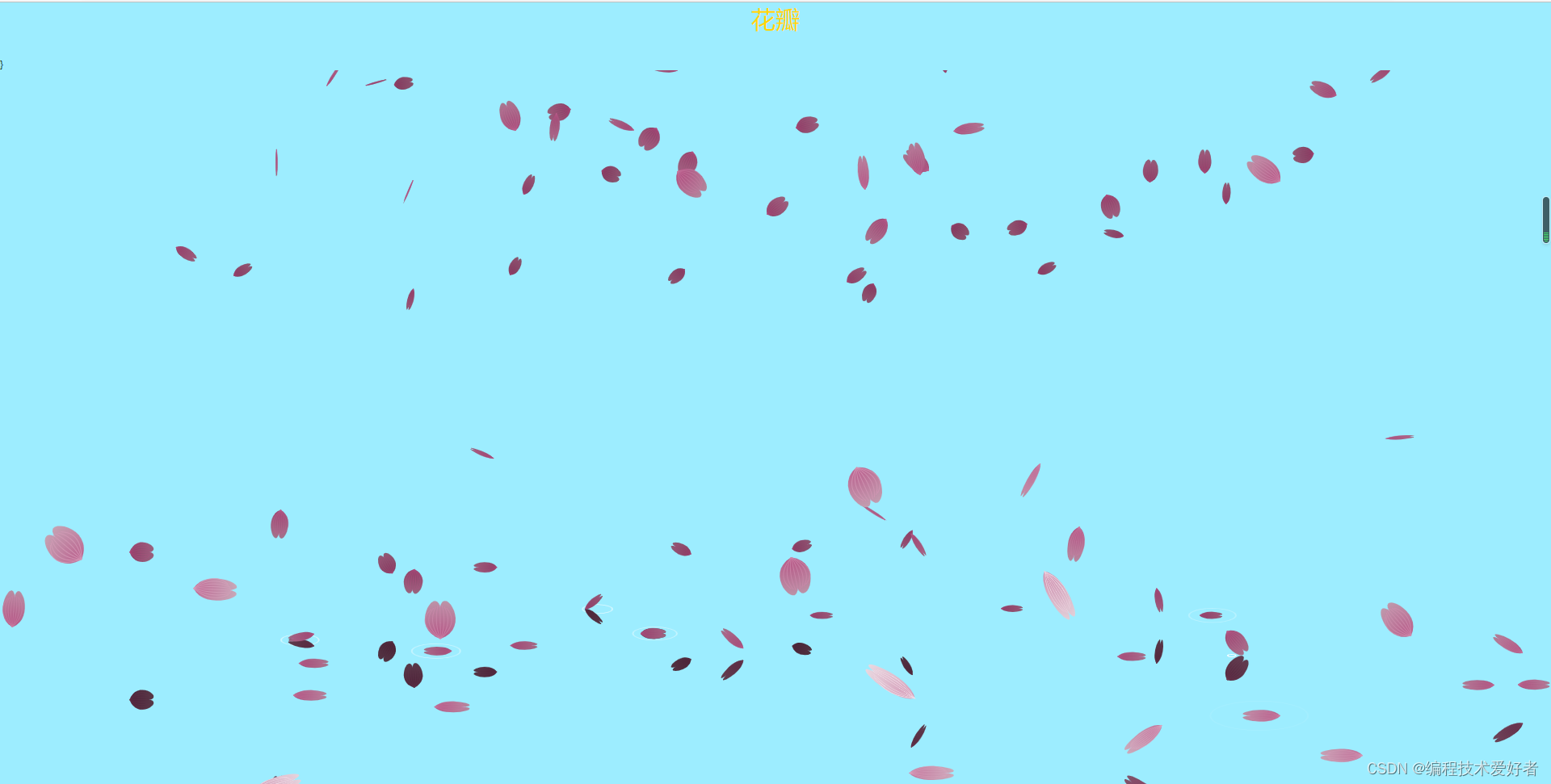
HTML源码大放送1
HTML源码大放送1
·
1.代码雨
<!DOCTYPE html>
<html>
<head>
<title>代码雨</title>
</head>
<body>
<canvas id="canvas"></canvas>
<style type="text/css">
body{margin: 0; padding: 0; overflow: hidden;}
</style>
<script type="text/javascript">
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');
canvas.height = window.innerHeight;
canvas.width = window.innerWidth;
var texts = '0123456789'.split('');
var fontSize = 16;
var columns = canvas.width/fontSize;
// 用于计算输出文字时坐标,所以长度即为列数
var drops = [];
//初始值
for(var x = 0; x < columns; x++){
drops[x] = 1;
}
function draw(){
//让背景逐渐由透明到不透明
ctx.fillStyle = 'rgba(0, 0, 0, 0.05)';
ctx.fillRect(0, 0, canvas.width, canvas.height);
//文字颜色
ctx.fillStyle = '#0F0';
ctx.font = fontSize + 'px arial';
//逐行输出文字
for(var i = 0; i < drops.length; i++){
var text = texts[Math.floor(Math.random()*texts.length)];
ctx.fillText(text, i*fontSize, drops[i]*fontSize);
if(drops[i]*fontSize > canvas.height || Math.random() > 0.95){
drops[i] = 0;
}
drops[i]++;
}
}
setInterval(draw, 33);
</script>
</body>
2.俄罗斯方块
<!doctype html><html><head></head><body>
<div id="box" style="width:252px;font:25px/25px 宋体;background:#000;color:#9f9;border:#999 20px ridge;text-shadow:2px 3px 1px #0f0;"></div>
<script>
var map=eval("["+Array(23).join("0x801,")+"0xfff]");
var tatris=[[0x6600],[0x2222,0xf00],[0xc600,0x2640],[0x6c00,0x4620],[0x4460,0x2e0,0x6220,0x740],[0x2260,0xe20,0x6440,0x4700],[0x2620,0x720,0x2320,0x2700]];
var keycom={"38":"rotate(1)","40":"down()","37":"move(2,1)","39":"move(0.5,-1)"};
var dia, pos, bak, run;
function start(){
dia=tatris[~~(Math.random()*7)];
bak=pos={fk:[],y:0,x:4,s:~~(Math.random()*4)};
rotate(0);
}
function over(){
document.onkeydown=null;
clearInterval(run);
alert("GAME OVER");
}
function update(t){
bak={fk:pos.fk.slice(0),y:pos.y,x:pos.x,s:pos.s};
if(t) return;
for(var i=0,a2=""; i<22; i++)
a2+=map[i].toString(2).slice(1,-1)+"<br/>";
for(var i=0,n; i<4; i++)
if(/([^0]+)/.test(bak.fk[i].toString(2).replace(/1/g,"\u25a1")))
a2=a2.substr(0,n=(bak.y+i+1)*15-RegExp.$_.length-4)+RegExp.$1+a2.slice(n+RegExp.$1.length);
document.getElementById("box").innerHTML=a2.replace(/1/g,"\u25a0").replace(/0/g,"\u3000");
29
}
function is(){
for(var i=0; i<4; i++)
if((pos.fk[i]&map[pos.y+i])!=0) return pos=bak;
}
function rotate(r){
var f=dia[pos.s=(pos.s+r)%dia.length];
for(var i=0; i<4; i++)
pos.fk[i]=(f>>(12-i*4)&15)<<pos.x;
update(is());
}
function down(){
++pos.y;
if(is()){
for(var i=0; i<4 && pos.y+i<22; i++)
if((map[pos.y+i]|=pos.fk[i])==0xfff)
map.splice(pos.y+i,1), map.unshift(0x801);
if(map[1]!=0x801) return over();
start();
}
update();
}
function move(t,k){
pos.x+=k;
for(var i=0; i<4; i++)
pos.fk[i]*=t;
update(is());
}
document.onkeydown=function(e){
eval(keycom[(e?e:event).keyCode]);
};
start();
run=setInterval("down()",400);
62
</script></body></html>
3.光点贪吃蛇
<html>
<body>
<canvas id="can" width="400" height="400" style="background:Black"></canvas>
<script>
var sn=[42,41],dz=43,fx=1,n,ctx=document.getElementById("can").getContext("2d");
function draw(t,c){
ctx.fillStyle=c;
ctx.fillRect(t%20*20+1,~~(t/20)*20+1,18,18);
}
document.onkeydown=function(e){fx=sn[1]-sn[0]==(n=[-1,-20,1,20][(e||event).keyCode-37]||fx)?fx:n};
!function(){
sn.unshift(n=sn[0]+fx);
if(sn.indexOf(n,1)>0 || n<0||n>399||fx==1&&n%20==0||fx==-1&&n%20==19){
return alert("GAME OVER");
refesh();
}
draw(n,"Lime");
if(n==dz){
while(sn.indexOf(dz=~~(Math.random()*400))>=0);
draw(dz,"Yellow");
}else{
draw(sn.pop(),"Black");
}
setTimeout(arguments.callee,300);数值大速度慢
}();
function refesh(){
location.reload();
}
</script>
</body>
</html>
4.泡泡
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title></title>
<style>
*{
margin:0;padding:0;
}
body{overflow:hidden;}
#canvas{
background-color:black;
/*width:100%;
height:100vh;*/
}
</style>
</head>
<body>
<canvas id="canvas" ></canvas>
</body>
<script>
var canvas = document.querySelector('#canvas');
var ctx = canvas.getContext("2d");
var starlist = [];
function init(){
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
}
init();
window.onresize = init;
canvas.addEventListener('mousemove',function(e){
starlist.push(new Star(e.offsetX,e.offsetY));
console.log(starlist)
})
function random(min,max){
return Math.floor((max-min)*Math.random()+ min);
}
function Star(x,y){
this.x = x;
this.y = y;
this.vx = (Math.random()-0.5)*2.5;
this.vy = (Math.random()-0.5)*2.5;
this.color = 'rgb('+random(0,200)+','+random(0,200)+','+random(0,200)+')';
this.a = 1;
console.log(this.color);
this.draw();
}
Star.prototype={
draw:function(){
ctx.beginPath();
ctx.fillStyle = this.color;
ctx.globalCompositeOperation='lighter'
ctx.globalAlpha= this.a;
ctx.arc(this.x,this.y,30,0,Math.PI*2,false);
ctx.fill();
this.updata();
},
updata(){
this.x+=this.vx;
this.y+=this.vy;
this.a*=.98;
}
}
console.log(new Star(150,200));
function render(){
ctx.clearRect(0,0,canvas.width,canvas.height)
starlist.forEach((item,i)=>{
item.draw();
if(item.a<0.05){
starlist.splice(i,1);
}
})
requestAnimationFrame(render);
}
render();
</script>
<div style="text-align:center;">
</div>
</html>
5.网格贪吃蛇
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>JS贪吃蛇</title>
<style type="text/css">
#pannel table {
border-collapse: collapse;
}
#pannel table td {
border: 1px solid #808080;
width: 10px;
height: 10px;
font-size: 0;
line-height: 0;
overflow: hidden;
}
#pannel table .snake {
background-color: green;
}
#pannel table .food {
background-color: blue;
}
</style>
<script type="text/javascript">
var Direction = new function () {
this.UP = 38;
this.RIGHT = 39;
this.DOWN = 40;
this.LEFT = 37;
};
var Common = new function () {
this.width = 20; /*水平方向方格数*/
this.height = 20; /*垂直方向方格数*/
this.speed = 250; /*速度 值越小越快*/
this.workThread = null;
};
var Main = new function () {
var control = new Control();
window.onload = function () {
control.Init("pannel");
/*开始按钮*/
document.getElementById("btnStart").onclick = function () {
control.Start();
this.disabled = true;
document.getElementById("selSpeed").disabled = true;
document.getElementById("selSize").disabled = true;
};
/*调速度按钮*/
document.getElementById("selSpeed").onchange = function () {
Common.speed = this.value;
}
/*调大小按钮*/
document.getElementById("selSize").onchange = function () {
Common.width = this.value;
Common.height = this.value;
control.Init("pannel");
}
};
};
/*控制器*/
function Control() {
this.snake = new Snake();
this.food = new Food();
/*初始化函数,创建表格*/
this.Init = function (pid) {
var html = [];
html.push("<table>");
for (var y = 0; y < Common.height; y++) {
html.push("<tr>");
for (var x = 0; x < Common.width; x++) {
html.push('<td id="box_' + x + "_" + y + '"> </td>');
}
html.push("</tr>");
}
html.push("</table>");
this.pannel = document.getElementById(pid);
this.pannel.innerHTML = html.join("");
};
/*开始游戏 - 监听键盘、创建食物、刷新界面线程*/
this.Start = function () {
var me = this;
this.MoveSnake = function (ev) {
var evt = window.event || ev;
me.snake.SetDir(evt.keyCode);
};
try {
document.attachEvent("onkeydown", this.MoveSnake);
} catch (e) {
document.addEventListener("keydown", this.MoveSnake, false);
}
this.food.Create();
Common.workThread = setInterval(function () {
me.snake.Eat(me.food); me.snake.Move();
}, Common.speed);
};
}
/*蛇*/
function Snake() {
this.isDone = false;
this.dir = Direction.RIGHT;
this.pos = new Array(new Position());
/*移动 - 擦除尾部,向前移动,判断游戏结束(咬到自己或者移出边界)*/
this.Move = function () {
document.getElementById("box_" + this.pos[0].X + "_" + this.pos[0].Y).className = "";
//所有 向前移动一步
for (var i = 0; i < this.pos.length - 1; i++) {
this.pos[i].X = this.pos[i + 1].X;
this.pos[i].Y = this.pos[i + 1].Y;
}
//重新设置头的位置
var head = this.pos[this.pos.length - 1];
switch (this.dir) {
case Direction.UP:
head.Y--;
break;
case Direction.RIGHT:
head.X++;
break;
case Direction.DOWN:
head.Y++;
break;
case Direction.LEFT:
head.X--;
break;
}
this.pos[this.pos.length - 1] = head;
//遍历画蛇,同时判断游戏结束
for (var i = 0; i < this.pos.length; i++) {
var isExits = false;
for (var j = i + 1; j < this.pos.length; j++)
if (this.pos[j].X == this.pos[i].X && this.pos[j].Y == this.pos[i].Y) {
isExits = true;
break;
}
if (isExits) {
this.Over();
/*咬自己*/
break;
}
var obj = document.getElementById("box_" + this.pos[i].X + "_" + this.pos[i].Y);
if (obj) obj.className = "snake";
else {
this.Over();
/*移出边界*/
break;
}
}
this.isDone = true;
};
/*游戏结束*/
this.Over = function () {
clearInterval(Common.workThread);
alert("游戏结束!");
location.reload();
}
/*吃食物*/
this.Eat = function (food) {
var head = this.pos[this.pos.length - 1];
var isEat = false;
switch (this.dir) {
case Direction.UP:
if (head.X == food.pos.X && head.Y == food.pos.Y + 1) isEat = true;
break;
case Direction.RIGHT:
if (head.Y == food.pos.Y && head.X == food.pos.X - 1) isEat = true;
break;
case Direction.DOWN:
if (head.X == food.pos.X && head.Y == food.pos.Y - 1) isEat = true;
break;
case Direction.LEFT:
if (head.Y == food.pos.Y && head.X == food.pos.X + 1) isEat = true;
break;
}
if (isEat) {
this.pos[this.pos.length] = new Position(food.pos.X, food.pos.Y);
food.Create(this.pos);
}
};
/*控制移动方向*/
this.SetDir = function (dir) {
switch (dir) {
case Direction.UP:
if (this.isDone && this.dir != Direction.DOWN) { this.dir = dir; this.isDone = false; }
break;
case Direction.RIGHT:
if (this.isDone && this.dir != Direction.LEFT) { this.dir = dir; this.isDone = false; }
break;
case Direction.DOWN:
if (this.isDone && this.dir != Direction.UP) { this.dir = dir; this.isDone = false; }
break;
case Direction.LEFT:
if (this.isDone && this.dir != Direction.RIGHT) { this.dir = dir; this.isDone = false; }
break;
}
};
}
/*食物*/
function Food() {
this.pos = new Position();
/*创建食物 - 随机位置创建立*/
this.Create = function (pos) {
document.getElementById("box_" + this.pos.X + "_" + this.pos.Y).className = "";
var x = 0, y = 0, isCover = false;
/*排除蛇的位置*/
do {
x = parseInt(Math.random() * (Common.width - 1));
y = parseInt(Math.random() * (Common.height - 1));
isCover = false;
if (pos instanceof Array) {
for (var i = 0; i < pos.length; i++) {
if (x == pos[i].X && y == pos[i].Y) {
isCover = true;
break;
}
}
}
} while (isCover);
this.pos = new Position(x, y);
document.getElementById("box_" + x + "_" + y).className = "food";
};
}
function Position(x, y) {
this.X = 0;
this.Y = 0;
if (arguments.length >= 1) this.X = x;
if (arguments.length >= 2) this.Y = y;
}
</script>
</head>
<body>
<div id="pannel" style="margin-bottom: 10px;"></div>
<select id="selSize">
<option value="20">20*20</option>
<option value="30">30*30</option>
<option value="40">40*40</option>
</select>
<select id="selSpeed">
<option value="500">速度-慢</option>
<option value="250" selected="selected">速度-中</option>
<option value="100">速度-快</option>
</select>
<input type="button" id="btnStart" value="开始" />
</body>
</html> -->
6.3D花瓣
这里分为两部分js+html
js部分
(function(){
var lastTime=0;
var vendors=['webkit','moz'];
for(var x=0;
x<vendors.length&&!window.requestAnimationFrame;++x)
{window.requestAnimationFrame=window[vendors[x]+'RequestAnimationFrame'];
window.cancelAnimationFrame=window[vendors[x]+'CancelAnimationFrame']||window[vendors[x]+'CancelRequestAnimationFrame'];
}
if(!window.requestAnimationFrame)window.requestAnimationFrame=function(callback,element){var currTime=new Date().getTime();
var timeToCall=Math.max(0,16-(currTime-lastTime));
var id=window.setTimeout(function(){callback(currTime+timeToCall);
},timeToCall);
lastTime=currTime+timeToCall;
return id;
};
if(!window.cancelAnimationFrame)window.cancelAnimationFrame=function(id){clearTimeout(id);
};
}());
(function($){$.snowfall=function(element,options)
{var defaults=
{flakeCount:35,
flakeColor:'#ffffff',
flakePosition:'absolute',
flakeIndex:999999,
minSize:1,maxSize:2,minSpeed:1,
maxSpeed:5,round:false,
shadow:false,collection:false,
collectionHeight:40,
deviceorientation:false},
options=$.extend(defaults,options),
random=function random(min,max){
return Math.round(min+Math.random()*
(max-min));
};
$(element).data("snowfall",this);
function Flake(_x,_y,_size,_speed,_id)
{this.id=_id;this.x=_x;this.y=_y;this.s
ize=_size;this.speed=_speed;this.step=0
;this.stepSize=random(1,10)/100;if(opti
ons.collection)
{this.target=canvasCollection[random(0,
canvasCollection.length-1)];}
var flakeMarkup=null;
if(options.image)
{flakeMarkup=$(document.createElement("img"));flakeMarkup[0].src=options.image;}else
{flakeMarkup=$(document.createElement("div"));flakeMarkup.css({'background':options.flakeColor});}
flakeMarkup.attr({'class':'snowfall-flakes','id':'flake-'+this.id}).css({'width':this.size,'height':this.size,'position':options.flakePosition,'top':this.y,'left':this.x,'fontSize':0,'zIndex':options.flakeIndex});if($(element).get(0).tagName===$(document).get(0).tagName){$('body').append(flakeMarkup);element=$('body');}else{$(element).append(flakeMarkup);}this.element=document.getElementById('flake-'+this.id);this.update=function(){this.y+=this.speed;if(this.y>(elHeight)-(this.size+6)){this.reset();}this.element.style.top=this.y+'px';this.element.style.left=this.x+'px';this.step+=this.stepSize;if(doRatio===false){this.x+=Math.cos(this.step);}else{this.x+=(doRatio+Math.cos(this.step));}if(options.collection){if(this.x>this.target.x&&this.x<this.target.width+this.target.x&&this.y>this.target.y&&this.y<this.target.height+this.target.y){var ctx=this.target.element.getContext("2d"),curX=this.x-this.target.x,curY=this.y-this.target.y,colData=this.target.colData;if(colData[parseInt(curX)][parseInt(curY+this.speed+this.size)]!==undefined||curY+this.speed+this.size>this.target.height){if(curY+this.speed+this.size>this.target.height){while(curY+this.speed+this.size>this.target.height&&this.speed>0){this.speed*=.5;}ctx.fillStyle="#fff";if(colData[parseInt(curX)][parseInt(curY+this.speed+this.size)]==undefined){colData[parseInt(curX)][parseInt(curY+this.speed+this.size)]=1;ctx.fillRect(curX,(curY)+this.speed+this.size,this.size,this.size);}else{colData[parseInt(curX)][parseInt(curY+this.speed)]=1;ctx.fillRect(curX,curY+this.speed,this.size,this.size);}this.reset();}else{this.speed=1;this.stepSize=0;if(parseInt(curX)+1<this.target.width&&colData[parseInt(curX)+1][parseInt(curY)+1]==undefined){this.x++;}else if(parseInt(curX)-1>0&&colData[parseInt(curX)-1][parseInt(curY)+1]==undefined){this.x--;}else{ctx.fillStyle="#fff";ctx.fillRect(curX,curY,this.size,this.size);colData[parseInt(curX)][parseInt(curY)]=1;this.reset();}}}}}if(this.x>(elWidth)-widthOffset||this.x<widthOffset){this.reset();}}this.reset=function(){this.y=0;this.x=random(widthOffset,elWidth-widthOffset);this.stepSize=random(1,10)/100;this.size=random((options.minSize*100),(options.maxSize*100))/100;this.speed=random(options.minSpeed,options.maxSpeed);}}var flakes=[],flakeId=0,i=0,elHeight=$(element).height(),elWidth=$(element).width(),widthOffset=0,snowTimeout=0;if(options.collection!==false){var testElem=document.createElement('canvas');if(!!(testElem.getContext&&testElem.getContext('2d'))){var canvasCollection=[],elements=$(options.collection),collectionHeight=options.collectionHeight;for(var i=0;i<elements.length;i++){var bounds=elements[i].getBoundingClientRect(),canvas=document.createElement('canvas'),collisionData=[];if(bounds.top-collectionHeight>0){document.body.appendChild(canvas);canvas.style.position=options.flakePosition;canvas.height=collectionHeight;canvas.width=bounds.width;canvas.style.left=bounds.left+'px';canvas.style.top=bounds.top-collectionHeight+'px';for(var w=0;w<bounds.width;w++){collisionData[w]=[];}canvasCollection.push({element:canvas,x:bounds.left,y:bounds.top-collectionHeight,width:bounds.width,height:collectionHeight,colData:collisionData});}}}else{options.collection=false;}}if($(element).get(0).tagName===$(document).get(0).tagName){widthOffset=25;}$(window).bind("resize",function(){elHeight=$(element)[0].clientHeight;elWidth=$(element)[0].offsetWidth;});for(i=0;i<options.flakeCount;i+=1){flakeId=flakes.length;flakes.push(new Flake(random(widthOffset,elWidth-widthOffset),random(0,elHeight),random((options.minSize*100),(options.maxSize*100))/100,random(options.minSpeed,options.maxSpeed),flakeId));}if(options.round){$('.snowfall-flakes').css({'-moz-border-radius':options.maxSize,'-webkit-border-radius':options.maxSize,'border-radius':options.maxSize});}if(options.shadow){$('.snowfall-flakes').css({'-moz-box-shadow':'1px 1px 1px #555','-webkit-box-shadow':'1px 1px 1px #555','box-shadow':'1px 1px 1px #555'});}var doRatio=false;if(options.deviceorientation){$(window).bind('deviceorientation',function(event){doRatio=event.originalEvent.gamma*0.1;});}function snow(){for(i=0;i<flakes.length;i+=1){flakes[i].update();}snowTimeout=requestAnimationFrame(function(){snow()});}snow();this.clear=function(){$(element).children('.snowfall-flakes').remove();flakes=[];cancelAnimationFrame(snowTimeout);}};$.fn.snowfall=function(options){if(typeof(options)=="object"||options==undefined){return this.each(function(i){(new $.snowfall(this,options));});}else if(typeof(options)=="string"){return this.each(function(i){var snow=$(this).data('snowfall');if(snow){snow.clear();}});}};})(jQuery);
html部分
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN">
<HTML>
<HEAD>
<TITLE>花瓣</TITLE>
<META NAME="Generator" CONTENT="EditPlus">
<META NAME="Author" CONTENT="">
<META NAME="Keywords" CONTENT="">
<META NAME="Description" CONTENT="">
<style>
html, body{
width: 100%;
height: 100%;
margin: 0;
padding: 0;
overflow: hidden;
}
.container{
width: 100%;
height: 100%;
margin: 0;
padding: 0;
background-color: #9dedff;
}
</style>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</script>
<style type="text/css">
.aa
{
font: "微软雅黑";
font-size: 30px;
color: gold;
text-align: center;
}
</style>
</head>
<body style="background:#9dedff;font:9px Arial">
<p class="aa">花瓣</p>
<audio src="audio/"></audio>
}
</HEAD>
<BODY>
<div id="jsi-cherry-container" class="container"></div>
<script>
var RENDERER = {
INIT_CHERRY_BLOSSOM_COUNT : 30,
MAX_ADDING_INTERVAL : 10,
init : function(){
this.setParameters();
this.reconstructMethods();
this.createCherries();
this.render();
},
setParameters : function(){
this.$container = $('#jsi-cherry-container');
this.width = this.$container.width();
this.height = this.$container.height();
this.context = $('<canvas />').attr({width : this.width, height : this.height}).appendTo(this.$container).get(0).getContext('2d');
this.cherries = [];
this.maxAddingInterval = Math.round(this.MAX_ADDING_INTERVAL * 1000 / this.width);
this.addingInterval = this.maxAddingInterval;
},
reconstructMethods : function(){
this.render = this.render.bind(this);
},
createCherries : function(){
for(var i = 0, length = Math.round(this.INIT_CHERRY_BLOSSOM_COUNT * this.width / 1000); i < length; i++){
this.cherries.push(new CHERRY_BLOSSOM(this, true));
}
},
render : function(){
requestAnimationFrame(this.render);
this.context.clearRect(0, 0, this.width, this.height);
this.cherries.sort(function(cherry1, cherry2){
return cherry1.z - cherry2.z;
});
for(var i = this.cherries.length - 1; i >= 0; i--){
if(!this.cherries[i].render(this.context)){
this.cherries.splice(i, 1);
}
}
if(--this.addingInterval == 0){
this.addingInterval = this.maxAddingInterval;
this.cherries.push(new CHERRY_BLOSSOM(this, false));
}
}
};
var CHERRY_BLOSSOM = function(renderer, isRandom){
this.renderer = renderer;
this.init(isRandom);
};
CHERRY_BLOSSOM.prototype = {
FOCUS_POSITION : 300,
FAR_LIMIT : 600,
MAX_RIPPLE_COUNT : 100,
RIPPLE_RADIUS : 100,
SURFACE_RATE : 0.5,
SINK_OFFSET : 20,
init : function(isRandom){
this.x = this.getRandomValue(-this.renderer.width, this.renderer.width);
this.y = isRandom ? this.getRandomValue(0, this.renderer.height) : this.renderer.height * 1.5;
this.z = this.getRandomValue(0, this.FAR_LIMIT);
this.vx = this.getRandomValue(-2, 2);
this.vy = -2;
this.theta = this.getRandomValue(0, Math.PI * 2);
this.phi = this.getRandomValue(0, Math.PI * 2);
this.psi = 0;
this.dpsi = this.getRandomValue(Math.PI / 600, Math.PI / 300);
this.opacity = 0;
this.endTheta = false;
this.endPhi = false;
this.rippleCount = 0;
var axis = this.getAxis(),
theta = this.theta + Math.ceil(-(this.y + this.renderer.height * this.SURFACE_RATE) / this.vy) * Math.PI / 500;
theta %= Math.PI * 2;
this.offsetY = 40 * ((theta <= Math.PI / 2 || theta >= Math.PI * 3 / 2) ? -1 : 1);
this.thresholdY = this.renderer.height / 2 + this.renderer.height * this.SURFACE_RATE * axis.rate;
this.entityColor = this.renderer.context.createRadialGradient(0, 40, 0, 0, 40, 80);
this.entityColor.addColorStop(0, 'hsl(330, 70%, ' + 50 * (0.3 + axis.rate) + '%)');
this.entityColor.addColorStop(0.05, 'hsl(330, 40%,' + 55 * (0.3 + axis.rate) + '%)');
this.entityColor.addColorStop(1, 'hsl(330, 20%, ' + 70 * (0.3 + axis.rate) + '%)');
this.shadowColor = this.renderer.context.createRadialGradient(0, 40, 0, 0, 40, 80);
this.shadowColor.addColorStop(0, 'hsl(330, 40%, ' + 30 * (0.3 + axis.rate) + '%)');
this.shadowColor.addColorStop(0.05, 'hsl(330, 40%,' + 30 * (0.3 + axis.rate) + '%)');
this.shadowColor.addColorStop(1, 'hsl(330, 20%, ' + 40 * (0.3 + axis.rate) + '%)');
},
getRandomValue : function(min, max){
return min + (max - min) * Math.random();
},
getAxis : function(){
var rate = this.FOCUS_POSITION / (this.z + this.FOCUS_POSITION),
x = this.renderer.width / 2 + this.x * rate,
y = this.renderer.height / 2 - this.y * rate;
return {rate : rate, x : x, y : y};
},
renderCherry : function(context, axis){
context.beginPath();
context.moveTo(0, 40);
context.bezierCurveTo(-60, 20, -10, -60, 0, -20);
context.bezierCurveTo(10, -60, 60, 20, 0, 40);
context.fill();
for(var i = -4; i < 4; i++){
context.beginPath();
context.moveTo(0, 40);
context.quadraticCurveTo(i * 12, 10, i * 4, -24 + Math.abs(i) * 2);
context.stroke();
}
},
render : function(context){
var axis = this.getAxis();
if(axis.y == this.thresholdY && this.rippleCount < this.MAX_RIPPLE_COUNT){
context.save();
context.lineWidth = 2;
context.strokeStyle = 'hsla(0, 0%, 100%, ' + (this.MAX_RIPPLE_COUNT - this.rippleCount) / this.MAX_RIPPLE_COUNT + ')';
context.translate(axis.x + this.offsetY * axis.rate * (this.theta <= Math.PI ? -1 : 1), axis.y);
context.scale(1, 0.3);
context.beginPath();
context.arc(0, 0, this.rippleCount / this.MAX_RIPPLE_COUNT * this.RIPPLE_RADIUS * axis.rate, 0, Math.PI * 2, false);
context.stroke();
context.restore();
this.rippleCount++;
}
if(axis.y < this.thresholdY || (!this.endTheta || !this.endPhi)){
if(this.y <= 0){
this.opacity = Math.min(this.opacity + 0.01, 1);
}
context.save();
context.globalAlpha = this.opacity;
context.fillStyle = this.shadowColor;
context.strokeStyle = 'hsl(330, 30%,' + 40 * (0.3 + axis.rate) + '%)';
context.translate(axis.x, Math.max(axis.y, this.thresholdY + this.thresholdY - axis.y));
context.rotate(Math.PI - this.theta);
context.scale(axis.rate * -Math.sin(this.phi), axis.rate);
context.translate(0, this.offsetY);
this.renderCherry(context, axis);
context.restore();
}
context.save();
context.fillStyle = this.entityColor;
context.strokeStyle = 'hsl(330, 40%,' + 70 * (0.3 + axis.rate) + '%)';
context.translate(axis.x, axis.y + Math.abs(this.SINK_OFFSET * Math.sin(this.psi) * axis.rate));
context.rotate(this.theta);
context.scale(axis.rate * Math.sin(this.phi), axis.rate);
context.translate(0, this.offsetY);
this.renderCherry(context, axis);
context.restore();
if(this.y <= -this.renderer.height / 4){
if(!this.endTheta){
for(var theta = Math.PI / 2, end = Math.PI * 3 / 2; theta <= end; theta += Math.PI){
if(this.theta < theta && this.theta + Math.PI / 200 > theta){
this.theta = theta;
this.endTheta = true;
break;
}
}
}
if(!this.endPhi){
for(var phi = Math.PI / 8, end = Math.PI * 7 / 8; phi <= end; phi += Math.PI * 3 / 4){
if(this.phi < phi && this.phi + Math.PI / 200 > phi){
this.phi = Math.PI / 8;
this.endPhi = true;
break;
}
}
}
}
if(!this.endTheta){
if(axis.y == this.thresholdY){
this.theta += Math.PI / 200 * ((this.theta < Math.PI / 2 || (this.theta >= Math.PI && this.theta < Math.PI * 3 / 2)) ? 1 : -1);
}else{
this.theta += Math.PI / 500;
}
this.theta %= Math.PI * 2;
}
if(this.endPhi){
if(this.rippleCount == this.MAX_RIPPLE_COUNT){
this.psi += this.dpsi;
this.psi %= Math.PI * 2;
}
}else{
this.phi += Math.PI / ((axis.y == this.thresholdY) ? 200 : 500);
this.phi %= Math.PI;
}
if(this.y <= -this.renderer.height * this.SURFACE_RATE){
this.x += 2;
this.y = -this.renderer.height * this.SURFACE_RATE;
}else{
this.x += this.vx;
this.y += this.vy;
}
return this.z > -this.FOCUS_POSITION && this.z < this.FAR_LIMIT && this.x < this.renderer.width * 1.5;
}
};
$(function(){
RENDERER.init();
});
</script>
</BODY>
</HTML>
放在同一文件夹中即可
7.粒子特效
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>粒子漩涡特效</title>
<style>
html,body{
margin:0px;
width:100%;
height:100%;
overflow:hidden;
background:#000;
}
#canvas{
position:absolute;
width:100%;
height:100%;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
function project3D(x,y,z,vars){
var p,d;
x-=vars.camX;
y-=vars.camY-8;
z-=vars.camZ;
p=Math.atan2(x,z);
d=Math.sqrt(x*x+z*z);
x=Math.sin(p-vars.yaw)*d;
z=Math.cos(p-vars.yaw)*d;
p=Math.atan2(y,z);
d=Math.sqrt(y*y+z*z);
y=Math.sin(p-vars.pitch)*d;
z=Math.cos(p-vars.pitch)*d;
var rx1=-1000;
var ry1=1;
var rx2=1000;
var ry2=1;
var rx3=0;
var ry3=0;
var rx4=x;
var ry4=z;
var uc=(ry4-ry3)*(rx2-rx1)-(rx4-rx3)*(ry2-ry1);
var ua=((rx4-rx3)*(ry1-ry3)-(ry4-ry3)*(rx1-rx3))/uc;
var ub=((rx2-rx1)*(ry1-ry3)-(ry2-ry1)*(rx1-rx3))/uc;
if(!z)z=0.000000001;
if(ua>0&&ua<1&&ub>0&&ub<1){
return {
x:vars.cx+(rx1+ua*(rx2-rx1))*vars.scale,
y:vars.cy+y/z*vars.scale,
d:(x*x+y*y+z*z)
};
}else{
return { d:-1 };
}
}
function elevation(x,y,z){
var dist = Math.sqrt(x*x+y*y+z*z);
if(dist && z/dist>=-1 && z/dist <=1) return Math.acos(z / dist);
return 0.00000001;
}
function rgb(col){
col += 0.000001;
var r = parseInt((0.5+Math.sin(col)*0.5)*16);
var g = parseInt((0.5+Math.cos(col)*0.5)*16);
var b = parseInt((0.5-Math.sin(col)*0.5)*16);
return "#"+r.toString(16)+g.toString(16)+b.toString(16);
}
function interpolateColors(RGB1,RGB2,degree){
var w2=degree;
var w1=1-w2;
return [w1*RGB1[0]+w2*RGB2[0],w1*RGB1[1]+w2*RGB2[1],w1*RGB1[2]+w2*RGB2[2]];
}
function rgbArray(col){
col += 0.000001;
var r = parseInt((0.5+Math.sin(col)*0.5)*256);
var g = parseInt((0.5+Math.cos(col)*0.5)*256);
var b = parseInt((0.5-Math.sin(col)*0.5)*256);
return [r, g, b];
}
function colorString(arr){
var r = parseInt(arr[0]);
var g = parseInt(arr[1]);
var b = parseInt(arr[2]);
return "#"+("0" + r.toString(16) ).slice (-2)+("0" + g.toString(16) ).slice (-2)+("0" + b.toString(16) ).slice (-2);
}
function process(vars){
if(vars.points.length<vars.initParticles) for(var i=0;i<5;++i) spawnParticle(vars);
var p,d,t;
p = Math.atan2(vars.camX, vars.camZ);
d = Math.sqrt(vars.camX * vars.camX + vars.camZ * vars.camZ);
d -= Math.sin(vars.frameNo / 80) / 25;
t = Math.cos(vars.frameNo / 300) / 165;
vars.camX = Math.sin(p + t) * d;
vars.camZ = Math.cos(p + t) * d;
vars.camY = -Math.sin(vars.frameNo / 220) * 15;
vars.yaw = Math.PI + p + t;
vars.pitch = elevation(vars.camX, vars.camZ, vars.camY) - Math.PI / 2;
var t;
for(var i=0;i<vars.points.length;++i){
x=vars.points[i].x;
y=vars.points[i].y;
z=vars.points[i].z;
d=Math.sqrt(x*x+z*z)/1.0075;
t=.1/(1+d*d/5);
p=Math.atan2(x,z)+t;
vars.points[i].x=Math.sin(p)*d;
vars.points[i].z=Math.cos(p)*d;
vars.points[i].y+=vars.points[i].vy*t*((Math.sqrt(vars.distributionRadius)-d)*2);
if(vars.points[i].y>vars.vortexHeight/2 || d<.25){
vars.points.splice(i,1);
spawnParticle(vars);
}
}
}
function drawFloor(vars){
var x,y,z,d,point,a;
for (var i = -25; i <= 25; i += 1) {
for (var j = -25; j <= 25; j += 1) {
x = i*2;
z = j*2;
y = vars.floor;
d = Math.sqrt(x * x + z * z);
point = project3D(x, y-d*d/85, z, vars);
if (point.d != -1) {
size = 1 + 15000 / (1 + point.d);
a = 0.15 - Math.pow(d / 50, 4) * 0.15;
if (a > 0) {
vars.ctx.fillStyle = colorString(interpolateColors(rgbArray(d/26-vars.frameNo/40),[0,128,32],.5+Math.sin(d/6-vars.frameNo/8)/2));
vars.ctx.globalAlpha = a;
vars.ctx.fillRect(point.x-size/2,point.y-size/2,size,size);
}
}
}
}
vars.ctx.fillStyle = "#82f";
for (var i = -25; i <= 25; i += 1) {
for (var j = -25; j <= 25; j += 1) {
x = i*2;
z = j*2;
y = -vars.floor;
d = Math.sqrt(x * x + z * z);
point = project3D(x, y+d*d/85, z, vars);
if (point.d != -1) {
size = 1 + 15000 / (1 + point.d);
a = 0.15 - Math.pow(d / 50, 4) * 0.15;
if (a > 0) {
vars.ctx.fillStyle = colorString(interpolateColors(rgbArray(-d/26-vars.frameNo/40),[32,0,128],.5+Math.sin(-d/6-vars.frameNo/8)/2));
vars.ctx.globalAlpha = a;
vars.ctx.fillRect(point.x-size/2,point.y-size/2,size,size);
}
}
}
}
}
function sortFunction(a,b){
return b.dist-a.dist;
}
function draw(vars){
vars.ctx.globalAlpha=.15;
vars.ctx.fillStyle="#000";
vars.ctx.fillRect(0, 0, canvas.width, canvas.height);
drawFloor(vars);
var point,x,y,z,a;
for(var i=0;i<vars.points.length;++i){
x=vars.points[i].x;
y=vars.points[i].y;
z=vars.points[i].z;
point=project3D(x,y,z,vars);
if(point.d != -1){
vars.points[i].dist=point.d;
size=1+vars.points[i].radius/(1+point.d);
d=Math.abs(vars.points[i].y);
a = .8 - Math.pow(d / (vars.vortexHeight/2), 1000) * .8;
vars.ctx.globalAlpha=a>=0&&a<=1?a:0;
vars.ctx.fillStyle=rgb(vars.points[i].color);
if(point.x>-1&&point.x<vars.canvas.width&&point.y>-1&&point.y<vars.canvas.height)vars.ctx.fillRect(point.x-size/2,point.y-size/2,size,size);
}
}
vars.points.sort(sortFunction);
}
function spawnParticle(vars){
var p,ls;
pt={};
p=Math.PI*2*Math.random();
ls=Math.sqrt(Math.random()*vars.distributionRadius);
pt.x=Math.sin(p)*ls;
pt.y=-vars.vortexHeight/2;
pt.vy=vars.initV/20+Math.random()*vars.initV;
pt.z=Math.cos(p)*ls;
pt.radius=200+800*Math.random();
pt.color=pt.radius/1000+vars.frameNo/250;
vars.points.push(pt);
}
function frame(vars) {
if(vars === undefined){
var vars={};
vars.canvas = document.querySelector("canvas");
vars.ctx = vars.canvas.getContext("2d");
vars.canvas.width = document.body.clientWidth;
vars.canvas.height = document.body.clientHeight;
window.addEventListener("resize", function(){
vars.canvas.width = document.body.clientWidth;
vars.canvas.height = document.body.clientHeight;
vars.cx=vars.canvas.width/2;
vars.cy=vars.canvas.height/2;
}, true);
vars.frameNo=0;
vars.camX = 0;
vars.camY = 0;
vars.camZ = -14;
vars.pitch = elevation(vars.camX, vars.camZ, vars.camY) - Math.PI / 2;
vars.yaw = 0;
vars.cx=vars.canvas.width/2;
vars.cy=vars.canvas.height/2;
vars.bounding=10;
vars.scale=500;
vars.floor=26.5;
vars.points=[];
vars.initParticles=700;
vars.initV=.01;
vars.distributionRadius=800;
vars.vortexHeight=25;
}
vars.frameNo++;
requestAnimationFrame(function() {
frame(vars);
});
process(vars);
draw(vars);
}
frame();
</script>
</body>
</html>
8.月亮
此处我只发关键代码 详细已上传至CSDN资源
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>夜晚天空月亮动画特效</title>
<link type="text/css" href="css/main.css" rel="stylesheet">
<link type="text/css" href="css/style.css" rel="stylesheet">
</head>
<body>
<div id="e_background">
<div id="e_smallstars"></div>
<div id="e_moon"></div>
</div>
</body>
</html>
以上所有源码已全部上传至CSDN资源
更多推荐
所有评论(0)