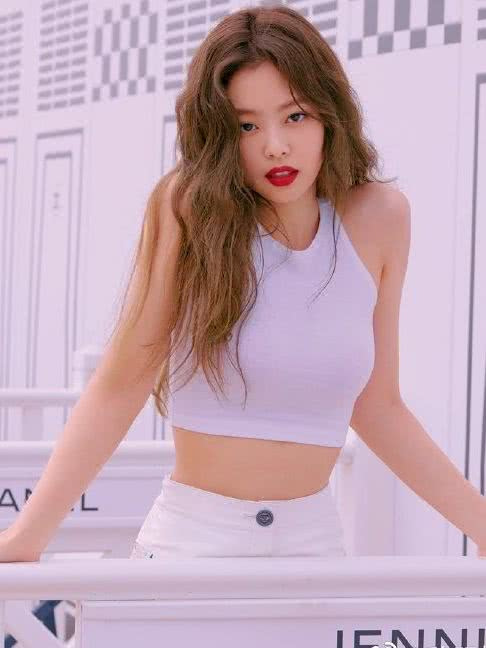
Spring Cloud Gateway 全局异常处理
对于spring boot 项目 全局异常拦截可以使用@RestControllerAdvice 和@ExceptionHandler(Exception.class) 注解 进行全局异常处理。对于Gateway 内部的异常处理需要使用如下方法package config.exception;import org.springframework.beans.factory.ObjectProvid
·
对于spring boot 项目 全局异常拦截可以使用 @RestControllerAdvice 和@ExceptionHandler(Exception.class) 注解 进行全局异常处理。对于Gateway 内部的异常处理需要使用如下方法
package config.exception;
import org.springframework.beans.factory.ObjectProvider;
import org.springframework.boot.autoconfigure.web.ResourceProperties;
import org.springframework.boot.autoconfigure.web.ServerProperties;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.boot.web.reactive.error.ErrorAttributes;
import org.springframework.boot.web.reactive.error.ErrorWebExceptionHandler;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.core.Ordered;
import org.springframework.core.annotation.Order;
import org.springframework.http.codec.ServerCodecConfigurer;
import org.springframework.web.reactive.result.view.ViewResolver;
import java.util.Collections;
import java.util.List;
@Configuration
@EnableConfigurationProperties({ServerProperties.class, ResourceProperties.class})
public class GatewayErrorConfiguration {
private final ServerProperties serverProperties;
private final ApplicationContext applicationContext;
private final ResourceProperties resourceProperties;
private final List<ViewResolver> viewResolvers;
private final ServerCodecConfigurer serverCodecConfigurer;
public GatewayErrorConfiguration(ServerProperties serverProperties,
ResourceProperties resourceProperties,
ObjectProvider<List<ViewResolver>> viewResolversProvider,
ServerCodecConfigurer serverCodecConfigurer,
ApplicationContext applicationContext) {
this.serverProperties = serverProperties;
this.applicationContext = applicationContext;
this.resourceProperties = resourceProperties;
this.viewResolvers = viewResolversProvider.getIfAvailable(Collections::emptyList);
this.serverCodecConfigurer = serverCodecConfigurer;
}
@Bean
@Order(Ordered.HIGHEST_PRECEDENCE)
public ErrorWebExceptionHandler errorWebExceptionHandler(ErrorAttributes errorAttributes) {
GatewayJsonExceptionHandler exceptionHandler = new GatewayJsonExceptionHandler(
errorAttributes,
this.resourceProperties,
this.serverProperties.getError(),
this.applicationContext);
exceptionHandler.setViewResolvers(this.viewResolvers);
exceptionHandler.setMessageWriters(this.serverCodecConfigurer.getWriters());
exceptionHandler.setMessageReaders(this.serverCodecConfigurer.getReaders());
return exceptionHandler;
}
}
package config.exception;
import common.BaseException;
import org.springframework.boot.autoconfigure.web.ErrorProperties;
import org.springframework.boot.autoconfigure.web.ResourceProperties;
import org.springframework.boot.autoconfigure.web.reactive.error.DefaultErrorWebExceptionHandler;
import org.springframework.boot.web.reactive.error.ErrorAttributes;
import org.springframework.context.ApplicationContext;
import org.springframework.http.HttpStatus;
import org.springframework.web.reactive.function.server.RequestPredicates;
import org.springframework.web.reactive.function.server.RouterFunction;
import org.springframework.web.reactive.function.server.RouterFunctions;
import org.springframework.web.reactive.function.server.ServerRequest;
import org.springframework.web.reactive.function.server.ServerResponse;
import java.util.HashMap;
import java.util.Map;
public class GatewayJsonExceptionHandler extends DefaultErrorWebExceptionHandler {
public GatewayJsonExceptionHandler(ErrorAttributes errorAttributes, ResourceProperties resourceProperties,
ErrorProperties errorProperties, ApplicationContext applicationContext) {
super(errorAttributes, resourceProperties, errorProperties, applicationContext);
}
@Override
protected Map<String, Object> getErrorAttributes(ServerRequest request, boolean includeStackTrace) {
int status = 500;
String code = "";
String errorMessage = "系统异常";
Throwable error = super.getError(request);
if (error instanceof BaseException) {
status = 200;
BaseException baseException = (BaseException) error;
code = baseException.getCode();
errorMessage = baseException.getMessage();
}
Map<String, Object> map = new HashMap<>();
map.put("status", status);
map.put("code", code);
map.put("message", errorMessage);
map.put("data", null);
return map;
}
@Override
protected RouterFunction<ServerResponse> getRoutingFunction(ErrorAttributes errorAttributes) {
return RouterFunctions.route(RequestPredicates.all(), this::renderErrorResponse);
}
// @Override
// protected RouterFunction<ServerResponse> getRoutingFunction(
// ErrorAttributes errorAttributes) {
// return RouterFunctions.route(acceptsTextHtml(), this::renderErrorView)
// .andRoute(RequestPredicates.all(), this::renderErrorResponse);
// }
@Override
protected HttpStatus getHttpStatus(Map<String, Object> errorAttributes) {
return HttpStatus.valueOf((int)errorAttributes.get("status"));
}
}
更多推荐
所有评论(0)