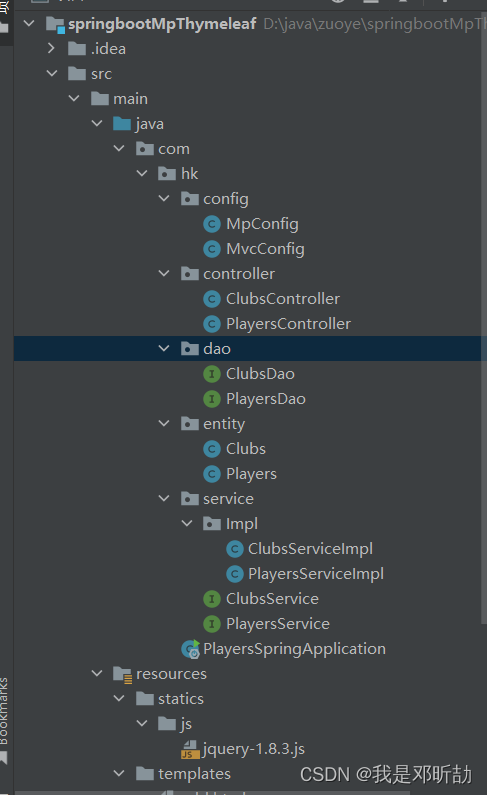
springboot加mybatisplus加thymeleaf模板写增删改查
朴实无华,如有不对,多多指教
·
项目创建配置信息
首先创建一个springboot项目,导入相关依赖,boot版本为2.6.2
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.2</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.2</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid-spring-boot-starter</artifactId>
<version>1.2.15</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
</dependencies>
springboot配置文件:
#配置端口
server:
port: 8080
mybatis-plus:
#别名扫描包
type-aliases-package: com.hk.entity
#开启运行日志
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
spring:
#配置数据源
datasource:
druid:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/ssmfb?characterEncoding=GBK&serverTimezone=UTC
username: root
password: root
#配置模板
thymeleaf:
prefix: classpath:/templates/
suffix: .html
#开发静态资源访问
mvc:
static-path-pattern: classpath:/statics/**
后端代码
项目结构
然后创建对应的dao层,service,serviceImpl,controller层
dao层:
@Mapper
public interface PlayersDao extends BaseMapper<Players> {
}
service和Impl层:
public interface PlayersService extends IService<Players> {
}
@Service
public class PlayersServiceImpl extends ServiceImpl<PlayersDao, Players> implements PlayersService {
}
controller层:
package com.hk.controller;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.hk.entity.Clubs;
import com.hk.entity.Players;
import com.hk.service.ClubsService;
import com.hk.service.PlayersService;
import org.apache.ibatis.annotations.Delete;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.servlet.ModelAndView;
import java.util.List;
/**
* @PACKAGE_NAME: com.hk.controller
* @USER: Admin_HK
* @DATE: 2022/11/21
* @DAY_NAME_SHORT: 周一
*/
@Controller
@RequestMapping("/players")
public class PlayersController {
@Autowired
private PlayersService playersService;
@Autowired
private ClubsService clubsService;
@GetMapping
public String index(){
System.out.println("=========");
return "forward:/templates/list.html";
}
@GetMapping("/list")
public ModelAndView list(int pageNum, int pageSize, ModelAndView modelAndView){
// 配置分页构造器,传入分页参数
Page<Players>page=new Page<>(pageNum,pageSize);
// 调用方法,将分页构造器传入,要有分页配置类,分页功能才会生效
Page<Players> playersPage = playersService.page(page,null);
// 两表操作,遍历查询出的单表对象
for (Players players : playersPage.getRecords()) {
// 根据每个对象中的外键关系去主表中查询出一个对象
Clubs clubs = clubsService.getById(players.getCid());
// 判断对象不为空
if(clubs!=null){
// 将对象存入我要返回的对象中,这样我这个对象有自己的属性,还有别的表的属性
players.setClubs(clubs);
}
}
// 将page对象存入模型对象中
modelAndView.addObject("list",playersPage);
// 设置跳转路径
modelAndView.setViewName("list");
return modelAndView;
}
@DeleteMapping("delete")
@ResponseBody
public boolean deleteById(Integer id){
return playersService.removeById(id);
}
@GetMapping("/updateById")
public ModelAndView updateById(Integer pid,ModelAndView modelAndView){
// 根据id查询返回一个对象
Players players = playersService.getById(pid);
// 页面有下拉框,去查询另一张表的全部数据
List<Clubs> clubs = clubsService.list(null);
// 将数据存入模型对象中
modelAndView.addObject("players",players);
modelAndView.addObject("list",clubs);
// 跳转到修改页面
modelAndView.setViewName("update");
return modelAndView;
}
@PutMapping("/update")
@ResponseBody
public boolean update( Players players){
// 接收对象,将对象传入修改方法中,然后返回boolean类型在页面判断是否修改成功
return playersService.updateById(players);
}
@PostMapping("/save")
@ResponseBody
public boolean save(Players players){
// 接收对象,将对象传入新增方法中,然后返回boolean类型在页面判断是否新增成功
return playersService.save(players);
}
}
如果需要分页还需要加一个配置类 配置在config层中:
package com.hk.config;
import com.baomidou.mybatisplus.extension.plugins.MybatisPlusInterceptor;
import com.baomidou.mybatisplus.extension.plugins.inner.PaginationInnerInterceptor;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
/**
* @PACKAGE_NAME: com.hk.config
* @USER: Admin_HK
* @DATE: 2022/11/21
* @DAY_NAME_SHORT: 周一
*/
@Configuration
public class MpConfig {
// 分页配置
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor(){
MybatisPlusInterceptor interceptor=new MybatisPlusInterceptor();
interceptor.addInnerInterceptor(new PaginationInnerInterceptor());
return interceptor;
}
}
配置类开放目录访问,put请求拿到请求参数
package com.hk.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.filter.FormContentFilter;
import org.springframework.web.filter.HttpPutFormContentFilter;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurationSupport;
import org.springframework.web.servlet.mvc.HttpRequestHandlerAdapter;
/**
* @PACKAGE_NAME: com.hk.config
* @USER: Admin_HK
* @DATE: 2022/11/21
* @DAY_NAME_SHORT: 周一
*/
@Configuration
public class MvcConfig extends WebMvcConfigurationSupport {
private static final String[] CLASSPATH_RESOURCE_LOCATIONS = {
"classpath:/META-INF/resources/", "classpath:/resources/",
"classpath:/statics/", "classpath:/public/","classpath:/templates/"};
// 开放资源下目录访问
@Override
protected void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/**").addResourceLocations(CLASSPATH_RESOURCE_LOCATIONS);
}
// 开放put请求
@Bean
public FormContentFilter formContentFilter(){
return new FormContentFilter();
}
}
前台页面显示数据上 导入jquery包:
然后通过访问controller查询方法跳转到页面带参数,通过使用thymeleaf遍历拿到
用之前需要在头部引入路径
<html xmlns:th="http://www.thymeleaf.org" lang="en">
引入jquery包
<script th:src="@{/js/jquery-1.8.3.js}" ></script>
然后在tr中通过 th:each遍历list集合 plaear是给的别名,因为我这是分页对象,通过对象去拿到list集合数据
然后通过 th:text 去显示数据 如果是input标签 就通过 th:value 去显示
在函数中需要传入参数,
th:οnclick="|deleteById(${players.pid})|" 这样传入即可,可以通过弹窗验证一下
<table border="1px">
<tr>
<th>球员编号</th>
<th>球员名称</th>
<th>出生时间(yyyy-MM-dd)</th>
<th>球员身高(单位:cm)</th>
<th>球员体重(单位:kg)</th>
<th>球员位置</th>
<th>所属球队</th>
<th>相关操作</th>
</tr>
<tr th:each="players:${list.records}">
<td th:text="${players.pid}" ></td>
<td th:text="${players.pname}"></td>
<td th:text="${players.birthday}"></td>
<td th:text="${players.height}"></td>
<td th:text="${players.weight}"></td>
<td th:text="${players.postition}"></td>
<td th:text="${players.clubs.cname}"></td>
<td>
<a href="#" th:onclick="|deleteById(${players.pid})|">删除</a>
<a href="#" th:onclick="|updateById(${players.pid})|">修改</a>
</td>
</tr>
</table>
js函数:通过ajax方式发送异步请求,通过回调函数拿到响应的数据,判断是否操作成功,给出提示,然后跳转查询路径
<script>
function updateById(pid){
location.href="/players/updateById?pid="+pid;
}
function add(){
location.href="/clubs/list";
}
function deleteById(pid){
alert(pid);
$.ajax({
url:"/players/delete",
data:{
"id":pid
},
success:function (res){
if(res===true){
alert("删除成功!!!")
location.href="/players/list?pageNum=1&pageSize=5";
}else{
alert("删除失败!!!")
}
}
})
}
</script>
修改页面回显数据
修改是input标签 通过 th:value去显示数据
单选框通过 th:checked 去判断回显过来的值和单选框的值是否相同,
如果相同就会显示为选中状态
下拉框通过list集合遍历
然后通过 th:selected 判断回显的id和我遍历的id是否相同,相同则为选中状态
th:selected="${players.cid}==${clubs.cid}
value是值,text是下拉框显示的值
<h1>修改球员信息</h1>
<form>
<input type="hidden" name="pid" id="pid" th:value="${players.pid}">
球员姓名: <input type="text" name="pname" th:value="${players.pname}" id="pname"> <br>
出生时间: <input type="text" name="birthday" th:value="${players.birthday}" id="birthday">(yyyy-MM-dd) <br>
球员身高: <input type="text" name="height" th:value="${players.height}" id="height">(单位:cm) <br>
球员体重: <input type="text" name="weight" th:value="${players.weight}" id="weight">(单位:kg) <br>
球员位置:
<input type="radio" value="控球后卫" th:checked="${players.postition eq '控球后卫'}" name="postition" id="">控球后卫
<input type="radio" value="得分后卫" th:checked="${players.postition eq '得分后卫'}" name="postition" id="">得分后卫
<input type="radio" value="小前锋" th:checked="${players.postition eq '小前锋'}" name="postition" id="">小前锋
<input type="radio" value="大前锋" th:checked="${players.postition eq '大前锋'}" name="postition" id="">大前锋
<input type="radio" value="中锋" th:checked="${players.postition eq '中锋'}" name="postition" id="">中锋
<br>
所属球队:
<select id="cname" name="cid">
<option>-请选择-</option>
<option th:each="clubs:${list}" th:value="${clubs.cid}" th:selected="${players.cid}==${clubs.cid}" th:text="${clubs.cname}"></option>
</select> <br>
相关操作: <input type="button" onclick="update()" value="修改">
<input type="button" value="返回" onclick="fh()">
</form>
修改和新增就通过异步方式请求,回调函数拿到响应判断是否操作成功,然后跳转查询方法
更多推荐
所有评论(0)