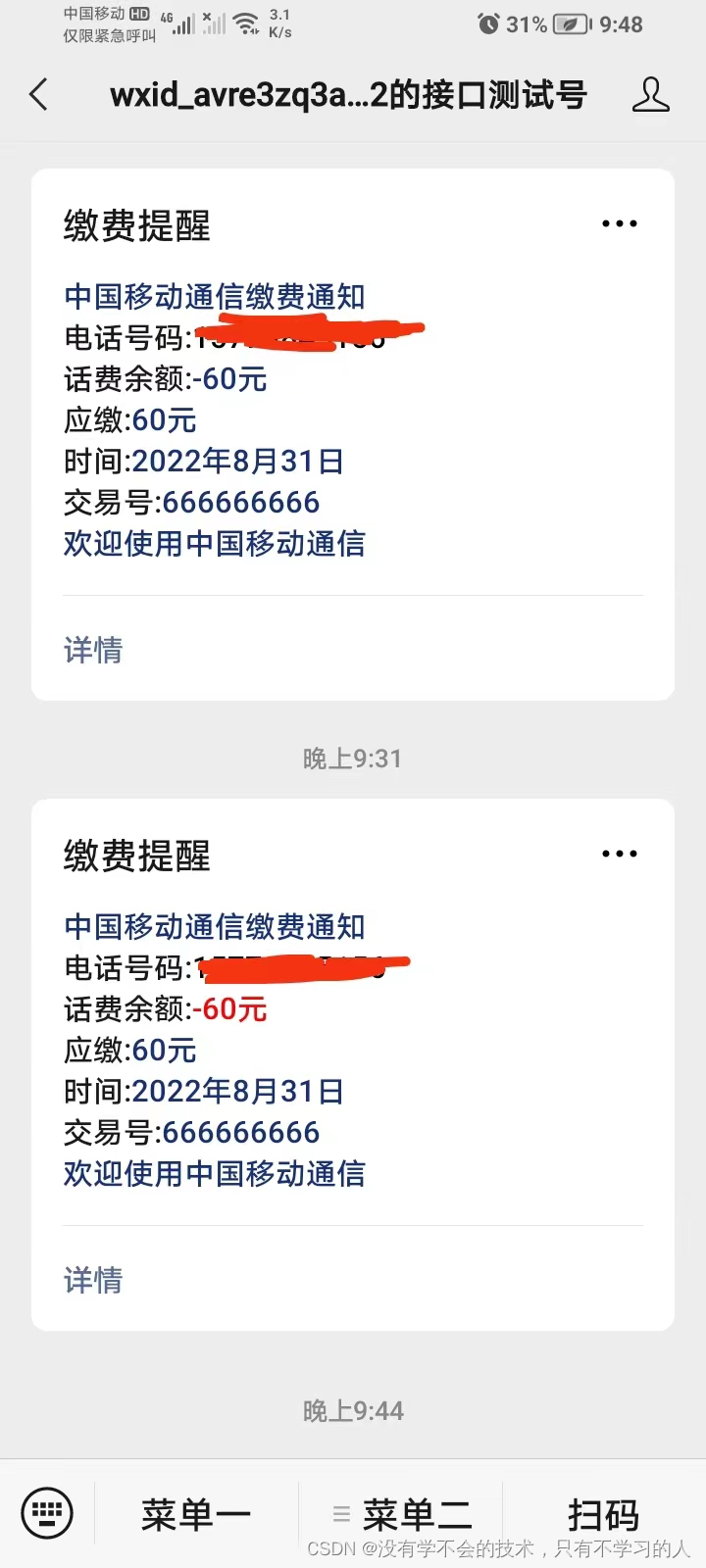
SpringBoot 实现微信模板消息通知推送提醒
SpringBoot 实现微信模板消息通知推送提醒
·
添加依赖
在SpringBoot项目中添加依赖
<!--微信模版消息推送三方sdk-->
<dependency>
<groupId>com.github.binarywang</groupId>
<artifactId>weixin-java-mp</artifactId>
<version>3.3.0</version>
</dependency>
控制层代码
package com.laoxu.demo.bootstrapcurd.controller;
import com.laoxu.demo.bootstrapcurd.service.message.TemplateService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
/**
* @author: wangxiaobo
* @create: 2022-08-31 21:03
* 模板微信推送消息处理接口
**/
@RestController
@RequestMapping("/v1/weChar")
public class TemplateController {
@Autowired
private TemplateService templateService;
/**
* 设置行业信息
* @param body 请求对象
* @return
* @throws IOException
*/
@RequestMapping("/setIndustry")
public String setIndustry(@RequestBody String body) throws IOException {
return templateService.setIndustry(body);
}
/**
* 获取行业信息
* @return
* @throws IOException
*/
@RequestMapping("/getIndustry")
public String setIndustry() throws IOException {
return templateService.getIndustry();
}
/**
* 获取微信公众平台全部模版消息
* @return
* @throws IOException
*/
@RequestMapping("/getTemplateList")
public String getTemplateList() throws IOException {
return templateService.getTemplateList();
}
/**
* 发送模版消息
* @param body
* @return
* @throws IOException
*/
@RequestMapping("/send")
public String send(@RequestBody String body) throws IOException {
return templateService.send(body);
}
}
去微信公众平台注册一个开发测试账号
个人开发,我们可以去微信公众号平台注册个测试账户点我直达,微信扫码登录,会给我们一个免费的:appID、appsecret,微信扫码关注公众号,会显示关注测试公众号的用户列表。全局错误码:点我直达
测试
关注测试公众号,创建模板,并发送指定模板内容
替换版本内容
在微信公众平台创建模板
语法:{{变量名.DATA}}
姓名:{{user_name.DATA}}
性别:{{sex.DATA}}
手机号:{{phone.DATA}}
邮箱:{{email.DATA}}
模板消息处理服务类代码
package com.laoxu.demo.bootstrapcurd.service.message;
import com.laoxu.demo.bootstrapcurd.bean.HttpClientUtils;
import com.laoxu.demo.bootstrapcurd.constant.WeCharConstant;
import com.laoxu.demo.bootstrapcurd.service.AccessTokenService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.io.IOException;
/**
* @author: wangxiaobo
* @create: 2022-08-31 20:59
* 模板消息处理服务类
**/
@Service
public class TemplateService {
@Autowired
private AccessTokenService accessTokenService;
/**
* 设置行业信息
*
* @param body 请求对象
* @return
* @throws IOException
*/
public String setIndustry(String body) throws IOException {
return HttpClientUtils.post(WeCharConstant.SET_INDUSTRY.replace("ACCESS_TOKEN", accessTokenService.getAccessToken()),
body);
}
/**
* 获取行业信息
*
* @return
* @throws IOException
*/
public String getIndustry() throws IOException {
return HttpClientUtils.get(WeCharConstant.GET_INDUSTRY.replace("ACCESS_TOKEN", accessTokenService.getAccessToken()));
}
/**
* 获取微信公众平台全部模版消息
*
* @return
* @throws IOException
*/
public String getTemplateList() throws IOException {
return HttpClientUtils.get(WeCharConstant.GET_ALL_PRIVATE_TEMPLATE.replace("ACCESS_TOKEN", accessTokenService.getAccessToken()));
}
/**
* 发送模版消息
*
* @param body 请求数据
* @return
* @throws IOException
*/
public String send(String body) throws IOException {
return HttpClientUtils.post(WeCharConstant.TEMPLATE_SEND.replace("ACCESS_TOKEN", accessTokenService.getAccessToken()), body);
}
}
获取微信Access token用来做获取其他接口验证鉴权
AccessTokenService.java
获取Access token服务层
package com.laoxu.demo.bootstrapcurd.service;
import com.alibaba.fastjson.JSONObject;
import com.laoxu.demo.bootstrapcurd.bean.AccessTokenBean;
import com.laoxu.demo.bootstrapcurd.bean.HttpClientUtils;
import com.laoxu.demo.bootstrapcurd.config.WeCharConfig;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.io.IOException;
/**
* @author: wangxiaobo
* @create: 2022-08-29 13:15
* 获取Access token服务层
**/
@Service
public class AccessTokenService {
@Autowired
private WeCharConfig weCharConfig;
/**
* access Token对象
*/
private AccessTokenBean accessTokenBean;
private static final String URL="https://api.weixin.qq.com/cgi-bin/token";
/**
* 获取 access Token
* @param appId
* @param appSecret
* @throws Exception
*/
private void refreshAccessToken(String appId, String appSecret) throws IOException {
String s = HttpClientUtils.get(URL,AccessTokenBean.requesOf (appId,appSecret));
JSONObject jsonObject = JSONObject.parseObject (s);
long expiresIn = jsonObject.getLong("expires_in");
String accessToken = jsonObject.getString("access_token");
accessTokenBean = AccessTokenBean.responseOf(accessToken,expiresIn);
System.out.println(s);
}
/**
* 获取 access Token
* @return
*/
public String getAccessToken() throws IOException {
if(accessTokenBean==null || accessTokenBean.isExpired()){
refreshAccessToken(weCharConfig.getAppId (),weCharConfig.getSecret());
}
return accessTokenBean.getAccess_token ();
}
public static void main(String[] args) throws IOException {
AccessTokenService accessTokenService = new AccessTokenService ();
accessTokenService.refreshAccessToken("wxc241d23b510947a4","5cfef2542ececfae0357f3f125e39f17");
}
}
AccessTokenBean.Java
access token对象
package com.laoxu.demo.bootstrapcurd.bean;
import lombok.Getter;
import java.io.Serializable;
import java.util.HashMap;
/**
* access token对象
* @author: wangxiaobo
* @create: 2022-08-29 12:51
*
**/
@Getter
public class AccessTokenBean implements Serializable {
/**
* 获取access_token接口对象参数
*/
/**
* 获取access_token填写client_credential
*/
private String grant_type="client_credential";
/**
* 第三方用户唯一凭证
*/
private String appid;
/**
* 第三方用户唯一凭证密钥,即appsecret
*/
private String secret;
/**
* 返回参数说明
*/
/**
* access_token获取到的凭证
*/
private String access_token;
/**
* 凭证有效时间,单位:秒
*/
private long expires_in;
public AccessTokenBean( String appid, String secret, String access_token, long expires_in) {
this.appid = appid;
this.secret = secret;
this.access_token = access_token;
//这样就拿到过期失效时间
if (expires_in > 0){
this.expires_in = System.currentTimeMillis() + expires_in * 1000;
}
this.expires_in = expires_in;
}
/**
* 构建获取 access_token请求对象
* @param appid 用户唯一标识
* @param secret 用户唯一标识密钥
* @return
*/
public static HashMap<String,String> requesOf(String appid, String secret){
HashMap<String, String> map = new HashMap<> (16);
map.put("appid",appid);
map.put("secret",secret);
map.put("grant_type","client_credential");
return map;
}
/**
* 构建access_token对象
* @param access_token token
* @param expires_in 过期时间
* @return
*/
public static AccessTokenBean responseOf(String access_token, long expires_in){
return new AccessTokenBean ("","", access_token,expires_in);
}
/**
* 判断token是否已经失效
* @return 是否失效 false 未失效 true 失效,需要重新申请
*/
public boolean isExpired(){
return System.currentTimeMillis ()>expires_in;
}
}
import lombok.Data;
import lombok.Getter;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
/**
* @author: wangxiaobo
* @create: 2022-08-29 13:17
**/
@Component
@Data
@ConfigurationProperties(prefix = "we-char")
@Getter
public class WeCharConfig {
/**
* 第三方用户唯一凭证
*/
private String appId;
/**
* 第三方用户唯一凭证密钥,即appsecret
*/
private String secret;
/**
* 加密token
*/
private String token;
}
测试获取微信Access token接口
package com.laoxu.demo.bootstrapcurd.controller;
import com.laoxu.demo.bootstrapcurd.service.AccessTokenService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* @author: wangxiaobo
* @create: 2022-08-29 13:56
**/
@RestController
@RequestMapping("/accessToken")
public class AccessTokenController {
@Autowired
private AccessTokenService accessTokenService;
/**
* 获取微信Access token接口
* @return
* @throws Exception
*/
@RequestMapping
public String getAccessToken() throws Exception {
return accessTokenService.getAccessToken();
}
}
access_token:接口调用凭证
expires_in:过期时间
{"access_token":"60_A8T9k5Plmyxkc6PG5dHYXhRsBvb9Ce1HN67a9awvHRWsS7nxWwKWuR6kU1G_yyINRJLPiEoluHKoGKqWvwls2wbz32tvxr4UdW2f_bqX4camjhuDXCkL-IlYZbukpc9zjaBjHACElJTokqflJVMjABAYKB","expires_in":7200}
微信公众平台常量类
package com.laoxu.demo.bootstrapcurd.constant;
/**
* @author: wangxiaobo
* @create: 2022-08-29 22:09
* 微信公众平台常量类
**/
public class WeCharConstant {
public static final String CREATE_TIME = "CreateTime";
public static final String CONTENT = "Content";
public static final String FROM_USER_NAME = "FromUserName";
public static final String TO_USER_NAME = "ToUserName";
public static final String MSG_TYPE = "MsgType";
public static final String MSG_ID = "MsgId";
/**
* 创建菜单URL
*/
public static final String CREATE_MENU_URL = "https://api.weixin.qq.com/cgi-bin/menu/create?access_token=ACCESS_TOKEN";
/**
* 删除菜单URL
*/
public static final String DELETE_MENU_URL = "https://api.weixin.qq.com/cgi-bin/menu/delete?access_token=ACCESS_TOKEN";
/**
* 模版服务相关-设置所属行业
*/
public static final String SET_INDUSTRY = "https://api.weixin.qq.com/cgi-bin/template/api_set_industry?access_token=ACCESS_TOKEN";
/**
* 获取所属行业信息
*/
public static final String GET_INDUSTRY = "https://api.weixin.qq.com/cgi-bin/template/get_industry?access_token=ACCESS_TOKEN";
/**
* 获取所有模版信息
*/
public static final String GET_ALL_PRIVATE_TEMPLATE = "https://api.weixin.qq.com/cgi-bin/template/get_all_private_template?access_token=ACCESS_TOKEN";
/**
* 发送模版消息
*/
public static final String TEMPLATE_SEND = "https://api.weixin.qq.com/cgi-bin/message/template/send?access_token=ACCESS_TOKEN";
/**
* 获取OAUTH认证的access_token
*/
public static final String OAUTH_GET_AT = "https://api.weixin.qq.com/sns/oauth2/access_token?appid=APPID&secret=SECRET&code=CODE&grant_type=authorization_code";
/**
* 获取用户基本信息
*/
public static final String OAUTH_USER_INFO = "https://api.weixin.qq.com/sns/userinfo?access_token=ACCESS_TOKEN&openid=OPENID&lang=zh_CN";
/**
* 引导链接
*/
public static final String OAUTH2_AUTHORIZE = "https://open.weixin.qq.com/connect/oauth2/authorize?appid=APPID&redirect_uri=REDIRECT_URI&response_type=code&scope=SCOPE&state=STATE#wechat_redirect";
}
接下来就是微信测试模板消息推送
package com.laoxu.demo.bootstrapcurd.controller;
import com.laoxu.demo.bootstrapcurd.service.message.TemplateService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
/**
* @author: wangxiaobo
* @create: 2022-08-31 21:03
* 模板微信推送消息处理接口
**/
@RestController
@RequestMapping("/v1/weChar")
public class TemplateController {
@Autowired
private TemplateService templateService;
/**
* 设置行业信息
* @param body 请求对象
* @return
* @throws IOException
*/
@RequestMapping("/setIndustry")
public String setIndustry(@RequestBody String body) throws IOException {
return templateService.setIndustry(body);
}
/**
* 获取行业信息
* @return
* @throws IOException
*/
@RequestMapping("/getIndustry")
public String setIndustry() throws IOException {
return templateService.getIndustry();
}
/**
* 获取微信公众平台全部模版消息
* @return
* @throws IOException
*/
@RequestMapping("/getTemplateList")
public String getTemplateList() throws IOException {
return templateService.getTemplateList();
}
/**
* 发送模版消息
* @param body
* @return
* @throws IOException
*/
@RequestMapping("/send")
public String send(@RequestBody String body) throws IOException {
return templateService.send(body);
}
}
测试发送模板接口之前首先要获取模板ID:template_id
测试发送模板接口
touser:填你的openId就是扫测试号的微信号
template_id:模板ID
url:要跳转的链接
{
"touser":"owfI16osHtU_UV277BYfm0EwPJPo",
"template_id":"yuaFG-DmpAZiCM22aBQ9we3Luk8g6Xv400qQXcfn84g",
"url":"http://www.baidu.com",
"data":{
"first": {
"value":"中国移动通信缴费通知",
"color":"#173177"
},
"keyword1":{
"value":"填写手机号"
},
"keyword2": {
"value":"-60元",
"color":"#FF0000"
},
"keyword3": {
"value":"60元",
"color":"#173177"
},
"keyword4": {
"value":"2022年8月31日",
"color":"#173177"
},
"keyword5": {
"value":"666666666",
"color":"#173177"
},
"remark":{
"value":"欢迎使用中国移动通信",
"color":"#173177"
}
}
}
接受推送消息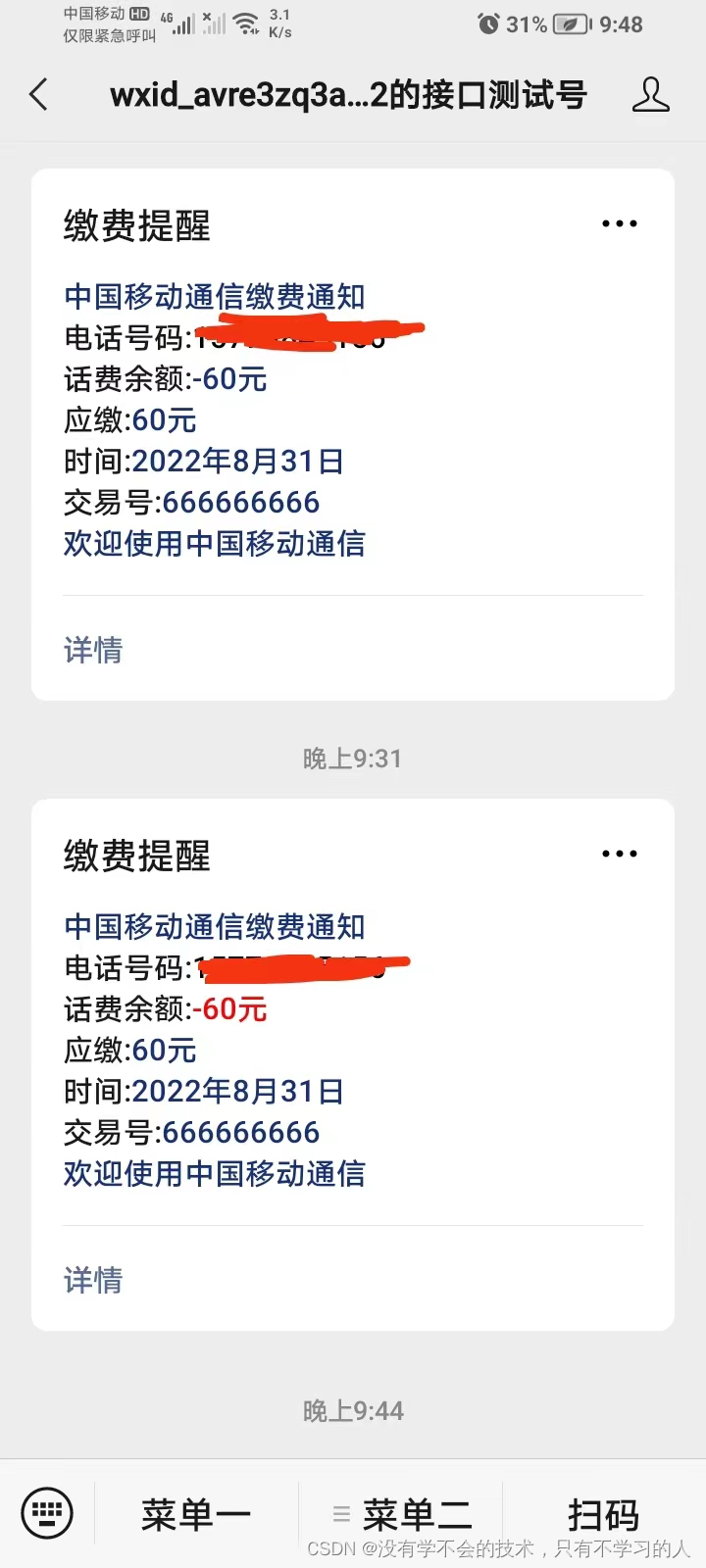
更多推荐
所有评论(0)