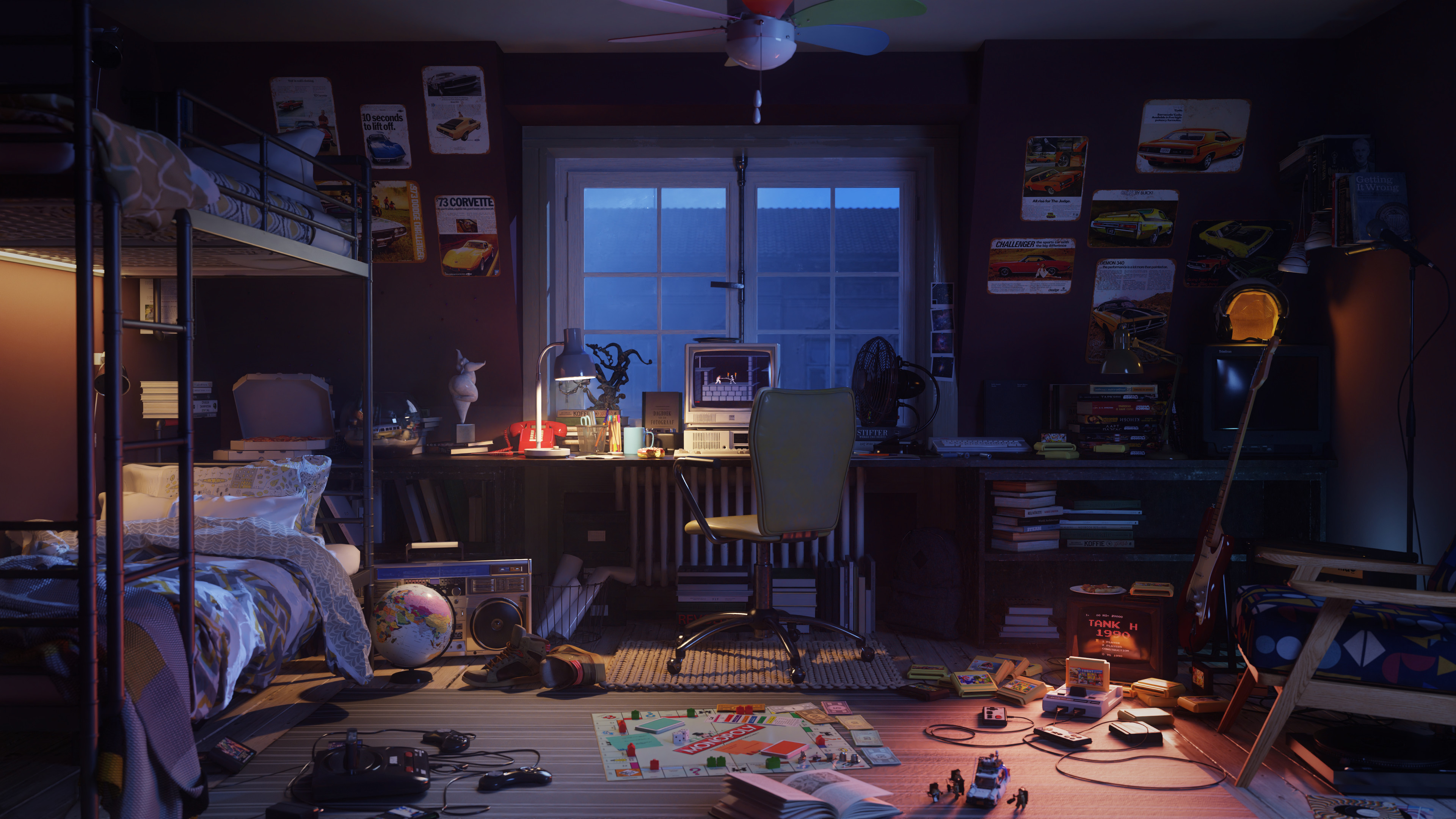
MyBatis-Plus代码生成器
MyBatis-Plus代码生成,附件相关代码+注释
·
这里用的是MyBatis-Plus的<version>2.2.0</version>版本,最新版本到了3.5.2,【注意,新版本的mybatis-plus不兼容老版本】
创建项目,我是把数据相关的封装抽出的新模块【可以不用】
domain、dto、vo数据封装 service服务
下面是代码生成器的封装:
我是把这个代码生成需要内容,新开的一个模块抽出来的,今后就可以直接打jar,引入直接使用了
第一步:导入pom
第二步:
resource下面
1.搞一个配置文件,用来设置代码输出相关的位置等..
2.生成的代码模板可以自己改,也可以用默认的
resource下创建一个templates文件夹,里面放入代码模板
如果自己想定义代码模板,可以从mybatis-plus下面存在的模板拷贝过来进行修改,具体怎么改就里面内容,官方可以去学习 MyBatis-Plus
第三步:编写代码生成器的类
代码:
pom:
<!--MyBatis-Plus的支持-->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!--模板引擎-->
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity-engine-core</artifactId>
<version>2.0</version>
</dependency>
<!--MySQL的JDBC驱动包,用JDBC连接MySQL数据库时必须使用该jar包。-->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
代码生成器类
package club.xler;
import com.baomidou.mybatisplus.exceptions.MybatisPlusException;
import com.baomidou.mybatisplus.generator.AutoGenerator;
import com.baomidou.mybatisplus.generator.InjectionConfig;
import com.baomidou.mybatisplus.generator.config.*;
import com.baomidou.mybatisplus.generator.config.converts.MySqlTypeConvert;
import com.baomidou.mybatisplus.generator.config.po.TableInfo;
import com.baomidou.mybatisplus.generator.config.rules.DbType;
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
import com.baomidou.mybatisplus.toolkit.StringUtils;
import java.util.*;
/**
* 生成代码的主类
* @author xler
*/
public class GeneratorCode {
/**
* 读取控制台内容
*/
public static String scanner(String tip) {
Scanner scanner = new Scanner(System.in);
StringBuilder help = new StringBuilder();
help.append("请输入" + tip + ":");
System.out.println(help.toString());
if (scanner.hasNext()) {
String ipt = scanner.next();
//MybatisPlus提供的工具类,做非空判断
if (StringUtils.isNotEmpty(ipt)) {
return ipt;
}
}
throw new MybatisPlusException("请输入正确的" + tip + "!");
}
public static void main(String[] args) throws InterruptedException {
//用来获取Mybatis-Plus.properties文件的配置信息
ResourceBundle rb = ResourceBundle.getBundle("mybatiesplus-config-wx");
AutoGenerator mpg = new AutoGenerator();
// 全局配置
GlobalConfig gc = new GlobalConfig();
gc.setOutputDir(rb.getString("OutputDir"));
gc.setFileOverride(true); //再次生成可以覆盖
gc.setActiveRecord(true);// 开启 activeRecord 模式
gc.setEnableCache(false);// XML 二级缓存
gc.setBaseResultMap(true);// XML ResultMap
gc.setBaseColumnList(true);// XML columList
gc.setAuthor(rb.getString("author"));
mpg.setGlobalConfig(gc);
// 数据源配置
DataSourceConfig dsc = new DataSourceConfig();
dsc.setDbType(DbType.MYSQL);
dsc.setTypeConvert(new MySqlTypeConvert());
dsc.setDriverName(rb.getString("jdbc.driver"));
dsc.setUsername(rb.getString("jdbc.user"));
dsc.setPassword(rb.getString("jdbc.pwd"));
dsc.setUrl(rb.getString("jdbc.url"));
mpg.setDataSource(dsc);
// 表策略配置
StrategyConfig strategy = new StrategyConfig();
//表名生成策略 underline_to_camel转驼峰命名,no_change默认的没变化
strategy.setNaming(NamingStrategy.underline_to_camel);
//列名生成策略 underline_to_camel转驼峰命名,no_change默认的没变化
strategy.setColumnNaming(NamingStrategy.underline_to_camel);
//strategy.setSuperEntityClass("com.baomidou.ant.common.BaseEntity");
// 是否使用Lombok优化代码
strategy.setEntityLombokModel(true);
// controller类是否直接返回json
strategy.setRestControllerStyle(true);
// 公共父类
//strategy.setSuperControllerClass("com.baomidou.ant.common.BaseController");
// 写于父类中的公共字段
//strategy.setSuperEntityColumns("id");
//要生成的表名(控制台输入)
// strategy.setInclude(scanner("表名,多个英文逗号分割").split(","));
// strategy.setControllerMappingHyphenStyle(true);
strategy.setInclude("xler_wx_user");
//生成的类名去掉前缀 如yw_sys_user ---> SysUser
strategy.setTablePrefix("xler_");
mpg.setStrategy(strategy);
// 包配置
PackageConfig pc = new PackageConfig();
pc.setParent(rb.getString("parent"));
pc.setController("web.controller");
pc.setService("service");
pc.setServiceImpl("service.impl");
pc.setEntity("entity");
pc.setMapper("mapper");
mpg.setPackageInfo(pc);
// 注入自定义配置,可以在 VM 中使用 cfg.abc 【可无】
InjectionConfig cfg = new InjectionConfig() {
@Override
public void initMap() {
Map<String, Object> map = new HashMap<String, Object>();
map.put("abc", this.getConfig().getGlobalConfig().getAuthor() + "-rb");
this.setMap(map);
}
};
List<FileOutConfig> focList = new ArrayList<FileOutConfig>();
//controller的输出配置
focList.add(new FileOutConfig("/templates/controller.java.vm") {
@Override
public String outputFile(TableInfo tableInfo) {
//合并好的内容输出到哪儿?
return rb.getString("OutputDir")+ "/club/xler/web/controller/" + tableInfo.getEntityName() + "Controller.java";
}
});
//query的输出配置
focList.add(new FileOutConfig("/templates/query.java.vm") {
@Override
public String outputFile(TableInfo tableInfo) {
return rb.getString("OutputDirBase")+ "/club/xler/query/" + tableInfo.getEntityName() + "Query.java";
}
});
// 调整 domain 生成目录演示
focList.add(new FileOutConfig("/templates/entity.java.vm") {
@Override
public String outputFile(TableInfo tableInfo) {
return rb.getString("OutputDirBase")+ "/club/xler/entity/" + tableInfo.getEntityName() + ".java";
}
});
// 调整 xml 生成目录演示
focList.add(new FileOutConfig("/templates/mapper.xml.vm") {
@Override
public String outputFile(TableInfo tableInfo) {
return rb.getString("OutputDirXml")+ "/club/xler/mapper/" + tableInfo.getEntityName() + "Mapper.xml";
}
});
cfg.setFileOutConfigList(focList);
mpg.setCfg(cfg);
// 自定义模板配置,可以 copy 源码 mybatis-plus/src/main/resources/templates 下面内容修改,
// 放置自己项目的 src/main/resources/templates 目录下, 默认名称一下可以不配置,也可以自定义模板名称
TemplateConfig tc = new TemplateConfig();
tc.setService("/templates/service.java.vm");
tc.setServiceImpl("/templates/serviceImpl.java.vm");
tc.setMapper("/templates/mapper.java.vm");
tc.setEntity(null);
tc.setController(null);
tc.setXml(null);
// 如上任何一个模块如果设置 空 OR Null 将不生成该模块。
mpg.setTemplate(tc);
// 执行生成
mpg.execute();
}
}
resource下面
mybatiesplus-config-wx.properties
#代码输出基本路径 就是创建的项目在本地的路径,
OutputDir=D:/xler/xler/xler-service/xler-service-wx/src/main/java
#mapper.xml SQL映射文件目录
OutputDirXml=D:/xler/xler/xler-service/xler-service-wx/src/main/resources
#domain的输出路径
OutputDirBase=D:/xler/xler/xler-pojo/xler-pojo-wx/src/main/java
#设置作者
author=xler
#自定义包路径:基础包的路径 ex:D:/xler/xler/xler-pojo/xler-pojo-wx/src/main/java/club/xler
parent=club.xler
#数据库连接信息
jdbc.driver=com.mysql.jdbc.Driver
jdbc.url=jdbc:mysql:///xler-wx?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
jdbc.user=root
jdbc.pwd=xler010817
templates【自定义代码模板,可以用自带的】
entity.java.vm
package ${package.Entity};
#foreach($pkg in ${table.importPackages})
import ${pkg};
#end
#if(${entityLombokModel})
import com.baomidou.mybatisplus.annotations.Version;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.experimental.Accessors;
#end
/**
* <p>
* $!{table.comment}
* </p>
*
* @author xler
* @since ${date}
*/
#if(${entityLombokModel})
@Data
#if(${superEntityClass})
@EqualsAndHashCode(callSuper = true)
#end
@Accessors(chain = true)
#end
#if(${table.convert})
@TableName("${table.name}")
#end
###if(${superEntityClass})
##public class ${entity} extends ${superEntityClass}#if(${activeRecord})<${entity}>#end {
###elseif(${activeRecord})
##public class ${entity} extends Model<${entity}> {
###else
public class ${entity} implements Serializable {
###end
private static final long serialVersionUID = 1L;
## ---------- BEGIN 字段循环遍历 ----------
#foreach($field in ${table.fields})
#if(${field.keyFlag})
#set($keyPropertyName=${field.propertyName})
#end
###if("$!field.comment" != "")
/**
* ${field.comment}
*/
###end
#if(${field.keyFlag})
## 主键
#if(${field.keyIdentityFlag})
@TableId(value = "${field.name}", type = IdType.AUTO)
#elseif(!$null.isNull(${idType}) && "$!idType" != "")
@TableId(value = "${field.name}", type = IdType.${idType})
#elseif(${field.convert})
@TableId("${field.name}")
#end
## 普通字段
#elseif(${field.fill})
## ----- 存在字段填充设置 -----
#if(${field.convert})
@TableField(value = "${field.name}", fill = FieldFill.${field.fill})
#else
@TableField(fill = FieldFill.${field.fill})
#end
#elseif(${field.convert})
@TableField("${field.name}")
#elseif(!${field.convert} && ${field.name} != "id")
@TableField("${field.name}")
#elseif(!${field.convert} && ${field.name} == "id")
@TableId(value = "${field.name}", type = IdType.NONE#* -没有 AUTO -自增*#)
#end
## 乐观锁注解
#if(${versionFieldName}==${field.name})
@Version
#end
## 逻辑删除注解
#if(${logicDeleteFieldName}==${field.name})
@TableLogic
#end
private ${field.propertyType} ${field.propertyName};
#end
## ---------- END 字段循环遍历 ----------
#if(!${entityLombokModel})
#foreach($field in ${table.fields})
#if(${field.propertyType.equals("boolean")})
#set($getprefix="is")
#else
#set($getprefix="get")
#end
public ${field.propertyType} ${getprefix}${field.capitalName}() {
return ${field.propertyName};
}
#if(${entityBuilderModel})
public ${entity} set${field.capitalName}(${field.propertyType} ${field.propertyName}) {
#else
public void set${field.capitalName}(${field.propertyType} ${field.propertyName}) {
#end
this.${field.propertyName} = ${field.propertyName};
#if(${entityBuilderModel})
return this;
#end
}
#end
#end
#if(${entityColumnConstant})
#foreach($field in ${table.fields})
public static final String ${field.name.toUpperCase()} = "${field.name}";
#end
#end
###if(${activeRecord})
## @Override
## protected Serializable pkVal() {
###if(${keyPropertyName})
## return this.${keyPropertyName};
###else
## return this.id;
###end
## }
##
###end
#if(!${entityLombokModel})
@Override
public String toString() {
return "${entity}{" +
#foreach($field in ${table.fields})
#if($!{velocityCount}==1)
"${field.propertyName}=" + ${field.propertyName} +
#else
", ${field.propertyName}=" + ${field.propertyName} +
#end
#end
"}";
}
#end
}
效果:
数据层:
更多推荐
所有评论(0)