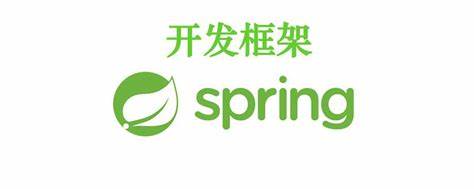
Vue + SpringBoot + MultipartFile 实现文件上传
前端使用 Vue 官方组件进行文件上传^_^
·
前端使用 Vue 官方组件进行文件上传:
<el-upload
ref="upload"
class="avatar-uploader"
action="/api/uploadfile/up"
:multiple="true"
:headers="headers"
:auto-upload="false"
:file-list="fileList"
:on-preview="handlePreview"
:before-upload="beforeAvatarUpload"
>
<el-button slot="trigger" size="small" type="primary"
>选取文件</el-button
>
<el-button
style="margin-left: 10px"
size="small"
type="success"
@click="submitUpload"
>上传到服务器</el-button
>
</el-upload>
后端使用的是 Spring MultipartFile 来接收上传之后的文件内容。MultipartFilehttps://docs.spring.io/spring-framework/docs/current/javadoc-api/org/springframework/web/multipart/MultipartFile.html
如 MultipartFile 官方文档所说:
The file contents are either stored in memory or temporarily on disk. In either case, the user is responsible for copying file contents to a session-level or persistent store as and if desired. The temporary storage will be cleared at the end of request processing.
文件内容要么存储在内存中,要么暂时存储在磁盘上。在任一情况下,用户都负责根据需要将文件内容复制到会话级或持久性存储。临时存储将在请求处理结束时清除。
所以这是要求我们将临时文件转存到固定目录:
void transferTo(File dest)
throws IOException,
IllegalStateException
Transfer the received file to the given destination file.
default void transferTo(Path dest)
throws IOException,
IllegalStateException
Transfer the received file to the given destination file.
The default implementation simply copies the file input stream.
所以我的实现是:
package org.democracy.rest.controller;
import com.alibaba.fastjson.JSONObject;
import org.democracy.commom.base.AjaxResult;
import org.democracy.dao.entity.UploadFile;
import org.democracy.service.UploadFileService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import java.io.File;
import java.io.IOException;
import java.util.List;
@RestController
@RequestMapping("uploadfile")
public class UploadFileController {
private static final Logger logger = LoggerFactory.getLogger(UploadFileController.class);
@Autowired
private UploadFileService uploadFileService;
@Value(value = "${app.file.directory}")
private String fileBaseDirectory;
@PostMapping("up")
public AjaxResult uploadFiles(HttpServletRequest request, MultipartFile[] file) throws IOException {
if (file != null && file.length > 0) {
for (int i=0;i < file.length; i++) {
MultipartFile multipartFile = file[i];
if (!multipartFile.isEmpty()){
String fileName = multipartFile.getOriginalFilename();
File tmpFile = new File(fileBaseDirectory, fileName);
if (!tmpFile.getParentFile().exists()) {
tmpFile.getParentFile().mkdir();
}
multipartFile.transferTo(new File(fileBaseDirectory + File.separator + fileName));
}
}
}
return AjaxResult.success("123");
}
}
至此完成文件上传功能!
更多推荐
所有评论(0)