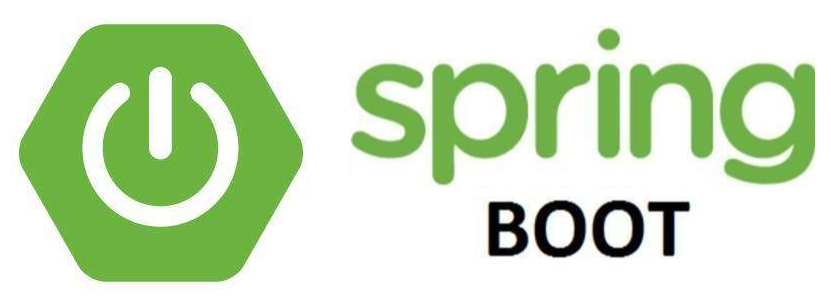
SpringBoot项目中读取.yml文件
SpringBoot项目中读取.yml文件
·
如果同时存在application.yml与application.yaml会优先读取application.yml。
application.yml文件
num: 111
str: 111
other:
dns: students.xin
schoolmate:
- 小明
- 小红
- 小张
方法一,注解的方式读取application.yml文件的内容
@RestController
@RequestMapping("/books")
public class BooksController {
@Value("${num}")
Integer num;
@Value("${str}")
String str;
@Value("${other.dns}")
String dns;
@Value("${schoolmate[0]}")
String index_1;
@RequestMapping("/xin")
public String getById(@RequestParam("id") Integer id) {
System.out.println(num + "--------" + str + "----------" + dns + "-----------" + index_1);
System.out.println("id==" + id);
return "hello spring boot";
}
}
方法二、使用工具类的方式读取application.yml文件
@RestController
@RequestMapping("/books")
public class BooksController {
@Autowired
Environment environment;
@RequestMapping("/xin")
public String getById(@RequestParam("id") Integer id) {
System.out.println(environment.getProperty("num"));
System.out.println(environment.getProperty("other.dns"));
System.out.println(environment.getProperty("schoolmate[0]"));
System.out.println("id==" + id);
return "hello spring boot";
}
}
用类去读取对应的属性
application.yml文件
object:
name: xin
age: 111
phone: 110
subject:
- 数学
- 英语
- 语文
创建对应实体类,加上@Component和@ConfigurationProperties注解后application.yml数据会自动写入到实体类中(前提是类是从Spring中获取的)。
@Component
@ConfigurationProperties(prefix = "student")
public class Student {
String name;
Integer age;
String phone;
String[] subject;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String[] getSubject() {
return subject;
}
public void setSubject(String[] subject) {
this.subject = subject;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
", phone='" + phone + '\'' +
", subject=" + Arrays.toString(subject) +
'}';
}
}
打印
@RestController
@RequestMapping("/books")
public class BooksController {
@Autowired
Student student;
@RequestMapping("/xin")
public String getById() {
System.out.println("------------" + student);
return "hello spring boot";
}
}
更多推荐
所有评论(0)