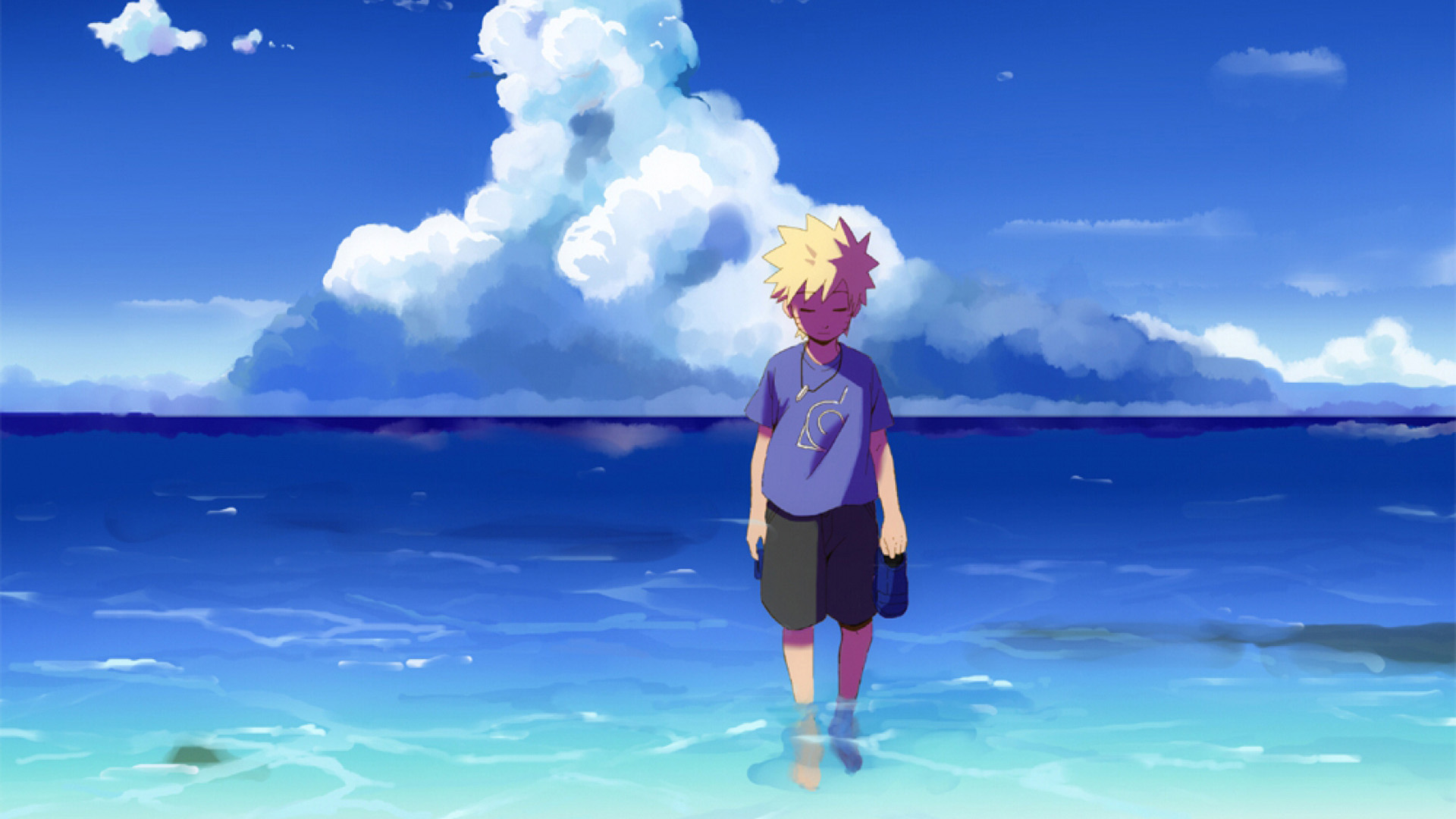
springboot-Echarts-动态饼状图、折线图、柱状图——代码实现-实例说明
1. 调用 Service 层查询所有 x、y 数据的方法,查出的数据封装到 List 表单对象 pojos 里面去;写好Dao层、Service层、Mapper层的代码 -> 就是查询出数据库 pojo 表中中所有的 x、y 的数据;连接好数据库 mysql(其他数据库也可),建一张表 pojo ,里面放两个字段 x、y;2. 返回该对象 pojos。3. 我的 controller 如下所示
·
springboot-Echarts-动态饼状图、折线图、柱状图——代码实现-实例说明
我用的工具是 idea ->
第一步:连接好数据库 mysql(其他数据库也可),建一张表 pojo ,里面放两个字段 x、y;
第二步:写好一个pojo实体类,我的实体类如下所示 ->
@Component
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Pojo {
private x;
private y;
}
第三步:写好Dao层、Service层、Mapper层的代码 -> 就是查询出数据库 pojo 表中中所有的 x、y 的数据;
第四步:写好一个 controller 控制层
1. 调用 Service 层查询所有 x、y 数据的方法,查出的数据封装到 List 表单对象 pojos 里面去;
2. 返回该对象 pojos
3. 我的 controller 如下所示 ->
@Controller
public class MyController {
@Autowired
private PojoService pojoService
//饼状图的数据查询
@ResponseBody
@RequestMapping("/pojos_bing")
public List<Pojo> gotoIndex() {
List<Pojo> pojos = pojoService.selectAllPojos();
return pojos;
}
}
接下来html 代码 java script 里面的代码有可能会报有 红色波浪,但是我这里没关系,仍然不影响运行;
第五步:写好前端 index.html 代码,我这里用的是 thymeleaf 模板引擎 -> 饼状图
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>index</title>
</head>
<body>
<!--饼状图-->
<div id="pie" style="width:800px;height:600px;"></div>
<script th:src="@{https://cdn.bootcdn.net/ajax/libs/echarts/5.4.0/echarts.min.js}"></script>
<script>
option = {
title: {
text:'饼图示例',
subtext:'纯属虚构',
left:'center'
},
legend: {
top: 'bottom'
},
tooltip:{
trigger:'item'
},
toolbox: {
show: true,
feature: {
mark: { show: true },
dataView: { show: true, readOnly: false },
restore: { show: true },
saveAsImage: { show: true }
}
},
series: [
{
name: 'Nightingale Chart',
type: 'pie',
radius: [50, 250],
center: ['50%', '50%'],
roseType: 'area',
itemStyle: {
borderRadius: 8
},
data: [
]
}
]
};
var chartDom = document.getElementById('pie');
var myChart = echarts.init(chartDom);
//######################################################################################
//#######################从上面到这里的代码全部复制粘贴即可###############################
//######################################################################################
fetch("/pojos_bing").then(response => response.json()).then(res => { //这里的 /pojos_bing 就是换成自己刚刚Controller层写好的查询数据的接口
res.forEach(item => {
option.series[0].data.push({name: item.x,value: item.y}) //这里遍历x和y就是数据库数据
})
myChart.setOption(option);
})
</script>
</body>
</html>
第五步:写好前端 index.html 代码,我这里用的是 thymeleaf 模板引擎 -> 线性图
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>index</title>
</head>
<body>
<script th:src="@{https://cdn.bootcdn.net/ajax/libs/echarts/5.4.0/echarts.min.js}"></script>
<!--线形图-->
<div style="height: 50px;"></div>
<div id="line" style="width: 1000px;height: 800px;"></div>
<script>
lineOption = {
title: {
text: '折线图堆叠'
},
legend: {
top: 'top'
},
tooltip: {
trigger: 'axis'
},
xAxis: {
type: 'category',
data: []
},
yAxis: {
type: 'value'
},
series: [
{
name: '11',
data: [],
type: 'line'
},
{
name: '22',
data: [],
type: 'line'
}
]
};
var lineDom = document.getElementById('line');
var lineChart = echarts.init(lineDom);
//######################################################################################
//#######################从上面到这里的代码全部复制粘贴即可###############################
//######################################################################################
fetch("/pojos_bing").then(response => response.json()).then(res => {//这里的 /pojos_bing 就是换成自己刚刚Controller层写好的查询数据的接口
const x= res.map(v => v.x);//这里拿到数据库中的 x
lineOption.xAxis.data = x
const y= res.map(v => v.y);//这里拿到数据库中的 y
lineOption.series[0].data = y
lineChart.setOption(lineOption)
})
</script>
</body>
</html>
第五步:写好前端 index.html 代码,我这里用的是 thymeleaf 模板引擎 -> 柱状图
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>index</title>
</head>
<body>
<script th:src="@{https://cdn.bootcdn.net/ajax/libs/echarts/5.4.0/echarts.min.js}"></script>
<!--柱状图-->
<div style="height: 50px;"></div>
<div id="bar" style="width: 1000px;height: 800px;"></div>
<script>
barOption = {
title: {
text: '柱状图'
},
legend: {
top: 'top'
},
tooltip: {
trigger: 'axis'
},
xAxis: {
type: 'category',
data: []
},
yAxis: {
type: 'value'
},
series: [
{
name: '11',
data: [],
type: 'bar'
},
{
name: '22',
data: [],
type: 'bar'
}
]
};
var barDom = document.getElementById('bar');
var barChart = echarts.init(barDom);
//######################################################################################
//#######################从上面到这里的代码全部复制粘贴即可###############################
//######################################################################################
fetch("/pojos_bing").then(response => response.json()).then(res => {//这里的 /pojos_bing 就是换成自己刚刚Controller层写好的查询数据的接口
const x= res.map(v => v.x);//这里拿到数据库中的 x
barOption.xAxis.data = x
const y= res.map(v => v.y);//这里拿到数据库中的 y
barOption.series[0].data = y
barChart.setOption(barOption)
})
</script>
</body>
</html>
更多推荐
所有评论(0)