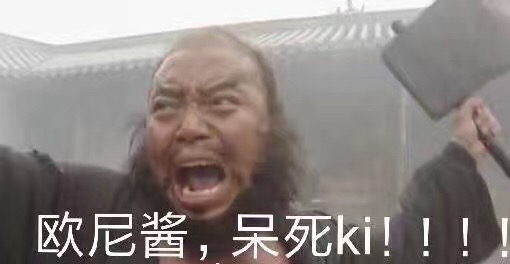
使用springboot创建javafx的几种方式
引言springboot开发项目现在是java开发的主流,springboot也集成了很多功能,如aop和表单检测,比常规的单体Javafx项目或者maven项目要好用多,springboot开发Javafx项目主要有两种方式,一种是直接继承实现CommandLineRunner,还有一种是实现AbstractJavaFxApplicationSupport,本文将详细说明。1,CommandLi
引言
springboot开发项目现在是java开发的主流,springboot也集成了很多功能,如aop和表单检测,比常规的单体Javafx项目或者maven项目要好用多,springboot开发Javafx项目主要有两种方式,一种是直接继承实现CommandLineRunner,还有一种是实现AbstractJavaFxApplicationSupport,本文将详细说明。
1,CommandLineRunner
在使用SpringBoot构建项目时,我们通常有一些预先数据的加载。那么SpringBoot提供了一个简单的方式来实现–CommandLineRunner。
在主调用接口实现这个接口,在接口方法中启动javafx主类窗口,这样好处是最小程度侵入,适合将原javafx项目直接移植到springboot,在此基础上使用springboot的相关插件,十分方便。
ConsoleApplication.java
import com.yz.sample.MainApp;
import javafx.application.Application;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class ConsoleApplication implements CommandLineRunner {
public static void main(String[] args) {
SpringApplication.run(ConsoleApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
Application.launch(MainApp.class, args);
}
}
MainApp.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.AnchorPane;
import javafx.stage.Stage;
public class MainApp extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
AnchorPane anchorPane=new AnchorPane();
Scene scene=new Scene(anchorPane);
primaryStage.setScene(scene);
primaryStage.show();
}
}
启动springboot,并且打开fx窗口
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.6.6)
2022-04-09 14:35:10.373 INFO 10784 --- [ main] com.yz.ConsoleApplication : Starting ConsoleApplication using Java 17.0.1 on yanzhao with PID 10784 (E:\ideaDownload\AutomatedScriptTextEditor\target\classes started by yanzhao in E:\ideaDownload\AutomatedScriptTextEditor)
2022-04-09 14:35:10.375 INFO 10784 --- [ main] com.yz.ConsoleApplication : No active profile set, falling back to 1 default profile: "default"
2022-04-09 14:35:11.374 INFO 10784 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2022-04-09 14:35:11.374 INFO 10784 --- [ main] o.a.catalina.core.AprLifecycleListener : An older version [1.2.16] of the Apache Tomcat Native library is installed, while Tomcat recommends a minimum version of [1.2.30]
2022-04-09 14:35:11.374 INFO 10784 --- [ main] o.a.catalina.core.AprLifecycleListener : Loaded Apache Tomcat Native library [1.2.16] using APR version [1.6.3].
2022-04-09 14:35:11.374 INFO 10784 --- [ main] o.a.catalina.core.AprLifecycleListener : APR capabilities: IPv6 [true], sendfile [true], accept filters [false], random [true], UDS [false].
2022-04-09 14:35:11.374 INFO 10784 --- [ main] o.a.catalina.core.AprLifecycleListener : APR/OpenSSL configuration: useAprConnector [false], useOpenSSL [true]
2022-04-09 14:35:12.411 INFO 10784 --- [ main] o.a.catalina.core.AprLifecycleListener : OpenSSL successfully initialized [OpenSSL 1.0.2m 2 Nov 2017]
2022-04-09 14:35:12.416 INFO 10784 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2022-04-09 14:35:12.416 INFO 10784 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.60]
2022-04-09 14:35:12.498 INFO 10784 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2022-04-09 14:35:12.498 INFO 10784 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 1970 ms
2022-04-09 14:35:13.127 INFO 10784 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2022-04-09 14:35:13.134 INFO 10784 --- [ main] com.yz.ConsoleApplication : Started ConsoleApplication in 2.957 seconds (JVM running for 3.359)
2022-04-09 14:35:13.140 WARN 10784 --- [JavaFX-Launcher] javafx : Unsupported JavaFX configuration: classes were loaded from 'unnamed module @6e20b53a'
Process finished with exit code 130
2,继承实现 AbstractJavaFxApplicationSupport
springboot-javafx-support将Spring Boot与JavaFx 8链接在一起。让您所有的视图和控制器类都成为Spring Bean,并利用Spring Universe中的所有功能。
jar包集成
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>de.roskenet</groupId>
<artifactId>springboot-javafx-support</artifactId>
<version>2.1.6</version>
</dependency>
<dependency>
<groupId>de.roskenet</groupId>
<artifactId>springboot-javafx-test</artifactId>
<version>1.3.0</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
spring-boot-starter和spring-boot-starter-test是springboot需要的,springboot-javafx-support和springboot-javafx-test是javafx整合springboot所需要的,其中test是测试需要的包。
AbstractJavaFxApplicationSupport是springboot-javafx-support的核心类,继承了javaFX的Application类,主类是需要继承AbstractJavaFxApplicationSupport这个类型,添加main方法和SpringBootApplication注解。使用launch方法代替SpringApplication.run
关于springboot-javafx-support 资料很多,这里就不再赘述直接放链接了,
avaFX之springboot-javafx-support解析
.
·
·
·
我是离离原上草,要是有用就请一键三连吧。
更多推荐
所有评论(0)