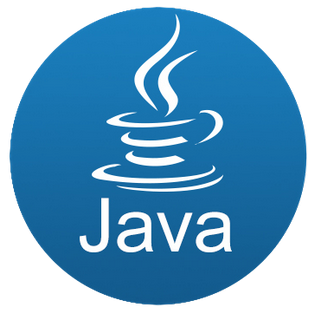
Vue前后端交互(整合springboot)
Axios 是一个基于 promise 的 HTTP 库,可以用在浏览器和 node.js 中。这些是创建请求时可以用的配置选项。某个请求的响应包含以下信息。
·
一、Axios的使用
1、简介
Axios 是一个基于 promise 的 HTTP 库,可以用在浏览器和 node.js 中。
2、请求配置
这些是创建请求时可以用的配置选项。只有 url
是必需的。如果没有指定 method
,请求将默认使用 get
方法。
{
// `url` 是用于请求的服务器 URL
url: '/user',
// `method` 是创建请求时使用的方法
method: 'get', // default
// `baseURL` 将自动加在 `url` 前面,除非 `url` 是一个绝对 URL。
// 它可以通过设置一个 `baseURL` 便于为 axios 实例的方法传递相对 URL
baseURL: 'https://some-domain.com/api/',
// `headers` 是即将被发送的自定义请求头
headers: {'X-Requested-With': 'XMLHttpRequest'},
// `params` 是即将与请求一起发送的 URL 参数
// 必须是一个无格式对象(plain object)或 URLSearchParams 对象
params: {
ID: 12345
},
// `data` 是作为请求主体被发送的数据
// 只适用于这些请求方法 'PUT', 'POST', 和 'PATCH'
// 在没有设置 `transformRequest` 时,必须是以下类型之一:
// - string, plain object, ArrayBuffer, ArrayBufferView, URLSearchParams
// - 浏览器专属:FormData, File, Blob
// - Node 专属: Stream
data: {
firstName: 'Fred'
},
// `responseType` 表示服务器响应的数据类型,可以是 'arraybuffer', 'blob', 'document', 'json', 'text', 'stream'
responseType: 'json', // default
// `transformRequest` 允许在向服务器发送前,修改请求数据
// 只能用在 'PUT', 'POST' 和 'PATCH' 这几个请求方法
// 后面数组中的函数必须返回一个字符串,或 ArrayBuffer,或 Stream
transformRequest: [function (data, headers) {
// 对 data 进行任意转换处理
return data;
}],
// `transformResponse` 在传递给 then/catch 前,允许修改响应数据
transformResponse: [function (data) {
// 对 data 进行任意转换处理
return data;
}],
......
}
3、响应结构
某个请求的响应包含以下信息
{
// `data` 由服务器提供的响应
data: {},
// `status` 来自服务器响应的 HTTP 状态码
status: 200,
// `statusText` 来自服务器响应的 HTTP 状态信息
statusText: 'OK',
// `headers` 服务器响应的头
headers: {},
// `config` 是为请求提供的配置信息
config: {},
// 'request'
// `request` is the request that generated this response
// It is the last ClientRequest instance in node.js (in redirects)
// and an XMLHttpRequest instance the browser
request: {}
}
使用 then
时,你将接收下面这样的响应 :
axios.get('/user/12345')
.then(function(response) {
console.log(response.data);
console.log(response.status);
console.log(response.statusText);
console.log(response.headers);
console.log(response.config);
});
4、安装
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
eg:
二、后端代码实现(整合springboot)
1、pom文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.8</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.wouniuxy</groupId>
<artifactId>bootvue</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
<hutool.version>5.4.3</hutool.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.24</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-configuration-processor</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.7</version>
</dependency>
<!--Hutool工具-->
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>${hutool.version}</version>
</dependency>
<!-- thymeleaf依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
2、yml文件
server:
port: 9000
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/store?characterEncoding=utf8&useSSL=false&serverTimezone=UTC
username: root
password: root
type: com.alibaba.druid.pool.DruidDataSource
mybatis:
typeAliasesPackage: com.woniuxy.bootvue.entity
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
lazy-loading-enabled: true
aggressive-lazy-loading: false
mapper-locations: classpath:mapper/*.xml
3、建立实体类、mapper.xml、controller(restful风格)等层
三、Vue前端代码实现
1、js依赖引入
<link rel="stylesheet" type="text/css" href="css/bootstrap.min.css">
<script type="text/javascript" src="js/jquery-3.2.1.min.js"> </script>
<script type="text/javascript" src="js/bootstrap.min.js"></script>
<script type="text/javascript" src="js/vue.js"></script>
<script type="text/javascript" src="js/axios.min.js"></script>
<script src="https://unpkg.com/dayjs@1.8.21/dayjs.min.js"></script>
2、html页面部分
<div id="app">
<div class="container" style="padding-top: 40px;">
<div class="form-group">
<div class="row">
<div class="col-md-8">
<input type="text" class="form-control swich" v-model="userName" />
</div>
<div class="col-md-3">
<button class="btn btn-danger sreach" @click="loadUser">搜索</button>
<button class="btn btn-default add" data-toggle="modal" data-target="#addModel">增加</button>
</div>
</div>
</div>
<table class="table table-bordered text-center">
<tr>
<td>编号</td>
<td>姓名</td>
<td>年龄</td>
<td>部门</td>
<td>创建时间</td>
<td>操作</td>
</tr>
<tr v-for="(item, index) in tableData" :key="index">
<td>{{item.id}}</td>
<td>{{item.userName}}</td>
<td>{{item.age}}</td>
<td>{{item.deptName}}</td>
<td>{{item.createDate|dateFormat}}</td>
<td>
<button class="btn btn-primary rev" data-toggle="modal" data-target="#myModal"
@click="edit(item.id)">修改</button>
<button class="btn btn-danger del" @click="del(item.id)">删除</button>
</td>
</tr>
</table>
</div>
<!-- //修改的模态框 -->
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel"
aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<form>
<div class="form-group">
<select class="form-select" v-model="updateForm.deptId">
<option v-for="(item, index) in deptList" :key="index" :value="item.deptId">
{{item.deptName}}</option>
</select>
</div>
<div class="form-group">
<input type="text" placeholder="名字" class="form-control"
v-model="updateForm.userName" />
</div>
<div class="form-group">
<input type="text" placeholder="年龄" class="form-control" v-model="updateForm.age" />
</div>
</form>
</div>
<div class="modal-footer">
<input type="hidden" v-model="updateForm.id" />
<button type="button" class="btn btn-default" data-dismiss="modal">关闭</button>
<button type="button" class="btn btn-primary olk" data-dismiss="modal"
@click="update()">提交更改</button>
</div>
</div>
<!-- /.modal-content -->
</div>
<!-- /.modal -->
</div>
<!-- //增加的模态框 -->
<div class="modal fade" id="addModel" tabindex="-1" role="dialog" aria-labelledby="myModalLabel"
aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<form>
<div class="form-group">
<select class="form-select" v-model="addForm.deptId">
<option v-for="(item, index) in deptList" :key="index" :value="item.deptId">
{{item.deptName}}</option>
</select>
</div>
<div class="form-group">
<input type="text" placeholder="名字" class="form-control" v-model="addForm.userName" />
</div>
<div class="form-group">
<input type="text" placeholder="年龄" class="form-control" v-model="addForm.age" />
</div>
</form>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">关闭</button>
<button type="button" class="btn btn-primary aad" data-dismiss="modal"
@click="save()">增加信息</button>
</div>
</div>
<!-- /.modal-content -->
</div>
<!-- /.modal -->
</div>
</div>
3、js操作部分
<script type="text/javascript">
Vue.filter('dateFormat', function (value) {
return dayjs(value).format('YYYY-MM-DD');
})
var vm = new Vue({
el: "#app",
data: {
tableData: [],
deptList: [],
addForm: {
userName: "",
age: "",
deptId: ""
},
updateForm: {
id: "",
userName: "",
age: "",
deptId: ""
},
userName: ""
},
methods: {
loadUser() {
axios.get("http://localhost:9000/emp/", {
params: {
userName: this.userName
}
})
.then(res => {
if (res.data.code == 200) {
this.tableData = res.data.data.userList;
}
})
},
loadDept() {
axios.get("http://localhost:9000/dept/")
.then(res => {
if (res.data.code == 200) {
this.deptList = res.data.data.deptList;
}
})
},
edit(id) {
axios.get("http://localhost:9000/emp/" + id)
.then(res => {
if (res.data.code == 200) {
this.updateForm = res.data.data.user;
}
})
},
save() {
axios.post("http://localhost:9000/emp/", this.addForm)
.then(res => {
if (res.data.code == 200) {
this.loadUser();
this.addForm = {}
}
})
},
update() {
axios.put("http://localhost:9000/emp/", this.updateForm)
.then(res => {
if (res.data.code == 200) {
this.loadUser();
this.updateForm = {}
}
})
},
del(id) {
axios.delete("http://localhost:9000/emp/" + id)
.then(res => {
if (res.data.code == 200) {
this.loadUser();
}
})
}
},
mounted() {
this.loadUser();
this.loadDept();
},
})
</script>
四、前后端分离跨域问题
方案:全局配置的方式
package com.wn.rbac.config;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
/**
* @author :fengSir
* @date :Created By 2022-05-06 22:08
* @description :TODO
*/
//@EnableWebMvc
@Configuration
public class MvcConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
//每次调用registry.addMapping可以添加一个跨域配置,需要多个配置可以多次调用registry.addMapping
registry.addMapping("/**")
.allowedOrigins("*") //放行哪些原始域
.allowedMethods("PUT", "DELETE","POST", "GET") //放行哪些请求方式
.allowedHeaders("*") //放行哪些原始请求头部信息
//.exposedHeaders("header1", "header2") //暴露哪些头部信息
.allowCredentials(false) //是否发送 Cookie
.maxAge(3600);
// Add more mappings...
}
}
更多推荐
所有评论(0)