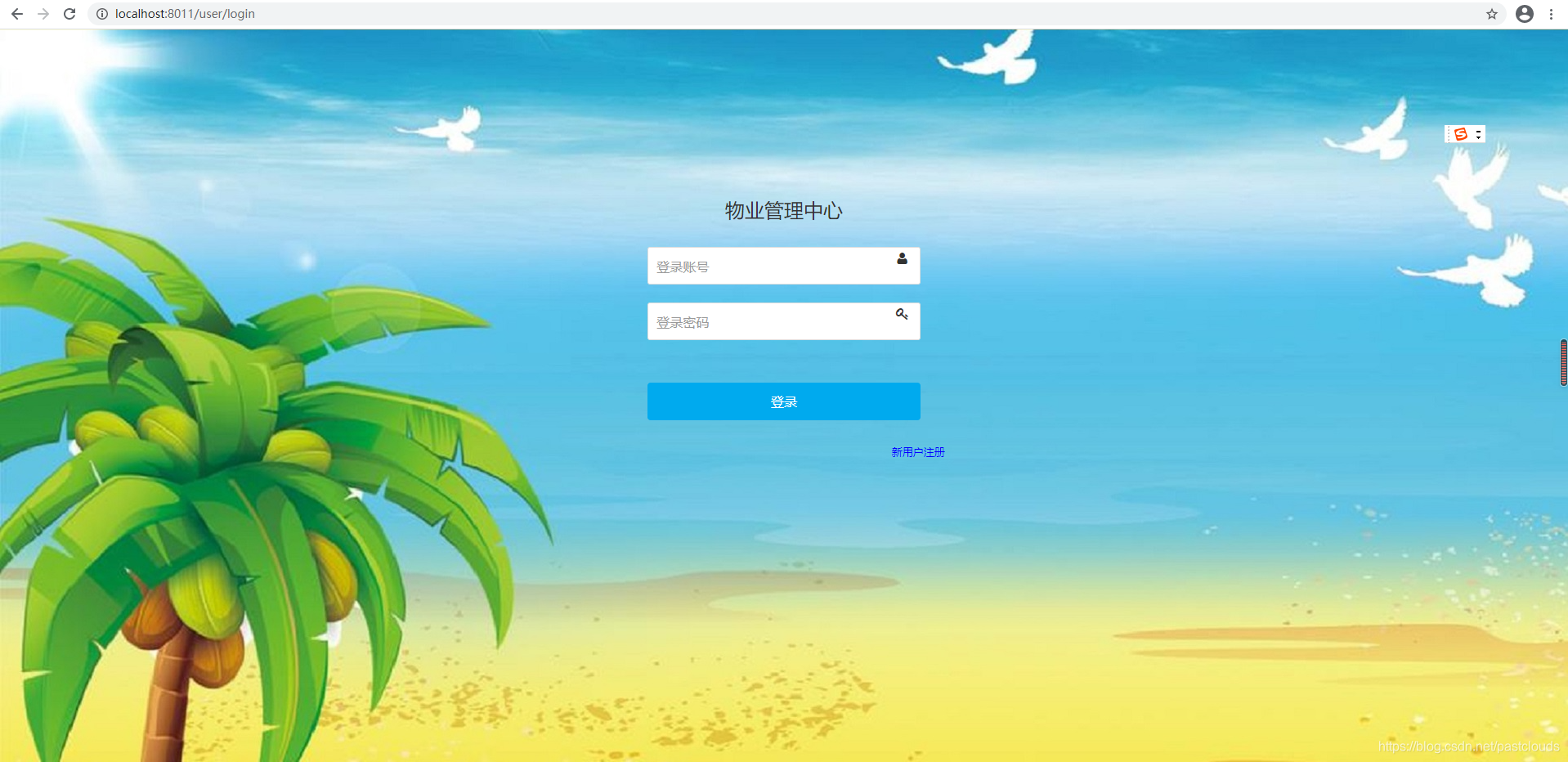
Java项目:小区物业管理系统(java+Springboot+ssm+mysql+maven+jsp)
一、项目简述功能:分为管理员及普通业主角色,业主信息,社区房 屋,维护管理,社区车辆,停车场管理,社区投诉,社区 缴费,社区业务信息维护等等功能。二、项目运行环境配置: Jdk1.8 + Tomcat8.5 + mysql + Eclispe (IntelliJ IDEA,Eclispe,MyEclispe,Sts 都支持)项目技术: JSP +SpringBoot + MyBatis + htm
·
源码获取:博客首页 "资源" 里下载!
一、项目简述
功能:分为管理员及普通业主角色,业主信息,社区房 屋,维护管理,社区车辆,停车场管理,社区投诉,社区 缴费,社区业务信息维护等等功能。
二、项目运行
环境配置: Jdk1.8 + Tomcat8.5 + mysql + Eclispe (IntelliJ IDEA,Eclispe,MyEclispe,Sts 都支持)
项目技术: JSP +SpringBoot + MyBatis + html+ css + JavaScript + JQuery + Ajax + Fileupload + maven等等。
装修控制器:
/**
* @category 装修控制器
*
*/
@Controller
public class AdornController {
@Autowired
AdornService adornService;
/**
* 查找所有
*/
@RequestMapping("/adornall")
public String adornAll(Model model) {
List<Adorn> list = adornService.findAll();
model.addAttribute("adornlist", list);
return "adorn";
}
/**
* 按类型删除
*/
@RequestMapping("/findbystate")
public String findByState(Model model, String state) {
List<Adorn> list = adornService.findByState(state);
model.addAttribute("adornlist", list);
return "adorn";
}
}
绿化的控制器:
/**
*
* @category 绿化的控制器
*/
@Controller
public class AfforestController {
@Autowired
AfforestService afforestService;
/**
* 查看所有绿化信息
*/
@RequestMapping("/afforestact1")
public String afforestact(Model model) {
List<Afforest>list=afforestService.selectByExample();
model.addAttribute("aflist", list);
return "showafforest";
}
/**
* 查看所有绿化信息
*/
@RequestMapping("/afforestact2")
public String afforestact2(Model model,String afplush) {
List<Afforest>list=afforestService.findAfplushList(afplush);
model.addAttribute("aflist", list);
return "showafforest2";
}
/**
* 保存
*/
@RequestMapping("/saveafforest")
public String save(Afforest afforest,Model model) {
afforestService.updatesave4(afforest);
return "redirect:afforestact1.action";
}
/**
* 根据ID查询所有内容
*/
@RequestMapping("/findAfforestByid")
public String find(Model model,int afid) {
Afforest afforest=new Afforest();
afforest=afforestService.findAfforestById(afid);
model.addAttribute("aflist", afforest);
return "showafforest";
}
/**
* 点击更改完成状态
*/
@RequestMapping("/updapushaf")
public String updapushaf(Afforest afforest) {
afforestService.updaaffomplish(afforest);
return "redirect:afforestact1.action";
}
/**
* 添加绿化任务
* @param maintain
* @return
*/
@RequestMapping("/addafforest")
public String addafforest(Afforest afforest) {
boolean flag = afforestService.insert(afforest);
if (flag == true) {
// 使用重定向,返回登录界面
return "redirect:afforestact1.action";
} else {
return "css";
}
}
/**
* 删除一个住户
*/
@RequestMapping("/deleteaff")
public String deleteone(int afid) {
afforestService.deleteByPrimaryKey(afid);
return "redirect:afforestact1.action";
}
/**
* 根据id 完成时间 提交时间 模糊查询
* @param model
* @param md
* @return
*/
@RequestMapping("/findaffor")
public String findclean(Model model,String affname) {
System.out.println("+++++++++++++");
System.out.println(affname);
List<Afforest>list3 = afforestService.findMainAfforest(affname);
model.addAttribute("aflist", list3);
return "showafforest";
}
}
房间处理器:
/**
* @category 房间处理器
*
*/
@Controller
public class HouseController {
@Autowired
private HouseNumberService hService;
/**
* @category 通过传入参数展示相应的房屋信息
* @param type
* @param model
* @return
*/
@RequestMapping("/showhouse")
public String showhouse(@RequestParam ("type") String type,Model model) {
System.out.println(type);
HousenumberExample example=new HousenumberExample();
List<Housenumber> list=hService.selectByExample(example);
List<Housenumber> list1=new ArrayList<Housenumber>();
if(type.equals("a")) {
model.addAttribute("list", list);
model.addAttribute("can", "a");
return "house";
}
else if(type.equals("x")) {
for (int i = 0; i < list.size(); i++) {
if(list.get(i).getStatus().equals("闲置")) {
list1.add(list.get(i));
}
}
model.addAttribute("can", "x");
model.addAttribute("list", list1);
return "house";
}
else if(type.equals("y")) {
for (int i = 0; i < list.size(); i++) {
if(list.get(i).getStatus().equals("已出售")) {
list1.add(list.get(i));
}
}
model.addAttribute("can", "y");
model.addAttribute("list", list1);
return "house";
}
return "mian";
}
/**
* @category 跳转到更新房屋信息页面
* @param model
* @param homeid
* @return
*/
@RequestMapping("/updatehouse")
public String updatehouse(Model model,@RequestParam ("homeid") int homeid) {
Housenumber house=hService.selectByPrimaryKey(homeid);
model.addAttribute("house", house);
return "updatehouse";
}
/**
* @category 跟新房屋信息
* @param model
* @param house
* @return
*/
@RequestMapping("/updatehouse1")
public String updatehouse1(Model model, Housenumber house) {
String type=house.getStatus();
if(type.equals("闲置")) {
type="x";
}else if(type.equals("已出售")) {
type="y";
}
System.out.println(house.getStatus());
hService.updateByPrimaryKeySelective(house);
model.addAttribute("house", house);
return "redirect:showhouse.action?type="+type;
}
/**
* @category 添加新的闲置房屋
* @param model
* @param house
* @return
*/
@RequestMapping("/addhouse")
public String addhouse(Model model,Housenumber house) {
house.setSaleprice(0);
hService.insert(house);
return "redirect:showhouse.action?type=a";
}
}
源码获取:博客首页 "资源" 里下载!
更多推荐
所有评论(0)