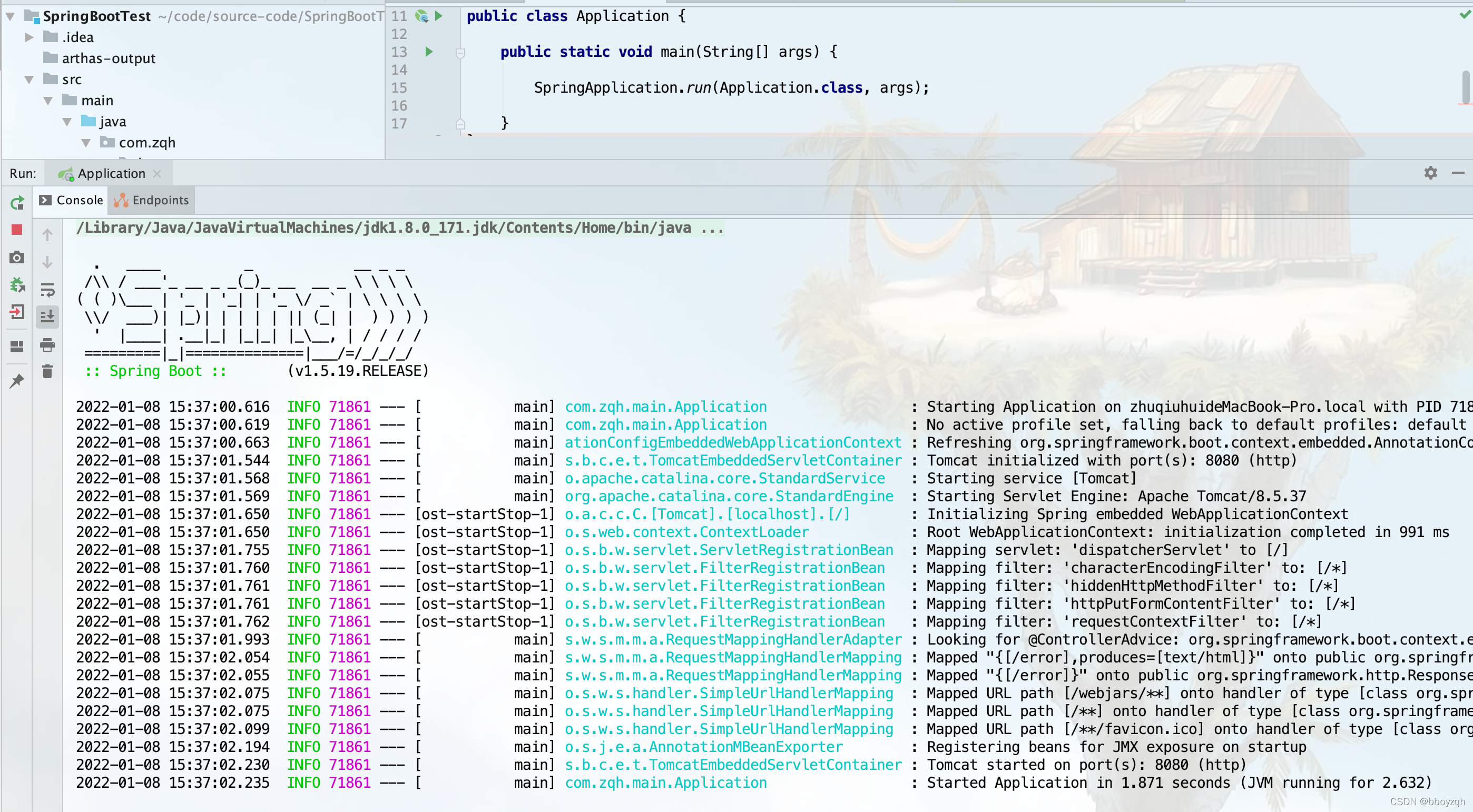
如何使用Arthas查看类变量值
如何使用Arthas查看类变量值
·
使用arthas查看类变量值核心思路是:通过实现ApplicationContextAware接口定义ApplicationUtil类,该类可以获取ApplicationContext的所有的Bean实例,然后通过arthas的ognl查看类实例中的属性值。
搭建简易Spring Boot工程
1. pom依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>zqh.test</groupId>
<artifactId>SpringBootTest</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.19.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
2. Spring Boot 启动类
package com.zqh.main;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
/**
* @author fangchen
* @date 2022-01-08 14:33
*/
@SpringBootApplication(scanBasePackages = {"com.zqh.bean"})
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
3. 实现 ApplicationContextAware 接口的工具类
package com.zqh.bean;
import org.springframework.beans.BeansException;
import org.springframework.context.ApplicationContext;
import org.springframework.context.ApplicationContextAware;
import org.springframework.stereotype.Component;
/**
* @author fangchen
* @date 2022-01-08 14:49
*/
@Component
public class ApplicationUtil implements ApplicationContextAware {
private static ApplicationContext applicationContext;
@Override
public void setApplicationContext(ApplicationContext args) throws BeansException {
applicationContext = args;
}
public static Object getBean(String beanName) {
return applicationContext.getBean(beanName);
}
}
4. 查看目标类
package com.zqh.bean;
import org.springframework.stereotype.Component;
/**
* @author fangchen
* @date 2022-01-08 14:35
*/
@Component("testBean")
public class TestBean {
private int a = 9;
private String str = "this is bboy zqh!";
}
使用Arthas查看类变量值
1. 启动 Spring Boot 中的 main 方法
运行Application类,启动成功日志:
2. 下载并启动arthas
# 下载arthas
curl -O https://arthas.aliyun.com/arthas-boot.jar
# 启动arthas,并找到相应进程,选择,这里是第一个
java -jar arthas-boot.jar
[INFO] Found existing java process, please choose one and input the serial number of the process, eg : 1. Then hit ENTER.
* [1]: 71175 com.zqh.main.Application
[2]: 70851 /Users/zhuqiuhui/.flow/resources/player.jar
[3]: 70850 /Users/zhuqiuhui/.flow/resources/kafka.jar
[4]: 70852 /Users/zhuqiuhui/.flow/resources/webapp.jar
[5]: 71174 org.jetbrains.jps.cmdline.Launcher
[6]: 36375
[7]: 68267 /Users/zhuqiuhui/.flow/resources/kafka.jar
[8]: 70861 org.jetbrains.idea.maven.server.RemoteMavenServer
[9]: 70732
3. 使用arthas查看类变量值
[arthas@71175]$ ognl '@com.zqh.bean.ApplicationUtil@getBean("testBean").a'
@Integer[9]
[arthas@71175]$ ognl '@com.zqh.bean.ApplicationUtil@getBean("testBean").str'
@String[this is bboy zqh!]
欢迎关注微信公众号:方辰的博客
更多推荐
所有评论(0)