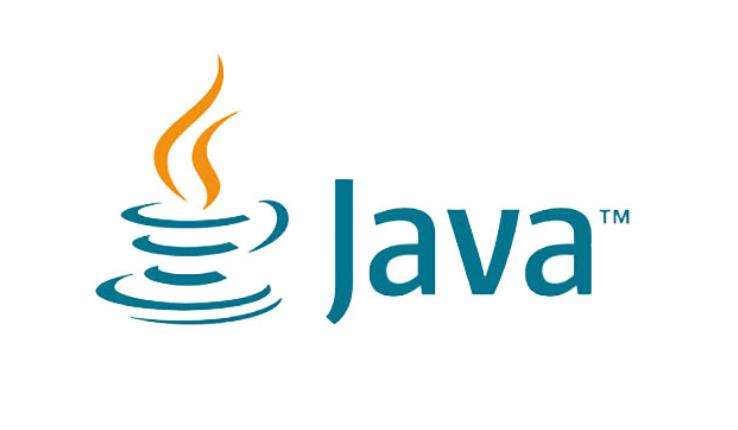
java实现限制接口访问次数
springboot项目下限制莫个接口在规定时间内的访问次数
·
1.添加pox依赖
<!-- AOP -->
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.9.4</version>
</dependency>
<!--Map依赖 -->
<dependency>
<groupId>net.jodah</groupId>
<artifactId>expiringmap</artifactId>
<version>0.5.10</version>
</dependency>
2.创建自定义注解;
@Documented
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface LimitRequest {
//毫秒,分钟,小时 之间的转换用算数
long time() default 60000*60*24; // 限制时间 单位:毫秒
int count() default Integer.MAX_VALUE; // 允许请求的次数
}
3.创建aop切面类:
@Aspect
@Component
public class LimitRequestAspect {
private static ConcurrentHashMap<String, ExpiringMap<String, Integer>> book = new ConcurrentHashMap<>();
// 定义切点
// 让所有有@LimitRequest注解的方法都执行切面方法
@Pointcut("@annotation(limitRequest)")
public void excudeService(LimitRequest limitRequest) {
}
@Around("excudeService(limitRequest)")
public Object doAround(ProceedingJoinPoint pjp, LimitRequest limitRequest) throws Throwable {
// 获得request对象
RequestAttributes ra = RequestContextHolder.getRequestAttributes();
ServletRequestAttributes sra = (ServletRequestAttributes) ra;
HttpServletRequest request = sra.getRequest();
// 获取Map对象, 如果没有则返回默认值
// 第一个参数是key, 第二个参数是默认值
ExpiringMap<String, Integer> map = book.getOrDefault(request.getRequestURI(), ExpiringMap.builder().variableExpiration().build());
Integer uCount = map.getOrDefault(request.getRemoteAddr(), 0);
if (uCount >= limitRequest.count()) { // 超过次数,不执行目标方法
//这里的返回对象类型根据controller方法的返回方式一致
return AjaxResult.error(500,"接口访问次数超过限制,请12小时候重试");
} else if (uCount == 0){ // 第一次请求时,设置开始有效时间
map.put(request.getRemoteAddr(), uCount + 1, ExpirationPolicy.CREATED, limitRequest.time(), TimeUnit.MILLISECONDS);
} else { // 未超过次数, 记录数据加一
map.put(request.getRemoteAddr(), uCount + 1);
}
book.put(request.getRequestURI(), map);
// result的值就是被拦截方法的返回值
Object result = pjp.proceed();
return result;
}
}
3.在需要限制访问的接口方法上添加我们自定义的注解:
@ApiOperation(value = "查询学生全部信息")
//其中count指的是规定时间内的访问次数,表示该接口在我们设置的默认时间内只能访问3次,默认时间在自定义注解里面设置的
@LimitRequest(count=3)
@GetMapping("/findAll")
public R findAll(Student student){
List<Student> students = studentService.findAll(student);
return R.ok().data("students",students);
}
更多推荐
所有评论(0)