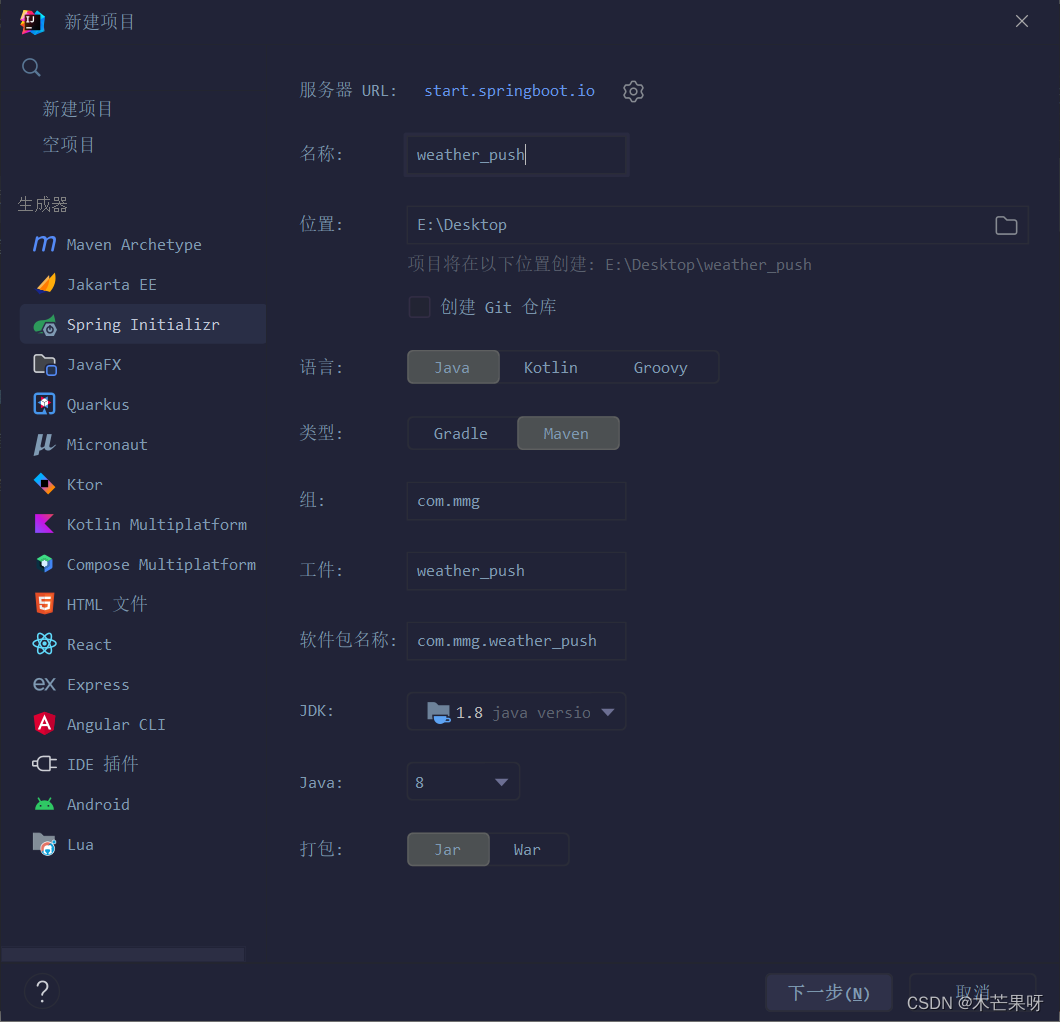
基于SpringBoot实现微信消息推送
SpringBoot微信推送
·
本微信消息推送实现的功能
1.当天指定城市的天气
2.当天指定城市的最低高气温
3.彩虹屁
技术栈
1.SpringBoot2.7.5
2.JDK1.8
实现步骤:
1. 新建一个springBoot项目,选择以下相关依赖
2. 引入相关依赖
<!--fastjson依赖-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>2.0.7</version>
</dependency>
<!--微信公众号依赖-->
<dependency>
<groupId>com.github.binarywang</groupId>
<artifactId>weixin-java-mp</artifactId>
<version>3.3.0</version>
</dependency>
3.启动类
/**
* 启动类
* Author:木芒果
*/
@Slf4j
@SpringBootApplication
public class WeatherPushApplication {
public static void main(String[] args) {
SpringApplication.run(WeatherPushApplication.class, args);
log.info("启动成功!");
}
}
4.配置类
/**
* 配置类
* Author:木芒果
*/
@Component
@ConfigurationProperties("wechat")
public class PushConfigure {
/**
* 微信公众平台的appID
*/
private static String appId;
/**
* 微信公众平台的appSecret
*/
private static String secret;
/**
* 天气查询的城市ID
*/
private static String district_id;
/**
* 应用AK
*/
private static String ak;
/**
* 纪念日
*/
private static String loveDate;
/**
* 生日
*/
private static String birthday;
/**
* 关注公众号的用户ID
*/
private static String userId;
/**
* 模板ID
*/
private static String templateId;
/**
* 天行数据apiKey
*/
private static String rainbowKey;
public static String getAppId() {
return appId;
}
public void setAppId(String appId) {
PushConfigure.appId = appId;
}
public static String getSecret() {
return secret;
}
public void setSecret(String secret) {
PushConfigure.secret = secret;
}
public static String getDistrict_id() {
return district_id;
}
public void setDistrict_id(String district_id) {
PushConfigure.district_id = district_id;
}
public static String getAk() {
return ak;
}
public void setAk(String ak) {
PushConfigure.ak = ak;
}
public static String getLoveDate() {
return loveDate;
}
public void setLoveDate(String loveDate) {
PushConfigure.loveDate = loveDate;
}
public static String getBirthday() {
return birthday;
}
public void setBirthday(String birthday) {
PushConfigure.birthday = birthday;
}
public static String getUserId() {
return userId;
}
public void setUserId(String userId) {
PushConfigure.userId = userId;
}
public static String getTemplateId() {
return templateId;
}
public void setTemplateId(String templateId) {
PushConfigure.templateId = templateId;
}
public static String getRainbowKey() {
return rainbowKey;
}
public void setRainbowKey(String rainbowKey) {
PushConfigure.rainbowKey = rainbowKey;
}
}
5.实体类
/**
* 天气实体类
* Author:木芒果
*/
@Data
public class Weather {
private String text_day;
private String text_night;
// 最高气温
private String high;
// 最低气温
private String low;
// 风力"3-4级"
private String wc_day;
// 风向"东北风"
private String wd_day;
private String wc_night;
private String wd_night;
private String date;
private String week;
// 当前天气
private String text_now;
// 当前温度
private String temp;
// 当前城市
private String city;
}
6.四大工具类
纪念日工具类
/**
* 纪念日工具类
* Author:木芒果
*/
public class MemoryDayUtil {
private static final ThreadLocal<SimpleDateFormat> THREAD_LOCAL = new ThreadLocal<>();
/**
* 获取SimpleDateFormat
*/
private static SimpleDateFormat get() {
SimpleDateFormat sdf = THREAD_LOCAL.get();
if (sdf == null) {
sdf = new SimpleDateFormat("yyyy-MM-dd");
THREAD_LOCAL.set(sdf);
}
return sdf;
}
/**
* 计算两个时间差
*/
public static long getDatePoor(Date endDate, Date nowDate) {
long nd = 1000 * 24 * 60 * 60;
// 获得两个时间的毫秒时间差异
long diff = endDate.getTime() - nowDate.getTime();
// 计算差多少天
long day = diff / nd;
return day;
}
/**
* 计算天数
*/
public static long calculationLianAi(String date) {
SimpleDateFormat simpleDateFormat = get();
Date startDate = new Date();
try {
startDate = simpleDateFormat.parse(date);
} catch (ParseException e) {
e.printStackTrace();
}
return getDatePoor(new Date(), startDate);
}
/**
* 计算生日
*/
public static long calculationBirthday(String birthday) {
SimpleDateFormat simpleDateFormat = get();
Calendar cToday = Calendar.getInstance();
Calendar cBirth = Calendar.getInstance();
Date now = new Date();
try {
now = simpleDateFormat.parse(birthday);
} catch (ParseException e) {
e.printStackTrace();
}
cBirth.setTime(now);
cBirth.set(Calendar.YEAR, cToday.get(Calendar.YEAR));
int days;
if (cBirth.get(Calendar.DAY_OF_YEAR) < cToday.get(Calendar.DAY_OF_YEAR)) {
days = cToday.getActualMaximum(Calendar.DAY_OF_YEAR) - cToday.get(Calendar.DAY_OF_YEAR);
days += cBirth.get(Calendar.DAY_OF_YEAR);
} else {
days = cBirth.get(Calendar.DAY_OF_YEAR) - cToday.get(Calendar.DAY_OF_YEAR);
}
return days;
}
}
天气工具类
/**
* 获取天气数据
* 对接百度天气api 的工具类
* Author:木芒果
*/
@Component
public class WeatherUtil {
public static Weather getWeather() {
RestTemplate restTemplate = new RestTemplate();
Map<String, String> map = new HashMap<>();
map.put("district_id", PushConfigure.getDistrict_id());
map.put("ak", PushConfigure.getAk());
String res = restTemplate.getForObject("https://api.map.baidu.com/weather/v1/?district_id={district_id}&data_type=all&ak={ak}", String.class, map);
JSONObject json = JSONObject.parseObject(res);
if (json == null) {
//接口地址有误或者接口没调通
return null;
}
JSONArray forecasts = json.getJSONObject("result").getJSONArray("forecasts");
List<Weather> weathers = forecasts.toJavaList(Weather.class);
Weather weather = weathers.get(0);
JSONObject now = json.getJSONObject("result").getJSONObject("now");
JSONObject location = json.getJSONObject("result").getJSONObject("location");
weather.setText_now(now.getString("text"));
weather.setTemp(now.getString("temp"));
weather.setCity(location.getString("city"));
return weather;
}
}
彩虹屁工具类
/**
* 彩虹屁接口调用
* 对接天行数据(彩虹屁)api的工具类
* Author:木芒果
*/
public class RainbowUtil {
public static String getRainbow() {
String httpUrl = "http://api.tianapi.com/caihongpi/index?key=" + PushConfigure.getRainbowKey();
BufferedReader reader = null;
String result = null;
StringBuilder stringBuilder = new StringBuilder();
try {
URL url = new URL(httpUrl);
HttpURLConnection connection = (HttpURLConnection) url
.openConnection();
connection.setRequestMethod("GET");
InputStream is = connection.getInputStream();
reader = new BufferedReader(new InputStreamReader(is, "UTF-8"));
String strRead = null;
while ((strRead = reader.readLine()) != null) {
stringBuilder.append(strRead);
stringBuilder.append("\r\n");
}
reader.close();
result = stringBuilder.toString();
} catch (Exception e) {
e.printStackTrace();
}
JSONObject jsonObject = JSONObject.parseObject(result);
if (jsonObject == null) {
return "";
}
JSONArray newslist = jsonObject.getJSONArray("newslist");
return "\n" + newslist.getJSONObject(0).getString("content");
}
}
微信推送工具类
/**
* 推送类
* Author:木芒果
*/
public class PushUtil {
/**
* 消息推送主要业务代码
*/
public static String push() {
//1,配置
WxMpInMemoryConfigStorage wxStorage = new WxMpInMemoryConfigStorage();
wxStorage.setAppId(PushConfigure.getAppId());
wxStorage.setSecret(PushConfigure.getSecret());
WxMpService wxMpService = new WxMpServiceImpl();
wxMpService.setWxMpConfigStorage(wxStorage);
// 推送消息
WxMpTemplateMessage templateMessage = WxMpTemplateMessage.builder()
.toUser(PushConfigure.getUserId())
.templateId(PushConfigure.getTemplateId())
.build();
// 配置你的信息
long loveDays = MemoryDayUtil.calculationLianAi(PushConfigure.getLoveDate());
long birthdays = MemoryDayUtil.calculationBirthday(PushConfigure.getBirthday());
Weather weather = WeatherUtil.getWeather();
if (weather == null) {
templateMessage.addData(new WxMpTemplateData("weather", "***", "#00FFFF"));
} else {
templateMessage.addData(new WxMpTemplateData("date", weather.getDate() + " " + weather.getWeek(), "#00BFFF"));
templateMessage.addData(new WxMpTemplateData("weather", weather.getText_now(), "#00FFFF"));
templateMessage.addData(new WxMpTemplateData("low", weather.getLow() + "", "#173177"));
templateMessage.addData(new WxMpTemplateData("temp", weather.getTemp() + "", "#EE212D"));
templateMessage.addData(new WxMpTemplateData("high", weather.getHigh() + "", "#FF6347"));
templateMessage.addData(new WxMpTemplateData("city", weather.getCity() + "", "#173177"));
}
templateMessage.addData(new WxMpTemplateData("loveDays", loveDays + "", "#FF1493"));
templateMessage.addData(new WxMpTemplateData("birthdays", birthdays + "", "#FFA500"));
String remark = "亲爱的乖乖宝贝,早上好!记得要吃早餐哦,今天也要开心哦 =^_^= ";
if (loveDays % 365 == 0) {
remark = "\n今天是恋爱" + (loveDays / 365) + "周年纪念日!";
}
if (birthdays == 0) {
remark = "\n今天是生日,生日快乐呀!";
}
if (loveDays % 365 == 0 && birthdays == 0) {
remark = "\n今天是生日,也是恋爱" + (loveDays / 365) + "周年纪念日!";
}
templateMessage.addData(new WxMpTemplateData("remark", remark, "#FF1493"));
templateMessage.addData(new WxMpTemplateData("rainbow", RainbowUtil.getRainbow(), "#FF69B4"));
System.out.println(templateMessage.toJson());
try {
wxMpService.getTemplateMsgService().sendTemplateMsg(templateMessage);
} catch (Exception e) {
System.out.println("推送失败:" + e.getMessage());
return "推送失败:" + e.getMessage();
}
return "推送成功!";
}
}
7.定时任务类,自动推送
/**
* 定时任务
* Author:木芒果
*/
@EnableScheduling
@Configuration
public class Task {
// 定时 早8点推送 0秒 0分 8时
//@Scheduled(cron = "0 0 8 * * ?")
// 定时 一个小时推送一次
@Scheduled(cron = "0 0 0/1 * * ?")
public void goodMorning() {
PushUtil.push();
}
}
8.测试控制类,手动推送
/**
* 手动推送
*/
@RestController
public class TestController {
@RequestMapping("test")
public String test() {
return PushUtil.push();
}
}
9.修改配置文件application.properties
#测试号信息中的appID
wechat.appId=
#测试号信息中的appsecret
wechat.secret=
#用户列表中的微信号id
wechat.userId=
#模板消息接口中模板ID
wechat.templateId=
#你查询天气城市的邮编
wechat.district_id=430300
#百度地图开放平台的AK
wechat.ak=
#在一起的时间
wechat.loveDate=2022-01-01
#生日
wechat.birthday=2003-12-05
#彩虹屁平台apiKey
wechat.rainbowKey=
10.申请对应接口平台的权限
微信公众号平台接口测试平台
微信公众平台接口测试账号申请:微信公众平台
申请登录之后
模板内容
{{date.DATA}}
{{remark.DATA}}
{{city.DATA}}的天气:{{weather.DATA}}
最低气温:{{low.DATA}}度
最高气温:{{high.DATA}}度
今天是我们恋爱的第{{loveDays.DATA}}天
距离宝宝的生日还有{{birthdays.DATA}}天{{rainbow.DATA}}
百度地图开放平台
百度地图开放平台:登录百度帐号
天气服务接口文档:webapi | 百度地图API SDK
创建应用(选择服务端):登录百度帐号
注意:在 src/main/resources/application.properties 里面填写"应用AK"和"城市ID/行政代码",如湘潭行政代码是430300
彩虹屁平台
注册天行数据账号并申请接口:天行数据TianAPI - 开发者API数据平台
申请彩虹屁的接口,别选错
注意:在 src/main/resources/application.properties 里面填写"apiKey"
11.启动WeatherPushApplication
12.通过访问http://localhost:8080/test即为手动推送
每一个小时有个定时任务推送,可根据需求自己改
quartz/Cron/Crontab表达式在线生成工具-BeJSON.com
13.效果图
感谢各位观看!如果对你有帮助,点个赞把!
更多推荐
所有评论(0)