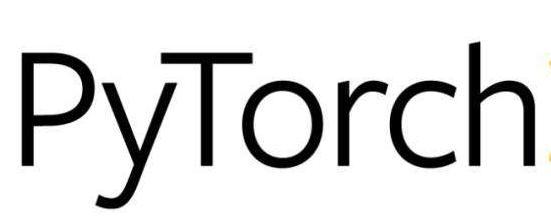
Pytorch几种修改Tensor尺寸(shape)的方法
Pytorch几种修改Tensor尺寸(shape)的方法
·
2. tensor.view()
根据tensor.view方法,可以调整tensor的形状,但必须保证调整前后元素总数一致(例如原tensor.shape = (1, 6)元素总数为1*6=6,调整好之后tensor.view(2, 3) 2*3 = 6保持前后总数一致。view不会改变自身数据,返回的新的tensor与源tensor共享内存,即更改其中一个,另外一个也会跟着改变。
#input
import torch
a = torch.arange(0, 6)
b = a.view(2, 3)
print(b)
#output
# tensor([[0, 1, 2],
[3, 4, 5]])
2.tensor.unqueeze()和tensor.squeeze()
tensor.unsqueeze 和 tensor.squeeze分别用于增加或减少tensor的某一维度。
import torch
a = torch.arange(0, 6)
b = a.view(2, 3)
#input1
b.unsqueeze(1)# 在第1维(下标从0开始)上增加“1”
print(b)
#output1
#tensor([[0, 1, 2],
[3, 4, 5]])
#input2
b.unsqueeze(-2) #-2表示倒数第二个维度
print(b)
#output2
#tensor([[0, 1, 2],
[3, 4, 5]])
#input3
c = b.view(1, 1, 1, 2, 3)
c.unsqueeze(0) #压缩第0维的“1”
print(c)
#output3
#tensor([[[[[0, 1, 2],
[3, 4, 5]]]]])
#input4
c.squeeze() #把所有维度为“1”的压缩
print(c)
#output4
tensor([[[[[0, 1, 2],
[3, 4, 5]]]]])
3.tensor.resize_()
tensor.resize_()功能和view都是修改shape的形状,但与view不同,它可以修改tensor的尺寸。如果新尺寸超过了原尺寸,会自动分配新的内存空间;如果新尺寸小于原尺寸,则之前的数据依旧会保存。(注意此方法对于使用求梯度require_grad的变量无法进行修改,会报错!)
import torch
a = torch.arange(0, 6)
b = a.view(2, 3)
b.resize_(1, 3)
print(b)
#output
#tensor([[0, 1, 2]])
更多推荐
所有评论(0)