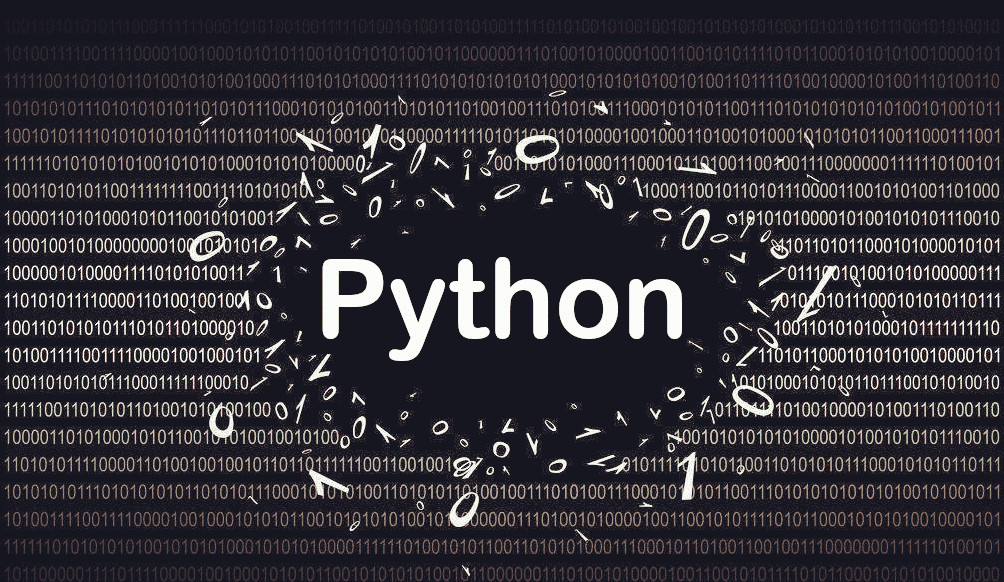
Python标准库——turtle库
目录一、概述二、函数1.窗体函数2.画笔状态函数3.画笔运动函数一、概述turtle(海龟)是Python重要的标准库之一,它能够进行基本的图形绘制,其概念诞生于1969年。turtle是最有价值的程序设计入门实践库,它是程序设计入门层面最常用的基本绘图库。turtle的绘图原理:有一只海龟处于画布正中心,由程序控制在画布上游走;海龟走过的轨迹形成了绘制的图形海龟由程序控制,可改变其大小,颜色等使
·
一、概述
turtle(海龟)是Python重要的标准库之一,它能够进行基本的图形绘制,其概念诞生于1969年。turtle是最有价值的程序设计入门实践库,它是程序设计入门层面最常用的基本绘图库。
turtle的绘图原理:
- 有一只海龟处于画布正中心,由程序控制在画布上游走;
- 海龟走过的轨迹形成了绘制的图形
- 海龟由程序控制,可改变其大小,颜色等
使用 import 保留字对 turtle 库的引用有以下三种方式:
- import turtle
import turtle
turtle.circle(200)
- from turtle import *
from turtle import *
circle(200)
- import turtle as t
import turtle as t
t.circle(200)
二、函数
1.窗体函数
turtle.setup()函数与窗体有关,定义如下:
turtle.setup(width,height,startx,starty)
作用:设置主窗体的大小和位置
参数:
width:窗口宽度。如果值是整数,表示像素值;如果值是小数,表示窗口宽度与屏幕的比例
height:窗口高度。如果值是整数,表示像素值;如果值是小数,表示窗口高度与屏幕的比例
startx:窗口左侧与屏幕右侧的像素距离。如果值是None,窗口位于屏幕水平正中央。
starty:窗口顶部与屏幕顶部的像素距离。如果值是None,窗口位于屏幕垂直正中央。
2.画笔状态函数
函数 | 描述 |
---|---|
pendown( ) | 放下画笔 |
penup( ) | 提起画笔 |
pensize(width) | 设置画笔线条的粗细为指定大小 |
pencolor( ) | 设置画笔的颜色 |
color( a,b) | 同时设置画笔和填充颜色 |
begin_fill( ) | 填充图形前,调用该方法 |
end_fill( ) | 填充图形结束 |
filling( ) | 返回填充的状态,True为填充。False为未填充 |
clear( ) | 清空当前窗口,但不改变当前画笔的位置 |
reset( ) | 清空当前窗口,并重置位置等状态为默认值 |
screensize( ) | 设置画布窗口的宽度、高度和背景颜色 |
hideturtle( ) | 隐藏画笔的turtle形状 |
showturtle( ) | 显示画笔的turtle形状 |
isvisible( ) | 如果turtle可见,则返回True |
write(str,font=None) | 输出font字体的字符串 |
注:pencolor(color)的color可以有三种形式:
- 颜色字符串:turtle.color(“purple”)
- RGB的小数值:turtle.color(0.63,0.13,0.94)
- RGB的元组值:turtle.color((0.63,0.13,0.94))
3.画笔运动函数
函数 | 描述 |
---|---|
fd(distance) | 沿着当前方向前进指定距离 |
bk(distance) | 沿着当前相反方向后退指定距离 |
right(angle) | 向右旋转angle角度 |
left(angle) | 向左旋转angle角度 |
goto(x,y) | 移动到绝对坐标(x,y)处 |
setx(x) | 修改画笔的横坐标到x,纵坐标不变 |
sety(y) | 修改画笔的纵坐标到y,横坐标不变 |
seth(angle) | 设置当前朝向为angle角度 |
home( ) | 设置当前画笔位置为原点,朝向东 |
circle(radius,e) | 绘制一个指定半径r和角度e的圆或弧形 |
dot(r,color) | 绘制一个指定半径r和颜色color的圆点 |
undo( ) | 撤销画笔的最后一步动作 |
speed( ) | 设置画笔的绘制速度,参数为0~10之间 |
三、实例
python蟒蛇绘制:
import turtle
turtle.setup(650,350,200,200)
turtle.penup()
turtle.fd(-250)
turtle.pendown()
turtle.pensize(25)
turtle.pencolor("tomato")
turtle.seth(-40)
for i in range(4):
turtle.circle(40,80)
turtle.circle(-40,80)
turtle.circle(40,80/2)
turtle.fd(40)
turtle.circle(16,180)
turtle.fd(40*2/3)
turtle.done()
turtle绘图汇总:
粉红爱心
'''
from turtle import *
color('red', 'pink')
begin_fill()
left(135)
fd(100)
right(180)
circle(50, -180)
left(90)
circle(50, -180)
right(180)
fd(100)
end_fill()
hideturtle()
done() '''
红色五角星
'''from turtle import *
setup(400,400)
penup()
goto(-100,50)
pendown()
color("red")
begin_fill()
for i in range(5):
forward(200)
right(144)
end_fill()
hideturtle()
done()'''
正方形螺旋线
'''
import turtle
n = 10
for i in range(1,10,1):
for j in [90,180,-90,0]:
turtle.seth (j)
turtle.fd(n)
n += 5 '''
城市剪影
'''
import turtle
turtle.setup(800,300)
turtle.penup()
turtle.fd(-350)
turtle.pendown()
def DrawLine(size):
for angle in [0,90,-90,-90,90]:
turtle.left(angle)
turtle.fd(size)
for i in [20,30,40,50,40,30,20]:
DrawLine(i)
turtle.hideturtle()
turtle.done() '''
四阶同心圆
'''
import turtle as t
def DrawCctCircle(n):
t.penup()
t.goto(0,-n)
t.pendown()
t.circle(n,360)
for i in range(20,100,20):
DrawCctCircle(i)
t.hideturtle()
t.done() '''
钢琴键
'''
import turtle as t
t.setup(500,300)
t.penup()
t.goto(-180,-50) #将画笔移动到绝对位置(–180,–50)处
t.pendown() #画笔落下
def Drawrect():
t.fd(40)
t.left(90)
t.fd(120)
t.left(90)
t.fd(40)
t.left(90)
t.fd(120)
t.penup()
t.left(90)
t.fd(42)
t.pendown()
for i in range(7):
Drawrect()
t.penup()
t.goto(-150,0)
t.pendown
def DrawRectBlack():
t.color('black')
t.begin_fill()
t.fd(30)
t.left(90)
t.fd(70)
t.left(90)
t.fd(30)
t.left(90)
t.fd(70)
t.end_fill()
t.penup()
t.left(90)
t.fd(40)
t.pendown()
DrawRectBlack()
DrawRectBlack()
t.penup()
t.fd(48)
t.pendown()
DrawRectBlack()
DrawRectBlack()
DrawRectBlack()
t.hideturtle()
t.done() '''
叠加等边三角形
'''
import turtle
turtle.pensize(2) # 设置画笔宽度为2像素
turtle.color('red')
turtle.fd(160) # 向小海龟当前行进方向前进160像素
turtle.seth(120)
turtle.fd(160)
turtle.seth(-120)
turtle.fd(160)
turtle.penup()
turtle.seth(0)
turtle.fd(80)
turtle.pendown()
turtle.seth(60)
turtle.fd(80)
turtle.seth(180)
turtle.fd(80)
turtle.seth(-60)
turtle.fd(80)
turtle.hideturtle()
turtle.done() '''
金色三角形
'''
import turtle as t
t.colormode(255)
t.color(255,215,0) #设置颜色取值为金色(255,215,0)
t.begin_fill()
for x in range(0,8): #绘制8条线
t.forward(200)
t.left(225)
t.end_fill()
t.hideturtle()
t.done() '''
五种多边形
'''
from turtle import *
for i in range(5):
penup() #画笔抬起
goto(-200+100*i,-50)
pendown()
circle(40,steps=3+i) #画某个形状
done() '''
绘制树形图
'''
import turtle as t
def tree(length,level): #树的层次
if level <= 0:
return
t.forward(length) #前进方向画 length距离
t.left(45)
tree(0.6*length,level-1)
t.right(90)
tree(0.6*length,level-1)
t.left(45)
t.backward(length)
return
t.pensize(3)
t.color('green')
t.left(90)
tree(100,6) '''
花形图
'''
import turtle
for i in range(4):
turtle.right(90)
turtle.circle(30,180) '''
星形图
'''
import turtle
for i in range(4):
turtle.circle(-90,90)
turtle.right(180)
'''
类斯诺克图
'''
import turtle
#绘制边长为20的圆形
def drawCircle():
turtle.pendown()
turtle.circle(20)
turtle.penup()
turtle.fd(40)
#绘制n层的如题干中描述的图形
def drawRowCircle(n):
for j in range(n,1,-1):
for i in range(j):
drawCircle()
turtle.fd(-j*40-20)
turtle.right(90)
turtle.fd(40)
turtle.left(90)
turtle.fd(40)
drawCircle()
#调用函数绘制图形
drawRowCircle(5)
turtle.hideturtle()
turtle.done()
'''
领结
'''
from turtle import *
pensize(6)
penup()
goto(-100,-50)
pendown()
fillcolor('red')
begin_fill()
goto(-100,50)
goto(100,-50)
goto(100,50)
goto(-100,-50)
penup()
goto(-10,0)
pendown()
right(90)
circle(10,360)
end_fill()
hideturtle()
done()
'''
三菱
'''
import turtle
def Draw():
turtle.fillcolor("red")
turtle.begin_fill()
turtle.fd(100)
turtle.left(60)
turtle.fd(100)
turtle.left(120)
turtle.fd(100)
turtle.left(60)
turtle.fd(100)
turtle.end_fill()
for i in range(3):
Draw()
turtle.hideturtle()
turtle.done()
'''
螺旋状正方形
'''
import turtle
d = 0
k = 1
for j in range(10):
for i in range(4):
turtle.seth(d)
d += 91
turtle.fd(k)
k += 4
turtle.done()
'''
嵌套五边形
'''
import turtle
edge=5
d = 0
k = 1
for j in range(10):
for i in range(edge):
turtle.fd(k)
d += 360/edge
turtle.seth(d)
k += 3
turtle.done() '''
八卦图
'''
import turtle as t
t.circle(100)
t.circle(50,180)
t.circle(-50,180)
t.penup()
t.goto(0,140)
t.pendown()
t.circle(10)
t.penup()
t.goto(0,40)
t.pendown()
t.circle(10)
t.done()
'''
同心圆
import turtle as t
def DrawCctCircle(n):
t.penup()
t.goto(0,-n)
t.pendown()
t.circle(n)
for i in range(20,80,20):
DrawCctCircle(i)
t.done()
更多推荐
所有评论(0)