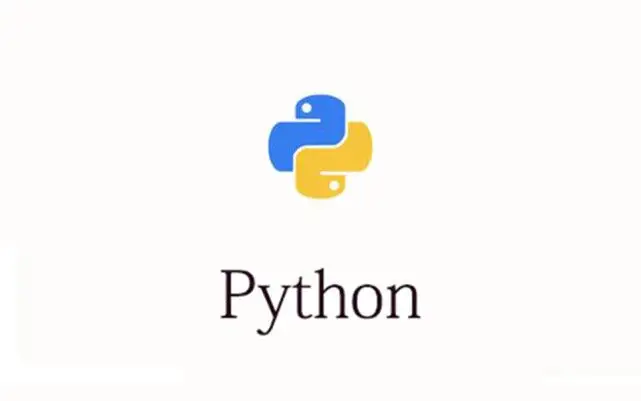
Python实验
Python实验
实验1 Python编程基础
(1)在交互式环境中打印“Hello world”字符串。记录操作过程。
略
(2)创建脚本helloworld.py,在命令符提示环境中执行程序,打印“Hello world”字符串。记录操作过程。
略
(3)使用pip命令查看当前已安装的所有模块。
pip list
(4)编写一个猜年龄的小游戏,如果输入的年龄正确输出“Yes,it is right!”否则输出“No, you are wrong!”
year = 20
guess_age = int(input("请输入你猜的年龄:"))
if year == guess_age:
print("Yes,it is right!")
else:
print("No,you are wrong!")
(5)编写程序,输入<人名 1>和<人名 2>,在屏幕上显示如下的新年贺卡
【源程序】
###################################
# <人名 1> 新年贺卡 <人名 2>
# python0101.py
# 2015
###################################
name1 = input("Please enter a value for name1:")
name2 = input("Please enter a value for name2:")
print(f'''
###################################
# {name1} 新年贺卡 {name2}
# python0101.py
# 2015
###################################
''')
(6)编写程序,输入球的半径,计算球的表面积和体积,半径为实数,用π,结果输出为浮点数,共10位其中2位有效数字。
import math
r = float(input("Please enter a valur for radius:"))
surface = '%.10f' % round(4 * math.pi * r * r, 2)
volume = '%.10f' % round(4 / 3 * math.pi * (r ** 3), 2)
print(f"The surface area of sphere is {surface}")
print(f'The volume of sphere if {volume}')
(7) 用id()函数输出变量地址的示例程序:
str1 = "这是一个变量";
print("变量str1的值是:"+str1);
print("变量str1的地址是:%d" %(id(str1)));
str2 = str1;
print("变量str2的值是:"+str2);
print("变量str2的地址是:%d" %(id(str2)));
str1 = "这是另一个变量";
print("变量str1的值是:"+str1);
print("变量str1的地址是:%d" %(id(str1)));
print("变量str2的值是:"+str2);
print("变量str2的地址是:%d" %(id(str2)));
str1 = "这是一个变量"
print("变量str1的值是:" + str1)
print("变量str1的地址是:%d" % (id(str1)))
str2 = str1
print("变量str2的值是:" + str2)
print("变量str2的地址是:%d" % (id(str2)))
str1 = "这是另一个变量"
print("变量str1的值是:" + str1)
print("变量str1的地址是:%d" % (id(str1)))
print("变量str2的值是:" + str2)
print("变量str2的地址是:%d" % (id(str2)))
(8)参照下面的步骤练习使用运算符
x =3
x += 3
print(x)
x -= 3
print(x)
x *= 3
print(x)
x /= 3
print(x)
x = 3
x += 3
print(x)
x -= 3
print(x)
x *= 3
print(x)
x /= 3
print(x)
实验2 Python序列、字符串处理
(1)有四个数字:1、2、3、4,能组成多少个互不相同且无重复数字的三位数?各是多少?
aList = [1, 2, 3, 4]
count = 0
for i in aList:
for j in aList:
for k in aList:
if (i != j) and (i != k) and (j != k):
count += 1
print(f"{i}{j}{k}", end=" ")
print("")
print(f"The count is {count}")
(2)输入某年某月某日,判断这一天是这一年的第几天?
year = int(input('请输入年份:'))
mouth = int(input('请输入月份:'))
day = int(input('请输入日:'))
mouths = [0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if year % 400 == 0 or year % 4 == 0:
mouths[3] = mouths[3] + 1
if 0 < mouth <= 12:
days = 0
for item in range(mouth):
sum = mouths[item]
days = days + sum
result = days + day
print(f'今天是今年的第{result}天')
else:
print('输入日期超出范围')
(3)输入三个整数x,y,z,请把这三个数由小到大输出。
aList = [int(input("Please input a value for x:")), int(input("Please input a value for y:")),
int(input("Please input a value for z:"))]
print(sorted(aList))
(4)输出9*9乘法口诀表。
print('\n'.join([' '.join([f"{j}x{i}={i * j}" for j in range(1, i + 1)]) for i in range(1, 10)]))
(5)判断101-200之间有多少个素数,并输出所有素数。
result = []
for i in range(101, 200):
for j in range(2, i - 1):
if i % j == 0:
break
else:
result.append(i)
print(result)
print(f"素数一共有{len(result)}个")
(6)输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。
str = input("请输入一行字符:")
count_alpha = 0
count_space = 0
count_digit = 0
count_other = 0
for i in str:
if i.isalpha():
count_alpha += 1
if i.isspace():
count_space += 1
if i.isdigit():
count_digit += 1
else:
count_other += 1
print(f"{str}中的英文字母个数为:{count_alpha}")
print(f"{str}中的空格个数为:{count_space}")
print(f"{str}中的数字个数为:{count_digit}")
print(f"{str}中的其他字符个数为:{count_other}")
(7)求1!+2!+3!+...+20!的和。
aList = []
for i in range(1, 21):
num = 1
for j in range(1, i + 1):
num *= j
aList.append(num)
print(sum(aList))
(8)设计一个字典,并编写程序,用户输入内容作为键,然后输出字典中对应的值,如果用户输入的键不存在,则输出“您输入的键不存在!”
d = {'sjn': '1', 'daning': 2, 'jit': 3, 'nanjing': 4}
a = input("请输入键:")
print(d.get(a, "您输入的键不存在!"))
(9)编写程序,生成包含20个随机数的列表,然后将前10个元素升序排列,后10个元素降序排列,并输出结果。
from random import choices
data = choices(range(100), k=20)
print(data)
data[:10] = sorted(data[:10])
data[10:] = sorted(data[10:], reverse=True)
print(data)
(10)字符串 a = "aAsmr3idd4bgs7Dlsf9eAF"
a.请将 a 字符串的数字取出,并输出成一个新的字符串。
b.请统计 a 字符串出现的每个字母的出现次数(忽略大小写,a 与 A 是同一个字母),并输出成一个字典。 例 {'a':3,'b':1}
c.请去除 a 字符串多次出现的字母,仅留最先出现的一个,大小写不敏感。例
'aAsmr3idd4bgs7Dlsf9eAF',经过去除后,输出 'asmr3id4bg7lf9e'
d.按 a 字符串中字符出现频率从高到低输出到列表,如果次数相同则按字母顺序排列。
a = "aAsmr3idd4bgs7Dlsf9eAF"
str_digit = ''
for i in a:
if i.isdigit():
str_digit += i
print(str_digit)
lower_a = a.lower()
c = {key: lower_a.count(key) for key in lower_a if key.isalpha()}
print(c)
result = []
for i in a:
if i not in result:
if ('A' <= i <= 'Z') and (i.lower() not in result):
result.append(i)
elif ('a' <= i <= 'z') and (i.upper() not in result):
result.append(i)
elif not i.isalpha():
result.append(i)
print("".join(result))
(11)回文是一种“从前向后读”和“从后向前读”都相同的字符串。如“rotor”是一个回文字符串。定义一个函数,判断一个字符串是否为回文字符串,并测试函数的正确性。
def isPalindrome(str):
rever_str = str[::-1]
if rever_str == str:
print(f"{str}是回文字符串!")
else:
print(f"{str}不是回文字符串!")
isPalindrome("1632")
isPalindrome("16322361")
实验3 函数及Python类的定义与使用
(1)定义一个函数,实验给定任意字符串,查找其中每个字符的最后一次出现,并按每个字符最后一次出现的先后顺序依次存入列表。例如对于字符串'abcda'的处理结果为['b', 'c', 'd', 'a'],而字符串'abcbda'的处理结果为['c', 'b', 'd', 'a']。测试函数的正确性。
aList = list()
str1 = input("Please enter a value for str1:")
for i in str1:
if aList.__contains__(i):
aList.remove(i)
aList.append(i)
else:
aList.append(i)
print(aList)
(2)编写函数,使用非递归方法对整数进行因数分解。
def g(h):
list1 = []
while h > 1:
for i in range(h-1):
k = i + 2
if h % k == 0:
list1.append(k)
h = h//k
break
print(list1)
g(12)
(3)编写总的“车”类(VehicleClass),其中描述车名、车轮数,以及机动车和非机动车变量, 以及开车方法;编写继承“车”类的公共汽车类(BusClass)和自行车类(BicycleClass)。
class VehicleClass:
def __int__(self, name, wheel, kind):
self.name = name
self.wheel = wheel
self.kind = kind
def drive_car(self):
print(f"我正在驾驶{self.name}汽车")
class BusClass(VehicleClass):
pass
class BicycleClass(VehicleClass):
pass
(4)自定义集合。模拟Python内置集合类型,实现元素添加、删除以及并集、交集、对称差集等基本运算。
set = []
def add():
num = input("input to add :")
for item in set:
if num.__eq__(item):
print("%s already exists \n" % num)
return
set.append(num)
print("%s has been add \n" % num)
def remove(item):
set.remove(item)
print("%s has remove \n" % item)
def intersection(other):
result = []
for item in other:
if set.__contains__(item):
result.append(item)
print("The intersection is:" + str(result))
def union(other):
result = set.copy()
for item in other:
if not result.__contains__(item):
result.append(item)
print("The union is:" + str(result))
def symmetric_difference(other):
intersection = []
for item in other:
if set.__contains__(item):
intersection.append(item)
union = set.copy()
for item in other:
if not union.__contains__(item):
union.append(item)
result = union.copy()
for item in union:
if intersection.__contains__(item):
result.remove(item)
print("The symmetric difference is:" + str(result))
def show_set():
print("The set is: " + str(set))
add()
add()
add()
add()
remove(input("Please enter the element to remove: "))
show_set()
other = ['1', '3', '5', '7']
intersection(other)
union(other)
symmetric_difference(other)
(5)自定义栈,实现基本的入栈、出栈操作。
stack = []
def push_stack():
num = input("input to push :")
stack.append(num)
print("%s has been push " % num)
def pop_stack():
print("%s has out" % stack.pop())
def show_stack():
print(stack)
def show_menu():
commands = {'0': push_stack,
'1': pop_stack,
'2': show_stack,
}
prompt = """
(0):push stack
(1):pop stack
(2):show stack
(3):exit
input what U want(0/1/2/3):"""
while True:
choice = input(prompt).strip()[0]
if choice == 3:
break
if choice not in '0123':
print("Invalid input,Try again")
commands[choice]()
show_menu()
(6)设计点类,并为这个点类设计一个方法计算两点之间的距离。
import math
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def getDistance(self, other):
print(f"两点之间的距离为:{round(math.sqrt((self.x - other.x) ** 2 + (self.y - other.y) ** 2),2)}")
point1 = Point(1, 1)
point2 = Point(2, 2)
point1.getDistance(point2)
(7)设计长方形类,并用其成员函数计算两个给定的长方形的周长和面积。
class Rectangle:
def __init__(self, x, y):
self.x = x
self.y = y
def getArea(self):
print(f"长方形的面积为{self.x * self.y}")
def getGirth(self):
print(f"长方形的周长为{self.x * 2 + self.y * 2}")
rectangle1 = Rectangle(2, 3)
rectangle1.getArea()
rectangle1.getGirth()
rectangle2 = Rectangle(5, 6)
rectangle2.getArea()
rectangle2.getGirth()
(8)试编码实现简单的银行业务:处理简单帐户存取款、查询。编写银行帐户类BankAccount,包含数据成员:余额(balance)、利率(interest);操作方法:查询余额、存款、取款、查询利率、设置利率。创建BankAccount类的对象,并完成相应操作。
# 试编码实现简单的银行业务:处理简单帐户存取款、查询。
# 编写银行帐户类BankAccount,包含数据成员:余额(balance)、利率(interest);
# 操作方法:查询余额、存款、取款、查询利率、设置利率。创建BankAccount类的对象,并完成相应操作。
class BankAccount:
def __init__(self, name, deposit, interest):
self.name = name
self.deposit = deposit
self.interest = interest
print(f"尊贵的{self.name}用户,您好!您于我行开户成功!当前余额为{self.deposit},利率为{self.interest}/年!")
print("-------------------------------------------------------------------")
def queryBalance(self):
print(f"尊贵的{self.name}用户,您好!您当前的账户余额为{self.deposit}")
def saveMoney(self, money):
self.deposit += money
print(f"尊贵的{self.name}用户,您好!当前您正在向我行存入{money}元钱...")
print(f"当前账上余额为{self.deposit},欢迎您的下次光临!")
def drawMoney(self, money):
print(f"尊贵的{self.name}用户,您好!当前您正在从我行取走{money}元钱...")
if self.deposit < money:
print("抱歉,您账户余额不足")
else:
self.deposit -= money
print(f"操作成功!当前账上余额为{self.deposit},欢迎您的下次光临!")
def queryInterest(self):
print(f"当前我行利率为{self.interest}/年!")
def setInterest(self, interest):
self.interest = interest
print(f"当前我行利率调整为{self.interest}/年!")
def userOperation(self):
while True:
print('''
0 -------> 查询余额
1 -------> 存款
2 -------> 取款
3 -------> 查询利率
4 -------> 设置利率
5 -------> 退出系统
''')
flag = int(input("请输入对应的数字:"))
print("-------------------------------------------------------------------")
if flag == 0:
self.queryBalance()
elif flag == 1:
self.saveMoney(int(input("请输入您的存钱数:")))
elif flag == 2:
self.drawMoney(int(input("请输入您的取款数:")))
elif flag == 3:
self.queryInterest()
elif flag == 4:
self.setInterest(float(input("请输入您要设置的利率:")))
else:
print("退出成功!")
exit(0)
myBank = BankAccount("大柠", 10000, 1.5)
myBank.userOperation()
实验4 Python综合应用
(1)假设有一个英文文本文件,编写程序读取其内容,并将其中的大写字母变为小写字母,小写字母变为大写字母。
with open("demo.txt", "r") as f:
data = f.read().swapcase()
print(data)
(2)读取文本文件data.txt中所有整数,将其按升序排序后再写入文本文件。
with open('data.txt', 'r') as fp:
data = fp.readlines()
data = [int(line.strip()) for line in data]
data.sort()
data = [str(i) + '\n' for i in data]
with open('data.txt', 'w') as fp:
fp.writelines(data)
(3)编写程序,将包含学生成绩的字典保存为二进制文件,然后再读取内容并显示。
import pickle
stu_dict = {"数据结构": 98,
"离散数学": 95,
"计算机组成原理": 93,
"Java": 94}
s = pickle.dumps(stu_dict)
with open("./dict.txt", "wb") as f:
f.write(s)
f.close()
with open("./dict.txt", "rb") as f:
a_dict = f.read()
f.close()
a_dict = pickle.loads(a_dict)
print(a_dict)
(4)编写程序,用户输入一个目录和一个文件名,搜索该目录及其子目录中是否存在该文件。
import os
catalogue = input("请输入目录:")
filename = input("请输入文件名:")
flag = True
# os.walk返回一个元组,包括3个元素:
# 所有路径名、所有目录列表与文件列表
tuple_dirs = os.walk(catalogue)
for root, dirs, files in tuple_dirs: # 遍历该元组的目录和文件信息
if filename in files:
print(f"{catalogue} 目录下有文件 {filename}")
flag = False
break
if flag:
print(f"{catalogue} 目录下没有文件 {filename}")
(5)不使用拷贝的方式计算((A+B)*(-A/2)) 。
import numpy as np
A = np.ones(3) * 1
B = np.ones(3) * 2
print(np.multiply(np.add(A, B, out=B), np.negative(np.divide(A, 2, out=A), out=A),
out=A))
(6)生成一个5*5的矩阵,其中每一行的值为0到4
import numpy as np
Z = np.mod(np.arange(25), 5).reshape(5, 5)
print(Z)
(7)生成一个包含10个元素的向量,其其取值范围为[0,1]
import numpy as np
print(np.random.random(10))
(8)给定一个4维向量,求倒数第一维、倒数第二维的和。
import numpy
arr = numpy.random.randint(2, size=(3, 3, 3, 3))
print('原始数据:')
print(arr)
ax1 = numpy.sum(arr, axis=2)
print('倒数第二维的和:')
print(ax1)
ax2 = numpy.sum(arr, axis=3)
print('倒数第一维的和:')
print(ax2)
print('最后两维的和:')
print(ax1 + ax2)
(9)画出以下函数,其取值范围为[0,20].需要增加坐标标签,图片标题等。
from matplotlib import pyplot as plt
import numpy as np
import math
x = list(np.arange(0, 20, 0.1))
y = []
for i in range(len(x)):
y.append(math.sin(x[i] - 2) ** 2 * math.e ** (0 - x[i] ** 2))
plt.plot(x, y)
plt.xlabel('x-axis', fontsize=14)
plt.ylabel('y-axis', fontsize=14)
plt.title('f(x) = sin²(x-2)e^(-x²)')
plt.show()
(10)画出正弦曲线, 需要增加坐标标签,图片标题等。
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-20, 20, 100)
y = np.sin(x)
plt.plot(x, y)
plt.xlabel('x-axis', fontsize=14)
plt.ylabel('y-axis', fontsize=14)
plt.title('f(x) = sinx')
plt.show()
(11)根据企业成本及利润多年的变化情况,分析下一年的企业利润会是多少。
cost = [400,450,486,500,510,525,540,549,558,590,610,640,680,750,900]
profit =[80,89,92,102,121,160,180,189,199,203,247,250,259,289,356]
import matplotlib.pyplot as plt
import numpy as np
from sklearn import linear_model
X = [[400], [450], [486], [500], [510], [525], [540], [549], [558], [590], [610], [640], [680], [750], [900]]
Y = [[80], [89], [92], [102], [121], [160], [180], [189], [199], [203], [247], [250], [259], [289], [356]]
clf = linear_model.LinearRegression()
clf.fit(X, Y)
X2 = [[400], [750], [950]]
Y2 = clf.predict(X2)
res = clf.predict(np.array([1200]).reshape(-1, 1))
print('预测成本1200元的利润:$%.1f' % res)
# plt.plot(X, Y, 'ks')
plt.scatter(X, Y)
plt.plot(X2, Y2, 'g-')
plt.show()
更多推荐
所有评论(0)