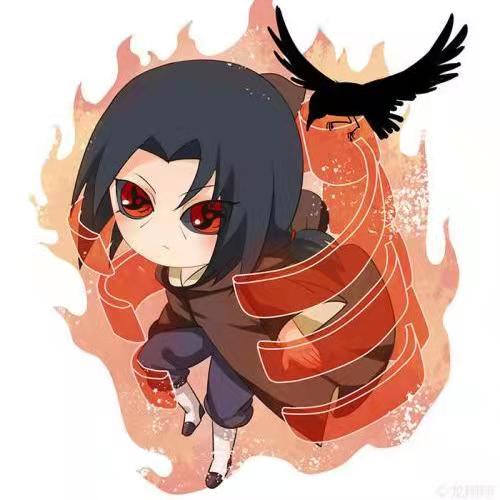
基于dlib进行人脸检测
1、dlib的基本概念1. Dlib是一个深度学习开源工具,基于C++开发,也支持Python开发接口。2. 由于Dlib对于人脸特征提取支持很好,有很多训练好的人脸特征提取模型供开发者使用,所以Dlib人脸识别开发很适合做人脸项目开发。3. HOG 方向梯度直方图(Histogram of Oriented Gradient)(1)HOG是一种特征描述子,通常用于从图像数据中提取特征。它广泛用于
·
1、dlib的基本概念
1. Dlib是一个深度学习开源工具,基于C++开发,也支持Python开发接口。
2. 由于Dlib对于人脸特征提取支持很好,有很多训练好的人脸特征提取模型供开发者使用,所以Dlib人脸识别开发很适合做人脸项目开发。
3. HOG 方向梯度直方图(Histogram of Oriented Gradient)
(1)HOG是一种特征描述子,通常用于从图像数据中提取特征。它广泛用于计算机视觉任务的物体检测。
(2)特征描述子的作用:它是图像的简化表示,仅包含有关图像的最重要信息。
2、dlib库安装的方法
1.打开pycharm
2.File—>settings—>Project Interpreter
选择你安装的python3.6.1版本作为解释器
3.点击右侧+号,在搜素框里输入dlib,然后在右下角的Specify version前面的方框里面打钩,打钩之后选择版本19.6.1(和刚才安装的版本一样)
4.点击左下角的Install Package进行安装,如果一次不行就多安装几次
(再次提醒:版本一定要选刚才安装的那个)
3、dlib的运用
1、识别图像
# 1 导入库
import cv2
import dlib
import numpy as np
import matplotlib.pyplot as plt
# 2 方法:显示图片
def show_image(image, title):
img_RGB = image[:, :, ::-1] # BGR to RGB
plt.title(title)
plt.imshow(img_RGB)
plt.axis("off")
# 3 方法:绘制人脸矩形框
def plot_rectangle(image, faces):
for face in faces:
cv2.rectangle(image, (face.left(), face.top()), (face.right(), face.bottom()), (255,0,0), 4)
# 画矩形,两个坐标
return image
def main():
# 4 读取一张图片
img = cv2.imread("21.jpg")
# 5 灰度转换
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 6 调用dlib库中的检测器
detector = dlib.get_frontal_face_detector()
dets_result = detector(gray, 1) # 1 :代表将图片放大一倍
# 7 给检测出的人脸绘制矩形框
img_result = plot_rectangle(img.copy(), dets_result)
# 8 创建画布
plt.figure(figsize=(9, 6))
plt.suptitle("face detection with dlib", fontsize=14, fontweight="bold")
# 9 显示最终的检测效果
show_image(img_result, "face detection")
plt.show()
if __name__ == '__main__':
main()
2、电脑摄像头识别
# 1 导入库
import cv2
import dlib
# 2 方法:绘制人脸矩形框
def plot_rectangle(image, faces):
for face in faces:
cv2.rectangle(image, (face.left(), face.top()), (face.right(), face.bottom()), (255,0,0), 4)
return image
def main():
# 3 打开摄像头,读取视频
capture = cv2.VideoCapture(0)
# 4 判断摄像头是否正常工作
if capture.isOpened() is False:
print("Camera Error !")
# 5 摄像头正常打开:循环读取每一帧
while True:
ret, frame = capture.read()
if ret:
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # BGR to GRAY
# 6 调用dlib库中的检测器
detector = dlib.get_frontal_face_detector()
det_result = detector(gray, 1) # 对图片进行检测,返回一个结果
# 7 绘制检测结果
dets_image = plot_rectangle(frame, det_result) # 原始图片和检测结果 结果绘制到图片上去。
# 8 实时显示最终的检测效果
cv2.imshow("face detection with dlib", dets_image)
# 9 按键"ESC",退出,关闭摄像头
if cv2.waitKey(1) == 27:
break
# 10 释放所有的资源
capture.release()
cv2.destroyAllWindows()
if __name__ == '__main__':
main()
更多推荐
所有评论(0)